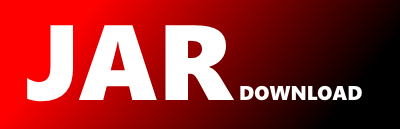
com.pulumi.azurenative.mixedreality.kotlin.MixedrealityFunctions.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.mixedreality.kotlin
import com.pulumi.azurenative.mixedreality.MixedrealityFunctions.getObjectAnchorsAccountPlain
import com.pulumi.azurenative.mixedreality.MixedrealityFunctions.getRemoteRenderingAccountPlain
import com.pulumi.azurenative.mixedreality.MixedrealityFunctions.getSpatialAnchorsAccountPlain
import com.pulumi.azurenative.mixedreality.MixedrealityFunctions.listObjectAnchorsAccountKeysPlain
import com.pulumi.azurenative.mixedreality.MixedrealityFunctions.listRemoteRenderingAccountKeysPlain
import com.pulumi.azurenative.mixedreality.MixedrealityFunctions.listSpatialAnchorsAccountKeysPlain
import com.pulumi.azurenative.mixedreality.kotlin.inputs.GetObjectAnchorsAccountPlainArgs
import com.pulumi.azurenative.mixedreality.kotlin.inputs.GetObjectAnchorsAccountPlainArgsBuilder
import com.pulumi.azurenative.mixedreality.kotlin.inputs.GetRemoteRenderingAccountPlainArgs
import com.pulumi.azurenative.mixedreality.kotlin.inputs.GetRemoteRenderingAccountPlainArgsBuilder
import com.pulumi.azurenative.mixedreality.kotlin.inputs.GetSpatialAnchorsAccountPlainArgs
import com.pulumi.azurenative.mixedreality.kotlin.inputs.GetSpatialAnchorsAccountPlainArgsBuilder
import com.pulumi.azurenative.mixedreality.kotlin.inputs.ListObjectAnchorsAccountKeysPlainArgs
import com.pulumi.azurenative.mixedreality.kotlin.inputs.ListObjectAnchorsAccountKeysPlainArgsBuilder
import com.pulumi.azurenative.mixedreality.kotlin.inputs.ListRemoteRenderingAccountKeysPlainArgs
import com.pulumi.azurenative.mixedreality.kotlin.inputs.ListRemoteRenderingAccountKeysPlainArgsBuilder
import com.pulumi.azurenative.mixedreality.kotlin.inputs.ListSpatialAnchorsAccountKeysPlainArgs
import com.pulumi.azurenative.mixedreality.kotlin.inputs.ListSpatialAnchorsAccountKeysPlainArgsBuilder
import com.pulumi.azurenative.mixedreality.kotlin.outputs.GetObjectAnchorsAccountResult
import com.pulumi.azurenative.mixedreality.kotlin.outputs.GetRemoteRenderingAccountResult
import com.pulumi.azurenative.mixedreality.kotlin.outputs.GetSpatialAnchorsAccountResult
import com.pulumi.azurenative.mixedreality.kotlin.outputs.ListObjectAnchorsAccountKeysResult
import com.pulumi.azurenative.mixedreality.kotlin.outputs.ListRemoteRenderingAccountKeysResult
import com.pulumi.azurenative.mixedreality.kotlin.outputs.ListSpatialAnchorsAccountKeysResult
import kotlinx.coroutines.future.await
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import com.pulumi.azurenative.mixedreality.kotlin.outputs.GetObjectAnchorsAccountResult.Companion.toKotlin as getObjectAnchorsAccountResultToKotlin
import com.pulumi.azurenative.mixedreality.kotlin.outputs.GetRemoteRenderingAccountResult.Companion.toKotlin as getRemoteRenderingAccountResultToKotlin
import com.pulumi.azurenative.mixedreality.kotlin.outputs.GetSpatialAnchorsAccountResult.Companion.toKotlin as getSpatialAnchorsAccountResultToKotlin
import com.pulumi.azurenative.mixedreality.kotlin.outputs.ListObjectAnchorsAccountKeysResult.Companion.toKotlin as listObjectAnchorsAccountKeysResultToKotlin
import com.pulumi.azurenative.mixedreality.kotlin.outputs.ListRemoteRenderingAccountKeysResult.Companion.toKotlin as listRemoteRenderingAccountKeysResultToKotlin
import com.pulumi.azurenative.mixedreality.kotlin.outputs.ListSpatialAnchorsAccountKeysResult.Companion.toKotlin as listSpatialAnchorsAccountKeysResultToKotlin
public object MixedrealityFunctions {
/**
* Retrieve an Object Anchors Account.
* Azure REST API version: 2021-03-01-preview.
* @param argument null
* @return ObjectAnchorsAccount Response.
*/
public suspend fun getObjectAnchorsAccount(argument: GetObjectAnchorsAccountPlainArgs): GetObjectAnchorsAccountResult =
getObjectAnchorsAccountResultToKotlin(getObjectAnchorsAccountPlain(argument.toJava()).await())
/**
* @see [getObjectAnchorsAccount].
* @param accountName Name of an Mixed Reality Account.
* @param resourceGroupName Name of an Azure resource group.
* @return ObjectAnchorsAccount Response.
*/
public suspend fun getObjectAnchorsAccount(accountName: String, resourceGroupName: String): GetObjectAnchorsAccountResult {
val argument = GetObjectAnchorsAccountPlainArgs(
accountName = accountName,
resourceGroupName = resourceGroupName,
)
return getObjectAnchorsAccountResultToKotlin(getObjectAnchorsAccountPlain(argument.toJava()).await())
}
/**
* @see [getObjectAnchorsAccount].
* @param argument Builder for [com.pulumi.azurenative.mixedreality.kotlin.inputs.GetObjectAnchorsAccountPlainArgs].
* @return ObjectAnchorsAccount Response.
*/
public suspend fun getObjectAnchorsAccount(argument: suspend GetObjectAnchorsAccountPlainArgsBuilder.() -> Unit): GetObjectAnchorsAccountResult {
val builder = GetObjectAnchorsAccountPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getObjectAnchorsAccountResultToKotlin(getObjectAnchorsAccountPlain(builtArgument.toJava()).await())
}
/**
* Retrieve a Remote Rendering Account.
* Azure REST API version: 2021-01-01.
* Other available API versions: 2021-03-01-preview.
* @param argument null
* @return RemoteRenderingAccount Response.
*/
public suspend fun getRemoteRenderingAccount(argument: GetRemoteRenderingAccountPlainArgs): GetRemoteRenderingAccountResult =
getRemoteRenderingAccountResultToKotlin(getRemoteRenderingAccountPlain(argument.toJava()).await())
/**
* @see [getRemoteRenderingAccount].
* @param accountName Name of an Mixed Reality Account.
* @param resourceGroupName Name of an Azure resource group.
* @return RemoteRenderingAccount Response.
*/
public suspend fun getRemoteRenderingAccount(accountName: String, resourceGroupName: String): GetRemoteRenderingAccountResult {
val argument = GetRemoteRenderingAccountPlainArgs(
accountName = accountName,
resourceGroupName = resourceGroupName,
)
return getRemoteRenderingAccountResultToKotlin(getRemoteRenderingAccountPlain(argument.toJava()).await())
}
/**
* @see [getRemoteRenderingAccount].
* @param argument Builder for [com.pulumi.azurenative.mixedreality.kotlin.inputs.GetRemoteRenderingAccountPlainArgs].
* @return RemoteRenderingAccount Response.
*/
public suspend fun getRemoteRenderingAccount(argument: suspend GetRemoteRenderingAccountPlainArgsBuilder.() -> Unit): GetRemoteRenderingAccountResult {
val builder = GetRemoteRenderingAccountPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getRemoteRenderingAccountResultToKotlin(getRemoteRenderingAccountPlain(builtArgument.toJava()).await())
}
/**
* Retrieve a Spatial Anchors Account.
* Azure REST API version: 2021-01-01.
* Other available API versions: 2019-02-28-preview, 2021-03-01-preview.
* @param argument null
* @return SpatialAnchorsAccount Response.
*/
public suspend fun getSpatialAnchorsAccount(argument: GetSpatialAnchorsAccountPlainArgs): GetSpatialAnchorsAccountResult =
getSpatialAnchorsAccountResultToKotlin(getSpatialAnchorsAccountPlain(argument.toJava()).await())
/**
* @see [getSpatialAnchorsAccount].
* @param accountName Name of an Mixed Reality Account.
* @param resourceGroupName Name of an Azure resource group.
* @return SpatialAnchorsAccount Response.
*/
public suspend fun getSpatialAnchorsAccount(accountName: String, resourceGroupName: String): GetSpatialAnchorsAccountResult {
val argument = GetSpatialAnchorsAccountPlainArgs(
accountName = accountName,
resourceGroupName = resourceGroupName,
)
return getSpatialAnchorsAccountResultToKotlin(getSpatialAnchorsAccountPlain(argument.toJava()).await())
}
/**
* @see [getSpatialAnchorsAccount].
* @param argument Builder for [com.pulumi.azurenative.mixedreality.kotlin.inputs.GetSpatialAnchorsAccountPlainArgs].
* @return SpatialAnchorsAccount Response.
*/
public suspend fun getSpatialAnchorsAccount(argument: suspend GetSpatialAnchorsAccountPlainArgsBuilder.() -> Unit): GetSpatialAnchorsAccountResult {
val builder = GetSpatialAnchorsAccountPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getSpatialAnchorsAccountResultToKotlin(getSpatialAnchorsAccountPlain(builtArgument.toJava()).await())
}
/**
* List Both of the 2 Keys of an object anchors Account
* Azure REST API version: 2021-03-01-preview.
* @param argument null
* @return Developer Keys of account
*/
public suspend fun listObjectAnchorsAccountKeys(argument: ListObjectAnchorsAccountKeysPlainArgs): ListObjectAnchorsAccountKeysResult =
listObjectAnchorsAccountKeysResultToKotlin(listObjectAnchorsAccountKeysPlain(argument.toJava()).await())
/**
* @see [listObjectAnchorsAccountKeys].
* @param accountName Name of an Mixed Reality Account.
* @param resourceGroupName Name of an Azure resource group.
* @return Developer Keys of account
*/
public suspend fun listObjectAnchorsAccountKeys(accountName: String, resourceGroupName: String): ListObjectAnchorsAccountKeysResult {
val argument = ListObjectAnchorsAccountKeysPlainArgs(
accountName = accountName,
resourceGroupName = resourceGroupName,
)
return listObjectAnchorsAccountKeysResultToKotlin(listObjectAnchorsAccountKeysPlain(argument.toJava()).await())
}
/**
* @see [listObjectAnchorsAccountKeys].
* @param argument Builder for [com.pulumi.azurenative.mixedreality.kotlin.inputs.ListObjectAnchorsAccountKeysPlainArgs].
* @return Developer Keys of account
*/
public suspend fun listObjectAnchorsAccountKeys(argument: suspend ListObjectAnchorsAccountKeysPlainArgsBuilder.() -> Unit): ListObjectAnchorsAccountKeysResult {
val builder = ListObjectAnchorsAccountKeysPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return listObjectAnchorsAccountKeysResultToKotlin(listObjectAnchorsAccountKeysPlain(builtArgument.toJava()).await())
}
/**
* List Both of the 2 Keys of a Remote Rendering Account
* Azure REST API version: 2021-01-01.
* Other available API versions: 2021-03-01-preview.
* @param argument null
* @return Developer Keys of account
*/
public suspend fun listRemoteRenderingAccountKeys(argument: ListRemoteRenderingAccountKeysPlainArgs): ListRemoteRenderingAccountKeysResult =
listRemoteRenderingAccountKeysResultToKotlin(listRemoteRenderingAccountKeysPlain(argument.toJava()).await())
/**
* @see [listRemoteRenderingAccountKeys].
* @param accountName Name of an Mixed Reality Account.
* @param resourceGroupName Name of an Azure resource group.
* @return Developer Keys of account
*/
public suspend fun listRemoteRenderingAccountKeys(accountName: String, resourceGroupName: String): ListRemoteRenderingAccountKeysResult {
val argument = ListRemoteRenderingAccountKeysPlainArgs(
accountName = accountName,
resourceGroupName = resourceGroupName,
)
return listRemoteRenderingAccountKeysResultToKotlin(listRemoteRenderingAccountKeysPlain(argument.toJava()).await())
}
/**
* @see [listRemoteRenderingAccountKeys].
* @param argument Builder for [com.pulumi.azurenative.mixedreality.kotlin.inputs.ListRemoteRenderingAccountKeysPlainArgs].
* @return Developer Keys of account
*/
public suspend fun listRemoteRenderingAccountKeys(argument: suspend ListRemoteRenderingAccountKeysPlainArgsBuilder.() -> Unit): ListRemoteRenderingAccountKeysResult {
val builder = ListRemoteRenderingAccountKeysPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return listRemoteRenderingAccountKeysResultToKotlin(listRemoteRenderingAccountKeysPlain(builtArgument.toJava()).await())
}
/**
* List Both of the 2 Keys of a Spatial Anchors Account
* Azure REST API version: 2021-01-01.
* Other available API versions: 2021-03-01-preview.
* @param argument null
* @return Developer Keys of account
*/
public suspend fun listSpatialAnchorsAccountKeys(argument: ListSpatialAnchorsAccountKeysPlainArgs): ListSpatialAnchorsAccountKeysResult =
listSpatialAnchorsAccountKeysResultToKotlin(listSpatialAnchorsAccountKeysPlain(argument.toJava()).await())
/**
* @see [listSpatialAnchorsAccountKeys].
* @param accountName Name of an Mixed Reality Account.
* @param resourceGroupName Name of an Azure resource group.
* @return Developer Keys of account
*/
public suspend fun listSpatialAnchorsAccountKeys(accountName: String, resourceGroupName: String): ListSpatialAnchorsAccountKeysResult {
val argument = ListSpatialAnchorsAccountKeysPlainArgs(
accountName = accountName,
resourceGroupName = resourceGroupName,
)
return listSpatialAnchorsAccountKeysResultToKotlin(listSpatialAnchorsAccountKeysPlain(argument.toJava()).await())
}
/**
* @see [listSpatialAnchorsAccountKeys].
* @param argument Builder for [com.pulumi.azurenative.mixedreality.kotlin.inputs.ListSpatialAnchorsAccountKeysPlainArgs].
* @return Developer Keys of account
*/
public suspend fun listSpatialAnchorsAccountKeys(argument: suspend ListSpatialAnchorsAccountKeysPlainArgsBuilder.() -> Unit): ListSpatialAnchorsAccountKeysResult {
val builder = ListSpatialAnchorsAccountKeysPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return listSpatialAnchorsAccountKeysResultToKotlin(listSpatialAnchorsAccountKeysPlain(builtArgument.toJava()).await())
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy