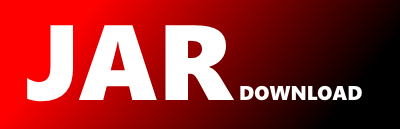
com.pulumi.azurenative.monitor.kotlin.inputs.PipelineArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.monitor.kotlin.inputs
import com.pulumi.azurenative.monitor.inputs.PipelineArgs.builder
import com.pulumi.azurenative.monitor.kotlin.enums.PipelineType
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Pipeline Info.
* @property exporters Reference to exporters configured for the pipeline.
* @property name Name of the pipeline.
* @property processors Reference to processors configured for the pipeline.
* @property receivers Reference to receivers configured for the pipeline.
* @property type The type of pipeline
*/
public data class PipelineArgs(
public val exporters: Output>,
public val name: Output,
public val processors: Output>? = null,
public val receivers: Output>,
public val type: Output>,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.monitor.inputs.PipelineArgs =
com.pulumi.azurenative.monitor.inputs.PipelineArgs.builder()
.exporters(exporters.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.name(name.applyValue({ args0 -> args0 }))
.processors(processors?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.receivers(receivers.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.type(
type.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
).build()
}
/**
* Builder for [PipelineArgs].
*/
@PulumiTagMarker
public class PipelineArgsBuilder internal constructor() {
private var exporters: Output>? = null
private var name: Output? = null
private var processors: Output>? = null
private var receivers: Output>? = null
private var type: Output>? = null
/**
* @param value Reference to exporters configured for the pipeline.
*/
@JvmName("widgoywekdlvpude")
public suspend fun exporters(`value`: Output>) {
this.exporters = value
}
@JvmName("jphudgysbleerxmd")
public suspend fun exporters(vararg values: Output) {
this.exporters = Output.all(values.asList())
}
/**
* @param values Reference to exporters configured for the pipeline.
*/
@JvmName("whghbilvvmistsgh")
public suspend fun exporters(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy