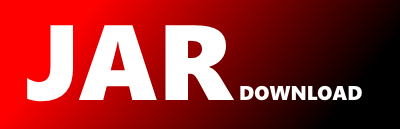
com.pulumi.azurenative.migrate.kotlin.inputs.ApacheTomcatWebApplicationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.migrate.kotlin.inputs
import com.pulumi.azurenative.migrate.inputs.ApacheTomcatWebApplicationArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* ApacheTomcat web application.
* @property applicationId Gets or sets the web application id.
* @property applicationName Gets or sets the web application name.
* @property applicationScratchPath Gets or sets application scratch path.
* @property bindings Gets or sets the bindings for the application.
* @property configurations Gets or sets application configuration.
* @property directories Gets or sets application directories.
* @property discoveredFrameworks Gets or sets the discovered frameworks of application.
* @property displayName Gets or sets the display name.
* @property limits Resource Requirements.
* @property path Second level entity for virtual directories.
* @property primaryFramework Framework specific data for a web application.
* @property requests Resource Requirements.
* @property webServerId Gets or sets the web server id.
* @property webServerName Gets or sets the web server name.
*/
public data class ApacheTomcatWebApplicationArgs(
public val applicationId: Output? = null,
public val applicationName: Output? = null,
public val applicationScratchPath: Output? = null,
public val bindings: Output>? = null,
public val configurations: Output>? = null,
public val directories: Output>? = null,
public val discoveredFrameworks: Output>? = null,
public val displayName: Output? = null,
public val limits: Output? = null,
public val path: Output? = null,
public val primaryFramework: Output? = null,
public val requests: Output? = null,
public val webServerId: Output? = null,
public val webServerName: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.migrate.inputs.ApacheTomcatWebApplicationArgs =
com.pulumi.azurenative.migrate.inputs.ApacheTomcatWebApplicationArgs.builder()
.applicationId(applicationId?.applyValue({ args0 -> args0 }))
.applicationName(applicationName?.applyValue({ args0 -> args0 }))
.applicationScratchPath(applicationScratchPath?.applyValue({ args0 -> args0 }))
.bindings(
bindings?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.configurations(
configurations?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.directories(
directories?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.discoveredFrameworks(
discoveredFrameworks?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.displayName(displayName?.applyValue({ args0 -> args0 }))
.limits(limits?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.path(path?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.primaryFramework(primaryFramework?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.requests(requests?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.webServerId(webServerId?.applyValue({ args0 -> args0 }))
.webServerName(webServerName?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ApacheTomcatWebApplicationArgs].
*/
@PulumiTagMarker
public class ApacheTomcatWebApplicationArgsBuilder internal constructor() {
private var applicationId: Output? = null
private var applicationName: Output? = null
private var applicationScratchPath: Output? = null
private var bindings: Output>? = null
private var configurations: Output>? = null
private var directories: Output>? = null
private var discoveredFrameworks: Output>? = null
private var displayName: Output? = null
private var limits: Output? = null
private var path: Output? = null
private var primaryFramework: Output? = null
private var requests: Output? = null
private var webServerId: Output? = null
private var webServerName: Output? = null
/**
* @param value Gets or sets the web application id.
*/
@JvmName("heepfadasoodqbhi")
public suspend fun applicationId(`value`: Output) {
this.applicationId = value
}
/**
* @param value Gets or sets the web application name.
*/
@JvmName("rcdbflauplybpjbk")
public suspend fun applicationName(`value`: Output) {
this.applicationName = value
}
/**
* @param value Gets or sets application scratch path.
*/
@JvmName("pjiquhbulhknhvgn")
public suspend fun applicationScratchPath(`value`: Output) {
this.applicationScratchPath = value
}
/**
* @param value Gets or sets the bindings for the application.
*/
@JvmName("iuouvewhcfegnwew")
public suspend fun bindings(`value`: Output>) {
this.bindings = value
}
@JvmName("dvkhnqtalhwwckvf")
public suspend fun bindings(vararg values: Output) {
this.bindings = Output.all(values.asList())
}
/**
* @param values Gets or sets the bindings for the application.
*/
@JvmName("wfhkulxmpqltmjqh")
public suspend fun bindings(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy