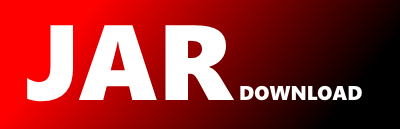
com.pulumi.azurenative.network.kotlin.VirtualNetworkGateway.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.network.kotlin
import com.pulumi.azurenative.network.kotlin.outputs.AddressSpaceResponse
import com.pulumi.azurenative.network.kotlin.outputs.BgpSettingsResponse
import com.pulumi.azurenative.network.kotlin.outputs.ExtendedLocationResponse
import com.pulumi.azurenative.network.kotlin.outputs.SubResourceResponse
import com.pulumi.azurenative.network.kotlin.outputs.VirtualNetworkGatewayIPConfigurationResponse
import com.pulumi.azurenative.network.kotlin.outputs.VirtualNetworkGatewayNatRuleResponse
import com.pulumi.azurenative.network.kotlin.outputs.VirtualNetworkGatewayPolicyGroupResponse
import com.pulumi.azurenative.network.kotlin.outputs.VirtualNetworkGatewaySkuResponse
import com.pulumi.azurenative.network.kotlin.outputs.VpnClientConfigurationResponse
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import com.pulumi.azurenative.network.kotlin.outputs.AddressSpaceResponse.Companion.toKotlin as addressSpaceResponseToKotlin
import com.pulumi.azurenative.network.kotlin.outputs.BgpSettingsResponse.Companion.toKotlin as bgpSettingsResponseToKotlin
import com.pulumi.azurenative.network.kotlin.outputs.ExtendedLocationResponse.Companion.toKotlin as extendedLocationResponseToKotlin
import com.pulumi.azurenative.network.kotlin.outputs.SubResourceResponse.Companion.toKotlin as subResourceResponseToKotlin
import com.pulumi.azurenative.network.kotlin.outputs.VirtualNetworkGatewayIPConfigurationResponse.Companion.toKotlin as virtualNetworkGatewayIPConfigurationResponseToKotlin
import com.pulumi.azurenative.network.kotlin.outputs.VirtualNetworkGatewayNatRuleResponse.Companion.toKotlin as virtualNetworkGatewayNatRuleResponseToKotlin
import com.pulumi.azurenative.network.kotlin.outputs.VirtualNetworkGatewayPolicyGroupResponse.Companion.toKotlin as virtualNetworkGatewayPolicyGroupResponseToKotlin
import com.pulumi.azurenative.network.kotlin.outputs.VirtualNetworkGatewaySkuResponse.Companion.toKotlin as virtualNetworkGatewaySkuResponseToKotlin
import com.pulumi.azurenative.network.kotlin.outputs.VpnClientConfigurationResponse.Companion.toKotlin as vpnClientConfigurationResponseToKotlin
/**
* Builder for [VirtualNetworkGateway].
*/
@PulumiTagMarker
public class VirtualNetworkGatewayResourceBuilder internal constructor() {
public var name: String? = null
public var args: VirtualNetworkGatewayArgs = VirtualNetworkGatewayArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend VirtualNetworkGatewayArgsBuilder.() -> Unit) {
val builder = VirtualNetworkGatewayArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): VirtualNetworkGateway {
val builtJavaResource =
com.pulumi.azurenative.network.VirtualNetworkGateway(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return VirtualNetworkGateway(builtJavaResource)
}
}
/**
* A common class for general resource information.
* Azure REST API version: 2023-02-01. Prior API version in Azure Native 1.x: 2020-11-01.
* Other available API versions: 2016-06-01, 2016-09-01, 2019-08-01, 2023-04-01, 2023-05-01, 2023-06-01, 2023-09-01, 2023-11-01, 2024-01-01.
* ## Example Usage
* ### UpdateVirtualNetworkGateway
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var virtualNetworkGateway = new AzureNative.Network.VirtualNetworkGateway("virtualNetworkGateway", new()
* {
* ActiveActive = false,
* AllowRemoteVnetTraffic = false,
* AllowVirtualWanTraffic = false,
* BgpSettings = new AzureNative.Network.Inputs.BgpSettingsArgs
* {
* Asn = 65515,
* BgpPeeringAddress = "10.0.1.30",
* PeerWeight = 0,
* },
* CustomRoutes = new AzureNative.Network.Inputs.AddressSpaceArgs
* {
* AddressPrefixes = new[]
* {
* "101.168.0.6/32",
* },
* },
* DisableIPSecReplayProtection = false,
* EnableBgp = false,
* EnableBgpRouteTranslationForNat = false,
* EnableDnsForwarding = true,
* GatewayType = AzureNative.Network.VirtualNetworkGatewayType.Vpn,
* IpConfigurations = new[]
* {
* new AzureNative.Network.Inputs.VirtualNetworkGatewayIPConfigurationArgs
* {
* Name = "gwipconfig1",
* PrivateIPAllocationMethod = AzureNative.Network.IPAllocationMethod.Dynamic,
* PublicIPAddress = new AzureNative.Network.Inputs.SubResourceArgs
* {
* Id = "/subscriptions/subid/resourceGroups/rg1/providers/Microsoft.Network/publicIPAddresses/gwpip",
* },
* Subnet = new AzureNative.Network.Inputs.SubResourceArgs
* {
* Id = "/subscriptions/subid/resourceGroups/rg1/providers/Microsoft.Network/virtualNetworks/vnet1/subnets/GatewaySubnet",
* },
* },
* },
* Location = "centralus",
* NatRules = new[]
* {
* new AzureNative.Network.Inputs.VirtualNetworkGatewayNatRuleArgs
* {
* ExternalMappings = new[]
* {
* new AzureNative.Network.Inputs.VpnNatRuleMappingArgs
* {
* AddressSpace = "50.0.0.0/24",
* },
* },
* Id = "/subscriptions/subid/resourceGroups/rg1/providers/Microsoft.Network/virtualNetworkGateways/vpngw/natRules/natRule1",
* InternalMappings = new[]
* {
* new AzureNative.Network.Inputs.VpnNatRuleMappingArgs
* {
* AddressSpace = "10.10.0.0/24",
* },
* },
* IpConfigurationId = "",
* Mode = AzureNative.Network.VpnNatRuleMode.EgressSnat,
* Name = "natRule1",
* Type = AzureNative.Network.VpnNatRuleType.Static,
* },
* new AzureNative.Network.Inputs.VirtualNetworkGatewayNatRuleArgs
* {
* ExternalMappings = new[]
* {
* new AzureNative.Network.Inputs.VpnNatRuleMappingArgs
* {
* AddressSpace = "30.0.0.0/24",
* },
* },
* Id = "/subscriptions/subid/resourceGroups/rg1/providers/Microsoft.Network/virtualNetworkGateways/vpngw/natRules/natRule2",
* InternalMappings = new[]
* {
* new AzureNative.Network.Inputs.VpnNatRuleMappingArgs
* {
* AddressSpace = "20.10.0.0/24",
* },
* },
* IpConfigurationId = "",
* Mode = AzureNative.Network.VpnNatRuleMode.IngressSnat,
* Name = "natRule2",
* Type = AzureNative.Network.VpnNatRuleType.Static,
* },
* },
* ResourceGroupName = "rg1",
* Sku = new AzureNative.Network.Inputs.VirtualNetworkGatewaySkuArgs
* {
* Name = AzureNative.Network.VirtualNetworkGatewaySkuName.VpnGw1,
* Tier = AzureNative.Network.VirtualNetworkGatewaySkuTier.VpnGw1,
* },
* VirtualNetworkGatewayName = "vpngw",
* VpnClientConfiguration = new AzureNative.Network.Inputs.VpnClientConfigurationArgs
* {
* RadiusServers = new[]
* {
* new AzureNative.Network.Inputs.RadiusServerArgs
* {
* RadiusServerAddress = "10.2.0.0",
* RadiusServerScore = 20,
* RadiusServerSecret = "radiusServerSecret",
* },
* },
* VpnClientProtocols = new[]
* {
* AzureNative.Network.VpnClientProtocol.OpenVPN,
* },
* VpnClientRevokedCertificates = new() { },
* VpnClientRootCertificates = new() { },
* },
* VpnType = AzureNative.Network.VpnType.RouteBased,
* });
* });
* ```
* ```go
* package main
* import (
* network "github.com/pulumi/pulumi-azure-native-sdk/network/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := network.NewVirtualNetworkGateway(ctx, "virtualNetworkGateway", &network.VirtualNetworkGatewayArgs{
* ActiveActive: pulumi.Bool(false),
* AllowRemoteVnetTraffic: pulumi.Bool(false),
* AllowVirtualWanTraffic: pulumi.Bool(false),
* BgpSettings: &network.BgpSettingsArgs{
* Asn: pulumi.Float64(65515),
* BgpPeeringAddress: pulumi.String("10.0.1.30"),
* PeerWeight: pulumi.Int(0),
* },
* CustomRoutes: &network.AddressSpaceArgs{
* AddressPrefixes: pulumi.StringArray{
* pulumi.String("101.168.0.6/32"),
* },
* },
* DisableIPSecReplayProtection: pulumi.Bool(false),
* EnableBgp: pulumi.Bool(false),
* EnableBgpRouteTranslationForNat: pulumi.Bool(false),
* EnableDnsForwarding: pulumi.Bool(true),
* GatewayType: pulumi.String(network.VirtualNetworkGatewayTypeVpn),
* IpConfigurations: network.VirtualNetworkGatewayIPConfigurationArray{
* &network.VirtualNetworkGatewayIPConfigurationArgs{
* Name: pulumi.String("gwipconfig1"),
* PrivateIPAllocationMethod: pulumi.String(network.IPAllocationMethodDynamic),
* PublicIPAddress: &network.SubResourceArgs{
* Id: pulumi.String("/subscriptions/subid/resourceGroups/rg1/providers/Microsoft.Network/publicIPAddresses/gwpip"),
* },
* Subnet: &network.SubResourceArgs{
* Id: pulumi.String("/subscriptions/subid/resourceGroups/rg1/providers/Microsoft.Network/virtualNetworks/vnet1/subnets/GatewaySubnet"),
* },
* },
* },
* Location: pulumi.String("centralus"),
* NatRules: network.VirtualNetworkGatewayNatRuleTypeArray{
* &network.VirtualNetworkGatewayNatRuleTypeArgs{
* ExternalMappings: network.VpnNatRuleMappingArray{
* &network.VpnNatRuleMappingArgs{
* AddressSpace: pulumi.String("50.0.0.0/24"),
* },
* },
* Id: pulumi.String("/subscriptions/subid/resourceGroups/rg1/providers/Microsoft.Network/virtualNetworkGateways/vpngw/natRules/natRule1"),
* InternalMappings: network.VpnNatRuleMappingArray{
* &network.VpnNatRuleMappingArgs{
* AddressSpace: pulumi.String("10.10.0.0/24"),
* },
* },
* IpConfigurationId: pulumi.String(""),
* Mode: pulumi.String(network.VpnNatRuleModeEgressSnat),
* Name: pulumi.String("natRule1"),
* Type: pulumi.String(network.VpnNatRuleTypeStatic),
* },
* &network.VirtualNetworkGatewayNatRuleTypeArgs{
* ExternalMappings: network.VpnNatRuleMappingArray{
* &network.VpnNatRuleMappingArgs{
* AddressSpace: pulumi.String("30.0.0.0/24"),
* },
* },
* Id: pulumi.String("/subscriptions/subid/resourceGroups/rg1/providers/Microsoft.Network/virtualNetworkGateways/vpngw/natRules/natRule2"),
* InternalMappings: network.VpnNatRuleMappingArray{
* &network.VpnNatRuleMappingArgs{
* AddressSpace: pulumi.String("20.10.0.0/24"),
* },
* },
* IpConfigurationId: pulumi.String(""),
* Mode: pulumi.String(network.VpnNatRuleModeIngressSnat),
* Name: pulumi.String("natRule2"),
* Type: pulumi.String(network.VpnNatRuleTypeStatic),
* },
* },
* ResourceGroupName: pulumi.String("rg1"),
* Sku: &network.VirtualNetworkGatewaySkuArgs{
* Name: pulumi.String(network.VirtualNetworkGatewaySkuNameVpnGw1),
* Tier: pulumi.String(network.VirtualNetworkGatewaySkuTierVpnGw1),
* },
* VirtualNetworkGatewayName: pulumi.String("vpngw"),
* VpnClientConfiguration: &network.VpnClientConfigurationArgs{
* RadiusServers: network.RadiusServerArray{
* &network.RadiusServerArgs{
* RadiusServerAddress: pulumi.String("10.2.0.0"),
* RadiusServerScore: pulumi.Float64(20),
* RadiusServerSecret: pulumi.String("radiusServerSecret"),
* },
* },
* VpnClientProtocols: pulumi.StringArray{
* pulumi.String(network.VpnClientProtocolOpenVPN),
* },
* VpnClientRevokedCertificates: network.VpnClientRevokedCertificateArray{},
* VpnClientRootCertificates: network.VpnClientRootCertificateArray{},
* },
* VpnType: pulumi.String(network.VpnTypeRouteBased),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.network.VirtualNetworkGateway;
* import com.pulumi.azurenative.network.VirtualNetworkGatewayArgs;
* import com.pulumi.azurenative.network.inputs.BgpSettingsArgs;
* import com.pulumi.azurenative.network.inputs.AddressSpaceArgs;
* import com.pulumi.azurenative.network.inputs.VirtualNetworkGatewayIPConfigurationArgs;
* import com.pulumi.azurenative.network.inputs.SubResourceArgs;
* import com.pulumi.azurenative.network.inputs.VirtualNetworkGatewayNatRuleArgs;
* import com.pulumi.azurenative.network.inputs.VirtualNetworkGatewaySkuArgs;
* import com.pulumi.azurenative.network.inputs.VpnClientConfigurationArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var virtualNetworkGateway = new VirtualNetworkGateway("virtualNetworkGateway", VirtualNetworkGatewayArgs.builder()
* .activeActive(false)
* .allowRemoteVnetTraffic(false)
* .allowVirtualWanTraffic(false)
* .bgpSettings(BgpSettingsArgs.builder()
* .asn(65515)
* .bgpPeeringAddress("10.0.1.30")
* .peerWeight(0)
* .build())
* .customRoutes(AddressSpaceArgs.builder()
* .addressPrefixes("101.168.0.6/32")
* .build())
* .disableIPSecReplayProtection(false)
* .enableBgp(false)
* .enableBgpRouteTranslationForNat(false)
* .enableDnsForwarding(true)
* .gatewayType("Vpn")
* .ipConfigurations(VirtualNetworkGatewayIPConfigurationArgs.builder()
* .name("gwipconfig1")
* .privateIPAllocationMethod("Dynamic")
* .publicIPAddress(SubResourceArgs.builder()
* .id("/subscriptions/subid/resourceGroups/rg1/providers/Microsoft.Network/publicIPAddresses/gwpip")
* .build())
* .subnet(SubResourceArgs.builder()
* .id("/subscriptions/subid/resourceGroups/rg1/providers/Microsoft.Network/virtualNetworks/vnet1/subnets/GatewaySubnet")
* .build())
* .build())
* .location("centralus")
* .natRules(
* VirtualNetworkGatewayNatRuleArgs.builder()
* .externalMappings(VpnNatRuleMappingArgs.builder()
* .addressSpace("50.0.0.0/24")
* .build())
* .id("/subscriptions/subid/resourceGroups/rg1/providers/Microsoft.Network/virtualNetworkGateways/vpngw/natRules/natRule1")
* .internalMappings(VpnNatRuleMappingArgs.builder()
* .addressSpace("10.10.0.0/24")
* .build())
* .ipConfigurationId("")
* .mode("EgressSnat")
* .name("natRule1")
* .type("Static")
* .build(),
* VirtualNetworkGatewayNatRuleArgs.builder()
* .externalMappings(VpnNatRuleMappingArgs.builder()
* .addressSpace("30.0.0.0/24")
* .build())
* .id("/subscriptions/subid/resourceGroups/rg1/providers/Microsoft.Network/virtualNetworkGateways/vpngw/natRules/natRule2")
* .internalMappings(VpnNatRuleMappingArgs.builder()
* .addressSpace("20.10.0.0/24")
* .build())
* .ipConfigurationId("")
* .mode("IngressSnat")
* .name("natRule2")
* .type("Static")
* .build())
* .resourceGroupName("rg1")
* .sku(VirtualNetworkGatewaySkuArgs.builder()
* .name("VpnGw1")
* .tier("VpnGw1")
* .build())
* .virtualNetworkGatewayName("vpngw")
* .vpnClientConfiguration(VpnClientConfigurationArgs.builder()
* .radiusServers(RadiusServerArgs.builder()
* .radiusServerAddress("10.2.0.0")
* .radiusServerScore(20)
* .radiusServerSecret("radiusServerSecret")
* .build())
* .vpnClientProtocols("OpenVPN")
* .vpnClientRevokedCertificates()
* .vpnClientRootCertificates()
* .build())
* .vpnType("RouteBased")
* .build());
* }
* }
* ```
* ## Import
* An existing resource can be imported using its type token, name, and identifier, e.g.
* ```sh
* $ pulumi import azure-native:network:VirtualNetworkGateway vpngw /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Network/virtualNetworkGateways/{virtualNetworkGatewayName}
* ```
*/
public class VirtualNetworkGateway internal constructor(
override val javaResource: com.pulumi.azurenative.network.VirtualNetworkGateway,
) : KotlinCustomResource(javaResource, VirtualNetworkGatewayMapper) {
/**
* ActiveActive flag.
*/
public val activeActive: Output?
get() = javaResource.activeActive().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Property to indicate if the Express Route Gateway serves traffic when there are multiple Express Route Gateways in the vnet
*/
public val adminState: Output?
get() = javaResource.adminState().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Configure this gateway to accept traffic from other Azure Virtual Networks. This configuration does not support connectivity to Azure Virtual WAN.
*/
public val allowRemoteVnetTraffic: Output?
get() = javaResource.allowRemoteVnetTraffic().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Configures this gateway to accept traffic from remote Virtual WAN networks.
*/
public val allowVirtualWanTraffic: Output?
get() = javaResource.allowVirtualWanTraffic().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Virtual network gateway's BGP speaker settings.
*/
public val bgpSettings: Output?
get() = javaResource.bgpSettings().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
bgpSettingsResponseToKotlin(args0)
})
}).orElse(null)
})
/**
* The reference to the address space resource which represents the custom routes address space specified by the customer for virtual network gateway and VpnClient.
*/
public val customRoutes: Output?
get() = javaResource.customRoutes().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
addressSpaceResponseToKotlin(args0)
})
}).orElse(null)
})
/**
* disableIPSecReplayProtection flag.
*/
public val disableIPSecReplayProtection: Output?
get() = javaResource.disableIPSecReplayProtection().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Whether BGP is enabled for this virtual network gateway or not.
*/
public val enableBgp: Output?
get() = javaResource.enableBgp().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* EnableBgpRouteTranslationForNat flag.
*/
public val enableBgpRouteTranslationForNat: Output?
get() = javaResource.enableBgpRouteTranslationForNat().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Whether dns forwarding is enabled or not.
*/
public val enableDnsForwarding: Output?
get() = javaResource.enableDnsForwarding().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Whether private IP needs to be enabled on this gateway for connections or not.
*/
public val enablePrivateIpAddress: Output?
get() = javaResource.enablePrivateIpAddress().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* A unique read-only string that changes whenever the resource is updated.
*/
public val etag: Output
get() = javaResource.etag().applyValue({ args0 -> args0 })
/**
* The extended location of type local virtual network gateway.
*/
public val extendedLocation: Output?
get() = javaResource.extendedLocation().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> extendedLocationResponseToKotlin(args0) })
}).orElse(null)
})
/**
* The reference to the LocalNetworkGateway resource which represents local network site having default routes. Assign Null value in case of removing existing default site setting.
*/
public val gatewayDefaultSite: Output?
get() = javaResource.gatewayDefaultSite().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> subResourceResponseToKotlin(args0) })
}).orElse(null)
})
/**
* The type of this virtual network gateway.
*/
public val gatewayType: Output?
get() = javaResource.gatewayType().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The IP address allocated by the gateway to which dns requests can be sent.
*/
public val inboundDnsForwardingEndpoint: Output
get() = javaResource.inboundDnsForwardingEndpoint().applyValue({ args0 -> args0 })
/**
* IP configurations for virtual network gateway.
*/
public val ipConfigurations: Output>?
get() = javaResource.ipConfigurations().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
virtualNetworkGatewayIPConfigurationResponseToKotlin(args0)
})
})
}).orElse(null)
})
/**
* Resource location.
*/
public val location: Output?
get() = javaResource.location().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* Resource name.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* NatRules for virtual network gateway.
*/
public val natRules: Output>?
get() = javaResource.natRules().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> virtualNetworkGatewayNatRuleResponseToKotlin(args0) })
})
}).orElse(null)
})
/**
* The provisioning state of the virtual network gateway resource.
*/
public val provisioningState: Output
get() = javaResource.provisioningState().applyValue({ args0 -> args0 })
/**
* The resource GUID property of the virtual network gateway resource.
*/
public val resourceGuid: Output
get() = javaResource.resourceGuid().applyValue({ args0 -> args0 })
/**
* The reference to the VirtualNetworkGatewaySku resource which represents the SKU selected for Virtual network gateway.
*/
public val sku: Output?
get() = javaResource.sku().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
virtualNetworkGatewaySkuResponseToKotlin(args0)
})
}).orElse(null)
})
/**
* Resource tags.
*/
public val tags: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy