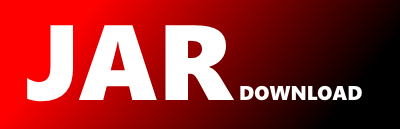
com.pulumi.azurenative.migrate.kotlin.SqlAssessmentV2OperationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.migrate.kotlin
import com.pulumi.azurenative.migrate.SqlAssessmentV2OperationArgs.builder
import com.pulumi.azurenative.migrate.kotlin.enums.AssessmentSizingCriterion
import com.pulumi.azurenative.migrate.kotlin.enums.AssessmentType
import com.pulumi.azurenative.migrate.kotlin.enums.AsyncCommitModeIntent
import com.pulumi.azurenative.migrate.kotlin.enums.AzureCurrency
import com.pulumi.azurenative.migrate.kotlin.enums.AzureLocation
import com.pulumi.azurenative.migrate.kotlin.enums.AzureOfferCode
import com.pulumi.azurenative.migrate.kotlin.enums.AzureReservedInstance
import com.pulumi.azurenative.migrate.kotlin.enums.AzureSecurityOfferingType
import com.pulumi.azurenative.migrate.kotlin.enums.EnvironmentType
import com.pulumi.azurenative.migrate.kotlin.enums.GroupType
import com.pulumi.azurenative.migrate.kotlin.enums.MultiSubnetIntent
import com.pulumi.azurenative.migrate.kotlin.enums.OptimizationLogic
import com.pulumi.azurenative.migrate.kotlin.enums.OsLicense
import com.pulumi.azurenative.migrate.kotlin.enums.Percentile
import com.pulumi.azurenative.migrate.kotlin.enums.ProvisioningState
import com.pulumi.azurenative.migrate.kotlin.enums.SqlServerLicense
import com.pulumi.azurenative.migrate.kotlin.enums.TimeRange
import com.pulumi.azurenative.migrate.kotlin.inputs.EntityUptimeArgs
import com.pulumi.azurenative.migrate.kotlin.inputs.EntityUptimeArgsBuilder
import com.pulumi.azurenative.migrate.kotlin.inputs.SqlDbSettingsArgs
import com.pulumi.azurenative.migrate.kotlin.inputs.SqlDbSettingsArgsBuilder
import com.pulumi.azurenative.migrate.kotlin.inputs.SqlMiSettingsArgs
import com.pulumi.azurenative.migrate.kotlin.inputs.SqlMiSettingsArgsBuilder
import com.pulumi.azurenative.migrate.kotlin.inputs.SqlVmSettingsArgs
import com.pulumi.azurenative.migrate.kotlin.inputs.SqlVmSettingsArgsBuilder
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Double
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* SQL Assessment REST resource.
* Azure REST API version: 2023-03-15.
* Other available API versions: 2023-04-01-preview.
* ## Example Usage
* ### SqlAssessmentV2Operations_Create_MaximumSet_Gen
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var sqlAssessmentV2Operation = new AzureNative.Migrate.SqlAssessmentV2Operation("sqlAssessmentV2Operation", new()
* {
* AssessmentName = "test_swagger_1",
* AsyncCommitModeIntent = AzureNative.Migrate.AsyncCommitModeIntent.DisasterRecovery,
* AzureLocation = "SoutheastAsia",
* AzureOfferCode = AzureNative.Migrate.AzureOfferCode.Msazr0003P,
* AzureOfferCodeForVm = AzureNative.Migrate.AzureOfferCode.Msazr0003P,
* AzureSqlDatabaseSettings = new AzureNative.Migrate.Inputs.SqlDbSettingsArgs
* {
* AzureSqlComputeTier = AzureNative.Migrate.ComputeTier.Automatic,
* AzureSqlDataBaseType = AzureNative.Migrate.AzureSqlDataBaseType.SingleDatabase,
* AzureSqlPurchaseModel = AzureNative.Migrate.AzureSqlPurchaseModel.VCore,
* AzureSqlServiceTier = AzureNative.Migrate.AzureSqlServiceTier.Automatic,
* },
* AzureSqlManagedInstanceSettings = new AzureNative.Migrate.Inputs.SqlMiSettingsArgs
* {
* AzureSqlInstanceType = AzureNative.Migrate.AzureSqlInstanceType.SingleInstance,
* AzureSqlServiceTier = AzureNative.Migrate.AzureSqlServiceTier.Automatic,
* },
* AzureSqlVmSettings = new AzureNative.Migrate.Inputs.SqlVmSettingsArgs
* {
* InstanceSeries = new[]
* {
* AzureNative.Migrate.AzureVmFamily.Eadsv5Series,
* },
* },
* Currency = AzureNative.Migrate.AzureCurrency.USD,
* DisasterRecoveryLocation = AzureNative.Migrate.AzureLocation.EastAsia,
* DiscountPercentage = 0,
* EnableHadrAssessment = true,
* EntityUptime = new AzureNative.Migrate.Inputs.EntityUptimeArgs
* {
* DaysPerMonth = 30,
* HoursPerDay = 24,
* },
* EnvironmentType = AzureNative.Migrate.EnvironmentType.Production,
* GroupName = "test_fci_hadr",
* MultiSubnetIntent = AzureNative.Migrate.MultiSubnetIntent.DisasterRecovery,
* OptimizationLogic = AzureNative.Migrate.OptimizationLogic.MinimizeCost,
* OsLicense = AzureNative.Migrate.OsLicense.Unknown,
* Percentile = AzureNative.Migrate.Percentile.Percentile95,
* ProjectName = "fci-test6904project",
* ReservedInstance = AzureNative.Migrate.AzureReservedInstance.None,
* ReservedInstanceForVm = AzureNative.Migrate.AzureReservedInstance.None,
* ResourceGroupName = "rgmigrate",
* ScalingFactor = 1,
* SizingCriterion = AzureNative.Migrate.AssessmentSizingCriterion.PerformanceBased,
* SqlServerLicense = AzureNative.Migrate.SqlServerLicense.Unknown,
* TimeRange = AzureNative.Migrate.TimeRange.Day,
* });
* });
* ```
* ```go
* package main
* import (
* migrate "github.com/pulumi/pulumi-azure-native-sdk/migrate/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := migrate.NewSqlAssessmentV2Operation(ctx, "sqlAssessmentV2Operation", &migrate.SqlAssessmentV2OperationArgs{
* AssessmentName: pulumi.String("test_swagger_1"),
* AsyncCommitModeIntent: pulumi.String(migrate.AsyncCommitModeIntentDisasterRecovery),
* AzureLocation: pulumi.String("SoutheastAsia"),
* AzureOfferCode: pulumi.String(migrate.AzureOfferCodeMsazr0003P),
* AzureOfferCodeForVm: pulumi.String(migrate.AzureOfferCodeMsazr0003P),
* AzureSqlDatabaseSettings: &migrate.SqlDbSettingsArgs{
* AzureSqlComputeTier: pulumi.String(migrate.ComputeTierAutomatic),
* AzureSqlDataBaseType: pulumi.String(migrate.AzureSqlDataBaseTypeSingleDatabase),
* AzureSqlPurchaseModel: pulumi.String(migrate.AzureSqlPurchaseModelVCore),
* AzureSqlServiceTier: pulumi.String(migrate.AzureSqlServiceTierAutomatic),
* },
* AzureSqlManagedInstanceSettings: &migrate.SqlMiSettingsArgs{
* AzureSqlInstanceType: pulumi.String(migrate.AzureSqlInstanceTypeSingleInstance),
* AzureSqlServiceTier: pulumi.String(migrate.AzureSqlServiceTierAutomatic),
* },
* AzureSqlVmSettings: &migrate.SqlVmSettingsArgs{
* InstanceSeries: pulumi.StringArray{
* pulumi.String(migrate.AzureVmFamilyEadsv5Series),
* },
* },
* Currency: pulumi.String(migrate.AzureCurrencyUSD),
* DisasterRecoveryLocation: pulumi.String(migrate.AzureLocationEastAsia),
* DiscountPercentage: pulumi.Float64(0),
* EnableHadrAssessment: pulumi.Bool(true),
* EntityUptime: &migrate.EntityUptimeArgs{
* DaysPerMonth: pulumi.Int(30),
* HoursPerDay: pulumi.Int(24),
* },
* EnvironmentType: pulumi.String(migrate.EnvironmentTypeProduction),
* GroupName: pulumi.String("test_fci_hadr"),
* MultiSubnetIntent: pulumi.String(migrate.MultiSubnetIntentDisasterRecovery),
* OptimizationLogic: pulumi.String(migrate.OptimizationLogicMinimizeCost),
* OsLicense: pulumi.String(migrate.OsLicenseUnknown),
* Percentile: pulumi.String(migrate.PercentilePercentile95),
* ProjectName: pulumi.String("fci-test6904project"),
* ReservedInstance: pulumi.String(migrate.AzureReservedInstanceNone),
* ReservedInstanceForVm: pulumi.String(migrate.AzureReservedInstanceNone),
* ResourceGroupName: pulumi.String("rgmigrate"),
* ScalingFactor: pulumi.Float64(1),
* SizingCriterion: pulumi.String(migrate.AssessmentSizingCriterionPerformanceBased),
* SqlServerLicense: pulumi.String(migrate.SqlServerLicenseUnknown),
* TimeRange: pulumi.String(migrate.TimeRangeDay),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.migrate.SqlAssessmentV2Operation;
* import com.pulumi.azurenative.migrate.SqlAssessmentV2OperationArgs;
* import com.pulumi.azurenative.migrate.inputs.SqlDbSettingsArgs;
* import com.pulumi.azurenative.migrate.inputs.SqlMiSettingsArgs;
* import com.pulumi.azurenative.migrate.inputs.SqlVmSettingsArgs;
* import com.pulumi.azurenative.migrate.inputs.EntityUptimeArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var sqlAssessmentV2Operation = new SqlAssessmentV2Operation("sqlAssessmentV2Operation", SqlAssessmentV2OperationArgs.builder()
* .assessmentName("test_swagger_1")
* .asyncCommitModeIntent("DisasterRecovery")
* .azureLocation("SoutheastAsia")
* .azureOfferCode("MSAZR0003P")
* .azureOfferCodeForVm("MSAZR0003P")
* .azureSqlDatabaseSettings(SqlDbSettingsArgs.builder()
* .azureSqlComputeTier("Automatic")
* .azureSqlDataBaseType("SingleDatabase")
* .azureSqlPurchaseModel("VCore")
* .azureSqlServiceTier("Automatic")
* .build())
* .azureSqlManagedInstanceSettings(SqlMiSettingsArgs.builder()
* .azureSqlInstanceType("SingleInstance")
* .azureSqlServiceTier("Automatic")
* .build())
* .azureSqlVmSettings(SqlVmSettingsArgs.builder()
* .instanceSeries("Eadsv5_series")
* .build())
* .currency("USD")
* .disasterRecoveryLocation("EastAsia")
* .discountPercentage(0)
* .enableHadrAssessment(true)
* .entityUptime(EntityUptimeArgs.builder()
* .daysPerMonth(30)
* .hoursPerDay(24)
* .build())
* .environmentType("Production")
* .groupName("test_fci_hadr")
* .multiSubnetIntent("DisasterRecovery")
* .optimizationLogic("MinimizeCost")
* .osLicense("Unknown")
* .percentile("Percentile95")
* .projectName("fci-test6904project")
* .reservedInstance("None")
* .reservedInstanceForVm("None")
* .resourceGroupName("rgmigrate")
* .scalingFactor(1)
* .sizingCriterion("PerformanceBased")
* .sqlServerLicense("Unknown")
* .timeRange("Day")
* .build());
* }
* }
* ```
* ## Import
* An existing resource can be imported using its type token, name, and identifier, e.g.
* ```sh
* $ pulumi import azure-native:migrate:SqlAssessmentV2Operation test_swagger_1 /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Migrate/assessmentProjects/{projectName}/groups/{groupName}/sqlAssessments/{assessmentName}
* ```
* @property assessmentName SQL Assessment arm name.
* @property assessmentType Assessment type of the assessment.
* @property asyncCommitModeIntent Gets or sets user preference indicating intent of async commit mode.
* @property azureLocation Azure Location or Azure region where to which the machines will be migrated.
* @property azureOfferCode Azure Offer Code.
* @property azureOfferCodeForVm Gets or sets Azure Offer Code for VM.
* @property azureSecurityOfferingType Gets or sets a value indicating azure security offering type.
* @property azureSqlDatabaseSettings Gets or sets user configurable SQL database settings.
* @property azureSqlManagedInstanceSettings Gets or sets user configurable SQL managed instance settings.
* @property azureSqlVmSettings Gets or sets user configurable SQL VM settings.
* @property confidenceRatingInPercentage Confidence Rating in Percentage.
* @property currency Currency in which prices should be reported.
* @property disasterRecoveryLocation Gets or sets the Azure Location or Azure region where to which the machines
* will be migrated.
* @property discountPercentage Custom discount percentage.
* @property eaSubscriptionId Gets or sets the Enterprise agreement subscription id.
* @property enableHadrAssessment Gets or sets a value indicating whether HADR assessments needs to be created.
* @property entityUptime Gets or sets the duration for which the entity (SQL, VMs) are up in the
* on-premises environment.
* @property environmentType Gets or sets user configurable setting to display the environment type.
* @property groupName Group ARM name
* @property groupType Gets the group type for the assessment.
* @property isInternetAccessAvailable Gets or sets a value indicating whether internet access is available.
* @property multiSubnetIntent Gets or sets user preference indicating intent of multi-subnet configuration.
* @property optimizationLogic Gets or sets SQL optimization logic.
* @property osLicense Gets or sets user configurable setting to display the azure hybrid use benefit.
* @property percentile Percentile of the utilization data values to be considered while assessing
* machines.
* @property perfDataEndTime Gets or sets the end time to consider performance data for assessment.
* @property perfDataStartTime Gets or sets the start time to consider performance data for assessment.
* @property projectName Assessment Project Name
* @property provisioningState The status of the last operation.
* @property reservedInstance Reserved instance.
* @property reservedInstanceForVm Gets or sets azure reserved instance for VM.
* @property resourceGroupName The name of the resource group. The name is case insensitive.
* @property scalingFactor Percentage of buffer that user wants on performance metrics when recommending
* Azure sizes.
* @property sizingCriterion Assessment sizing criterion.
* @property sqlServerLicense SQL server license.
* @property timeRange Time Range for which the historic utilization data should be considered for
* assessment.
*/
public data class SqlAssessmentV2OperationArgs(
public val assessmentName: Output? = null,
public val assessmentType: Output>? = null,
public val asyncCommitModeIntent: Output>? = null,
public val azureLocation: Output? = null,
public val azureOfferCode: Output>? = null,
public val azureOfferCodeForVm: Output>? = null,
public val azureSecurityOfferingType: Output>? = null,
public val azureSqlDatabaseSettings: Output? = null,
public val azureSqlManagedInstanceSettings: Output? = null,
public val azureSqlVmSettings: Output? = null,
public val confidenceRatingInPercentage: Output? = null,
public val currency: Output>? = null,
public val disasterRecoveryLocation: Output>? = null,
public val discountPercentage: Output? = null,
public val eaSubscriptionId: Output? = null,
public val enableHadrAssessment: Output? = null,
public val entityUptime: Output? = null,
public val environmentType: Output>? = null,
public val groupName: Output? = null,
public val groupType: Output>? = null,
public val isInternetAccessAvailable: Output? = null,
public val multiSubnetIntent: Output>? = null,
public val optimizationLogic: Output>? = null,
public val osLicense: Output>? = null,
public val percentile: Output>? = null,
public val perfDataEndTime: Output? = null,
public val perfDataStartTime: Output? = null,
public val projectName: Output? = null,
public val provisioningState: Output>? = null,
public val reservedInstance: Output>? = null,
public val reservedInstanceForVm: Output>? = null,
public val resourceGroupName: Output? = null,
public val scalingFactor: Output? = null,
public val sizingCriterion: Output>? = null,
public val sqlServerLicense: Output>? = null,
public val timeRange: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.migrate.SqlAssessmentV2OperationArgs =
com.pulumi.azurenative.migrate.SqlAssessmentV2OperationArgs.builder()
.assessmentName(assessmentName?.applyValue({ args0 -> args0 }))
.assessmentType(
assessmentType?.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.asyncCommitModeIntent(
asyncCommitModeIntent?.applyValue({ args0 ->
args0.transform({ args0 ->
args0
}, { args0 -> args0.let({ args0 -> args0.toJava() }) })
}),
)
.azureLocation(azureLocation?.applyValue({ args0 -> args0 }))
.azureOfferCode(
azureOfferCode?.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.azureOfferCodeForVm(
azureOfferCodeForVm?.applyValue({ args0 ->
args0.transform(
{ args0 -> args0 },
{ args0 -> args0.let({ args0 -> args0.toJava() }) },
)
}),
)
.azureSecurityOfferingType(
azureSecurityOfferingType?.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 -> args0.let({ args0 -> args0.toJava() }) })
}),
)
.azureSqlDatabaseSettings(
azureSqlDatabaseSettings?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.azureSqlManagedInstanceSettings(
azureSqlManagedInstanceSettings?.applyValue({ args0 ->
args0.let({ args0 -> args0.toJava() })
}),
)
.azureSqlVmSettings(
azureSqlVmSettings?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.confidenceRatingInPercentage(confidenceRatingInPercentage?.applyValue({ args0 -> args0 }))
.currency(
currency?.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.disasterRecoveryLocation(
disasterRecoveryLocation?.applyValue({ args0 ->
args0.transform({ args0 ->
args0
}, { args0 -> args0.let({ args0 -> args0.toJava() }) })
}),
)
.discountPercentage(discountPercentage?.applyValue({ args0 -> args0 }))
.eaSubscriptionId(eaSubscriptionId?.applyValue({ args0 -> args0 }))
.enableHadrAssessment(enableHadrAssessment?.applyValue({ args0 -> args0 }))
.entityUptime(entityUptime?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.environmentType(
environmentType?.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.groupName(groupName?.applyValue({ args0 -> args0 }))
.groupType(
groupType?.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.isInternetAccessAvailable(isInternetAccessAvailable?.applyValue({ args0 -> args0 }))
.multiSubnetIntent(
multiSubnetIntent?.applyValue({ args0 ->
args0.transform(
{ args0 -> args0 },
{ args0 -> args0.let({ args0 -> args0.toJava() }) },
)
}),
)
.optimizationLogic(
optimizationLogic?.applyValue({ args0 ->
args0.transform(
{ args0 -> args0 },
{ args0 -> args0.let({ args0 -> args0.toJava() }) },
)
}),
)
.osLicense(
osLicense?.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.percentile(
percentile?.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.perfDataEndTime(perfDataEndTime?.applyValue({ args0 -> args0 }))
.perfDataStartTime(perfDataStartTime?.applyValue({ args0 -> args0 }))
.projectName(projectName?.applyValue({ args0 -> args0 }))
.provisioningState(
provisioningState?.applyValue({ args0 ->
args0.transform(
{ args0 -> args0 },
{ args0 -> args0.let({ args0 -> args0.toJava() }) },
)
}),
)
.reservedInstance(
reservedInstance?.applyValue({ args0 ->
args0.transform(
{ args0 -> args0 },
{ args0 -> args0.let({ args0 -> args0.toJava() }) },
)
}),
)
.reservedInstanceForVm(
reservedInstanceForVm?.applyValue({ args0 ->
args0.transform({ args0 ->
args0
}, { args0 -> args0.let({ args0 -> args0.toJava() }) })
}),
)
.resourceGroupName(resourceGroupName?.applyValue({ args0 -> args0 }))
.scalingFactor(scalingFactor?.applyValue({ args0 -> args0 }))
.sizingCriterion(
sizingCriterion?.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.sqlServerLicense(
sqlServerLicense?.applyValue({ args0 ->
args0.transform(
{ args0 -> args0 },
{ args0 -> args0.let({ args0 -> args0.toJava() }) },
)
}),
)
.timeRange(
timeRange?.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
).build()
}
/**
* Builder for [SqlAssessmentV2OperationArgs].
*/
@PulumiTagMarker
public class SqlAssessmentV2OperationArgsBuilder internal constructor() {
private var assessmentName: Output? = null
private var assessmentType: Output>? = null
private var asyncCommitModeIntent: Output>? = null
private var azureLocation: Output? = null
private var azureOfferCode: Output>? = null
private var azureOfferCodeForVm: Output>? = null
private var azureSecurityOfferingType: Output>? = null
private var azureSqlDatabaseSettings: Output? = null
private var azureSqlManagedInstanceSettings: Output? = null
private var azureSqlVmSettings: Output? = null
private var confidenceRatingInPercentage: Output? = null
private var currency: Output>? = null
private var disasterRecoveryLocation: Output>? = null
private var discountPercentage: Output? = null
private var eaSubscriptionId: Output? = null
private var enableHadrAssessment: Output? = null
private var entityUptime: Output? = null
private var environmentType: Output>? = null
private var groupName: Output? = null
private var groupType: Output>? = null
private var isInternetAccessAvailable: Output? = null
private var multiSubnetIntent: Output>? = null
private var optimizationLogic: Output>? = null
private var osLicense: Output>? = null
private var percentile: Output>? = null
private var perfDataEndTime: Output? = null
private var perfDataStartTime: Output? = null
private var projectName: Output? = null
private var provisioningState: Output>? = null
private var reservedInstance: Output>? = null
private var reservedInstanceForVm: Output>? = null
private var resourceGroupName: Output? = null
private var scalingFactor: Output? = null
private var sizingCriterion: Output>? = null
private var sqlServerLicense: Output>? = null
private var timeRange: Output>? = null
/**
* @param value SQL Assessment arm name.
*/
@JvmName("bivbxtdpaxruwyqa")
public suspend fun assessmentName(`value`: Output) {
this.assessmentName = value
}
/**
* @param value Assessment type of the assessment.
*/
@JvmName("altgruurngupoeui")
public suspend fun assessmentType(`value`: Output>) {
this.assessmentType = value
}
/**
* @param value Gets or sets user preference indicating intent of async commit mode.
*/
@JvmName("vytjigtxhvgflyxu")
public suspend fun asyncCommitModeIntent(`value`: Output>) {
this.asyncCommitModeIntent = value
}
/**
* @param value Azure Location or Azure region where to which the machines will be migrated.
*/
@JvmName("gxeomuswtkwarccb")
public suspend fun azureLocation(`value`: Output) {
this.azureLocation = value
}
/**
* @param value Azure Offer Code.
*/
@JvmName("rrdciwjmoasbqxat")
public suspend fun azureOfferCode(`value`: Output>) {
this.azureOfferCode = value
}
/**
* @param value Gets or sets Azure Offer Code for VM.
*/
@JvmName("gdsmdmyyqdmirlmm")
public suspend fun azureOfferCodeForVm(`value`: Output>) {
this.azureOfferCodeForVm = value
}
/**
* @param value Gets or sets a value indicating azure security offering type.
*/
@JvmName("vjqumyoorwsyayql")
public suspend fun azureSecurityOfferingType(`value`: Output>) {
this.azureSecurityOfferingType = value
}
/**
* @param value Gets or sets user configurable SQL database settings.
*/
@JvmName("hckustyadbggfrhc")
public suspend fun azureSqlDatabaseSettings(`value`: Output) {
this.azureSqlDatabaseSettings = value
}
/**
* @param value Gets or sets user configurable SQL managed instance settings.
*/
@JvmName("cxlukrmbqafensio")
public suspend fun azureSqlManagedInstanceSettings(`value`: Output) {
this.azureSqlManagedInstanceSettings = value
}
/**
* @param value Gets or sets user configurable SQL VM settings.
*/
@JvmName("gxedowfbgfkxoong")
public suspend fun azureSqlVmSettings(`value`: Output) {
this.azureSqlVmSettings = value
}
/**
* @param value Confidence Rating in Percentage.
*/
@JvmName("detflflwklubuxsp")
public suspend fun confidenceRatingInPercentage(`value`: Output) {
this.confidenceRatingInPercentage = value
}
/**
* @param value Currency in which prices should be reported.
*/
@JvmName("wsauricsyyyhcysg")
public suspend fun currency(`value`: Output>) {
this.currency = value
}
/**
* @param value Gets or sets the Azure Location or Azure region where to which the machines
* will be migrated.
*/
@JvmName("mswhceimrbtkqmdb")
public suspend fun disasterRecoveryLocation(`value`: Output>) {
this.disasterRecoveryLocation = value
}
/**
* @param value Custom discount percentage.
*/
@JvmName("jntojpllbadxycjv")
public suspend fun discountPercentage(`value`: Output) {
this.discountPercentage = value
}
/**
* @param value Gets or sets the Enterprise agreement subscription id.
*/
@JvmName("rudydhtkdqumnnsw")
public suspend fun eaSubscriptionId(`value`: Output) {
this.eaSubscriptionId = value
}
/**
* @param value Gets or sets a value indicating whether HADR assessments needs to be created.
*/
@JvmName("yvmwfuoveptspmgj")
public suspend fun enableHadrAssessment(`value`: Output) {
this.enableHadrAssessment = value
}
/**
* @param value Gets or sets the duration for which the entity (SQL, VMs) are up in the
* on-premises environment.
*/
@JvmName("prmbnwvvbjlxxlkr")
public suspend fun entityUptime(`value`: Output) {
this.entityUptime = value
}
/**
* @param value Gets or sets user configurable setting to display the environment type.
*/
@JvmName("xrykabvxrkxpieuc")
public suspend fun environmentType(`value`: Output>) {
this.environmentType = value
}
/**
* @param value Group ARM name
*/
@JvmName("gdgryfmsuabyjadj")
public suspend fun groupName(`value`: Output) {
this.groupName = value
}
/**
* @param value Gets the group type for the assessment.
*/
@JvmName("clvpsikujctsurhm")
public suspend fun groupType(`value`: Output>) {
this.groupType = value
}
/**
* @param value Gets or sets a value indicating whether internet access is available.
*/
@JvmName("naescckwxbpvdqyf")
public suspend fun isInternetAccessAvailable(`value`: Output) {
this.isInternetAccessAvailable = value
}
/**
* @param value Gets or sets user preference indicating intent of multi-subnet configuration.
*/
@JvmName("calkvahiyxjiguxe")
public suspend fun multiSubnetIntent(`value`: Output>) {
this.multiSubnetIntent = value
}
/**
* @param value Gets or sets SQL optimization logic.
*/
@JvmName("rajtamdgplhejaaw")
public suspend fun optimizationLogic(`value`: Output>) {
this.optimizationLogic = value
}
/**
* @param value Gets or sets user configurable setting to display the azure hybrid use benefit.
*/
@JvmName("jdgpyingpfnpsrmd")
public suspend fun osLicense(`value`: Output>) {
this.osLicense = value
}
/**
* @param value Percentile of the utilization data values to be considered while assessing
* machines.
*/
@JvmName("rwtrlsavfxmfobgp")
public suspend fun percentile(`value`: Output>) {
this.percentile = value
}
/**
* @param value Gets or sets the end time to consider performance data for assessment.
*/
@JvmName("yweldkoohymsncfx")
public suspend fun perfDataEndTime(`value`: Output) {
this.perfDataEndTime = value
}
/**
* @param value Gets or sets the start time to consider performance data for assessment.
*/
@JvmName("yxyxqfesqofjhwit")
public suspend fun perfDataStartTime(`value`: Output) {
this.perfDataStartTime = value
}
/**
* @param value Assessment Project Name
*/
@JvmName("mtttyijeipyucsoq")
public suspend fun projectName(`value`: Output) {
this.projectName = value
}
/**
* @param value The status of the last operation.
*/
@JvmName("vagrkumkrxoxdlpw")
public suspend fun provisioningState(`value`: Output>) {
this.provisioningState = value
}
/**
* @param value Reserved instance.
*/
@JvmName("djjodxvfodlspamh")
public suspend fun reservedInstance(`value`: Output>) {
this.reservedInstance = value
}
/**
* @param value Gets or sets azure reserved instance for VM.
*/
@JvmName("rgpsvbgxqswpohpg")
public suspend fun reservedInstanceForVm(`value`: Output>) {
this.reservedInstanceForVm = value
}
/**
* @param value The name of the resource group. The name is case insensitive.
*/
@JvmName("ttswaxdwmjeatlxq")
public suspend fun resourceGroupName(`value`: Output) {
this.resourceGroupName = value
}
/**
* @param value Percentage of buffer that user wants on performance metrics when recommending
* Azure sizes.
*/
@JvmName("diplfcrwugkckedc")
public suspend fun scalingFactor(`value`: Output) {
this.scalingFactor = value
}
/**
* @param value Assessment sizing criterion.
*/
@JvmName("ayoydubtylstdtia")
public suspend fun sizingCriterion(`value`: Output>) {
this.sizingCriterion = value
}
/**
* @param value SQL server license.
*/
@JvmName("kfenyqguwliqdfii")
public suspend fun sqlServerLicense(`value`: Output>) {
this.sqlServerLicense = value
}
/**
* @param value Time Range for which the historic utilization data should be considered for
* assessment.
*/
@JvmName("xptrspiqoqekuith")
public suspend fun timeRange(`value`: Output>) {
this.timeRange = value
}
/**
* @param value SQL Assessment arm name.
*/
@JvmName("awdtcidcuwlajorf")
public suspend fun assessmentName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.assessmentName = mapped
}
/**
* @param value Assessment type of the assessment.
*/
@JvmName("shemeqklybolarod")
public suspend fun assessmentType(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.assessmentType = mapped
}
/**
* @param value Assessment type of the assessment.
*/
@JvmName("umvskdfrohjjkddt")
public fun assessmentType(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.assessmentType = mapped
}
/**
* @param value Assessment type of the assessment.
*/
@JvmName("sbtnlplgjrgmguwo")
public fun assessmentType(`value`: AssessmentType) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.assessmentType = mapped
}
/**
* @param value Gets or sets user preference indicating intent of async commit mode.
*/
@JvmName("sttsgqdxgoetbait")
public suspend fun asyncCommitModeIntent(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.asyncCommitModeIntent = mapped
}
/**
* @param value Gets or sets user preference indicating intent of async commit mode.
*/
@JvmName("tkwuqaxfrulgdbtq")
public fun asyncCommitModeIntent(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.asyncCommitModeIntent = mapped
}
/**
* @param value Gets or sets user preference indicating intent of async commit mode.
*/
@JvmName("ldfnempaekgddwds")
public fun asyncCommitModeIntent(`value`: AsyncCommitModeIntent) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.asyncCommitModeIntent = mapped
}
/**
* @param value Azure Location or Azure region where to which the machines will be migrated.
*/
@JvmName("venrkgswumurjudv")
public suspend fun azureLocation(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.azureLocation = mapped
}
/**
* @param value Azure Offer Code.
*/
@JvmName("ntenbntwfjymccor")
public suspend fun azureOfferCode(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.azureOfferCode = mapped
}
/**
* @param value Azure Offer Code.
*/
@JvmName("glwggsbpaemnglcj")
public fun azureOfferCode(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.azureOfferCode = mapped
}
/**
* @param value Azure Offer Code.
*/
@JvmName("qiupdrujsxxugkmw")
public fun azureOfferCode(`value`: AzureOfferCode) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.azureOfferCode = mapped
}
/**
* @param value Gets or sets Azure Offer Code for VM.
*/
@JvmName("lcmtbnhexexilccs")
public suspend fun azureOfferCodeForVm(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.azureOfferCodeForVm = mapped
}
/**
* @param value Gets or sets Azure Offer Code for VM.
*/
@JvmName("jyyrvrxnxoefxijf")
public fun azureOfferCodeForVm(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.azureOfferCodeForVm = mapped
}
/**
* @param value Gets or sets Azure Offer Code for VM.
*/
@JvmName("dlfqutwlcwhewlms")
public fun azureOfferCodeForVm(`value`: AzureOfferCode) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.azureOfferCodeForVm = mapped
}
/**
* @param value Gets or sets a value indicating azure security offering type.
*/
@JvmName("vglrilewijbvwfpq")
public suspend fun azureSecurityOfferingType(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.azureSecurityOfferingType = mapped
}
/**
* @param value Gets or sets a value indicating azure security offering type.
*/
@JvmName("rxtwbfgcbeknsuxv")
public fun azureSecurityOfferingType(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.azureSecurityOfferingType = mapped
}
/**
* @param value Gets or sets a value indicating azure security offering type.
*/
@JvmName("rbybhqdheodccgit")
public fun azureSecurityOfferingType(`value`: AzureSecurityOfferingType) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.azureSecurityOfferingType = mapped
}
/**
* @param value Gets or sets user configurable SQL database settings.
*/
@JvmName("kmbanafnnwqkjbkw")
public suspend fun azureSqlDatabaseSettings(`value`: SqlDbSettingsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.azureSqlDatabaseSettings = mapped
}
/**
* @param argument Gets or sets user configurable SQL database settings.
*/
@JvmName("ltpghigggyeyadhy")
public suspend fun azureSqlDatabaseSettings(argument: suspend SqlDbSettingsArgsBuilder.() -> Unit) {
val toBeMapped = SqlDbSettingsArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.azureSqlDatabaseSettings = mapped
}
/**
* @param value Gets or sets user configurable SQL managed instance settings.
*/
@JvmName("clutdptnphxhfvwg")
public suspend fun azureSqlManagedInstanceSettings(`value`: SqlMiSettingsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.azureSqlManagedInstanceSettings = mapped
}
/**
* @param argument Gets or sets user configurable SQL managed instance settings.
*/
@JvmName("iwpdjjmeedmjhtkq")
public suspend fun azureSqlManagedInstanceSettings(argument: suspend SqlMiSettingsArgsBuilder.() -> Unit) {
val toBeMapped = SqlMiSettingsArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.azureSqlManagedInstanceSettings = mapped
}
/**
* @param value Gets or sets user configurable SQL VM settings.
*/
@JvmName("nrfsuvhfhdbobfce")
public suspend fun azureSqlVmSettings(`value`: SqlVmSettingsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.azureSqlVmSettings = mapped
}
/**
* @param argument Gets or sets user configurable SQL VM settings.
*/
@JvmName("cxskunshhpajcvdm")
public suspend fun azureSqlVmSettings(argument: suspend SqlVmSettingsArgsBuilder.() -> Unit) {
val toBeMapped = SqlVmSettingsArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.azureSqlVmSettings = mapped
}
/**
* @param value Confidence Rating in Percentage.
*/
@JvmName("ykencfgubsenjdck")
public suspend fun confidenceRatingInPercentage(`value`: Double?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.confidenceRatingInPercentage = mapped
}
/**
* @param value Currency in which prices should be reported.
*/
@JvmName("iuicnaqrlodmrqyg")
public suspend fun currency(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.currency = mapped
}
/**
* @param value Currency in which prices should be reported.
*/
@JvmName("fsccrjmsptrgefms")
public fun currency(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.currency = mapped
}
/**
* @param value Currency in which prices should be reported.
*/
@JvmName("syavgilgobrhuxul")
public fun currency(`value`: AzureCurrency) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.currency = mapped
}
/**
* @param value Gets or sets the Azure Location or Azure region where to which the machines
* will be migrated.
*/
@JvmName("nxuedsluemanbtrf")
public suspend fun disasterRecoveryLocation(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.disasterRecoveryLocation = mapped
}
/**
* @param value Gets or sets the Azure Location or Azure region where to which the machines
* will be migrated.
*/
@JvmName("whsvtgngysjmcvsr")
public fun disasterRecoveryLocation(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.disasterRecoveryLocation = mapped
}
/**
* @param value Gets or sets the Azure Location or Azure region where to which the machines
* will be migrated.
*/
@JvmName("umswaxdjhtdtehrj")
public fun disasterRecoveryLocation(`value`: AzureLocation) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.disasterRecoveryLocation = mapped
}
/**
* @param value Custom discount percentage.
*/
@JvmName("qcbcbufweohsudrp")
public suspend fun discountPercentage(`value`: Double?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.discountPercentage = mapped
}
/**
* @param value Gets or sets the Enterprise agreement subscription id.
*/
@JvmName("uiiicbhsvesvblno")
public suspend fun eaSubscriptionId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.eaSubscriptionId = mapped
}
/**
* @param value Gets or sets a value indicating whether HADR assessments needs to be created.
*/
@JvmName("qygrbybvkekydsan")
public suspend fun enableHadrAssessment(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.enableHadrAssessment = mapped
}
/**
* @param value Gets or sets the duration for which the entity (SQL, VMs) are up in the
* on-premises environment.
*/
@JvmName("lropicsmdpqtwcbe")
public suspend fun entityUptime(`value`: EntityUptimeArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.entityUptime = mapped
}
/**
* @param argument Gets or sets the duration for which the entity (SQL, VMs) are up in the
* on-premises environment.
*/
@JvmName("rtaeuwxdveibhakv")
public suspend fun entityUptime(argument: suspend EntityUptimeArgsBuilder.() -> Unit) {
val toBeMapped = EntityUptimeArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.entityUptime = mapped
}
/**
* @param value Gets or sets user configurable setting to display the environment type.
*/
@JvmName("arnhxbumklqyqdnw")
public suspend fun environmentType(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.environmentType = mapped
}
/**
* @param value Gets or sets user configurable setting to display the environment type.
*/
@JvmName("jdgomnognxyjyadw")
public fun environmentType(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.environmentType = mapped
}
/**
* @param value Gets or sets user configurable setting to display the environment type.
*/
@JvmName("judjrcnmkgcvhlic")
public fun environmentType(`value`: EnvironmentType) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.environmentType = mapped
}
/**
* @param value Group ARM name
*/
@JvmName("jbaiggngqqujasln")
public suspend fun groupName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.groupName = mapped
}
/**
* @param value Gets the group type for the assessment.
*/
@JvmName("ucqkakcswsvalgfu")
public suspend fun groupType(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.groupType = mapped
}
/**
* @param value Gets the group type for the assessment.
*/
@JvmName("yykcqacboodurrjk")
public fun groupType(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.groupType = mapped
}
/**
* @param value Gets the group type for the assessment.
*/
@JvmName("wjyfbyhlwsknumwj")
public fun groupType(`value`: GroupType) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.groupType = mapped
}
/**
* @param value Gets or sets a value indicating whether internet access is available.
*/
@JvmName("mcjsscaklotysrtn")
public suspend fun isInternetAccessAvailable(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.isInternetAccessAvailable = mapped
}
/**
* @param value Gets or sets user preference indicating intent of multi-subnet configuration.
*/
@JvmName("jkxdickjppoggrcd")
public suspend fun multiSubnetIntent(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.multiSubnetIntent = mapped
}
/**
* @param value Gets or sets user preference indicating intent of multi-subnet configuration.
*/
@JvmName("yyrjhloirsvkulsi")
public fun multiSubnetIntent(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.multiSubnetIntent = mapped
}
/**
* @param value Gets or sets user preference indicating intent of multi-subnet configuration.
*/
@JvmName("dvvpekialkgvgbmg")
public fun multiSubnetIntent(`value`: MultiSubnetIntent) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.multiSubnetIntent = mapped
}
/**
* @param value Gets or sets SQL optimization logic.
*/
@JvmName("kjoplmuxbpuwjole")
public suspend fun optimizationLogic(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.optimizationLogic = mapped
}
/**
* @param value Gets or sets SQL optimization logic.
*/
@JvmName("ardwagqftnxxrmcq")
public fun optimizationLogic(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.optimizationLogic = mapped
}
/**
* @param value Gets or sets SQL optimization logic.
*/
@JvmName("cbnnusrsclbthgsr")
public fun optimizationLogic(`value`: OptimizationLogic) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.optimizationLogic = mapped
}
/**
* @param value Gets or sets user configurable setting to display the azure hybrid use benefit.
*/
@JvmName("dswapisdjqktlsmx")
public suspend fun osLicense(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.osLicense = mapped
}
/**
* @param value Gets or sets user configurable setting to display the azure hybrid use benefit.
*/
@JvmName("kcgyvnaxcluvmrts")
public fun osLicense(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.osLicense = mapped
}
/**
* @param value Gets or sets user configurable setting to display the azure hybrid use benefit.
*/
@JvmName("wihpkpanvujjiosd")
public fun osLicense(`value`: OsLicense) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.osLicense = mapped
}
/**
* @param value Percentile of the utilization data values to be considered while assessing
* machines.
*/
@JvmName("xulnuuvcjfhldlrm")
public suspend fun percentile(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.percentile = mapped
}
/**
* @param value Percentile of the utilization data values to be considered while assessing
* machines.
*/
@JvmName("bpoghqdriejxtrco")
public fun percentile(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.percentile = mapped
}
/**
* @param value Percentile of the utilization data values to be considered while assessing
* machines.
*/
@JvmName("mapnrsinrccprhvm")
public fun percentile(`value`: Percentile) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.percentile = mapped
}
/**
* @param value Gets or sets the end time to consider performance data for assessment.
*/
@JvmName("gruwmwpffethlkkv")
public suspend fun perfDataEndTime(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.perfDataEndTime = mapped
}
/**
* @param value Gets or sets the start time to consider performance data for assessment.
*/
@JvmName("cvcjacxngawclgna")
public suspend fun perfDataStartTime(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.perfDataStartTime = mapped
}
/**
* @param value Assessment Project Name
*/
@JvmName("xpmfgprqfpqqnayk")
public suspend fun projectName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.projectName = mapped
}
/**
* @param value The status of the last operation.
*/
@JvmName("lpmcfcpiwkaxdjvi")
public suspend fun provisioningState(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.provisioningState = mapped
}
/**
* @param value The status of the last operation.
*/
@JvmName("sukdctidqomibryx")
public fun provisioningState(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.provisioningState = mapped
}
/**
* @param value The status of the last operation.
*/
@JvmName("pqmckwmsyfisxnfj")
public fun provisioningState(`value`: ProvisioningState) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.provisioningState = mapped
}
/**
* @param value Reserved instance.
*/
@JvmName("ancrmcmbquolbgcf")
public suspend fun reservedInstance(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.reservedInstance = mapped
}
/**
* @param value Reserved instance.
*/
@JvmName("ankvkqfkwfpsjdxn")
public fun reservedInstance(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.reservedInstance = mapped
}
/**
* @param value Reserved instance.
*/
@JvmName("sxwpjauxvwajiqtt")
public fun reservedInstance(`value`: AzureReservedInstance) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.reservedInstance = mapped
}
/**
* @param value Gets or sets azure reserved instance for VM.
*/
@JvmName("ufnhhcjwymadtosn")
public suspend fun reservedInstanceForVm(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.reservedInstanceForVm = mapped
}
/**
* @param value Gets or sets azure reserved instance for VM.
*/
@JvmName("lsnmutesnnsyswuf")
public fun reservedInstanceForVm(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.reservedInstanceForVm = mapped
}
/**
* @param value Gets or sets azure reserved instance for VM.
*/
@JvmName("hoqescxwofambbuw")
public fun reservedInstanceForVm(`value`: AzureReservedInstance) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.reservedInstanceForVm = mapped
}
/**
* @param value The name of the resource group. The name is case insensitive.
*/
@JvmName("pmeveqpoiytoyaej")
public suspend fun resourceGroupName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.resourceGroupName = mapped
}
/**
* @param value Percentage of buffer that user wants on performance metrics when recommending
* Azure sizes.
*/
@JvmName("ylrihgrbkodlttig")
public suspend fun scalingFactor(`value`: Double?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.scalingFactor = mapped
}
/**
* @param value Assessment sizing criterion.
*/
@JvmName("nxyeoyrjopcbtscf")
public suspend fun sizingCriterion(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sizingCriterion = mapped
}
/**
* @param value Assessment sizing criterion.
*/
@JvmName("wmqfawykiragbbod")
public fun sizingCriterion(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.sizingCriterion = mapped
}
/**
* @param value Assessment sizing criterion.
*/
@JvmName("klijgjvrhutfqoju")
public fun sizingCriterion(`value`: AssessmentSizingCriterion) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.sizingCriterion = mapped
}
/**
* @param value SQL server license.
*/
@JvmName("vwcsoaklnxccgibc")
public suspend fun sqlServerLicense(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sqlServerLicense = mapped
}
/**
* @param value SQL server license.
*/
@JvmName("blypegtasouikxlx")
public fun sqlServerLicense(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.sqlServerLicense = mapped
}
/**
* @param value SQL server license.
*/
@JvmName("piqxrkgpstxerrac")
public fun sqlServerLicense(`value`: SqlServerLicense) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.sqlServerLicense = mapped
}
/**
* @param value Time Range for which the historic utilization data should be considered for
* assessment.
*/
@JvmName("nbbgoumumepnqcna")
public suspend fun timeRange(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.timeRange = mapped
}
/**
* @param value Time Range for which the historic utilization data should be considered for
* assessment.
*/
@JvmName("bunqpbkgxypkamtw")
public fun timeRange(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.timeRange = mapped
}
/**
* @param value Time Range for which the historic utilization data should be considered for
* assessment.
*/
@JvmName("bofavalnaifkbcmh")
public fun timeRange(`value`: TimeRange) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.timeRange = mapped
}
internal fun build(): SqlAssessmentV2OperationArgs = SqlAssessmentV2OperationArgs(
assessmentName = assessmentName,
assessmentType = assessmentType,
asyncCommitModeIntent = asyncCommitModeIntent,
azureLocation = azureLocation,
azureOfferCode = azureOfferCode,
azureOfferCodeForVm = azureOfferCodeForVm,
azureSecurityOfferingType = azureSecurityOfferingType,
azureSqlDatabaseSettings = azureSqlDatabaseSettings,
azureSqlManagedInstanceSettings = azureSqlManagedInstanceSettings,
azureSqlVmSettings = azureSqlVmSettings,
confidenceRatingInPercentage = confidenceRatingInPercentage,
currency = currency,
disasterRecoveryLocation = disasterRecoveryLocation,
discountPercentage = discountPercentage,
eaSubscriptionId = eaSubscriptionId,
enableHadrAssessment = enableHadrAssessment,
entityUptime = entityUptime,
environmentType = environmentType,
groupName = groupName,
groupType = groupType,
isInternetAccessAvailable = isInternetAccessAvailable,
multiSubnetIntent = multiSubnetIntent,
optimizationLogic = optimizationLogic,
osLicense = osLicense,
percentile = percentile,
perfDataEndTime = perfDataEndTime,
perfDataStartTime = perfDataStartTime,
projectName = projectName,
provisioningState = provisioningState,
reservedInstance = reservedInstance,
reservedInstanceForVm = reservedInstanceForVm,
resourceGroupName = resourceGroupName,
scalingFactor = scalingFactor,
sizingCriterion = sizingCriterion,
sqlServerLicense = sqlServerLicense,
timeRange = timeRange,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy