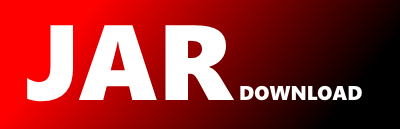
com.pulumi.azurenative.migrate.kotlin.inputs.AKSAssessmentSettingsArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.migrate.kotlin.inputs
import com.pulumi.azurenative.migrate.inputs.AKSAssessmentSettingsArgs.builder
import com.pulumi.azurenative.migrate.kotlin.enums.AssessmentSizingCriterion
import com.pulumi.azurenative.migrate.kotlin.enums.AzureCurrency
import com.pulumi.azurenative.migrate.kotlin.enums.AzureEnvironmentType
import com.pulumi.azurenative.migrate.kotlin.enums.AzureVmCategory
import com.pulumi.azurenative.migrate.kotlin.enums.ConsolidationType
import com.pulumi.azurenative.migrate.kotlin.enums.LicensingProgram
import com.pulumi.azurenative.migrate.kotlin.enums.PricingTier
import com.pulumi.azurenative.migrate.kotlin.enums.SavingsOptions
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Double
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Data model of AKS Assessment Settings.
* @property azureLocation Gets or sets azure location.
* @property category Gets or sets azure VM category.
* @property consolidation Gets or sets consolidation type.
* @property currency Gets or sets currency.
* @property discountPercentage Gets or sets discount percentage.
* @property environmentType Gets or sets environment type.
* @property licensingProgram Gets or sets licensing program.
* @property performanceData Gets or sets performance data settings.
* @property pricingTier Gets or sets pricing tier.
* @property savingsOptions Gets or sets savings options.
* @property scalingFactor Gets or sets scaling factor.
* @property sizingCriteria Gets or sets sizing criteria.
*/
public data class AKSAssessmentSettingsArgs(
public val azureLocation: Output,
public val category: Output>,
public val consolidation: Output>,
public val currency: Output>,
public val discountPercentage: Output? = null,
public val environmentType: Output>,
public val licensingProgram: Output>,
public val performanceData: Output? = null,
public val pricingTier: Output>,
public val savingsOptions: Output>,
public val scalingFactor: Output? = null,
public val sizingCriteria: Output>,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.migrate.inputs.AKSAssessmentSettingsArgs =
com.pulumi.azurenative.migrate.inputs.AKSAssessmentSettingsArgs.builder()
.azureLocation(azureLocation.applyValue({ args0 -> args0 }))
.category(
category.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.consolidation(
consolidation.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.currency(
currency.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.discountPercentage(discountPercentage?.applyValue({ args0 -> args0 }))
.environmentType(
environmentType.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.licensingProgram(
licensingProgram.applyValue({ args0 ->
args0.transform(
{ args0 -> args0 },
{ args0 -> args0.let({ args0 -> args0.toJava() }) },
)
}),
)
.performanceData(performanceData?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.pricingTier(
pricingTier.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.savingsOptions(
savingsOptions.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.scalingFactor(scalingFactor?.applyValue({ args0 -> args0 }))
.sizingCriteria(
sizingCriteria.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
).build()
}
/**
* Builder for [AKSAssessmentSettingsArgs].
*/
@PulumiTagMarker
public class AKSAssessmentSettingsArgsBuilder internal constructor() {
private var azureLocation: Output? = null
private var category: Output>? = null
private var consolidation: Output>? = null
private var currency: Output>? = null
private var discountPercentage: Output? = null
private var environmentType: Output>? = null
private var licensingProgram: Output>? = null
private var performanceData: Output? = null
private var pricingTier: Output>? = null
private var savingsOptions: Output>? = null
private var scalingFactor: Output? = null
private var sizingCriteria: Output>? = null
/**
* @param value Gets or sets azure location.
*/
@JvmName("mvardjqoneouufrj")
public suspend fun azureLocation(`value`: Output) {
this.azureLocation = value
}
/**
* @param value Gets or sets azure VM category.
*/
@JvmName("rqqnoqclrijykjto")
public suspend fun category(`value`: Output>) {
this.category = value
}
/**
* @param value Gets or sets consolidation type.
*/
@JvmName("huboabxvffpqccdk")
public suspend fun consolidation(`value`: Output>) {
this.consolidation = value
}
/**
* @param value Gets or sets currency.
*/
@JvmName("ibotpegaqwcporkp")
public suspend fun currency(`value`: Output>) {
this.currency = value
}
/**
* @param value Gets or sets discount percentage.
*/
@JvmName("dqcpiedofpcbyqnr")
public suspend fun discountPercentage(`value`: Output) {
this.discountPercentage = value
}
/**
* @param value Gets or sets environment type.
*/
@JvmName("iumjhxpkdlwvpqnw")
public suspend fun environmentType(`value`: Output>) {
this.environmentType = value
}
/**
* @param value Gets or sets licensing program.
*/
@JvmName("wljyycokserlhvhb")
public suspend fun licensingProgram(`value`: Output>) {
this.licensingProgram = value
}
/**
* @param value Gets or sets performance data settings.
*/
@JvmName("gnfpcondvalcyahs")
public suspend fun performanceData(`value`: Output) {
this.performanceData = value
}
/**
* @param value Gets or sets pricing tier.
*/
@JvmName("tlorjpmamfsnditl")
public suspend fun pricingTier(`value`: Output>) {
this.pricingTier = value
}
/**
* @param value Gets or sets savings options.
*/
@JvmName("vfgfecdhntcvdyne")
public suspend fun savingsOptions(`value`: Output>) {
this.savingsOptions = value
}
/**
* @param value Gets or sets scaling factor.
*/
@JvmName("vqnhwxommbuyshik")
public suspend fun scalingFactor(`value`: Output) {
this.scalingFactor = value
}
/**
* @param value Gets or sets sizing criteria.
*/
@JvmName("kvgyprnspjlajijn")
public suspend fun sizingCriteria(`value`: Output>) {
this.sizingCriteria = value
}
/**
* @param value Gets or sets azure location.
*/
@JvmName("skfbwumfcxmmniof")
public suspend fun azureLocation(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.azureLocation = mapped
}
/**
* @param value Gets or sets azure VM category.
*/
@JvmName("qmetucesxgfbcfyu")
public suspend fun category(`value`: Either) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.category = mapped
}
/**
* @param value Gets or sets azure VM category.
*/
@JvmName("mlrbpdwxjorjhoeh")
public fun category(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.category = mapped
}
/**
* @param value Gets or sets azure VM category.
*/
@JvmName("pgqreenfmcsqbhif")
public fun category(`value`: AzureVmCategory) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.category = mapped
}
/**
* @param value Gets or sets consolidation type.
*/
@JvmName("iagcuewjseaydthn")
public suspend fun consolidation(`value`: Either) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.consolidation = mapped
}
/**
* @param value Gets or sets consolidation type.
*/
@JvmName("ngloqqebmunqgqyx")
public fun consolidation(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.consolidation = mapped
}
/**
* @param value Gets or sets consolidation type.
*/
@JvmName("rmqktlhbgrhhoowc")
public fun consolidation(`value`: ConsolidationType) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.consolidation = mapped
}
/**
* @param value Gets or sets currency.
*/
@JvmName("puyugbnjmtplxolu")
public suspend fun currency(`value`: Either) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.currency = mapped
}
/**
* @param value Gets or sets currency.
*/
@JvmName("hxirswhuphfabtiv")
public fun currency(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.currency = mapped
}
/**
* @param value Gets or sets currency.
*/
@JvmName("diywqgniuxyyukbb")
public fun currency(`value`: AzureCurrency) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.currency = mapped
}
/**
* @param value Gets or sets discount percentage.
*/
@JvmName("qixsnykdwlcemtmq")
public suspend fun discountPercentage(`value`: Double?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.discountPercentage = mapped
}
/**
* @param value Gets or sets environment type.
*/
@JvmName("wvoxxkaxvnvevxvo")
public suspend fun environmentType(`value`: Either) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.environmentType = mapped
}
/**
* @param value Gets or sets environment type.
*/
@JvmName("merxhaiiafxmfvqx")
public fun environmentType(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.environmentType = mapped
}
/**
* @param value Gets or sets environment type.
*/
@JvmName("etviruvfmdohoqma")
public fun environmentType(`value`: AzureEnvironmentType) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.environmentType = mapped
}
/**
* @param value Gets or sets licensing program.
*/
@JvmName("kwixiesihrwjmgfh")
public suspend fun licensingProgram(`value`: Either) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.licensingProgram = mapped
}
/**
* @param value Gets or sets licensing program.
*/
@JvmName("vpvsdlbhjcodvfrm")
public fun licensingProgram(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.licensingProgram = mapped
}
/**
* @param value Gets or sets licensing program.
*/
@JvmName("hptigengehmyrddx")
public fun licensingProgram(`value`: LicensingProgram) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.licensingProgram = mapped
}
/**
* @param value Gets or sets performance data settings.
*/
@JvmName("rncoghcooultekov")
public suspend fun performanceData(`value`: PerfDataSettingsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.performanceData = mapped
}
/**
* @param argument Gets or sets performance data settings.
*/
@JvmName("kjrblkyacqjalufu")
public suspend fun performanceData(argument: suspend PerfDataSettingsArgsBuilder.() -> Unit) {
val toBeMapped = PerfDataSettingsArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.performanceData = mapped
}
/**
* @param value Gets or sets pricing tier.
*/
@JvmName("rdrqsbpjriheereb")
public suspend fun pricingTier(`value`: Either) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.pricingTier = mapped
}
/**
* @param value Gets or sets pricing tier.
*/
@JvmName("dxjtgmskonkhtesf")
public fun pricingTier(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.pricingTier = mapped
}
/**
* @param value Gets or sets pricing tier.
*/
@JvmName("erqkjaaebyitpgys")
public fun pricingTier(`value`: PricingTier) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.pricingTier = mapped
}
/**
* @param value Gets or sets savings options.
*/
@JvmName("apbceesjqrmgchmx")
public suspend fun savingsOptions(`value`: Either) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.savingsOptions = mapped
}
/**
* @param value Gets or sets savings options.
*/
@JvmName("qrlmehvtphkgbryn")
public fun savingsOptions(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.savingsOptions = mapped
}
/**
* @param value Gets or sets savings options.
*/
@JvmName("exeghwbnolnbtlpw")
public fun savingsOptions(`value`: SavingsOptions) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.savingsOptions = mapped
}
/**
* @param value Gets or sets scaling factor.
*/
@JvmName("csxawmtfbhfnypkr")
public suspend fun scalingFactor(`value`: Double?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.scalingFactor = mapped
}
/**
* @param value Gets or sets sizing criteria.
*/
@JvmName("riedfgofmgppfmik")
public suspend fun sizingCriteria(`value`: Either) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.sizingCriteria = mapped
}
/**
* @param value Gets or sets sizing criteria.
*/
@JvmName("urfhhcffvshmaxbn")
public fun sizingCriteria(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.sizingCriteria = mapped
}
/**
* @param value Gets or sets sizing criteria.
*/
@JvmName("pyeqkpyoylhujyyl")
public fun sizingCriteria(`value`: AssessmentSizingCriterion) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.sizingCriteria = mapped
}
internal fun build(): AKSAssessmentSettingsArgs = AKSAssessmentSettingsArgs(
azureLocation = azureLocation ?: throw PulumiNullFieldException("azureLocation"),
category = category ?: throw PulumiNullFieldException("category"),
consolidation = consolidation ?: throw PulumiNullFieldException("consolidation"),
currency = currency ?: throw PulumiNullFieldException("currency"),
discountPercentage = discountPercentage,
environmentType = environmentType ?: throw PulumiNullFieldException("environmentType"),
licensingProgram = licensingProgram ?: throw PulumiNullFieldException("licensingProgram"),
performanceData = performanceData,
pricingTier = pricingTier ?: throw PulumiNullFieldException("pricingTier"),
savingsOptions = savingsOptions ?: throw PulumiNullFieldException("savingsOptions"),
scalingFactor = scalingFactor,
sizingCriteria = sizingCriteria ?: throw PulumiNullFieldException("sizingCriteria"),
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy