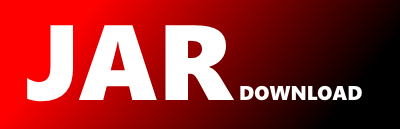
com.pulumi.azurenative.monitor.kotlin.PipelineGroupArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.monitor.kotlin
import com.pulumi.azurenative.monitor.PipelineGroupArgs.builder
import com.pulumi.azurenative.monitor.kotlin.inputs.AzureResourceManagerCommonTypesExtendedLocationArgs
import com.pulumi.azurenative.monitor.kotlin.inputs.AzureResourceManagerCommonTypesExtendedLocationArgsBuilder
import com.pulumi.azurenative.monitor.kotlin.inputs.ExporterArgs
import com.pulumi.azurenative.monitor.kotlin.inputs.ExporterArgsBuilder
import com.pulumi.azurenative.monitor.kotlin.inputs.NetworkingConfigurationArgs
import com.pulumi.azurenative.monitor.kotlin.inputs.NetworkingConfigurationArgsBuilder
import com.pulumi.azurenative.monitor.kotlin.inputs.ProcessorArgs
import com.pulumi.azurenative.monitor.kotlin.inputs.ProcessorArgsBuilder
import com.pulumi.azurenative.monitor.kotlin.inputs.ReceiverArgs
import com.pulumi.azurenative.monitor.kotlin.inputs.ReceiverArgsBuilder
import com.pulumi.azurenative.monitor.kotlin.inputs.ServiceArgs
import com.pulumi.azurenative.monitor.kotlin.inputs.ServiceArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Int
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* A pipeline group definition.
* Azure REST API version: 2023-10-01-preview.
* ## Example Usage
* ### Create a PipelineGroup instance using UDP receiver
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var pipelineGroup = new AzureNative.Monitor.PipelineGroup("pipelineGroup", new()
* {
* Exporters = new[]
* {
* new AzureNative.Monitor.Inputs.ExporterArgs
* {
* AzureMonitorWorkspaceLogs = new AzureNative.Monitor.Inputs.AzureMonitorWorkspaceLogsExporterArgs
* {
* Api = new AzureNative.Monitor.Inputs.AzureMonitorWorkspaceLogsApiConfigArgs
* {
* DataCollectionEndpointUrl = "https://logs-myingestion-eb0s.eastus-1.ingest.monitor.azure.com",
* DataCollectionRule = "dcr-00000000000000000000000000000000",
* Schema = new AzureNative.Monitor.Inputs.SchemaMapArgs
* {
* RecordMap = new[]
* {
* new AzureNative.Monitor.Inputs.RecordMapArgs
* {
* From = "body",
* To = "Body",
* },
* new AzureNative.Monitor.Inputs.RecordMapArgs
* {
* From = "severity_text",
* To = "SeverityText",
* },
* new AzureNative.Monitor.Inputs.RecordMapArgs
* {
* From = "time_unix_nano",
* To = "TimeGenerated",
* },
* },
* },
* Stream = "Custom-MyTableRawData_CL",
* },
* Concurrency = new AzureNative.Monitor.Inputs.ConcurrencyConfigurationArgs
* {
* BatchQueueSize = 100,
* WorkerCount = 4,
* },
* },
* Name = "my-workspace-logs-exporter1",
* Type = AzureNative.Monitor.ExporterType.AzureMonitorWorkspaceLogs,
* },
* },
* ExtendedLocation = new AzureNative.Monitor.Inputs.AzureResourceManagerCommonTypesExtendedLocationArgs
* {
* Name = "/subscriptions/00000000-0000-0000-0000-000000000000/resourcegroups/myResourceGroup/providers/microsoft.extendedlocation/customlocations/myTestCustomLocation",
* Type = AzureNative.Monitor.ExtendedLocationType.CustomLocation,
* },
* Location = "eastus2",
* PipelineGroupName = "plGroup1",
* Processors = new[] {},
* Receivers = new[]
* {
* new AzureNative.Monitor.Inputs.ReceiverArgs
* {
* Name = "udp-receiver1",
* Type = AzureNative.Monitor.ReceiverType.UDP,
* Udp = new AzureNative.Monitor.Inputs.UdpReceiverArgs
* {
* Encoding = AzureNative.Monitor.StreamEncodingType.Utf_8,
* Endpoint = "0.0.0.0:518",
* },
* },
* },
* ResourceGroupName = "myResourceGroup",
* Service = new AzureNative.Monitor.Inputs.ServiceArgs
* {
* Pipelines = new[]
* {
* new AzureNative.Monitor.Inputs.PipelineArgs
* {
* Exporters = new[]
* {
* "my-workspace-logs-exporter1",
* },
* Name = "MyPipelineForLogs1",
* Processors = new() { },
* Receivers = new[]
* {
* "udp-receiver1",
* },
* Type = AzureNative.Monitor.PipelineType.Logs,
* },
* },
* },
* Tags =
* {
* { "tag1", "A" },
* { "tag2", "B" },
* },
* });
* });
* ```
* ```go
* package main
* import (
* monitor "github.com/pulumi/pulumi-azure-native-sdk/monitor/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := monitor.NewPipelineGroup(ctx, "pipelineGroup", &monitor.PipelineGroupArgs{
* Exporters: monitor.ExporterArray{
* &monitor.ExporterArgs{
* AzureMonitorWorkspaceLogs: &monitor.AzureMonitorWorkspaceLogsExporterArgs{
* Api: &monitor.AzureMonitorWorkspaceLogsApiConfigArgs{
* DataCollectionEndpointUrl: pulumi.String("https://logs-myingestion-eb0s.eastus-1.ingest.monitor.azure.com"),
* DataCollectionRule: pulumi.String("dcr-00000000000000000000000000000000"),
* Schema: &monitor.SchemaMapArgs{
* RecordMap: monitor.RecordMapArray{
* &monitor.RecordMapArgs{
* From: pulumi.String("body"),
* To: pulumi.String("Body"),
* },
* &monitor.RecordMapArgs{
* From: pulumi.String("severity_text"),
* To: pulumi.String("SeverityText"),
* },
* &monitor.RecordMapArgs{
* From: pulumi.String("time_unix_nano"),
* To: pulumi.String("TimeGenerated"),
* },
* },
* },
* Stream: pulumi.String("Custom-MyTableRawData_CL"),
* },
* Concurrency: &monitor.ConcurrencyConfigurationArgs{
* BatchQueueSize: pulumi.Int(100),
* WorkerCount: pulumi.Int(4),
* },
* },
* Name: pulumi.String("my-workspace-logs-exporter1"),
* Type: pulumi.String(monitor.ExporterTypeAzureMonitorWorkspaceLogs),
* },
* },
* ExtendedLocation: &monitor.AzureResourceManagerCommonTypesExtendedLocationArgs{
* Name: pulumi.String("/subscriptions/00000000-0000-0000-0000-000000000000/resourcegroups/myResourceGroup/providers/microsoft.extendedlocation/customlocations/myTestCustomLocation"),
* Type: pulumi.String(monitor.ExtendedLocationTypeCustomLocation),
* },
* Location: pulumi.String("eastus2"),
* PipelineGroupName: pulumi.String("plGroup1"),
* Processors: monitor.ProcessorArray{},
* Receivers: monitor.ReceiverArray{
* &monitor.ReceiverArgs{
* Name: pulumi.String("udp-receiver1"),
* Type: pulumi.String(monitor.ReceiverTypeUDP),
* Udp: &monitor.UdpReceiverArgs{
* Encoding: pulumi.String(monitor.StreamEncodingType_Utf_8),
* Endpoint: pulumi.String("0.0.0.0:518"),
* },
* },
* },
* ResourceGroupName: pulumi.String("myResourceGroup"),
* Service: &monitor.ServiceArgs{
* Pipelines: monitor.PipelineArray{
* &monitor.PipelineArgs{
* Exporters: pulumi.StringArray{
* pulumi.String("my-workspace-logs-exporter1"),
* },
* Name: pulumi.String("MyPipelineForLogs1"),
* Processors: pulumi.StringArray{},
* Receivers: pulumi.StringArray{
* pulumi.String("udp-receiver1"),
* },
* Type: pulumi.String(monitor.PipelineTypeLogs),
* },
* },
* },
* Tags: pulumi.StringMap{
* "tag1": pulumi.String("A"),
* "tag2": pulumi.String("B"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.monitor.PipelineGroup;
* import com.pulumi.azurenative.monitor.PipelineGroupArgs;
* import com.pulumi.azurenative.monitor.inputs.ExporterArgs;
* import com.pulumi.azurenative.monitor.inputs.AzureMonitorWorkspaceLogsExporterArgs;
* import com.pulumi.azurenative.monitor.inputs.AzureMonitorWorkspaceLogsApiConfigArgs;
* import com.pulumi.azurenative.monitor.inputs.SchemaMapArgs;
* import com.pulumi.azurenative.monitor.inputs.ConcurrencyConfigurationArgs;
* import com.pulumi.azurenative.monitor.inputs.AzureResourceManagerCommonTypesExtendedLocationArgs;
* import com.pulumi.azurenative.monitor.inputs.ReceiverArgs;
* import com.pulumi.azurenative.monitor.inputs.UdpReceiverArgs;
* import com.pulumi.azurenative.monitor.inputs.ServiceArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var pipelineGroup = new PipelineGroup("pipelineGroup", PipelineGroupArgs.builder()
* .exporters(ExporterArgs.builder()
* .azureMonitorWorkspaceLogs(AzureMonitorWorkspaceLogsExporterArgs.builder()
* .api(AzureMonitorWorkspaceLogsApiConfigArgs.builder()
* .dataCollectionEndpointUrl("https://logs-myingestion-eb0s.eastus-1.ingest.monitor.azure.com")
* .dataCollectionRule("dcr-00000000000000000000000000000000")
* .schema(SchemaMapArgs.builder()
* .recordMap(
* RecordMapArgs.builder()
* .from("body")
* .to("Body")
* .build(),
* RecordMapArgs.builder()
* .from("severity_text")
* .to("SeverityText")
* .build(),
* RecordMapArgs.builder()
* .from("time_unix_nano")
* .to("TimeGenerated")
* .build())
* .build())
* .stream("Custom-MyTableRawData_CL")
* .build())
* .concurrency(ConcurrencyConfigurationArgs.builder()
* .batchQueueSize(100)
* .workerCount(4)
* .build())
* .build())
* .name("my-workspace-logs-exporter1")
* .type("AzureMonitorWorkspaceLogs")
* .build())
* .extendedLocation(AzureResourceManagerCommonTypesExtendedLocationArgs.builder()
* .name("/subscriptions/00000000-0000-0000-0000-000000000000/resourcegroups/myResourceGroup/providers/microsoft.extendedlocation/customlocations/myTestCustomLocation")
* .type("CustomLocation")
* .build())
* .location("eastus2")
* .pipelineGroupName("plGroup1")
* .processors()
* .receivers(ReceiverArgs.builder()
* .name("udp-receiver1")
* .type("UDP")
* .udp(UdpReceiverArgs.builder()
* .encoding("utf-8")
* .endpoint("0.0.0.0:518")
* .build())
* .build())
* .resourceGroupName("myResourceGroup")
* .service(ServiceArgs.builder()
* .pipelines(PipelineArgs.builder()
* .exporters("my-workspace-logs-exporter1")
* .name("MyPipelineForLogs1")
* .processors()
* .receivers("udp-receiver1")
* .type("logs")
* .build())
* .build())
* .tags(Map.ofEntries(
* Map.entry("tag1", "A"),
* Map.entry("tag2", "B")
* ))
* .build());
* }
* }
* ```
* ### Create a PipelineGroup instance using a syslog receiver
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var pipelineGroup = new AzureNative.Monitor.PipelineGroup("pipelineGroup", new()
* {
* Exporters = new[]
* {
* new AzureNative.Monitor.Inputs.ExporterArgs
* {
* AzureMonitorWorkspaceLogs = new AzureNative.Monitor.Inputs.AzureMonitorWorkspaceLogsExporterArgs
* {
* Api = new AzureNative.Monitor.Inputs.AzureMonitorWorkspaceLogsApiConfigArgs
* {
* DataCollectionEndpointUrl = "https://logs-myingestion-eb0s.eastus-1.ingest.monitor.azure.com",
* DataCollectionRule = "dcr-00000000000000000000000000000000",
* Schema = new AzureNative.Monitor.Inputs.SchemaMapArgs
* {
* RecordMap = new[]
* {
* new AzureNative.Monitor.Inputs.RecordMapArgs
* {
* From = "body",
* To = "Body",
* },
* new AzureNative.Monitor.Inputs.RecordMapArgs
* {
* From = "severity_text",
* To = "SeverityText",
* },
* new AzureNative.Monitor.Inputs.RecordMapArgs
* {
* From = "time_unix_nano",
* To = "TimeGenerated",
* },
* },
* },
* Stream = "Custom-MyTableRawData_CL",
* },
* Concurrency = new AzureNative.Monitor.Inputs.ConcurrencyConfigurationArgs
* {
* BatchQueueSize = 100,
* WorkerCount = 4,
* },
* },
* Name = "my-workspace-logs-exporter1",
* Type = AzureNative.Monitor.ExporterType.AzureMonitorWorkspaceLogs,
* },
* },
* ExtendedLocation = new AzureNative.Monitor.Inputs.AzureResourceManagerCommonTypesExtendedLocationArgs
* {
* Name = "/subscriptions/00000000-0000-0000-0000-000000000000/resourcegroups/myResourceGroup/providers/microsoft.extendedlocation/customlocations/myTestCustomLocation",
* Type = AzureNative.Monitor.ExtendedLocationType.CustomLocation,
* },
* Location = "eastus2",
* PipelineGroupName = "plGroup1",
* Processors = new[]
* {
* new AzureNative.Monitor.Inputs.ProcessorArgs
* {
* Name = "batch-processor1",
* Type = AzureNative.Monitor.ProcessorType.Batch,
* },
* },
* Receivers = new[]
* {
* new AzureNative.Monitor.Inputs.ReceiverArgs
* {
* Name = "syslog-receiver1",
* Syslog = new AzureNative.Monitor.Inputs.SyslogReceiverArgs
* {
* Endpoint = "0.0.0.0:514",
* },
* Type = AzureNative.Monitor.ReceiverType.Syslog,
* },
* },
* ResourceGroupName = "myResourceGroup",
* Service = new AzureNative.Monitor.Inputs.ServiceArgs
* {
* Pipelines = new[]
* {
* new AzureNative.Monitor.Inputs.PipelineArgs
* {
* Exporters = new[]
* {
* "my-workspace-logs-exporter1",
* },
* Name = "MyPipelineForLogs1",
* Processors = new[]
* {
* "batch-processor1",
* },
* Receivers = new[]
* {
* "syslog-receiver1",
* },
* Type = AzureNative.Monitor.PipelineType.Logs,
* },
* },
* },
* Tags =
* {
* { "tag1", "A" },
* { "tag2", "B" },
* },
* });
* });
* ```
* ```go
* package main
* import (
* monitor "github.com/pulumi/pulumi-azure-native-sdk/monitor/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := monitor.NewPipelineGroup(ctx, "pipelineGroup", &monitor.PipelineGroupArgs{
* Exporters: monitor.ExporterArray{
* &monitor.ExporterArgs{
* AzureMonitorWorkspaceLogs: &monitor.AzureMonitorWorkspaceLogsExporterArgs{
* Api: &monitor.AzureMonitorWorkspaceLogsApiConfigArgs{
* DataCollectionEndpointUrl: pulumi.String("https://logs-myingestion-eb0s.eastus-1.ingest.monitor.azure.com"),
* DataCollectionRule: pulumi.String("dcr-00000000000000000000000000000000"),
* Schema: &monitor.SchemaMapArgs{
* RecordMap: monitor.RecordMapArray{
* &monitor.RecordMapArgs{
* From: pulumi.String("body"),
* To: pulumi.String("Body"),
* },
* &monitor.RecordMapArgs{
* From: pulumi.String("severity_text"),
* To: pulumi.String("SeverityText"),
* },
* &monitor.RecordMapArgs{
* From: pulumi.String("time_unix_nano"),
* To: pulumi.String("TimeGenerated"),
* },
* },
* },
* Stream: pulumi.String("Custom-MyTableRawData_CL"),
* },
* Concurrency: &monitor.ConcurrencyConfigurationArgs{
* BatchQueueSize: pulumi.Int(100),
* WorkerCount: pulumi.Int(4),
* },
* },
* Name: pulumi.String("my-workspace-logs-exporter1"),
* Type: pulumi.String(monitor.ExporterTypeAzureMonitorWorkspaceLogs),
* },
* },
* ExtendedLocation: &monitor.AzureResourceManagerCommonTypesExtendedLocationArgs{
* Name: pulumi.String("/subscriptions/00000000-0000-0000-0000-000000000000/resourcegroups/myResourceGroup/providers/microsoft.extendedlocation/customlocations/myTestCustomLocation"),
* Type: pulumi.String(monitor.ExtendedLocationTypeCustomLocation),
* },
* Location: pulumi.String("eastus2"),
* PipelineGroupName: pulumi.String("plGroup1"),
* Processors: monitor.ProcessorArray{
* &monitor.ProcessorArgs{
* Name: pulumi.String("batch-processor1"),
* Type: pulumi.String(monitor.ProcessorTypeBatch),
* },
* },
* Receivers: monitor.ReceiverArray{
* &monitor.ReceiverArgs{
* Name: pulumi.String("syslog-receiver1"),
* Syslog: &monitor.SyslogReceiverArgs{
* Endpoint: pulumi.String("0.0.0.0:514"),
* },
* Type: pulumi.String(monitor.ReceiverTypeSyslog),
* },
* },
* ResourceGroupName: pulumi.String("myResourceGroup"),
* Service: &monitor.ServiceArgs{
* Pipelines: monitor.PipelineArray{
* &monitor.PipelineArgs{
* Exporters: pulumi.StringArray{
* pulumi.String("my-workspace-logs-exporter1"),
* },
* Name: pulumi.String("MyPipelineForLogs1"),
* Processors: pulumi.StringArray{
* pulumi.String("batch-processor1"),
* },
* Receivers: pulumi.StringArray{
* pulumi.String("syslog-receiver1"),
* },
* Type: pulumi.String(monitor.PipelineTypeLogs),
* },
* },
* },
* Tags: pulumi.StringMap{
* "tag1": pulumi.String("A"),
* "tag2": pulumi.String("B"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.monitor.PipelineGroup;
* import com.pulumi.azurenative.monitor.PipelineGroupArgs;
* import com.pulumi.azurenative.monitor.inputs.ExporterArgs;
* import com.pulumi.azurenative.monitor.inputs.AzureMonitorWorkspaceLogsExporterArgs;
* import com.pulumi.azurenative.monitor.inputs.AzureMonitorWorkspaceLogsApiConfigArgs;
* import com.pulumi.azurenative.monitor.inputs.SchemaMapArgs;
* import com.pulumi.azurenative.monitor.inputs.ConcurrencyConfigurationArgs;
* import com.pulumi.azurenative.monitor.inputs.AzureResourceManagerCommonTypesExtendedLocationArgs;
* import com.pulumi.azurenative.monitor.inputs.ProcessorArgs;
* import com.pulumi.azurenative.monitor.inputs.ReceiverArgs;
* import com.pulumi.azurenative.monitor.inputs.SyslogReceiverArgs;
* import com.pulumi.azurenative.monitor.inputs.ServiceArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var pipelineGroup = new PipelineGroup("pipelineGroup", PipelineGroupArgs.builder()
* .exporters(ExporterArgs.builder()
* .azureMonitorWorkspaceLogs(AzureMonitorWorkspaceLogsExporterArgs.builder()
* .api(AzureMonitorWorkspaceLogsApiConfigArgs.builder()
* .dataCollectionEndpointUrl("https://logs-myingestion-eb0s.eastus-1.ingest.monitor.azure.com")
* .dataCollectionRule("dcr-00000000000000000000000000000000")
* .schema(SchemaMapArgs.builder()
* .recordMap(
* RecordMapArgs.builder()
* .from("body")
* .to("Body")
* .build(),
* RecordMapArgs.builder()
* .from("severity_text")
* .to("SeverityText")
* .build(),
* RecordMapArgs.builder()
* .from("time_unix_nano")
* .to("TimeGenerated")
* .build())
* .build())
* .stream("Custom-MyTableRawData_CL")
* .build())
* .concurrency(ConcurrencyConfigurationArgs.builder()
* .batchQueueSize(100)
* .workerCount(4)
* .build())
* .build())
* .name("my-workspace-logs-exporter1")
* .type("AzureMonitorWorkspaceLogs")
* .build())
* .extendedLocation(AzureResourceManagerCommonTypesExtendedLocationArgs.builder()
* .name("/subscriptions/00000000-0000-0000-0000-000000000000/resourcegroups/myResourceGroup/providers/microsoft.extendedlocation/customlocations/myTestCustomLocation")
* .type("CustomLocation")
* .build())
* .location("eastus2")
* .pipelineGroupName("plGroup1")
* .processors(ProcessorArgs.builder()
* .name("batch-processor1")
* .type("Batch")
* .build())
* .receivers(ReceiverArgs.builder()
* .name("syslog-receiver1")
* .syslog(SyslogReceiverArgs.builder()
* .endpoint("0.0.0.0:514")
* .build())
* .type("Syslog")
* .build())
* .resourceGroupName("myResourceGroup")
* .service(ServiceArgs.builder()
* .pipelines(PipelineArgs.builder()
* .exporters("my-workspace-logs-exporter1")
* .name("MyPipelineForLogs1")
* .processors("batch-processor1")
* .receivers("syslog-receiver1")
* .type("logs")
* .build())
* .build())
* .tags(Map.ofEntries(
* Map.entry("tag1", "A"),
* Map.entry("tag2", "B")
* ))
* .build());
* }
* }
* ```
* ### Create a PipelineGroup instance using a syslog receiver and cache.
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var pipelineGroup = new AzureNative.Monitor.PipelineGroup("pipelineGroup", new()
* {
* Exporters = new[]
* {
* new AzureNative.Monitor.Inputs.ExporterArgs
* {
* AzureMonitorWorkspaceLogs = new AzureNative.Monitor.Inputs.AzureMonitorWorkspaceLogsExporterArgs
* {
* Api = new AzureNative.Monitor.Inputs.AzureMonitorWorkspaceLogsApiConfigArgs
* {
* DataCollectionEndpointUrl = "https://logs-myingestion-eb0s.eastus-1.ingest.monitor.azure.com",
* DataCollectionRule = "dcr-00000000000000000000000000000000",
* Schema = new AzureNative.Monitor.Inputs.SchemaMapArgs
* {
* RecordMap = new[]
* {
* new AzureNative.Monitor.Inputs.RecordMapArgs
* {
* From = "body",
* To = "Body",
* },
* new AzureNative.Monitor.Inputs.RecordMapArgs
* {
* From = "severity_text",
* To = "SeverityText",
* },
* new AzureNative.Monitor.Inputs.RecordMapArgs
* {
* From = "time_unix_nano",
* To = "TimeGenerated",
* },
* },
* },
* Stream = "Custom-MyTableRawData_CL",
* },
* Cache = new AzureNative.Monitor.Inputs.CacheConfigurationArgs
* {
* MaxStorageUsage = 100,
* RetentionPeriod = 10,
* },
* Concurrency = new AzureNative.Monitor.Inputs.ConcurrencyConfigurationArgs
* {
* BatchQueueSize = 100,
* WorkerCount = 4,
* },
* },
* Name = "my-workspace-logs-exporter1",
* Type = AzureNative.Monitor.ExporterType.AzureMonitorWorkspaceLogs,
* },
* },
* ExtendedLocation = new AzureNative.Monitor.Inputs.AzureResourceManagerCommonTypesExtendedLocationArgs
* {
* Name = "/subscriptions/00000000-0000-0000-0000-000000000000/resourcegroups/myResourceGroup/providers/microsoft.extendedlocation/customlocations/myTestCustomLocation",
* Type = AzureNative.Monitor.ExtendedLocationType.CustomLocation,
* },
* Location = "eastus2",
* PipelineGroupName = "plGroup1",
* Processors = new[]
* {
* new AzureNative.Monitor.Inputs.ProcessorArgs
* {
* Name = "batch-processor1",
* Type = AzureNative.Monitor.ProcessorType.Batch,
* },
* },
* Receivers = new[]
* {
* new AzureNative.Monitor.Inputs.ReceiverArgs
* {
* Name = "syslog-receiver1",
* Syslog = new AzureNative.Monitor.Inputs.SyslogReceiverArgs
* {
* Endpoint = "0.0.0.0:514",
* },
* Type = AzureNative.Monitor.ReceiverType.Syslog,
* },
* },
* ResourceGroupName = "myResourceGroup",
* Service = new AzureNative.Monitor.Inputs.ServiceArgs
* {
* Pipelines = new[]
* {
* new AzureNative.Monitor.Inputs.PipelineArgs
* {
* Exporters = new[]
* {
* "my-workspace-logs-exporter1",
* },
* Name = "MyPipelineForLogs1",
* Processors = new[]
* {
* "batch-processor1",
* },
* Receivers = new[]
* {
* "syslog-receiver1",
* },
* Type = AzureNative.Monitor.PipelineType.Logs,
* },
* },
* },
* Tags =
* {
* { "tag1", "A" },
* { "tag2", "B" },
* },
* });
* });
* ```
* ```go
* package main
* import (
* monitor "github.com/pulumi/pulumi-azure-native-sdk/monitor/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := monitor.NewPipelineGroup(ctx, "pipelineGroup", &monitor.PipelineGroupArgs{
* Exporters: monitor.ExporterArray{
* &monitor.ExporterArgs{
* AzureMonitorWorkspaceLogs: &monitor.AzureMonitorWorkspaceLogsExporterArgs{
* Api: &monitor.AzureMonitorWorkspaceLogsApiConfigArgs{
* DataCollectionEndpointUrl: pulumi.String("https://logs-myingestion-eb0s.eastus-1.ingest.monitor.azure.com"),
* DataCollectionRule: pulumi.String("dcr-00000000000000000000000000000000"),
* Schema: &monitor.SchemaMapArgs{
* RecordMap: monitor.RecordMapArray{
* &monitor.RecordMapArgs{
* From: pulumi.String("body"),
* To: pulumi.String("Body"),
* },
* &monitor.RecordMapArgs{
* From: pulumi.String("severity_text"),
* To: pulumi.String("SeverityText"),
* },
* &monitor.RecordMapArgs{
* From: pulumi.String("time_unix_nano"),
* To: pulumi.String("TimeGenerated"),
* },
* },
* },
* Stream: pulumi.String("Custom-MyTableRawData_CL"),
* },
* Cache: &monitor.CacheConfigurationArgs{
* MaxStorageUsage: pulumi.Int(100),
* RetentionPeriod: pulumi.Int(10),
* },
* Concurrency: &monitor.ConcurrencyConfigurationArgs{
* BatchQueueSize: pulumi.Int(100),
* WorkerCount: pulumi.Int(4),
* },
* },
* Name: pulumi.String("my-workspace-logs-exporter1"),
* Type: pulumi.String(monitor.ExporterTypeAzureMonitorWorkspaceLogs),
* },
* },
* ExtendedLocation: &monitor.AzureResourceManagerCommonTypesExtendedLocationArgs{
* Name: pulumi.String("/subscriptions/00000000-0000-0000-0000-000000000000/resourcegroups/myResourceGroup/providers/microsoft.extendedlocation/customlocations/myTestCustomLocation"),
* Type: pulumi.String(monitor.ExtendedLocationTypeCustomLocation),
* },
* Location: pulumi.String("eastus2"),
* PipelineGroupName: pulumi.String("plGroup1"),
* Processors: monitor.ProcessorArray{
* &monitor.ProcessorArgs{
* Name: pulumi.String("batch-processor1"),
* Type: pulumi.String(monitor.ProcessorTypeBatch),
* },
* },
* Receivers: monitor.ReceiverArray{
* &monitor.ReceiverArgs{
* Name: pulumi.String("syslog-receiver1"),
* Syslog: &monitor.SyslogReceiverArgs{
* Endpoint: pulumi.String("0.0.0.0:514"),
* },
* Type: pulumi.String(monitor.ReceiverTypeSyslog),
* },
* },
* ResourceGroupName: pulumi.String("myResourceGroup"),
* Service: &monitor.ServiceArgs{
* Pipelines: monitor.PipelineArray{
* &monitor.PipelineArgs{
* Exporters: pulumi.StringArray{
* pulumi.String("my-workspace-logs-exporter1"),
* },
* Name: pulumi.String("MyPipelineForLogs1"),
* Processors: pulumi.StringArray{
* pulumi.String("batch-processor1"),
* },
* Receivers: pulumi.StringArray{
* pulumi.String("syslog-receiver1"),
* },
* Type: pulumi.String(monitor.PipelineTypeLogs),
* },
* },
* },
* Tags: pulumi.StringMap{
* "tag1": pulumi.String("A"),
* "tag2": pulumi.String("B"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.monitor.PipelineGroup;
* import com.pulumi.azurenative.monitor.PipelineGroupArgs;
* import com.pulumi.azurenative.monitor.inputs.ExporterArgs;
* import com.pulumi.azurenative.monitor.inputs.AzureMonitorWorkspaceLogsExporterArgs;
* import com.pulumi.azurenative.monitor.inputs.AzureMonitorWorkspaceLogsApiConfigArgs;
* import com.pulumi.azurenative.monitor.inputs.SchemaMapArgs;
* import com.pulumi.azurenative.monitor.inputs.CacheConfigurationArgs;
* import com.pulumi.azurenative.monitor.inputs.ConcurrencyConfigurationArgs;
* import com.pulumi.azurenative.monitor.inputs.AzureResourceManagerCommonTypesExtendedLocationArgs;
* import com.pulumi.azurenative.monitor.inputs.ProcessorArgs;
* import com.pulumi.azurenative.monitor.inputs.ReceiverArgs;
* import com.pulumi.azurenative.monitor.inputs.SyslogReceiverArgs;
* import com.pulumi.azurenative.monitor.inputs.ServiceArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var pipelineGroup = new PipelineGroup("pipelineGroup", PipelineGroupArgs.builder()
* .exporters(ExporterArgs.builder()
* .azureMonitorWorkspaceLogs(AzureMonitorWorkspaceLogsExporterArgs.builder()
* .api(AzureMonitorWorkspaceLogsApiConfigArgs.builder()
* .dataCollectionEndpointUrl("https://logs-myingestion-eb0s.eastus-1.ingest.monitor.azure.com")
* .dataCollectionRule("dcr-00000000000000000000000000000000")
* .schema(SchemaMapArgs.builder()
* .recordMap(
* RecordMapArgs.builder()
* .from("body")
* .to("Body")
* .build(),
* RecordMapArgs.builder()
* .from("severity_text")
* .to("SeverityText")
* .build(),
* RecordMapArgs.builder()
* .from("time_unix_nano")
* .to("TimeGenerated")
* .build())
* .build())
* .stream("Custom-MyTableRawData_CL")
* .build())
* .cache(CacheConfigurationArgs.builder()
* .maxStorageUsage(100)
* .retentionPeriod(10)
* .build())
* .concurrency(ConcurrencyConfigurationArgs.builder()
* .batchQueueSize(100)
* .workerCount(4)
* .build())
* .build())
* .name("my-workspace-logs-exporter1")
* .type("AzureMonitorWorkspaceLogs")
* .build())
* .extendedLocation(AzureResourceManagerCommonTypesExtendedLocationArgs.builder()
* .name("/subscriptions/00000000-0000-0000-0000-000000000000/resourcegroups/myResourceGroup/providers/microsoft.extendedlocation/customlocations/myTestCustomLocation")
* .type("CustomLocation")
* .build())
* .location("eastus2")
* .pipelineGroupName("plGroup1")
* .processors(ProcessorArgs.builder()
* .name("batch-processor1")
* .type("Batch")
* .build())
* .receivers(ReceiverArgs.builder()
* .name("syslog-receiver1")
* .syslog(SyslogReceiverArgs.builder()
* .endpoint("0.0.0.0:514")
* .build())
* .type("Syslog")
* .build())
* .resourceGroupName("myResourceGroup")
* .service(ServiceArgs.builder()
* .pipelines(PipelineArgs.builder()
* .exporters("my-workspace-logs-exporter1")
* .name("MyPipelineForLogs1")
* .processors("batch-processor1")
* .receivers("syslog-receiver1")
* .type("logs")
* .build())
* .build())
* .tags(Map.ofEntries(
* Map.entry("tag1", "A"),
* Map.entry("tag2", "B")
* ))
* .build());
* }
* }
* ```
* ### Create a PipelineGroup instance using a syslog receiver and networking configurations.
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var pipelineGroup = new AzureNative.Monitor.PipelineGroup("pipelineGroup", new()
* {
* Exporters = new[]
* {
* new AzureNative.Monitor.Inputs.ExporterArgs
* {
* AzureMonitorWorkspaceLogs = new AzureNative.Monitor.Inputs.AzureMonitorWorkspaceLogsExporterArgs
* {
* Api = new AzureNative.Monitor.Inputs.AzureMonitorWorkspaceLogsApiConfigArgs
* {
* DataCollectionEndpointUrl = "https://logs-myingestion-eb0s.eastus-1.ingest.monitor.azure.com",
* DataCollectionRule = "dcr-00000000000000000000000000000000",
* Schema = new AzureNative.Monitor.Inputs.SchemaMapArgs
* {
* RecordMap = new[]
* {
* new AzureNative.Monitor.Inputs.RecordMapArgs
* {
* From = "body",
* To = "Body",
* },
* new AzureNative.Monitor.Inputs.RecordMapArgs
* {
* From = "severity_text",
* To = "SeverityText",
* },
* new AzureNative.Monitor.Inputs.RecordMapArgs
* {
* From = "time_unix_nano",
* To = "TimeGenerated",
* },
* },
* },
* Stream = "Custom-MyTableRawData_CL",
* },
* Concurrency = new AzureNative.Monitor.Inputs.ConcurrencyConfigurationArgs
* {
* BatchQueueSize = 100,
* WorkerCount = 4,
* },
* },
* Name = "my-workspace-logs-exporter1",
* Type = AzureNative.Monitor.ExporterType.AzureMonitorWorkspaceLogs,
* },
* },
* ExtendedLocation = new AzureNative.Monitor.Inputs.AzureResourceManagerCommonTypesExtendedLocationArgs
* {
* Name = "/subscriptions/00000000-0000-0000-0000-000000000000/resourcegroups/myResourceGroup/providers/microsoft.extendedlocation/customlocations/myTestCustomLocation",
* Type = AzureNative.Monitor.ExtendedLocationType.CustomLocation,
* },
* Location = "eastus2",
* NetworkingConfigurations = new[]
* {
* new AzureNative.Monitor.Inputs.NetworkingConfigurationArgs
* {
* ExternalNetworkingMode = AzureNative.Monitor.ExternalNetworkingMode.LoadBalancerOnly,
* Host = "azuremonitorpipeline.contoso.com",
* Routes = new[]
* {
* new AzureNative.Monitor.Inputs.NetworkingRouteArgs
* {
* Receiver = "syslog-receiver1",
* },
* },
* },
* },
* PipelineGroupName = "plGroup1",
* Processors = new[] {},
* Receivers = new[]
* {
* new AzureNative.Monitor.Inputs.ReceiverArgs
* {
* Name = "syslog-receiver1",
* Syslog = new AzureNative.Monitor.Inputs.SyslogReceiverArgs
* {
* Endpoint = "0.0.0.0:514",
* },
* Type = AzureNative.Monitor.ReceiverType.Syslog,
* },
* },
* ResourceGroupName = "myResourceGroup",
* Service = new AzureNative.Monitor.Inputs.ServiceArgs
* {
* Pipelines = new[]
* {
* new AzureNative.Monitor.Inputs.PipelineArgs
* {
* Exporters = new[]
* {
* "my-workspace-logs-exporter1",
* },
* Name = "MyPipelineForLogs1",
* Processors = new() { },
* Receivers = new[]
* {
* "syslog-receiver1",
* },
* Type = AzureNative.Monitor.PipelineType.Logs,
* },
* },
* },
* Tags =
* {
* { "tag1", "A" },
* { "tag2", "B" },
* },
* });
* });
* ```
* ```go
* package main
* import (
* monitor "github.com/pulumi/pulumi-azure-native-sdk/monitor/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := monitor.NewPipelineGroup(ctx, "pipelineGroup", &monitor.PipelineGroupArgs{
* Exporters: monitor.ExporterArray{
* &monitor.ExporterArgs{
* AzureMonitorWorkspaceLogs: &monitor.AzureMonitorWorkspaceLogsExporterArgs{
* Api: &monitor.AzureMonitorWorkspaceLogsApiConfigArgs{
* DataCollectionEndpointUrl: pulumi.String("https://logs-myingestion-eb0s.eastus-1.ingest.monitor.azure.com"),
* DataCollectionRule: pulumi.String("dcr-00000000000000000000000000000000"),
* Schema: &monitor.SchemaMapArgs{
* RecordMap: monitor.RecordMapArray{
* &monitor.RecordMapArgs{
* From: pulumi.String("body"),
* To: pulumi.String("Body"),
* },
* &monitor.RecordMapArgs{
* From: pulumi.String("severity_text"),
* To: pulumi.String("SeverityText"),
* },
* &monitor.RecordMapArgs{
* From: pulumi.String("time_unix_nano"),
* To: pulumi.String("TimeGenerated"),
* },
* },
* },
* Stream: pulumi.String("Custom-MyTableRawData_CL"),
* },
* Concurrency: &monitor.ConcurrencyConfigurationArgs{
* BatchQueueSize: pulumi.Int(100),
* WorkerCount: pulumi.Int(4),
* },
* },
* Name: pulumi.String("my-workspace-logs-exporter1"),
* Type: pulumi.String(monitor.ExporterTypeAzureMonitorWorkspaceLogs),
* },
* },
* ExtendedLocation: &monitor.AzureResourceManagerCommonTypesExtendedLocationArgs{
* Name: pulumi.String("/subscriptions/00000000-0000-0000-0000-000000000000/resourcegroups/myResourceGroup/providers/microsoft.extendedlocation/customlocations/myTestCustomLocation"),
* Type: pulumi.String(monitor.ExtendedLocationTypeCustomLocation),
* },
* Location: pulumi.String("eastus2"),
* NetworkingConfigurations: monitor.NetworkingConfigurationArray{
* &monitor.NetworkingConfigurationArgs{
* ExternalNetworkingMode: pulumi.String(monitor.ExternalNetworkingModeLoadBalancerOnly),
* Host: pulumi.String("azuremonitorpipeline.contoso.com"),
* Routes: monitor.NetworkingRouteArray{
* &monitor.NetworkingRouteArgs{
* Receiver: pulumi.String("syslog-receiver1"),
* },
* },
* },
* },
* PipelineGroupName: pulumi.String("plGroup1"),
* Processors: monitor.ProcessorArray{},
* Receivers: monitor.ReceiverArray{
* &monitor.ReceiverArgs{
* Name: pulumi.String("syslog-receiver1"),
* Syslog: &monitor.SyslogReceiverArgs{
* Endpoint: pulumi.String("0.0.0.0:514"),
* },
* Type: pulumi.String(monitor.ReceiverTypeSyslog),
* },
* },
* ResourceGroupName: pulumi.String("myResourceGroup"),
* Service: &monitor.ServiceArgs{
* Pipelines: monitor.PipelineArray{
* &monitor.PipelineArgs{
* Exporters: pulumi.StringArray{
* pulumi.String("my-workspace-logs-exporter1"),
* },
* Name: pulumi.String("MyPipelineForLogs1"),
* Processors: pulumi.StringArray{},
* Receivers: pulumi.StringArray{
* pulumi.String("syslog-receiver1"),
* },
* Type: pulumi.String(monitor.PipelineTypeLogs),
* },
* },
* },
* Tags: pulumi.StringMap{
* "tag1": pulumi.String("A"),
* "tag2": pulumi.String("B"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.monitor.PipelineGroup;
* import com.pulumi.azurenative.monitor.PipelineGroupArgs;
* import com.pulumi.azurenative.monitor.inputs.ExporterArgs;
* import com.pulumi.azurenative.monitor.inputs.AzureMonitorWorkspaceLogsExporterArgs;
* import com.pulumi.azurenative.monitor.inputs.AzureMonitorWorkspaceLogsApiConfigArgs;
* import com.pulumi.azurenative.monitor.inputs.SchemaMapArgs;
* import com.pulumi.azurenative.monitor.inputs.ConcurrencyConfigurationArgs;
* import com.pulumi.azurenative.monitor.inputs.AzureResourceManagerCommonTypesExtendedLocationArgs;
* import com.pulumi.azurenative.monitor.inputs.NetworkingConfigurationArgs;
* import com.pulumi.azurenative.monitor.inputs.ReceiverArgs;
* import com.pulumi.azurenative.monitor.inputs.SyslogReceiverArgs;
* import com.pulumi.azurenative.monitor.inputs.ServiceArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var pipelineGroup = new PipelineGroup("pipelineGroup", PipelineGroupArgs.builder()
* .exporters(ExporterArgs.builder()
* .azureMonitorWorkspaceLogs(AzureMonitorWorkspaceLogsExporterArgs.builder()
* .api(AzureMonitorWorkspaceLogsApiConfigArgs.builder()
* .dataCollectionEndpointUrl("https://logs-myingestion-eb0s.eastus-1.ingest.monitor.azure.com")
* .dataCollectionRule("dcr-00000000000000000000000000000000")
* .schema(SchemaMapArgs.builder()
* .recordMap(
* RecordMapArgs.builder()
* .from("body")
* .to("Body")
* .build(),
* RecordMapArgs.builder()
* .from("severity_text")
* .to("SeverityText")
* .build(),
* RecordMapArgs.builder()
* .from("time_unix_nano")
* .to("TimeGenerated")
* .build())
* .build())
* .stream("Custom-MyTableRawData_CL")
* .build())
* .concurrency(ConcurrencyConfigurationArgs.builder()
* .batchQueueSize(100)
* .workerCount(4)
* .build())
* .build())
* .name("my-workspace-logs-exporter1")
* .type("AzureMonitorWorkspaceLogs")
* .build())
* .extendedLocation(AzureResourceManagerCommonTypesExtendedLocationArgs.builder()
* .name("/subscriptions/00000000-0000-0000-0000-000000000000/resourcegroups/myResourceGroup/providers/microsoft.extendedlocation/customlocations/myTestCustomLocation")
* .type("CustomLocation")
* .build())
* .location("eastus2")
* .networkingConfigurations(NetworkingConfigurationArgs.builder()
* .externalNetworkingMode("LoadBalancerOnly")
* .host("azuremonitorpipeline.contoso.com")
* .routes(NetworkingRouteArgs.builder()
* .receiver("syslog-receiver1")
* .build())
* .build())
* .pipelineGroupName("plGroup1")
* .processors()
* .receivers(ReceiverArgs.builder()
* .name("syslog-receiver1")
* .syslog(SyslogReceiverArgs.builder()
* .endpoint("0.0.0.0:514")
* .build())
* .type("Syslog")
* .build())
* .resourceGroupName("myResourceGroup")
* .service(ServiceArgs.builder()
* .pipelines(PipelineArgs.builder()
* .exporters("my-workspace-logs-exporter1")
* .name("MyPipelineForLogs1")
* .processors()
* .receivers("syslog-receiver1")
* .type("logs")
* .build())
* .build())
* .tags(Map.ofEntries(
* Map.entry("tag1", "A"),
* Map.entry("tag2", "B")
* ))
* .build());
* }
* }
* ```
* ## Import
* An existing resource can be imported using its type token, name, and identifier, e.g.
* ```sh
* $ pulumi import azure-native:monitor:PipelineGroup plGroup1 /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Monitor/pipelineGroups/{pipelineGroupName}
* ```
* @property exporters The exporters specified for a pipeline group instance.
* @property extendedLocation The extended location for given pipeline group.
* @property location The geo-location where the resource lives
* @property networkingConfigurations Networking configurations for the pipeline group instance.
* @property pipelineGroupName The name of pipeline group. The name is case insensitive.
* @property processors The processors specified for a pipeline group instance.
* @property receivers The receivers specified for a pipeline group instance.
* @property replicas Defines the amount of replicas of the pipeline group instance.
* @property resourceGroupName The name of the resource group. The name is case insensitive.
* @property service The service section for a given pipeline group instance.
* @property tags Resource tags.
*/
public data class PipelineGroupArgs(
public val exporters: Output>? = null,
public val extendedLocation: Output? = null,
public val location: Output? = null,
public val networkingConfigurations: Output>? = null,
public val pipelineGroupName: Output? = null,
public val processors: Output>? = null,
public val receivers: Output>? = null,
public val replicas: Output? = null,
public val resourceGroupName: Output? = null,
public val service: Output? = null,
public val tags: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy