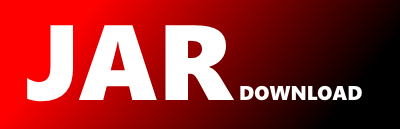
com.pulumi.azurenative.network.kotlin.inputs.ApplicationGatewayProbeArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.network.kotlin.inputs
import com.pulumi.azurenative.network.inputs.ApplicationGatewayProbeArgs.builder
import com.pulumi.azurenative.network.kotlin.enums.ApplicationGatewayProtocol
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Probe of the application gateway.
* @property host Host name to send the probe to.
* @property id Resource ID.
* @property interval The probing interval in seconds. This is the time interval between two consecutive probes. Acceptable values are from 1 second to 86400 seconds.
* @property match Criterion for classifying a healthy probe response.
* @property minServers Minimum number of servers that are always marked healthy. Default value is 0.
* @property name Name of the probe that is unique within an Application Gateway.
* @property path Relative path of probe. Valid path starts from '/'. Probe is sent to ://:.
* @property pickHostNameFromBackendHttpSettings Whether the host header should be picked from the backend http settings. Default value is false.
* @property pickHostNameFromBackendSettings Whether the server name indication should be picked from the backend settings for Tls protocol. Default value is false.
* @property port Custom port which will be used for probing the backend servers. The valid value ranges from 1 to 65535. In case not set, port from http settings will be used. This property is valid for Standard_v2 and WAF_v2 only.
* @property protocol The protocol used for the probe.
* @property timeout The probe timeout in seconds. Probe marked as failed if valid response is not received with this timeout period. Acceptable values are from 1 second to 86400 seconds.
* @property unhealthyThreshold The probe retry count. Backend server is marked down after consecutive probe failure count reaches UnhealthyThreshold. Acceptable values are from 1 second to 20.
*/
public data class ApplicationGatewayProbeArgs(
public val host: Output? = null,
public val id: Output? = null,
public val interval: Output? = null,
public val match: Output? = null,
public val minServers: Output? = null,
public val name: Output? = null,
public val path: Output? = null,
public val pickHostNameFromBackendHttpSettings: Output? = null,
public val pickHostNameFromBackendSettings: Output? = null,
public val port: Output? = null,
public val protocol: Output>? = null,
public val timeout: Output? = null,
public val unhealthyThreshold: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.network.inputs.ApplicationGatewayProbeArgs =
com.pulumi.azurenative.network.inputs.ApplicationGatewayProbeArgs.builder()
.host(host?.applyValue({ args0 -> args0 }))
.id(id?.applyValue({ args0 -> args0 }))
.interval(interval?.applyValue({ args0 -> args0 }))
.match(match?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.minServers(minServers?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.path(path?.applyValue({ args0 -> args0 }))
.pickHostNameFromBackendHttpSettings(
pickHostNameFromBackendHttpSettings?.applyValue({ args0 ->
args0
}),
)
.pickHostNameFromBackendSettings(pickHostNameFromBackendSettings?.applyValue({ args0 -> args0 }))
.port(port?.applyValue({ args0 -> args0 }))
.protocol(
protocol?.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.timeout(timeout?.applyValue({ args0 -> args0 }))
.unhealthyThreshold(unhealthyThreshold?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ApplicationGatewayProbeArgs].
*/
@PulumiTagMarker
public class ApplicationGatewayProbeArgsBuilder internal constructor() {
private var host: Output? = null
private var id: Output? = null
private var interval: Output? = null
private var match: Output? = null
private var minServers: Output? = null
private var name: Output? = null
private var path: Output? = null
private var pickHostNameFromBackendHttpSettings: Output? = null
private var pickHostNameFromBackendSettings: Output? = null
private var port: Output? = null
private var protocol: Output>? = null
private var timeout: Output? = null
private var unhealthyThreshold: Output? = null
/**
* @param value Host name to send the probe to.
*/
@JvmName("gbasvmeiiadnlyki")
public suspend fun host(`value`: Output) {
this.host = value
}
/**
* @param value Resource ID.
*/
@JvmName("cjaupydsgddfdxby")
public suspend fun id(`value`: Output) {
this.id = value
}
/**
* @param value The probing interval in seconds. This is the time interval between two consecutive probes. Acceptable values are from 1 second to 86400 seconds.
*/
@JvmName("luvguibrlvlmrbdp")
public suspend fun interval(`value`: Output) {
this.interval = value
}
/**
* @param value Criterion for classifying a healthy probe response.
*/
@JvmName("uqbvylmrfgiffxpw")
public suspend fun match(`value`: Output) {
this.match = value
}
/**
* @param value Minimum number of servers that are always marked healthy. Default value is 0.
*/
@JvmName("nfsyuslubnldgjtd")
public suspend fun minServers(`value`: Output) {
this.minServers = value
}
/**
* @param value Name of the probe that is unique within an Application Gateway.
*/
@JvmName("etrxpymarkstopir")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value Relative path of probe. Valid path starts from '/'. Probe is sent to ://:.
*/
@JvmName("rutvgbcrnfufudyo")
public suspend fun path(`value`: Output) {
this.path = value
}
/**
* @param value Whether the host header should be picked from the backend http settings. Default value is false.
*/
@JvmName("djcfqdwblvlaealu")
public suspend fun pickHostNameFromBackendHttpSettings(`value`: Output) {
this.pickHostNameFromBackendHttpSettings = value
}
/**
* @param value Whether the server name indication should be picked from the backend settings for Tls protocol. Default value is false.
*/
@JvmName("jvresumdbxojksrt")
public suspend fun pickHostNameFromBackendSettings(`value`: Output) {
this.pickHostNameFromBackendSettings = value
}
/**
* @param value Custom port which will be used for probing the backend servers. The valid value ranges from 1 to 65535. In case not set, port from http settings will be used. This property is valid for Standard_v2 and WAF_v2 only.
*/
@JvmName("fkgbrcgnwfxtlfly")
public suspend fun port(`value`: Output) {
this.port = value
}
/**
* @param value The protocol used for the probe.
*/
@JvmName("opbncbhcmwinjqid")
public suspend fun protocol(`value`: Output>) {
this.protocol = value
}
/**
* @param value The probe timeout in seconds. Probe marked as failed if valid response is not received with this timeout period. Acceptable values are from 1 second to 86400 seconds.
*/
@JvmName("hpbutngmnyvmhlau")
public suspend fun timeout(`value`: Output) {
this.timeout = value
}
/**
* @param value The probe retry count. Backend server is marked down after consecutive probe failure count reaches UnhealthyThreshold. Acceptable values are from 1 second to 20.
*/
@JvmName("lwdaprenfnalhsfp")
public suspend fun unhealthyThreshold(`value`: Output) {
this.unhealthyThreshold = value
}
/**
* @param value Host name to send the probe to.
*/
@JvmName("fwtlutydcfyydddp")
public suspend fun host(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.host = mapped
}
/**
* @param value Resource ID.
*/
@JvmName("qougxvsjhmsmajty")
public suspend fun id(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.id = mapped
}
/**
* @param value The probing interval in seconds. This is the time interval between two consecutive probes. Acceptable values are from 1 second to 86400 seconds.
*/
@JvmName("aamcyfkhlibxmyrm")
public suspend fun interval(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.interval = mapped
}
/**
* @param value Criterion for classifying a healthy probe response.
*/
@JvmName("tmagkrccxvuvjbge")
public suspend fun match(`value`: ApplicationGatewayProbeHealthResponseMatchArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.match = mapped
}
/**
* @param argument Criterion for classifying a healthy probe response.
*/
@JvmName("duxxxtdibgvghlxp")
public suspend fun match(argument: suspend ApplicationGatewayProbeHealthResponseMatchArgsBuilder.() -> Unit) {
val toBeMapped = ApplicationGatewayProbeHealthResponseMatchArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.match = mapped
}
/**
* @param value Minimum number of servers that are always marked healthy. Default value is 0.
*/
@JvmName("jygukwcuyxlculcn")
public suspend fun minServers(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.minServers = mapped
}
/**
* @param value Name of the probe that is unique within an Application Gateway.
*/
@JvmName("ykoixocixvxipocs")
public suspend fun name(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value Relative path of probe. Valid path starts from '/'. Probe is sent to ://:.
*/
@JvmName("kcyuciqdrtirettn")
public suspend fun path(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.path = mapped
}
/**
* @param value Whether the host header should be picked from the backend http settings. Default value is false.
*/
@JvmName("ptxkarnsocpdkvta")
public suspend fun pickHostNameFromBackendHttpSettings(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.pickHostNameFromBackendHttpSettings = mapped
}
/**
* @param value Whether the server name indication should be picked from the backend settings for Tls protocol. Default value is false.
*/
@JvmName("gpafaonsbdwflons")
public suspend fun pickHostNameFromBackendSettings(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.pickHostNameFromBackendSettings = mapped
}
/**
* @param value Custom port which will be used for probing the backend servers. The valid value ranges from 1 to 65535. In case not set, port from http settings will be used. This property is valid for Standard_v2 and WAF_v2 only.
*/
@JvmName("lgbalidxugjqlwtu")
public suspend fun port(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.port = mapped
}
/**
* @param value The protocol used for the probe.
*/
@JvmName("irxrcewvcxrnbcyf")
public suspend fun protocol(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.protocol = mapped
}
/**
* @param value The protocol used for the probe.
*/
@JvmName("mwdwdnkdpcisktla")
public fun protocol(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.protocol = mapped
}
/**
* @param value The protocol used for the probe.
*/
@JvmName("amnxmkensruqvokk")
public fun protocol(`value`: ApplicationGatewayProtocol) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.protocol = mapped
}
/**
* @param value The probe timeout in seconds. Probe marked as failed if valid response is not received with this timeout period. Acceptable values are from 1 second to 86400 seconds.
*/
@JvmName("dloatmbbaklrbtkd")
public suspend fun timeout(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.timeout = mapped
}
/**
* @param value The probe retry count. Backend server is marked down after consecutive probe failure count reaches UnhealthyThreshold. Acceptable values are from 1 second to 20.
*/
@JvmName("hpthkwhxwmpledhu")
public suspend fun unhealthyThreshold(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.unhealthyThreshold = mapped
}
internal fun build(): ApplicationGatewayProbeArgs = ApplicationGatewayProbeArgs(
host = host,
id = id,
interval = interval,
match = match,
minServers = minServers,
name = name,
path = path,
pickHostNameFromBackendHttpSettings = pickHostNameFromBackendHttpSettings,
pickHostNameFromBackendSettings = pickHostNameFromBackendSettings,
port = port,
protocol = protocol,
timeout = timeout,
unhealthyThreshold = unhealthyThreshold,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy