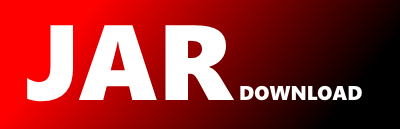
com.pulumi.azurenative.migrate.kotlin.outputs.GetAvsAssessmentsOperationResult.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.migrate.kotlin.outputs
import kotlin.Boolean
import kotlin.Double
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.Map
/**
* AVS assessment resource.
* @property assessmentErrorSummary Gets the assessment error summary.
* This is the number of machines
* affected by each type of error in this assessment.
* @property assessmentType Assessment type of the assessment.
* @property azureLocation Azure Location or Azure region where to which the machines will be migrated.
* @property azureOfferCode Azure Offer code according to which cost estimation is done.
* @property confidenceRatingInPercentage Confidence Rating in Percentage.
* @property cpuUtilization Predicted CPU utilization.
* @property createdTimestamp Date and Time when assessment was created.
* @property currency Currency in which prices should be reported.
* @property dedupeCompression De-duplication compression.
* @property discountPercentage Custom discount percentage.
* @property failuresToTolerateAndRaidLevel Failures to tolerate and RAID level in a common property.
* @property groupType Gets the group type for the assessment.
* @property id Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
* @property isStretchClusterEnabled Is Stretch Cluster Enabled.
* @property limitingFactor Limiting factor.
* @property memOvercommit Memory overcommit.
* @property name The name of the resource
* @property nodeType AVS node type.
* @property numberOfMachines Number of machines part of the assessment.
* @property numberOfNodes Recommended number of nodes.
* @property percentile Percentile of the utilization data values to be considered while assessing
* machines.
* @property perfDataEndTime Gets or sets the end time to consider performance data for assessment.
* @property perfDataStartTime Gets or sets the start time to consider performance data for assessment.
* @property pricesTimestamp Time when the Azure Prices were queried. Date-Time represented in ISO-8601
* format.
* @property provisioningState The status of the last operation.
* @property ramUtilization Predicted RAM utilization.
* @property reservedInstance Reserved instance.
* @property scalingFactor Percentage of buffer that user wants on performance metrics when recommending
* Azure sizes.
* @property schemaVersion Schema version.
* @property sizingCriterion Assessment sizing criterion.
* @property stage User configurable setting to display the Stage of Assessment.
* @property status Whether assessment is in valid state and all machines have been assessed.
* @property storageUtilization Predicted storage utilization.
* @property suitability Gets or sets the Assessment cloud suitability.
* @property suitabilityExplanation Gets or sets the Assessment suitability explanation.
* @property suitabilitySummary Cloud suitability summary for all the machines in the assessment.
* @property systemData Azure Resource Manager metadata containing createdBy and modifiedBy information.
* @property timeRange Time Range for which the historic utilization data should be considered for
* assessment.
* @property totalCpuCores Predicted total CPU cores used.
* @property totalMonthlyCost Total monthly cost.
* @property totalRamInGB Predicted total RAM used in GB.
* @property totalStorageInGB Predicted total Storage used in GB.
* @property type The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
* @property updatedTimestamp Date and Time when assessment was last updated.
* @property vcpuOversubscription VCPU over subscription.
*/
public data class GetAvsAssessmentsOperationResult(
public val assessmentErrorSummary: Map,
public val assessmentType: String,
public val azureLocation: String? = null,
public val azureOfferCode: String? = null,
public val confidenceRatingInPercentage: Double,
public val cpuUtilization: Double,
public val createdTimestamp: String,
public val currency: String? = null,
public val dedupeCompression: Double? = null,
public val discountPercentage: Double? = null,
public val failuresToTolerateAndRaidLevel: String? = null,
public val groupType: String,
public val id: String,
public val isStretchClusterEnabled: Boolean? = null,
public val limitingFactor: String,
public val memOvercommit: Double? = null,
public val name: String,
public val nodeType: String? = null,
public val numberOfMachines: Int,
public val numberOfNodes: Int,
public val percentile: String? = null,
public val perfDataEndTime: String? = null,
public val perfDataStartTime: String? = null,
public val pricesTimestamp: String,
public val provisioningState: String? = null,
public val ramUtilization: Double,
public val reservedInstance: String? = null,
public val scalingFactor: Double? = null,
public val schemaVersion: String,
public val sizingCriterion: String? = null,
public val stage: String,
public val status: String,
public val storageUtilization: Double,
public val suitability: String,
public val suitabilityExplanation: String,
public val suitabilitySummary: Map,
public val systemData: SystemDataResponse,
public val timeRange: String? = null,
public val totalCpuCores: Double,
public val totalMonthlyCost: Double,
public val totalRamInGB: Double,
public val totalStorageInGB: Double,
public val type: String,
public val updatedTimestamp: String,
public val vcpuOversubscription: Double? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.azurenative.migrate.outputs.GetAvsAssessmentsOperationResult): GetAvsAssessmentsOperationResult = GetAvsAssessmentsOperationResult(
assessmentErrorSummary = javaType.assessmentErrorSummary().map({ args0 ->
args0.key.to(args0.value)
}).toMap(),
assessmentType = javaType.assessmentType(),
azureLocation = javaType.azureLocation().map({ args0 -> args0 }).orElse(null),
azureOfferCode = javaType.azureOfferCode().map({ args0 -> args0 }).orElse(null),
confidenceRatingInPercentage = javaType.confidenceRatingInPercentage(),
cpuUtilization = javaType.cpuUtilization(),
createdTimestamp = javaType.createdTimestamp(),
currency = javaType.currency().map({ args0 -> args0 }).orElse(null),
dedupeCompression = javaType.dedupeCompression().map({ args0 -> args0 }).orElse(null),
discountPercentage = javaType.discountPercentage().map({ args0 -> args0 }).orElse(null),
failuresToTolerateAndRaidLevel = javaType.failuresToTolerateAndRaidLevel().map({ args0 ->
args0
}).orElse(null),
groupType = javaType.groupType(),
id = javaType.id(),
isStretchClusterEnabled = javaType.isStretchClusterEnabled().map({ args0 -> args0 }).orElse(null),
limitingFactor = javaType.limitingFactor(),
memOvercommit = javaType.memOvercommit().map({ args0 -> args0 }).orElse(null),
name = javaType.name(),
nodeType = javaType.nodeType().map({ args0 -> args0 }).orElse(null),
numberOfMachines = javaType.numberOfMachines(),
numberOfNodes = javaType.numberOfNodes(),
percentile = javaType.percentile().map({ args0 -> args0 }).orElse(null),
perfDataEndTime = javaType.perfDataEndTime().map({ args0 -> args0 }).orElse(null),
perfDataStartTime = javaType.perfDataStartTime().map({ args0 -> args0 }).orElse(null),
pricesTimestamp = javaType.pricesTimestamp(),
provisioningState = javaType.provisioningState().map({ args0 -> args0 }).orElse(null),
ramUtilization = javaType.ramUtilization(),
reservedInstance = javaType.reservedInstance().map({ args0 -> args0 }).orElse(null),
scalingFactor = javaType.scalingFactor().map({ args0 -> args0 }).orElse(null),
schemaVersion = javaType.schemaVersion(),
sizingCriterion = javaType.sizingCriterion().map({ args0 -> args0 }).orElse(null),
stage = javaType.stage(),
status = javaType.status(),
storageUtilization = javaType.storageUtilization(),
suitability = javaType.suitability(),
suitabilityExplanation = javaType.suitabilityExplanation(),
suitabilitySummary = javaType.suitabilitySummary().map({ args0 ->
args0.key.to(args0.value)
}).toMap(),
systemData = javaType.systemData().let({ args0 ->
com.pulumi.azurenative.migrate.kotlin.outputs.SystemDataResponse.Companion.toKotlin(args0)
}),
timeRange = javaType.timeRange().map({ args0 -> args0 }).orElse(null),
totalCpuCores = javaType.totalCpuCores(),
totalMonthlyCost = javaType.totalMonthlyCost(),
totalRamInGB = javaType.totalRamInGB(),
totalStorageInGB = javaType.totalStorageInGB(),
type = javaType.type(),
updatedTimestamp = javaType.updatedTimestamp(),
vcpuOversubscription = javaType.vcpuOversubscription().map({ args0 -> args0 }).orElse(null),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy