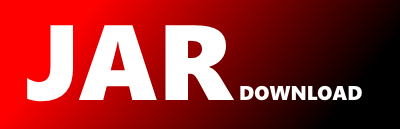
com.pulumi.azurenative.network.kotlin.ExpressRouteCrossConnectionPeeringArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.network.kotlin
import com.pulumi.azurenative.network.ExpressRouteCrossConnectionPeeringArgs.builder
import com.pulumi.azurenative.network.kotlin.enums.ExpressRoutePeeringState
import com.pulumi.azurenative.network.kotlin.enums.ExpressRoutePeeringType
import com.pulumi.azurenative.network.kotlin.inputs.ExpressRouteCircuitPeeringConfigArgs
import com.pulumi.azurenative.network.kotlin.inputs.ExpressRouteCircuitPeeringConfigArgsBuilder
import com.pulumi.azurenative.network.kotlin.inputs.Ipv6ExpressRouteCircuitPeeringConfigArgs
import com.pulumi.azurenative.network.kotlin.inputs.Ipv6ExpressRouteCircuitPeeringConfigArgsBuilder
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Double
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Peering in an ExpressRoute Cross Connection resource.
* Azure REST API version: 2023-02-01. Prior API version in Azure Native 1.x: 2020-11-01.
* Other available API versions: 2019-08-01, 2023-04-01, 2023-05-01, 2023-06-01, 2023-09-01, 2023-11-01, 2024-01-01.
* ## Example Usage
* ### ExpressRouteCrossConnectionBgpPeeringCreate
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var expressRouteCrossConnectionPeering = new AzureNative.Network.ExpressRouteCrossConnectionPeering("expressRouteCrossConnectionPeering", new()
* {
* CrossConnectionName = "",
* Ipv6PeeringConfig = new AzureNative.Network.Inputs.Ipv6ExpressRouteCircuitPeeringConfigArgs
* {
* PrimaryPeerAddressPrefix = "3FFE:FFFF:0:CD30::/126",
* SecondaryPeerAddressPrefix = "3FFE:FFFF:0:CD30::4/126",
* },
* PeerASN = 200,
* PeeringName = "AzurePrivatePeering",
* PrimaryPeerAddressPrefix = "192.168.16.252/30",
* ResourceGroupName = "CrossConnection-SiliconValley",
* SecondaryPeerAddressPrefix = "192.168.18.252/30",
* VlanId = 200,
* });
* });
* ```
* ```go
* package main
* import (
* network "github.com/pulumi/pulumi-azure-native-sdk/network/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := network.NewExpressRouteCrossConnectionPeering(ctx, "expressRouteCrossConnectionPeering", &network.ExpressRouteCrossConnectionPeeringArgs{
* CrossConnectionName: pulumi.String(""),
* Ipv6PeeringConfig: &network.Ipv6ExpressRouteCircuitPeeringConfigArgs{
* PrimaryPeerAddressPrefix: pulumi.String("3FFE:FFFF:0:CD30::/126"),
* SecondaryPeerAddressPrefix: pulumi.String("3FFE:FFFF:0:CD30::4/126"),
* },
* PeerASN: pulumi.Float64(200),
* PeeringName: pulumi.String("AzurePrivatePeering"),
* PrimaryPeerAddressPrefix: pulumi.String("192.168.16.252/30"),
* ResourceGroupName: pulumi.String("CrossConnection-SiliconValley"),
* SecondaryPeerAddressPrefix: pulumi.String("192.168.18.252/30"),
* VlanId: pulumi.Int(200),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.network.ExpressRouteCrossConnectionPeering;
* import com.pulumi.azurenative.network.ExpressRouteCrossConnectionPeeringArgs;
* import com.pulumi.azurenative.network.inputs.Ipv6ExpressRouteCircuitPeeringConfigArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var expressRouteCrossConnectionPeering = new ExpressRouteCrossConnectionPeering("expressRouteCrossConnectionPeering", ExpressRouteCrossConnectionPeeringArgs.builder()
* .crossConnectionName("")
* .ipv6PeeringConfig(Ipv6ExpressRouteCircuitPeeringConfigArgs.builder()
* .primaryPeerAddressPrefix("3FFE:FFFF:0:CD30::/126")
* .secondaryPeerAddressPrefix("3FFE:FFFF:0:CD30::4/126")
* .build())
* .peerASN(200)
* .peeringName("AzurePrivatePeering")
* .primaryPeerAddressPrefix("192.168.16.252/30")
* .resourceGroupName("CrossConnection-SiliconValley")
* .secondaryPeerAddressPrefix("192.168.18.252/30")
* .vlanId(200)
* .build());
* }
* }
* ```
* ## Import
* An existing resource can be imported using its type token, name, and identifier, e.g.
* ```sh
* $ pulumi import azure-native:network:ExpressRouteCrossConnectionPeering AzurePrivatePeering /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Network/expressRouteCrossConnections/{crossConnectionName}/peerings/{peeringName}
* ```
* @property crossConnectionName The name of the ExpressRouteCrossConnection.
* @property gatewayManagerEtag The GatewayManager Etag.
* @property id Resource ID.
* @property ipv6PeeringConfig The IPv6 peering configuration.
* @property microsoftPeeringConfig The Microsoft peering configuration.
* @property name The name of the resource that is unique within a resource group. This name can be used to access the resource.
* @property peerASN The peer ASN.
* @property peeringName The name of the peering.
* @property peeringType The peering type.
* @property primaryPeerAddressPrefix The primary address prefix.
* @property resourceGroupName The name of the resource group.
* @property secondaryPeerAddressPrefix The secondary address prefix.
* @property sharedKey The shared key.
* @property state The peering state.
* @property vlanId The VLAN ID.
*/
public data class ExpressRouteCrossConnectionPeeringArgs(
public val crossConnectionName: Output? = null,
public val gatewayManagerEtag: Output? = null,
public val id: Output? = null,
public val ipv6PeeringConfig: Output? = null,
public val microsoftPeeringConfig: Output? = null,
public val name: Output? = null,
public val peerASN: Output? = null,
public val peeringName: Output? = null,
public val peeringType: Output>? = null,
public val primaryPeerAddressPrefix: Output? = null,
public val resourceGroupName: Output? = null,
public val secondaryPeerAddressPrefix: Output? = null,
public val sharedKey: Output? = null,
public val state: Output>? = null,
public val vlanId: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.network.ExpressRouteCrossConnectionPeeringArgs =
com.pulumi.azurenative.network.ExpressRouteCrossConnectionPeeringArgs.builder()
.crossConnectionName(crossConnectionName?.applyValue({ args0 -> args0 }))
.gatewayManagerEtag(gatewayManagerEtag?.applyValue({ args0 -> args0 }))
.id(id?.applyValue({ args0 -> args0 }))
.ipv6PeeringConfig(ipv6PeeringConfig?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.microsoftPeeringConfig(
microsoftPeeringConfig?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.name(name?.applyValue({ args0 -> args0 }))
.peerASN(peerASN?.applyValue({ args0 -> args0 }))
.peeringName(peeringName?.applyValue({ args0 -> args0 }))
.peeringType(
peeringType?.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.primaryPeerAddressPrefix(primaryPeerAddressPrefix?.applyValue({ args0 -> args0 }))
.resourceGroupName(resourceGroupName?.applyValue({ args0 -> args0 }))
.secondaryPeerAddressPrefix(secondaryPeerAddressPrefix?.applyValue({ args0 -> args0 }))
.sharedKey(sharedKey?.applyValue({ args0 -> args0 }))
.state(
state?.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.vlanId(vlanId?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ExpressRouteCrossConnectionPeeringArgs].
*/
@PulumiTagMarker
public class ExpressRouteCrossConnectionPeeringArgsBuilder internal constructor() {
private var crossConnectionName: Output? = null
private var gatewayManagerEtag: Output? = null
private var id: Output? = null
private var ipv6PeeringConfig: Output? = null
private var microsoftPeeringConfig: Output? = null
private var name: Output? = null
private var peerASN: Output? = null
private var peeringName: Output? = null
private var peeringType: Output>? = null
private var primaryPeerAddressPrefix: Output? = null
private var resourceGroupName: Output? = null
private var secondaryPeerAddressPrefix: Output? = null
private var sharedKey: Output? = null
private var state: Output>? = null
private var vlanId: Output? = null
/**
* @param value The name of the ExpressRouteCrossConnection.
*/
@JvmName("nvfvfsrmfjapcfpj")
public suspend fun crossConnectionName(`value`: Output) {
this.crossConnectionName = value
}
/**
* @param value The GatewayManager Etag.
*/
@JvmName("dyisrmreuwjexlne")
public suspend fun gatewayManagerEtag(`value`: Output) {
this.gatewayManagerEtag = value
}
/**
* @param value Resource ID.
*/
@JvmName("hedtpbhxohmmhjdt")
public suspend fun id(`value`: Output) {
this.id = value
}
/**
* @param value The IPv6 peering configuration.
*/
@JvmName("kxskyxmqgkbvihtn")
public suspend fun ipv6PeeringConfig(`value`: Output) {
this.ipv6PeeringConfig = value
}
/**
* @param value The Microsoft peering configuration.
*/
@JvmName("nonjtkcxsqitpeus")
public suspend fun microsoftPeeringConfig(`value`: Output) {
this.microsoftPeeringConfig = value
}
/**
* @param value The name of the resource that is unique within a resource group. This name can be used to access the resource.
*/
@JvmName("ohypueeqvorjqxpu")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value The peer ASN.
*/
@JvmName("yiwhtecyydfjqcio")
public suspend fun peerASN(`value`: Output) {
this.peerASN = value
}
/**
* @param value The name of the peering.
*/
@JvmName("vnkbbuadbjndfuso")
public suspend fun peeringName(`value`: Output) {
this.peeringName = value
}
/**
* @param value The peering type.
*/
@JvmName("obhiakdctjtidfsm")
public suspend fun peeringType(`value`: Output>) {
this.peeringType = value
}
/**
* @param value The primary address prefix.
*/
@JvmName("eriyjwmehwnpenav")
public suspend fun primaryPeerAddressPrefix(`value`: Output) {
this.primaryPeerAddressPrefix = value
}
/**
* @param value The name of the resource group.
*/
@JvmName("fwbqmtpnsivuelbo")
public suspend fun resourceGroupName(`value`: Output) {
this.resourceGroupName = value
}
/**
* @param value The secondary address prefix.
*/
@JvmName("gxrdcfyfucnntkjs")
public suspend fun secondaryPeerAddressPrefix(`value`: Output) {
this.secondaryPeerAddressPrefix = value
}
/**
* @param value The shared key.
*/
@JvmName("rillunhkmoorpctk")
public suspend fun sharedKey(`value`: Output) {
this.sharedKey = value
}
/**
* @param value The peering state.
*/
@JvmName("ivryurydjnhoodum")
public suspend fun state(`value`: Output>) {
this.state = value
}
/**
* @param value The VLAN ID.
*/
@JvmName("gyucgxphnfaowaue")
public suspend fun vlanId(`value`: Output) {
this.vlanId = value
}
/**
* @param value The name of the ExpressRouteCrossConnection.
*/
@JvmName("gynhmskvrnmqiqrb")
public suspend fun crossConnectionName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.crossConnectionName = mapped
}
/**
* @param value The GatewayManager Etag.
*/
@JvmName("vupxausoyrwdwpkj")
public suspend fun gatewayManagerEtag(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.gatewayManagerEtag = mapped
}
/**
* @param value Resource ID.
*/
@JvmName("kpphajrfwwmfpwcc")
public suspend fun id(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.id = mapped
}
/**
* @param value The IPv6 peering configuration.
*/
@JvmName("sfyjuwfqobxxboif")
public suspend fun ipv6PeeringConfig(`value`: Ipv6ExpressRouteCircuitPeeringConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.ipv6PeeringConfig = mapped
}
/**
* @param argument The IPv6 peering configuration.
*/
@JvmName("tfupjwkbferbkyji")
public suspend fun ipv6PeeringConfig(argument: suspend Ipv6ExpressRouteCircuitPeeringConfigArgsBuilder.() -> Unit) {
val toBeMapped = Ipv6ExpressRouteCircuitPeeringConfigArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.ipv6PeeringConfig = mapped
}
/**
* @param value The Microsoft peering configuration.
*/
@JvmName("vgeesvecuwmlqivv")
public suspend fun microsoftPeeringConfig(`value`: ExpressRouteCircuitPeeringConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.microsoftPeeringConfig = mapped
}
/**
* @param argument The Microsoft peering configuration.
*/
@JvmName("uubgokenltxwdxom")
public suspend fun microsoftPeeringConfig(argument: suspend ExpressRouteCircuitPeeringConfigArgsBuilder.() -> Unit) {
val toBeMapped = ExpressRouteCircuitPeeringConfigArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.microsoftPeeringConfig = mapped
}
/**
* @param value The name of the resource that is unique within a resource group. This name can be used to access the resource.
*/
@JvmName("unpwbtfiwybnqcnw")
public suspend fun name(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value The peer ASN.
*/
@JvmName("rssqhlhdmfvidcdn")
public suspend fun peerASN(`value`: Double?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.peerASN = mapped
}
/**
* @param value The name of the peering.
*/
@JvmName("xwbbbsbinkurtvqk")
public suspend fun peeringName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.peeringName = mapped
}
/**
* @param value The peering type.
*/
@JvmName("ctfnyejbmalcnylm")
public suspend fun peeringType(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.peeringType = mapped
}
/**
* @param value The peering type.
*/
@JvmName("uskpfrirdfkyxwrb")
public fun peeringType(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.peeringType = mapped
}
/**
* @param value The peering type.
*/
@JvmName("dwwirvdgkvadynrp")
public fun peeringType(`value`: ExpressRoutePeeringType) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.peeringType = mapped
}
/**
* @param value The primary address prefix.
*/
@JvmName("mvqyqtutjftiddks")
public suspend fun primaryPeerAddressPrefix(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.primaryPeerAddressPrefix = mapped
}
/**
* @param value The name of the resource group.
*/
@JvmName("uweotsabtmxkjpet")
public suspend fun resourceGroupName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.resourceGroupName = mapped
}
/**
* @param value The secondary address prefix.
*/
@JvmName("fnmmusggtxujcnnf")
public suspend fun secondaryPeerAddressPrefix(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.secondaryPeerAddressPrefix = mapped
}
/**
* @param value The shared key.
*/
@JvmName("oswswbycjwlfnkyy")
public suspend fun sharedKey(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sharedKey = mapped
}
/**
* @param value The peering state.
*/
@JvmName("sjkxuhegoynpxydq")
public suspend fun state(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.state = mapped
}
/**
* @param value The peering state.
*/
@JvmName("jmkqefmggvsrewnj")
public fun state(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.state = mapped
}
/**
* @param value The peering state.
*/
@JvmName("gbdsekagnvquvecn")
public fun state(`value`: ExpressRoutePeeringState) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.state = mapped
}
/**
* @param value The VLAN ID.
*/
@JvmName("mtuphkmbvbnmgfiu")
public suspend fun vlanId(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.vlanId = mapped
}
internal fun build(): ExpressRouteCrossConnectionPeeringArgs =
ExpressRouteCrossConnectionPeeringArgs(
crossConnectionName = crossConnectionName,
gatewayManagerEtag = gatewayManagerEtag,
id = id,
ipv6PeeringConfig = ipv6PeeringConfig,
microsoftPeeringConfig = microsoftPeeringConfig,
name = name,
peerASN = peerASN,
peeringName = peeringName,
peeringType = peeringType,
primaryPeerAddressPrefix = primaryPeerAddressPrefix,
resourceGroupName = resourceGroupName,
secondaryPeerAddressPrefix = secondaryPeerAddressPrefix,
sharedKey = sharedKey,
state = state,
vlanId = vlanId,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy