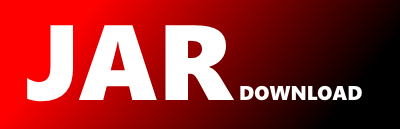
com.pulumi.azurenative.network.kotlin.VirtualNetworkGatewayConnection.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.network.kotlin
import com.pulumi.azurenative.network.kotlin.outputs.GatewayCustomBgpIpAddressIpConfigurationResponse
import com.pulumi.azurenative.network.kotlin.outputs.IpsecPolicyResponse
import com.pulumi.azurenative.network.kotlin.outputs.LocalNetworkGatewayResponse
import com.pulumi.azurenative.network.kotlin.outputs.SubResourceResponse
import com.pulumi.azurenative.network.kotlin.outputs.TrafficSelectorPolicyResponse
import com.pulumi.azurenative.network.kotlin.outputs.TunnelConnectionHealthResponse
import com.pulumi.azurenative.network.kotlin.outputs.VirtualNetworkGatewayResponse
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Double
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import com.pulumi.azurenative.network.kotlin.outputs.GatewayCustomBgpIpAddressIpConfigurationResponse.Companion.toKotlin as gatewayCustomBgpIpAddressIpConfigurationResponseToKotlin
import com.pulumi.azurenative.network.kotlin.outputs.IpsecPolicyResponse.Companion.toKotlin as ipsecPolicyResponseToKotlin
import com.pulumi.azurenative.network.kotlin.outputs.LocalNetworkGatewayResponse.Companion.toKotlin as localNetworkGatewayResponseToKotlin
import com.pulumi.azurenative.network.kotlin.outputs.SubResourceResponse.Companion.toKotlin as subResourceResponseToKotlin
import com.pulumi.azurenative.network.kotlin.outputs.TrafficSelectorPolicyResponse.Companion.toKotlin as trafficSelectorPolicyResponseToKotlin
import com.pulumi.azurenative.network.kotlin.outputs.TunnelConnectionHealthResponse.Companion.toKotlin as tunnelConnectionHealthResponseToKotlin
import com.pulumi.azurenative.network.kotlin.outputs.VirtualNetworkGatewayResponse.Companion.toKotlin as virtualNetworkGatewayResponseToKotlin
/**
* Builder for [VirtualNetworkGatewayConnection].
*/
@PulumiTagMarker
public class VirtualNetworkGatewayConnectionResourceBuilder internal constructor() {
public var name: String? = null
public var args: VirtualNetworkGatewayConnectionArgs = VirtualNetworkGatewayConnectionArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend VirtualNetworkGatewayConnectionArgsBuilder.() -> Unit) {
val builder = VirtualNetworkGatewayConnectionArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): VirtualNetworkGatewayConnection {
val builtJavaResource =
com.pulumi.azurenative.network.VirtualNetworkGatewayConnection(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return VirtualNetworkGatewayConnection(builtJavaResource)
}
}
/**
* A common class for general resource information.
* Azure REST API version: 2023-02-01. Prior API version in Azure Native 1.x: 2020-11-01.
* Other available API versions: 2016-06-01, 2019-08-01, 2023-04-01, 2023-05-01, 2023-06-01, 2023-09-01, 2023-11-01, 2024-01-01.
* ## Example Usage
* ### CreateVirtualNetworkGatewayConnection_S2S
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var virtualNetworkGatewayConnection = new AzureNative.Network.VirtualNetworkGatewayConnection("virtualNetworkGatewayConnection", new()
* {
* ConnectionMode = AzureNative.Network.VirtualNetworkGatewayConnectionMode.Default,
* ConnectionProtocol = AzureNative.Network.VirtualNetworkGatewayConnectionProtocol.IKEv2,
* ConnectionType = AzureNative.Network.VirtualNetworkGatewayConnectionType.IPsec,
* DpdTimeoutSeconds = 30,
* EgressNatRules = new[]
* {
* new AzureNative.Network.Inputs.SubResourceArgs
* {
* Id = "/subscriptions/subid/resourceGroups/rg1/providers/Microsoft.Network/virtualNetworkGateways/vpngw/natRules/natRule2",
* },
* },
* EnableBgp = false,
* GatewayCustomBgpIpAddresses = new[]
* {
* new AzureNative.Network.Inputs.GatewayCustomBgpIpAddressIpConfigurationArgs
* {
* CustomBgpIpAddress = "169.254.21.1",
* IpConfigurationId = "/subscriptions/subid/resourceGroups/rg1/providers/Microsoft.Network/virtualNetworkGateways/vpngw/ipConfigurations/default",
* },
* new AzureNative.Network.Inputs.GatewayCustomBgpIpAddressIpConfigurationArgs
* {
* CustomBgpIpAddress = "169.254.21.3",
* IpConfigurationId = "/subscriptions/subid/resourceGroups/rg1/providers/Microsoft.Network/virtualNetworkGateways/vpngw/ipConfigurations/ActiveActive",
* },
* },
* IngressNatRules = new[]
* {
* new AzureNative.Network.Inputs.SubResourceArgs
* {
* Id = "/subscriptions/subid/resourceGroups/rg1/providers/Microsoft.Network/virtualNetworkGateways/vpngw/natRules/natRule1",
* },
* },
* IpsecPolicies = new[] {},
* LocalNetworkGateway2 = new AzureNative.Network.Inputs.LocalNetworkGatewayArgs
* {
* GatewayIpAddress = "x.x.x.x",
* Id = "/subscriptions/subid/resourceGroups/rg1/providers/Microsoft.Network/localNetworkGateways/localgw",
* LocalNetworkAddressSpace = new AzureNative.Network.Inputs.AddressSpaceArgs
* {
* AddressPrefixes = new[]
* {
* "10.1.0.0/16",
* },
* },
* Location = "centralus",
* Tags = null,
* },
* Location = "centralus",
* ResourceGroupName = "rg1",
* RoutingWeight = 0,
* SharedKey = "Abc123",
* TrafficSelectorPolicies = new[] {},
* UsePolicyBasedTrafficSelectors = false,
* VirtualNetworkGateway1 = new AzureNative.Network.Inputs.VirtualNetworkGatewayArgs
* {
* ActiveActive = false,
* BgpSettings = new AzureNative.Network.Inputs.BgpSettingsArgs
* {
* Asn = 65514,
* BgpPeeringAddress = "10.0.1.30",
* PeerWeight = 0,
* },
* EnableBgp = false,
* GatewayType = AzureNative.Network.VirtualNetworkGatewayType.Vpn,
* Id = "/subscriptions/subid/resourceGroups/rg1/providers/Microsoft.Network/virtualNetworkGateways/vpngw",
* IpConfigurations = new[]
* {
* new AzureNative.Network.Inputs.VirtualNetworkGatewayIPConfigurationArgs
* {
* Id = "/subscriptions/subid/resourceGroups/rg1/providers/Microsoft.Network/virtualNetworkGateways/vpngw/ipConfigurations/gwipconfig1",
* Name = "gwipconfig1",
* PrivateIPAllocationMethod = AzureNative.Network.IPAllocationMethod.Dynamic,
* PublicIPAddress = new AzureNative.Network.Inputs.SubResourceArgs
* {
* Id = "/subscriptions/subid/resourceGroups/rg1/providers/Microsoft.Network/publicIPAddresses/gwpip",
* },
* Subnet = new AzureNative.Network.Inputs.SubResourceArgs
* {
* Id = "/subscriptions/subid/resourceGroups/rg1/providers/Microsoft.Network/virtualNetworks/vnet1/subnets/GatewaySubnet",
* },
* },
* },
* Location = "centralus",
* Sku = new AzureNative.Network.Inputs.VirtualNetworkGatewaySkuArgs
* {
* Name = AzureNative.Network.VirtualNetworkGatewaySkuName.VpnGw1,
* Tier = AzureNative.Network.VirtualNetworkGatewaySkuTier.VpnGw1,
* },
* Tags = null,
* VpnType = AzureNative.Network.VpnType.RouteBased,
* },
* VirtualNetworkGatewayConnectionName = "connS2S",
* });
* });
* ```
* ```go
* package main
* import (
* network "github.com/pulumi/pulumi-azure-native-sdk/network/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := network.NewVirtualNetworkGatewayConnection(ctx, "virtualNetworkGatewayConnection", &network.VirtualNetworkGatewayConnectionArgs{
* ConnectionMode: pulumi.String(network.VirtualNetworkGatewayConnectionModeDefault),
* ConnectionProtocol: pulumi.String(network.VirtualNetworkGatewayConnectionProtocolIKEv2),
* ConnectionType: pulumi.String(network.VirtualNetworkGatewayConnectionTypeIPsec),
* DpdTimeoutSeconds: pulumi.Int(30),
* EgressNatRules: network.SubResourceArray{
* &network.SubResourceArgs{
* Id: pulumi.String("/subscriptions/subid/resourceGroups/rg1/providers/Microsoft.Network/virtualNetworkGateways/vpngw/natRules/natRule2"),
* },
* },
* EnableBgp: pulumi.Bool(false),
* GatewayCustomBgpIpAddresses: network.GatewayCustomBgpIpAddressIpConfigurationArray{
* &network.GatewayCustomBgpIpAddressIpConfigurationArgs{
* CustomBgpIpAddress: pulumi.String("169.254.21.1"),
* IpConfigurationId: pulumi.String("/subscriptions/subid/resourceGroups/rg1/providers/Microsoft.Network/virtualNetworkGateways/vpngw/ipConfigurations/default"),
* },
* &network.GatewayCustomBgpIpAddressIpConfigurationArgs{
* CustomBgpIpAddress: pulumi.String("169.254.21.3"),
* IpConfigurationId: pulumi.String("/subscriptions/subid/resourceGroups/rg1/providers/Microsoft.Network/virtualNetworkGateways/vpngw/ipConfigurations/ActiveActive"),
* },
* },
* IngressNatRules: network.SubResourceArray{
* &network.SubResourceArgs{
* Id: pulumi.String("/subscriptions/subid/resourceGroups/rg1/providers/Microsoft.Network/virtualNetworkGateways/vpngw/natRules/natRule1"),
* },
* },
* IpsecPolicies: network.IpsecPolicyArray{},
* LocalNetworkGateway2: &network.LocalNetworkGatewayTypeArgs{
* GatewayIpAddress: pulumi.String("x.x.x.x"),
* Id: pulumi.String("/subscriptions/subid/resourceGroups/rg1/providers/Microsoft.Network/localNetworkGateways/localgw"),
* LocalNetworkAddressSpace: &network.AddressSpaceArgs{
* AddressPrefixes: pulumi.StringArray{
* pulumi.String("10.1.0.0/16"),
* },
* },
* Location: pulumi.String("centralus"),
* Tags: nil,
* },
* Location: pulumi.String("centralus"),
* ResourceGroupName: pulumi.String("rg1"),
* RoutingWeight: pulumi.Int(0),
* SharedKey: pulumi.String("Abc123"),
* TrafficSelectorPolicies: network.TrafficSelectorPolicyArray{},
* UsePolicyBasedTrafficSelectors: pulumi.Bool(false),
* VirtualNetworkGateway1: &network.VirtualNetworkGatewayTypeArgs{
* ActiveActive: pulumi.Bool(false),
* BgpSettings: &network.BgpSettingsArgs{
* Asn: pulumi.Float64(65514),
* BgpPeeringAddress: pulumi.String("10.0.1.30"),
* PeerWeight: pulumi.Int(0),
* },
* EnableBgp: pulumi.Bool(false),
* GatewayType: pulumi.String(network.VirtualNetworkGatewayTypeVpn),
* Id: pulumi.String("/subscriptions/subid/resourceGroups/rg1/providers/Microsoft.Network/virtualNetworkGateways/vpngw"),
* IpConfigurations: network.VirtualNetworkGatewayIPConfigurationArray{
* &network.VirtualNetworkGatewayIPConfigurationArgs{
* Id: pulumi.String("/subscriptions/subid/resourceGroups/rg1/providers/Microsoft.Network/virtualNetworkGateways/vpngw/ipConfigurations/gwipconfig1"),
* Name: pulumi.String("gwipconfig1"),
* PrivateIPAllocationMethod: pulumi.String(network.IPAllocationMethodDynamic),
* PublicIPAddress: &network.SubResourceArgs{
* Id: pulumi.String("/subscriptions/subid/resourceGroups/rg1/providers/Microsoft.Network/publicIPAddresses/gwpip"),
* },
* Subnet: &network.SubResourceArgs{
* Id: pulumi.String("/subscriptions/subid/resourceGroups/rg1/providers/Microsoft.Network/virtualNetworks/vnet1/subnets/GatewaySubnet"),
* },
* },
* },
* Location: pulumi.String("centralus"),
* Sku: &network.VirtualNetworkGatewaySkuArgs{
* Name: pulumi.String(network.VirtualNetworkGatewaySkuNameVpnGw1),
* Tier: pulumi.String(network.VirtualNetworkGatewaySkuTierVpnGw1),
* },
* Tags: nil,
* VpnType: pulumi.String(network.VpnTypeRouteBased),
* },
* VirtualNetworkGatewayConnectionName: pulumi.String("connS2S"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.network.VirtualNetworkGatewayConnection;
* import com.pulumi.azurenative.network.VirtualNetworkGatewayConnectionArgs;
* import com.pulumi.azurenative.network.inputs.SubResourceArgs;
* import com.pulumi.azurenative.network.inputs.GatewayCustomBgpIpAddressIpConfigurationArgs;
* import com.pulumi.azurenative.network.inputs.LocalNetworkGatewayArgs;
* import com.pulumi.azurenative.network.inputs.AddressSpaceArgs;
* import com.pulumi.azurenative.network.inputs.VirtualNetworkGatewayArgs;
* import com.pulumi.azurenative.network.inputs.BgpSettingsArgs;
* import com.pulumi.azurenative.network.inputs.VirtualNetworkGatewaySkuArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var virtualNetworkGatewayConnection = new VirtualNetworkGatewayConnection("virtualNetworkGatewayConnection", VirtualNetworkGatewayConnectionArgs.builder()
* .connectionMode("Default")
* .connectionProtocol("IKEv2")
* .connectionType("IPsec")
* .dpdTimeoutSeconds(30)
* .egressNatRules(SubResourceArgs.builder()
* .id("/subscriptions/subid/resourceGroups/rg1/providers/Microsoft.Network/virtualNetworkGateways/vpngw/natRules/natRule2")
* .build())
* .enableBgp(false)
* .gatewayCustomBgpIpAddresses(
* GatewayCustomBgpIpAddressIpConfigurationArgs.builder()
* .customBgpIpAddress("169.254.21.1")
* .ipConfigurationId("/subscriptions/subid/resourceGroups/rg1/providers/Microsoft.Network/virtualNetworkGateways/vpngw/ipConfigurations/default")
* .build(),
* GatewayCustomBgpIpAddressIpConfigurationArgs.builder()
* .customBgpIpAddress("169.254.21.3")
* .ipConfigurationId("/subscriptions/subid/resourceGroups/rg1/providers/Microsoft.Network/virtualNetworkGateways/vpngw/ipConfigurations/ActiveActive")
* .build())
* .ingressNatRules(SubResourceArgs.builder()
* .id("/subscriptions/subid/resourceGroups/rg1/providers/Microsoft.Network/virtualNetworkGateways/vpngw/natRules/natRule1")
* .build())
* .ipsecPolicies()
* .localNetworkGateway2(LocalNetworkGatewayArgs.builder()
* .gatewayIpAddress("x.x.x.x")
* .id("/subscriptions/subid/resourceGroups/rg1/providers/Microsoft.Network/localNetworkGateways/localgw")
* .localNetworkAddressSpace(AddressSpaceArgs.builder()
* .addressPrefixes("10.1.0.0/16")
* .build())
* .location("centralus")
* .tags()
* .build())
* .location("centralus")
* .resourceGroupName("rg1")
* .routingWeight(0)
* .sharedKey("Abc123")
* .trafficSelectorPolicies()
* .usePolicyBasedTrafficSelectors(false)
* .virtualNetworkGateway1(VirtualNetworkGatewayArgs.builder()
* .activeActive(false)
* .bgpSettings(BgpSettingsArgs.builder()
* .asn(65514)
* .bgpPeeringAddress("10.0.1.30")
* .peerWeight(0)
* .build())
* .enableBgp(false)
* .gatewayType("Vpn")
* .id("/subscriptions/subid/resourceGroups/rg1/providers/Microsoft.Network/virtualNetworkGateways/vpngw")
* .ipConfigurations(VirtualNetworkGatewayIPConfigurationArgs.builder()
* .id("/subscriptions/subid/resourceGroups/rg1/providers/Microsoft.Network/virtualNetworkGateways/vpngw/ipConfigurations/gwipconfig1")
* .name("gwipconfig1")
* .privateIPAllocationMethod("Dynamic")
* .publicIPAddress(SubResourceArgs.builder()
* .id("/subscriptions/subid/resourceGroups/rg1/providers/Microsoft.Network/publicIPAddresses/gwpip")
* .build())
* .subnet(SubResourceArgs.builder()
* .id("/subscriptions/subid/resourceGroups/rg1/providers/Microsoft.Network/virtualNetworks/vnet1/subnets/GatewaySubnet")
* .build())
* .build())
* .location("centralus")
* .sku(VirtualNetworkGatewaySkuArgs.builder()
* .name("VpnGw1")
* .tier("VpnGw1")
* .build())
* .tags()
* .vpnType("RouteBased")
* .build())
* .virtualNetworkGatewayConnectionName("connS2S")
* .build());
* }
* }
* ```
* ## Import
* An existing resource can be imported using its type token, name, and identifier, e.g.
* ```sh
* $ pulumi import azure-native:network:VirtualNetworkGatewayConnection connS2S /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Network/connections/{virtualNetworkGatewayConnectionName}
* ```
*/
public class VirtualNetworkGatewayConnection internal constructor(
override val javaResource: com.pulumi.azurenative.network.VirtualNetworkGatewayConnection,
) : KotlinCustomResource(javaResource, VirtualNetworkGatewayConnectionMapper) {
/**
* The authorizationKey.
*/
public val authorizationKey: Output?
get() = javaResource.authorizationKey().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The connection mode for this connection.
*/
public val connectionMode: Output?
get() = javaResource.connectionMode().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Connection protocol used for this connection.
*/
public val connectionProtocol: Output?
get() = javaResource.connectionProtocol().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Virtual Network Gateway connection status.
*/
public val connectionStatus: Output
get() = javaResource.connectionStatus().applyValue({ args0 -> args0 })
/**
* Gateway connection type.
*/
public val connectionType: Output
get() = javaResource.connectionType().applyValue({ args0 -> args0 })
/**
* The dead peer detection timeout of this connection in seconds.
*/
public val dpdTimeoutSeconds: Output?
get() = javaResource.dpdTimeoutSeconds().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The egress bytes transferred in this connection.
*/
public val egressBytesTransferred: Output
get() = javaResource.egressBytesTransferred().applyValue({ args0 -> args0 })
/**
* List of egress NatRules.
*/
public val egressNatRules: Output>?
get() = javaResource.egressNatRules().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
subResourceResponseToKotlin(args0)
})
})
}).orElse(null)
})
/**
* EnableBgp flag.
*/
public val enableBgp: Output?
get() = javaResource.enableBgp().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* Bypass the ExpressRoute gateway when accessing private-links. ExpressRoute FastPath (expressRouteGatewayBypass) must be enabled.
*/
public val enablePrivateLinkFastPath: Output?
get() = javaResource.enablePrivateLinkFastPath().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* A unique read-only string that changes whenever the resource is updated.
*/
public val etag: Output
get() = javaResource.etag().applyValue({ args0 -> args0 })
/**
* Bypass ExpressRoute Gateway for data forwarding.
*/
public val expressRouteGatewayBypass: Output?
get() = javaResource.expressRouteGatewayBypass().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* GatewayCustomBgpIpAddresses to be used for virtual network gateway Connection.
*/
public val gatewayCustomBgpIpAddresses:
Output>?
get() = javaResource.gatewayCustomBgpIpAddresses().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
gatewayCustomBgpIpAddressIpConfigurationResponseToKotlin(args0)
})
})
}).orElse(null)
})
/**
* The ingress bytes transferred in this connection.
*/
public val ingressBytesTransferred: Output
get() = javaResource.ingressBytesTransferred().applyValue({ args0 -> args0 })
/**
* List of ingress NatRules.
*/
public val ingressNatRules: Output>?
get() = javaResource.ingressNatRules().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
subResourceResponseToKotlin(args0)
})
})
}).orElse(null)
})
/**
* The IPSec Policies to be considered by this connection.
*/
public val ipsecPolicies: Output>?
get() = javaResource.ipsecPolicies().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
ipsecPolicyResponseToKotlin(args0)
})
})
}).orElse(null)
})
/**
* The reference to local network gateway resource.
*/
public val localNetworkGateway2: Output?
get() = javaResource.localNetworkGateway2().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> localNetworkGatewayResponseToKotlin(args0) })
}).orElse(null)
})
/**
* Resource location.
*/
public val location: Output?
get() = javaResource.location().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* Resource name.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* The reference to peerings resource.
*/
public val peer: Output?
get() = javaResource.peer().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
subResourceResponseToKotlin(args0)
})
}).orElse(null)
})
/**
* The provisioning state of the virtual network gateway connection resource.
*/
public val provisioningState: Output
get() = javaResource.provisioningState().applyValue({ args0 -> args0 })
/**
* The resource GUID property of the virtual network gateway connection resource.
*/
public val resourceGuid: Output
get() = javaResource.resourceGuid().applyValue({ args0 -> args0 })
/**
* The routing weight.
*/
public val routingWeight: Output?
get() = javaResource.routingWeight().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The IPSec shared key.
*/
public val sharedKey: Output?
get() = javaResource.sharedKey().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* Resource tags.
*/
public val tags: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy