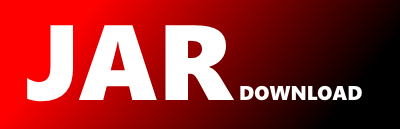
com.pulumi.azurenative.netapp.kotlin.outputs.ActiveDirectoryResponse.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.netapp.kotlin.outputs
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
/**
* Active Directory
* @property activeDirectoryId Id of the Active Directory
* @property adName Name of the active directory machine. This optional parameter is used only while creating kerberos volume
* @property administrators Users to be added to the Built-in Administrators active directory group. A list of unique usernames without domain specifier
* @property aesEncryption If enabled, AES encryption will be enabled for SMB communication.
* @property allowLocalNfsUsersWithLdap If enabled, NFS client local users can also (in addition to LDAP users) access the NFS volumes.
* @property backupOperators Users to be added to the Built-in Backup Operator active directory group. A list of unique usernames without domain specifier
* @property dns Comma separated list of DNS server IP addresses (IPv4 only) for the Active Directory domain
* @property domain Name of the Active Directory domain
* @property encryptDCConnections If enabled, Traffic between the SMB server to Domain Controller (DC) will be encrypted.
* @property kdcIP kdc server IP addresses for the active directory machine. This optional parameter is used only while creating kerberos volume.
* @property ldapOverTLS Specifies whether or not the LDAP traffic needs to be secured via TLS.
* @property ldapSearchScope LDAP Search scope options
* @property ldapSigning Specifies whether or not the LDAP traffic needs to be signed.
* @property organizationalUnit The Organizational Unit (OU) within the Windows Active Directory
* @property password Plain text password of Active Directory domain administrator, value is masked in the response
* @property preferredServersForLdapClient Comma separated list of IPv4 addresses of preferred servers for LDAP client. At most two comma separated IPv4 addresses can be passed.
* @property securityOperators Domain Users in the Active directory to be given SeSecurityPrivilege privilege (Needed for SMB Continuously available shares for SQL). A list of unique usernames without domain specifier
* @property serverRootCACertificate When LDAP over SSL/TLS is enabled, the LDAP client is required to have base64 encoded Active Directory Certificate Service's self-signed root CA certificate, this optional parameter is used only for dual protocol with LDAP user-mapping volumes.
* @property site The Active Directory site the service will limit Domain Controller discovery to
* @property smbServerName NetBIOS name of the SMB server. This name will be registered as a computer account in the AD and used to mount volumes
* @property status Status of the Active Directory
* @property statusDetails Any details in regards to the Status of the Active Directory
* @property username A domain user account with permission to create machine accounts
*/
public data class ActiveDirectoryResponse(
public val activeDirectoryId: String? = null,
public val adName: String? = null,
public val administrators: List? = null,
public val aesEncryption: Boolean? = null,
public val allowLocalNfsUsersWithLdap: Boolean? = null,
public val backupOperators: List? = null,
public val dns: String? = null,
public val domain: String? = null,
public val encryptDCConnections: Boolean? = null,
public val kdcIP: String? = null,
public val ldapOverTLS: Boolean? = null,
public val ldapSearchScope: LdapSearchScopeOptResponse? = null,
public val ldapSigning: Boolean? = null,
public val organizationalUnit: String? = null,
public val password: String? = null,
public val preferredServersForLdapClient: String? = null,
public val securityOperators: List? = null,
public val serverRootCACertificate: String? = null,
public val site: String? = null,
public val smbServerName: String? = null,
public val status: String,
public val statusDetails: String,
public val username: String? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.azurenative.netapp.outputs.ActiveDirectoryResponse): ActiveDirectoryResponse = ActiveDirectoryResponse(
activeDirectoryId = javaType.activeDirectoryId().map({ args0 -> args0 }).orElse(null),
adName = javaType.adName().map({ args0 -> args0 }).orElse(null),
administrators = javaType.administrators().map({ args0 -> args0 }),
aesEncryption = javaType.aesEncryption().map({ args0 -> args0 }).orElse(null),
allowLocalNfsUsersWithLdap = javaType.allowLocalNfsUsersWithLdap().map({ args0 ->
args0
}).orElse(null),
backupOperators = javaType.backupOperators().map({ args0 -> args0 }),
dns = javaType.dns().map({ args0 -> args0 }).orElse(null),
domain = javaType.domain().map({ args0 -> args0 }).orElse(null),
encryptDCConnections = javaType.encryptDCConnections().map({ args0 -> args0 }).orElse(null),
kdcIP = javaType.kdcIP().map({ args0 -> args0 }).orElse(null),
ldapOverTLS = javaType.ldapOverTLS().map({ args0 -> args0 }).orElse(null),
ldapSearchScope = javaType.ldapSearchScope().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.netapp.kotlin.outputs.LdapSearchScopeOptResponse.Companion.toKotlin(args0)
})
}).orElse(null),
ldapSigning = javaType.ldapSigning().map({ args0 -> args0 }).orElse(null),
organizationalUnit = javaType.organizationalUnit().map({ args0 -> args0 }).orElse(null),
password = javaType.password().map({ args0 -> args0 }).orElse(null),
preferredServersForLdapClient = javaType.preferredServersForLdapClient().map({ args0 ->
args0
}).orElse(null),
securityOperators = javaType.securityOperators().map({ args0 -> args0 }),
serverRootCACertificate = javaType.serverRootCACertificate().map({ args0 -> args0 }).orElse(null),
site = javaType.site().map({ args0 -> args0 }).orElse(null),
smbServerName = javaType.smbServerName().map({ args0 -> args0 }).orElse(null),
status = javaType.status(),
statusDetails = javaType.statusDetails(),
username = javaType.username().map({ args0 -> args0 }).orElse(null),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy