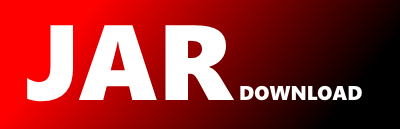
com.pulumi.azurenative.network.kotlin.inputs.FrontendIPConfigurationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.network.kotlin.inputs
import com.pulumi.azurenative.network.inputs.FrontendIPConfigurationArgs.builder
import com.pulumi.azurenative.network.kotlin.enums.IPAllocationMethod
import com.pulumi.azurenative.network.kotlin.enums.IPVersion
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Frontend IP address of the load balancer.
* @property gatewayLoadBalancer The reference to gateway load balancer frontend IP.
* @property id Resource ID.
* @property name The name of the resource that is unique within the set of frontend IP configurations used by the load balancer. This name can be used to access the resource.
* @property privateIPAddress The private IP address of the IP configuration.
* @property privateIPAddressVersion Whether the specific ipconfiguration is IPv4 or IPv6. Default is taken as IPv4.
* @property privateIPAllocationMethod The Private IP allocation method.
* @property publicIPAddress The reference to the Public IP resource.
* @property publicIPPrefix The reference to the Public IP Prefix resource.
* @property subnet The reference to the subnet resource.
* @property zones A list of availability zones denoting the IP allocated for the resource needs to come from.
*/
public data class FrontendIPConfigurationArgs(
public val gatewayLoadBalancer: Output? = null,
public val id: Output? = null,
public val name: Output? = null,
public val privateIPAddress: Output? = null,
public val privateIPAddressVersion: Output>? = null,
public val privateIPAllocationMethod: Output>? = null,
public val publicIPAddress: Output? = null,
public val publicIPPrefix: Output? = null,
public val subnet: Output? = null,
public val zones: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.network.inputs.FrontendIPConfigurationArgs =
com.pulumi.azurenative.network.inputs.FrontendIPConfigurationArgs.builder()
.gatewayLoadBalancer(
gatewayLoadBalancer?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.id(id?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.privateIPAddress(privateIPAddress?.applyValue({ args0 -> args0 }))
.privateIPAddressVersion(
privateIPAddressVersion?.applyValue({ args0 ->
args0.transform({ args0 ->
args0
}, { args0 -> args0.let({ args0 -> args0.toJava() }) })
}),
)
.privateIPAllocationMethod(
privateIPAllocationMethod?.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 -> args0.let({ args0 -> args0.toJava() }) })
}),
)
.publicIPAddress(publicIPAddress?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.publicIPPrefix(publicIPPrefix?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.subnet(subnet?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.zones(zones?.applyValue({ args0 -> args0.map({ args0 -> args0 }) })).build()
}
/**
* Builder for [FrontendIPConfigurationArgs].
*/
@PulumiTagMarker
public class FrontendIPConfigurationArgsBuilder internal constructor() {
private var gatewayLoadBalancer: Output? = null
private var id: Output? = null
private var name: Output? = null
private var privateIPAddress: Output? = null
private var privateIPAddressVersion: Output>? = null
private var privateIPAllocationMethod: Output>? = null
private var publicIPAddress: Output? = null
private var publicIPPrefix: Output? = null
private var subnet: Output? = null
private var zones: Output>? = null
/**
* @param value The reference to gateway load balancer frontend IP.
*/
@JvmName("vlkgcvibfxcydhah")
public suspend fun gatewayLoadBalancer(`value`: Output) {
this.gatewayLoadBalancer = value
}
/**
* @param value Resource ID.
*/
@JvmName("mcyiwtoinvsstlgx")
public suspend fun id(`value`: Output) {
this.id = value
}
/**
* @param value The name of the resource that is unique within the set of frontend IP configurations used by the load balancer. This name can be used to access the resource.
*/
@JvmName("ukjiobcrhwoargfp")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value The private IP address of the IP configuration.
*/
@JvmName("wogcasbrtkalgslo")
public suspend fun privateIPAddress(`value`: Output) {
this.privateIPAddress = value
}
/**
* @param value Whether the specific ipconfiguration is IPv4 or IPv6. Default is taken as IPv4.
*/
@JvmName("wbmjwadhlkqlrwam")
public suspend fun privateIPAddressVersion(`value`: Output>) {
this.privateIPAddressVersion = value
}
/**
* @param value The Private IP allocation method.
*/
@JvmName("nxjtslkdbqvchimg")
public suspend fun privateIPAllocationMethod(`value`: Output>) {
this.privateIPAllocationMethod = value
}
/**
* @param value The reference to the Public IP resource.
*/
@JvmName("beqhohwfyrhwfhom")
public suspend fun publicIPAddress(`value`: Output) {
this.publicIPAddress = value
}
/**
* @param value The reference to the Public IP Prefix resource.
*/
@JvmName("csfldsdcowbhcqpw")
public suspend fun publicIPPrefix(`value`: Output) {
this.publicIPPrefix = value
}
/**
* @param value The reference to the subnet resource.
*/
@JvmName("mscargxequltmivn")
public suspend fun subnet(`value`: Output) {
this.subnet = value
}
/**
* @param value A list of availability zones denoting the IP allocated for the resource needs to come from.
*/
@JvmName("uunlndgtvedbvffs")
public suspend fun zones(`value`: Output>) {
this.zones = value
}
@JvmName("loctdwbgkecqiaax")
public suspend fun zones(vararg values: Output) {
this.zones = Output.all(values.asList())
}
/**
* @param values A list of availability zones denoting the IP allocated for the resource needs to come from.
*/
@JvmName("jivamqljuditpdwx")
public suspend fun zones(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy