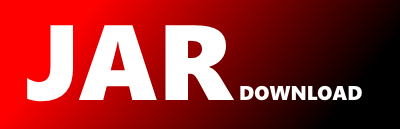
com.pulumi.azurenative.migrate.kotlin.outputs.AssessmentPropertiesResponse.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.migrate.kotlin.outputs
import kotlin.Double
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
/**
* Properties of an assessment.
* @property azureDiskType Storage type selected for this disk.
* @property azureHybridUseBenefit AHUB discount on windows virtual machines.
* @property azureLocation Target Azure location for which the machines should be assessed. These enums are the same as used by Compute API.
* @property azureOfferCode Offer code according to which cost estimation is done.
* @property azurePricingTier Pricing tier for Size evaluation.
* @property azureStorageRedundancy Storage Redundancy type offered by Azure.
* @property azureVmFamilies List of azure VM families.
* @property confidenceRatingInPercentage Confidence rating percentage for assessment. Can be in the range [0, 100].
* @property createdTimestamp Time when this project was created. Date-Time represented in ISO-8601 format.
* @property currency Currency to report prices in.
* @property discountPercentage Custom discount percentage to be applied on final costs. Can be in the range [0, 100].
* @property eaSubscriptionId Enterprise agreement subscription arm id.
* @property monthlyBandwidthCost Monthly network cost estimate for the machines that are part of this assessment as a group, for a 31-day month.
* @property monthlyComputeCost Monthly compute cost estimate for the machines that are part of this assessment as a group, for a 31-day month.
* @property monthlyPremiumStorageCost Monthly premium storage cost estimate for the machines that are part of this assessment as a group, for a 31-day month.
* @property monthlyStandardSSDStorageCost Monthly standard SSD storage cost estimate for the machines that are part of this assessment as a group, for a 31-day month.
* @property monthlyStorageCost Monthly storage cost estimate for the machines that are part of this assessment as a group, for a 31-day month.
* @property numberOfMachines Number of assessed machines part of this assessment.
* @property percentile Percentile of performance data used to recommend Azure size.
* @property perfDataEndTime End time to consider performance data for assessment
* @property perfDataStartTime Start time to consider performance data for assessment
* @property pricesTimestamp Time when the Azure Prices were queried. Date-Time represented in ISO-8601 format.
* @property reservedInstance Azure reserved instance.
* @property scalingFactor Scaling factor used over utilization data to add a performance buffer for new machines to be created in Azure. Min Value = 1.0, Max value = 1.9, Default = 1.3.
* @property sizingCriterion Assessment sizing criterion.
* @property stage User configurable setting that describes the status of the assessment.
* @property status Whether the assessment has been created and is valid.
* @property timeRange Time range of performance data used to recommend a size.
* @property updatedTimestamp Time when this project was last updated. Date-Time represented in ISO-8601 format.
* @property vmUptime Specify the duration for which the VMs are up in the on-premises environment.
*/
public data class AssessmentPropertiesResponse(
public val azureDiskType: String,
public val azureHybridUseBenefit: String,
public val azureLocation: String,
public val azureOfferCode: String,
public val azurePricingTier: String,
public val azureStorageRedundancy: String,
public val azureVmFamilies: List,
public val confidenceRatingInPercentage: Double,
public val createdTimestamp: String,
public val currency: String,
public val discountPercentage: Double,
public val eaSubscriptionId: String,
public val monthlyBandwidthCost: Double,
public val monthlyComputeCost: Double,
public val monthlyPremiumStorageCost: Double,
public val monthlyStandardSSDStorageCost: Double,
public val monthlyStorageCost: Double,
public val numberOfMachines: Int,
public val percentile: String,
public val perfDataEndTime: String,
public val perfDataStartTime: String,
public val pricesTimestamp: String,
public val reservedInstance: String,
public val scalingFactor: Double,
public val sizingCriterion: String,
public val stage: String,
public val status: String,
public val timeRange: String,
public val updatedTimestamp: String,
public val vmUptime: VmUptimeResponse,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.azurenative.migrate.outputs.AssessmentPropertiesResponse): AssessmentPropertiesResponse = AssessmentPropertiesResponse(
azureDiskType = javaType.azureDiskType(),
azureHybridUseBenefit = javaType.azureHybridUseBenefit(),
azureLocation = javaType.azureLocation(),
azureOfferCode = javaType.azureOfferCode(),
azurePricingTier = javaType.azurePricingTier(),
azureStorageRedundancy = javaType.azureStorageRedundancy(),
azureVmFamilies = javaType.azureVmFamilies().map({ args0 -> args0 }),
confidenceRatingInPercentage = javaType.confidenceRatingInPercentage(),
createdTimestamp = javaType.createdTimestamp(),
currency = javaType.currency(),
discountPercentage = javaType.discountPercentage(),
eaSubscriptionId = javaType.eaSubscriptionId(),
monthlyBandwidthCost = javaType.monthlyBandwidthCost(),
monthlyComputeCost = javaType.monthlyComputeCost(),
monthlyPremiumStorageCost = javaType.monthlyPremiumStorageCost(),
monthlyStandardSSDStorageCost = javaType.monthlyStandardSSDStorageCost(),
monthlyStorageCost = javaType.monthlyStorageCost(),
numberOfMachines = javaType.numberOfMachines(),
percentile = javaType.percentile(),
perfDataEndTime = javaType.perfDataEndTime(),
perfDataStartTime = javaType.perfDataStartTime(),
pricesTimestamp = javaType.pricesTimestamp(),
reservedInstance = javaType.reservedInstance(),
scalingFactor = javaType.scalingFactor(),
sizingCriterion = javaType.sizingCriterion(),
stage = javaType.stage(),
status = javaType.status(),
timeRange = javaType.timeRange(),
updatedTimestamp = javaType.updatedTimestamp(),
vmUptime = javaType.vmUptime().let({ args0 ->
com.pulumi.azurenative.migrate.kotlin.outputs.VmUptimeResponse.Companion.toKotlin(args0)
}),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy