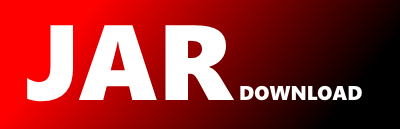
com.pulumi.azurenative.migrate.kotlin.AssessmentsOperationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.migrate.kotlin
import com.pulumi.azurenative.migrate.AssessmentsOperationArgs.builder
import com.pulumi.azurenative.migrate.kotlin.enums.AssessmentSizingCriterion
import com.pulumi.azurenative.migrate.kotlin.enums.AzureCurrency
import com.pulumi.azurenative.migrate.kotlin.enums.AzureDiskType
import com.pulumi.azurenative.migrate.kotlin.enums.AzureHybridUseBenefit
import com.pulumi.azurenative.migrate.kotlin.enums.AzureOfferCode
import com.pulumi.azurenative.migrate.kotlin.enums.AzurePricingTier
import com.pulumi.azurenative.migrate.kotlin.enums.AzureReservedInstance
import com.pulumi.azurenative.migrate.kotlin.enums.AzureStorageRedundancy
import com.pulumi.azurenative.migrate.kotlin.enums.AzureVmFamily
import com.pulumi.azurenative.migrate.kotlin.enums.Percentile
import com.pulumi.azurenative.migrate.kotlin.enums.ProvisioningState
import com.pulumi.azurenative.migrate.kotlin.enums.TimeRange
import com.pulumi.azurenative.migrate.kotlin.inputs.VmUptimeArgs
import com.pulumi.azurenative.migrate.kotlin.inputs.VmUptimeArgsBuilder
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Double
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Machine assessment resource.
* Azure REST API version: 2023-04-01-preview.
* Other available API versions: 2023-03-15.
* ## Example Usage
* ### AssessmentsOperations_Create_MaximumSet_Gen
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var assessmentsOperation = new AzureNative.Migrate.AssessmentsOperation("assessmentsOperation", new()
* {
* AssessmentName = "asm1",
* AzureDiskTypes = new[]
* {
* AzureNative.Migrate.AzureDiskType.Premium,
* AzureNative.Migrate.AzureDiskType.StandardSSD,
* },
* AzureHybridUseBenefit = AzureNative.Migrate.AzureHybridUseBenefit.Unknown,
* AzureLocation = "njxbwdtsxzhichsnk",
* AzureOfferCode = AzureNative.Migrate.AzureOfferCode.Unknown,
* AzurePricingTier = AzureNative.Migrate.AzurePricingTier.Standard,
* AzureStorageRedundancy = AzureNative.Migrate.AzureStorageRedundancy.Unknown,
* AzureVmFamilies = new[]
* {
* AzureNative.Migrate.AzureVmFamily.DSeries,
* AzureNative.Migrate.AzureVmFamily.Lsv2Series,
* AzureNative.Migrate.AzureVmFamily.MSeries,
* AzureNative.Migrate.AzureVmFamily.Mdsv2Series,
* AzureNative.Migrate.AzureVmFamily.Msv2Series,
* AzureNative.Migrate.AzureVmFamily.Mv2Series,
* },
* Currency = AzureNative.Migrate.AzureCurrency.Unknown,
* DiscountPercentage = 6,
* EaSubscriptionId = "kwsu",
* GroupName = "kuchatur-test",
* LinuxAzureHybridUseBenefit = AzureNative.Migrate.AzureHybridUseBenefit.Unknown,
* Percentile = AzureNative.Migrate.Percentile.Percentile50,
* PerfDataEndTime = "2023-09-26T09:36:48.491Z",
* PerfDataStartTime = "2023-09-26T09:36:48.491Z",
* ProjectName = "app18700project",
* ProvisioningState = AzureNative.Migrate.ProvisioningState.Succeeded,
* ReservedInstance = AzureNative.Migrate.AzureReservedInstance.None,
* ResourceGroupName = "ayagrawrg",
* ScalingFactor = 24,
* SizingCriterion = AzureNative.Migrate.AssessmentSizingCriterion.PerformanceBased,
* TimeRange = AzureNative.Migrate.TimeRange.Day,
* VmUptime = new AzureNative.Migrate.Inputs.VmUptimeArgs
* {
* DaysPerMonth = 13,
* HoursPerDay = 26,
* },
* });
* });
* ```
* ```go
* package main
* import (
* migrate "github.com/pulumi/pulumi-azure-native-sdk/migrate/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := migrate.NewAssessmentsOperation(ctx, "assessmentsOperation", &migrate.AssessmentsOperationArgs{
* AssessmentName: pulumi.String("asm1"),
* AzureDiskTypes: pulumi.StringArray{
* pulumi.String(migrate.AzureDiskTypePremium),
* pulumi.String(migrate.AzureDiskTypeStandardSSD),
* },
* AzureHybridUseBenefit: pulumi.String(migrate.AzureHybridUseBenefitUnknown),
* AzureLocation: pulumi.String("njxbwdtsxzhichsnk"),
* AzureOfferCode: pulumi.String(migrate.AzureOfferCodeUnknown),
* AzurePricingTier: pulumi.String(migrate.AzurePricingTierStandard),
* AzureStorageRedundancy: pulumi.String(migrate.AzureStorageRedundancyUnknown),
* AzureVmFamilies: pulumi.StringArray{
* pulumi.String(migrate.AzureVmFamilyDSeries),
* pulumi.String(migrate.AzureVmFamilyLsv2Series),
* pulumi.String(migrate.AzureVmFamilyMSeries),
* pulumi.String(migrate.AzureVmFamilyMdsv2Series),
* pulumi.String(migrate.AzureVmFamilyMsv2Series),
* pulumi.String(migrate.AzureVmFamilyMv2Series),
* },
* Currency: pulumi.String(migrate.AzureCurrencyUnknown),
* DiscountPercentage: pulumi.Float64(6),
* EaSubscriptionId: pulumi.String("kwsu"),
* GroupName: pulumi.String("kuchatur-test"),
* LinuxAzureHybridUseBenefit: pulumi.String(migrate.AzureHybridUseBenefitUnknown),
* Percentile: pulumi.String(migrate.PercentilePercentile50),
* PerfDataEndTime: pulumi.String("2023-09-26T09:36:48.491Z"),
* PerfDataStartTime: pulumi.String("2023-09-26T09:36:48.491Z"),
* ProjectName: pulumi.String("app18700project"),
* ProvisioningState: pulumi.String(migrate.ProvisioningStateSucceeded),
* ReservedInstance: pulumi.String(migrate.AzureReservedInstanceNone),
* ResourceGroupName: pulumi.String("ayagrawrg"),
* ScalingFactor: pulumi.Float64(24),
* SizingCriterion: pulumi.String(migrate.AssessmentSizingCriterionPerformanceBased),
* TimeRange: pulumi.String(migrate.TimeRangeDay),
* VmUptime: &migrate.VmUptimeArgs{
* DaysPerMonth: pulumi.Float64(13),
* HoursPerDay: pulumi.Float64(26),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.migrate.AssessmentsOperation;
* import com.pulumi.azurenative.migrate.AssessmentsOperationArgs;
* import com.pulumi.azurenative.migrate.inputs.VmUptimeArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var assessmentsOperation = new AssessmentsOperation("assessmentsOperation", AssessmentsOperationArgs.builder()
* .assessmentName("asm1")
* .azureDiskTypes(
* "Premium",
* "StandardSSD")
* .azureHybridUseBenefit("Unknown")
* .azureLocation("njxbwdtsxzhichsnk")
* .azureOfferCode("Unknown")
* .azurePricingTier("Standard")
* .azureStorageRedundancy("Unknown")
* .azureVmFamilies(
* "D_series",
* "Lsv2_series",
* "M_series",
* "Mdsv2_series",
* "Msv2_series",
* "Mv2_series")
* .currency("Unknown")
* .discountPercentage(6)
* .eaSubscriptionId("kwsu")
* .groupName("kuchatur-test")
* .linuxAzureHybridUseBenefit("Unknown")
* .percentile("Percentile50")
* .perfDataEndTime("2023-09-26T09:36:48.491Z")
* .perfDataStartTime("2023-09-26T09:36:48.491Z")
* .projectName("app18700project")
* .provisioningState("Succeeded")
* .reservedInstance("None")
* .resourceGroupName("ayagrawrg")
* .scalingFactor(24)
* .sizingCriterion("PerformanceBased")
* .timeRange("Day")
* .vmUptime(VmUptimeArgs.builder()
* .daysPerMonth(13)
* .hoursPerDay(26)
* .build())
* .build());
* }
* }
* ```
* ## Import
* An existing resource can be imported using its type token, name, and identifier, e.g.
* ```sh
* $ pulumi import azure-native:migrate:AssessmentsOperation asm1 /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Migrate/assessmentProjects/{projectName}/groups/{groupName}/assessments/{assessmentName}
* ```
* @property assessmentName Machine Assessment ARM name
* @property azureDiskTypes Gets or sets the azure storage type. Premium, Standard etc.
* @property azureHybridUseBenefit Gets or sets the user configurable setting to display the azure hybrid use
* benefit.
* @property azureLocation Azure Location or Azure region where to which the machines will be migrated.
* @property azureOfferCode Azure Offer Code.
* @property azurePricingTier Gets or sets Azure Pricing Tier - Free, Basic, etc.
* @property azureStorageRedundancy Gets or sets the Azure Storage Redundancy. Example: Locally Redundant Storage.
* @property azureVmFamilies Gets or sets the Azure VM families.
* @property currency Currency in which prices should be reported.
* @property discountPercentage Custom discount percentage.
* @property eaSubscriptionId Gets or sets enterprise agreement subscription id.
* @property groupName Group ARM name
* @property linuxAzureHybridUseBenefit Gets or sets the user configurable setting to display the linux azure hybrid use
* benefit.
* @property percentile Percentile of the utilization data values to be considered while assessing
* machines.
* @property perfDataEndTime Gets or sets the end time to consider performance data for assessment.
* @property perfDataStartTime Gets or sets the start time to consider performance data for assessment.
* @property projectName Assessment Project Name
* @property provisioningState The status of the last operation.
* @property reservedInstance Gets or sets the Azure Reserved Instance - 1-Year, 3-Year.
* @property resourceGroupName The name of the resource group. The name is case insensitive.
* @property scalingFactor Percentage of buffer that user wants on performance metrics when recommending
* Azure sizes.
* @property sizingCriterion Assessment sizing criterion.
* @property timeRange Time Range for which the historic utilization data should be considered for
* assessment.
* @property vmUptime Gets or sets the duration for which the VMs are up in the on-premises
* environment.
*/
public data class AssessmentsOperationArgs(
public val assessmentName: Output? = null,
public val azureDiskTypes: Output>>? = null,
public val azureHybridUseBenefit: Output>? = null,
public val azureLocation: Output? = null,
public val azureOfferCode: Output>? = null,
public val azurePricingTier: Output>? = null,
public val azureStorageRedundancy: Output>? = null,
public val azureVmFamilies: Output>>? = null,
public val currency: Output>? = null,
public val discountPercentage: Output? = null,
public val eaSubscriptionId: Output? = null,
public val groupName: Output? = null,
public val linuxAzureHybridUseBenefit: Output>? = null,
public val percentile: Output>? = null,
public val perfDataEndTime: Output? = null,
public val perfDataStartTime: Output? = null,
public val projectName: Output? = null,
public val provisioningState: Output>? = null,
public val reservedInstance: Output>? = null,
public val resourceGroupName: Output? = null,
public val scalingFactor: Output? = null,
public val sizingCriterion: Output>? = null,
public val timeRange: Output>? = null,
public val vmUptime: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.migrate.AssessmentsOperationArgs =
com.pulumi.azurenative.migrate.AssessmentsOperationArgs.builder()
.assessmentName(assessmentName?.applyValue({ args0 -> args0 }))
.azureDiskTypes(
azureDiskTypes?.applyValue({ args0 ->
args0.map({ args0 ->
args0.transform({ args0 ->
args0
}, { args0 -> args0.let({ args0 -> args0.toJava() }) })
})
}),
)
.azureHybridUseBenefit(
azureHybridUseBenefit?.applyValue({ args0 ->
args0.transform({ args0 ->
args0
}, { args0 -> args0.let({ args0 -> args0.toJava() }) })
}),
)
.azureLocation(azureLocation?.applyValue({ args0 -> args0 }))
.azureOfferCode(
azureOfferCode?.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.azurePricingTier(
azurePricingTier?.applyValue({ args0 ->
args0.transform(
{ args0 -> args0 },
{ args0 -> args0.let({ args0 -> args0.toJava() }) },
)
}),
)
.azureStorageRedundancy(
azureStorageRedundancy?.applyValue({ args0 ->
args0.transform({ args0 ->
args0
}, { args0 -> args0.let({ args0 -> args0.toJava() }) })
}),
)
.azureVmFamilies(
azureVmFamilies?.applyValue({ args0 ->
args0.map({ args0 ->
args0.transform({ args0 -> args0 }, { args0 -> args0.let({ args0 -> args0.toJava() }) })
})
}),
)
.currency(
currency?.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.discountPercentage(discountPercentage?.applyValue({ args0 -> args0 }))
.eaSubscriptionId(eaSubscriptionId?.applyValue({ args0 -> args0 }))
.groupName(groupName?.applyValue({ args0 -> args0 }))
.linuxAzureHybridUseBenefit(
linuxAzureHybridUseBenefit?.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 -> args0.let({ args0 -> args0.toJava() }) })
}),
)
.percentile(
percentile?.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.perfDataEndTime(perfDataEndTime?.applyValue({ args0 -> args0 }))
.perfDataStartTime(perfDataStartTime?.applyValue({ args0 -> args0 }))
.projectName(projectName?.applyValue({ args0 -> args0 }))
.provisioningState(
provisioningState?.applyValue({ args0 ->
args0.transform(
{ args0 -> args0 },
{ args0 -> args0.let({ args0 -> args0.toJava() }) },
)
}),
)
.reservedInstance(
reservedInstance?.applyValue({ args0 ->
args0.transform(
{ args0 -> args0 },
{ args0 -> args0.let({ args0 -> args0.toJava() }) },
)
}),
)
.resourceGroupName(resourceGroupName?.applyValue({ args0 -> args0 }))
.scalingFactor(scalingFactor?.applyValue({ args0 -> args0 }))
.sizingCriterion(
sizingCriterion?.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.timeRange(
timeRange?.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.vmUptime(vmUptime?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [AssessmentsOperationArgs].
*/
@PulumiTagMarker
public class AssessmentsOperationArgsBuilder internal constructor() {
private var assessmentName: Output? = null
private var azureDiskTypes: Output>>? = null
private var azureHybridUseBenefit: Output>? = null
private var azureLocation: Output? = null
private var azureOfferCode: Output>? = null
private var azurePricingTier: Output>? = null
private var azureStorageRedundancy: Output>? = null
private var azureVmFamilies: Output>>? = null
private var currency: Output>? = null
private var discountPercentage: Output? = null
private var eaSubscriptionId: Output? = null
private var groupName: Output? = null
private var linuxAzureHybridUseBenefit: Output>? = null
private var percentile: Output>? = null
private var perfDataEndTime: Output? = null
private var perfDataStartTime: Output? = null
private var projectName: Output? = null
private var provisioningState: Output>? = null
private var reservedInstance: Output>? = null
private var resourceGroupName: Output? = null
private var scalingFactor: Output? = null
private var sizingCriterion: Output>? = null
private var timeRange: Output>? = null
private var vmUptime: Output? = null
/**
* @param value Machine Assessment ARM name
*/
@JvmName("njqaoolyyqongyvo")
public suspend fun assessmentName(`value`: Output) {
this.assessmentName = value
}
/**
* @param value Gets or sets the azure storage type. Premium, Standard etc.
*/
@JvmName("apkpiigoivolewul")
public suspend fun azureDiskTypes(`value`: Output>>) {
this.azureDiskTypes = value
}
@JvmName("opljxmhjbaarthsx")
public suspend fun azureDiskTypes(vararg values: Output>) {
this.azureDiskTypes = Output.all(values.asList())
}
/**
* @param values Gets or sets the azure storage type. Premium, Standard etc.
*/
@JvmName("tdjpvbttncoelmdk")
public suspend fun azureDiskTypes(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy