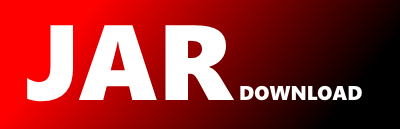
com.pulumi.azurenative.media.kotlin.inputs.RectangleArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.media.kotlin.inputs
import com.pulumi.azurenative.media.inputs.RectangleArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Describes the properties of a rectangular window applied to the input media before processing it.
* @property height The height of the rectangular region in pixels. This can be absolute pixel value (e.g 100), or relative to the size of the video (For example, 50%).
* @property left The number of pixels from the left-margin. This can be absolute pixel value (e.g 100), or relative to the size of the video (For example, 50%).
* @property top The number of pixels from the top-margin. This can be absolute pixel value (e.g 100), or relative to the size of the video (For example, 50%).
* @property width The width of the rectangular region in pixels. This can be absolute pixel value (e.g 100), or relative to the size of the video (For example, 50%).
*/
public data class RectangleArgs(
public val height: Output? = null,
public val left: Output? = null,
public val top: Output? = null,
public val width: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.media.inputs.RectangleArgs =
com.pulumi.azurenative.media.inputs.RectangleArgs.builder()
.height(height?.applyValue({ args0 -> args0 }))
.left(left?.applyValue({ args0 -> args0 }))
.top(top?.applyValue({ args0 -> args0 }))
.width(width?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [RectangleArgs].
*/
@PulumiTagMarker
public class RectangleArgsBuilder internal constructor() {
private var height: Output? = null
private var left: Output? = null
private var top: Output? = null
private var width: Output? = null
/**
* @param value The height of the rectangular region in pixels. This can be absolute pixel value (e.g 100), or relative to the size of the video (For example, 50%).
*/
@JvmName("rqdcvsjcgtcfuuus")
public suspend fun height(`value`: Output) {
this.height = value
}
/**
* @param value The number of pixels from the left-margin. This can be absolute pixel value (e.g 100), or relative to the size of the video (For example, 50%).
*/
@JvmName("ksyanbvqihckpkqi")
public suspend fun left(`value`: Output) {
this.left = value
}
/**
* @param value The number of pixels from the top-margin. This can be absolute pixel value (e.g 100), or relative to the size of the video (For example, 50%).
*/
@JvmName("rewvooyahajhxdvm")
public suspend fun top(`value`: Output) {
this.top = value
}
/**
* @param value The width of the rectangular region in pixels. This can be absolute pixel value (e.g 100), or relative to the size of the video (For example, 50%).
*/
@JvmName("ruivomxkdthbqspu")
public suspend fun width(`value`: Output) {
this.width = value
}
/**
* @param value The height of the rectangular region in pixels. This can be absolute pixel value (e.g 100), or relative to the size of the video (For example, 50%).
*/
@JvmName("sgmgpafnnanwixad")
public suspend fun height(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.height = mapped
}
/**
* @param value The number of pixels from the left-margin. This can be absolute pixel value (e.g 100), or relative to the size of the video (For example, 50%).
*/
@JvmName("gayyotnbllltmxur")
public suspend fun left(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.left = mapped
}
/**
* @param value The number of pixels from the top-margin. This can be absolute pixel value (e.g 100), or relative to the size of the video (For example, 50%).
*/
@JvmName("dsjbwdhffxsdjlyf")
public suspend fun top(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.top = mapped
}
/**
* @param value The width of the rectangular region in pixels. This can be absolute pixel value (e.g 100), or relative to the size of the video (For example, 50%).
*/
@JvmName("edmkycndjecfxjet")
public suspend fun width(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.width = mapped
}
internal fun build(): RectangleArgs = RectangleArgs(
height = height,
left = left,
top = top,
width = width,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy