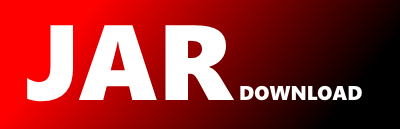
com.pulumi.azurenative.network.kotlin.inputs.RoutingConfigurationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.network.kotlin.inputs
import com.pulumi.azurenative.network.inputs.RoutingConfigurationArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Routing Configuration indicating the associated and propagated route tables for this connection.
* @property associatedRouteTable The resource id RouteTable associated with this RoutingConfiguration.
* @property inboundRouteMap The resource id of the RouteMap associated with this RoutingConfiguration for inbound learned routes.
* @property outboundRouteMap The resource id of theRouteMap associated with this RoutingConfiguration for outbound advertised routes.
* @property propagatedRouteTables The list of RouteTables to advertise the routes to.
* @property vnetRoutes List of routes that control routing from VirtualHub into a virtual network connection.
*/
public data class RoutingConfigurationArgs(
public val associatedRouteTable: Output? = null,
public val inboundRouteMap: Output? = null,
public val outboundRouteMap: Output? = null,
public val propagatedRouteTables: Output? = null,
public val vnetRoutes: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.network.inputs.RoutingConfigurationArgs =
com.pulumi.azurenative.network.inputs.RoutingConfigurationArgs.builder()
.associatedRouteTable(
associatedRouteTable?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.inboundRouteMap(inboundRouteMap?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.outboundRouteMap(outboundRouteMap?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.propagatedRouteTables(
propagatedRouteTables?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.vnetRoutes(vnetRoutes?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [RoutingConfigurationArgs].
*/
@PulumiTagMarker
public class RoutingConfigurationArgsBuilder internal constructor() {
private var associatedRouteTable: Output? = null
private var inboundRouteMap: Output? = null
private var outboundRouteMap: Output? = null
private var propagatedRouteTables: Output? = null
private var vnetRoutes: Output? = null
/**
* @param value The resource id RouteTable associated with this RoutingConfiguration.
*/
@JvmName("bogrylyusbqogqdo")
public suspend fun associatedRouteTable(`value`: Output) {
this.associatedRouteTable = value
}
/**
* @param value The resource id of the RouteMap associated with this RoutingConfiguration for inbound learned routes.
*/
@JvmName("axlxvjdsftbbfiyo")
public suspend fun inboundRouteMap(`value`: Output) {
this.inboundRouteMap = value
}
/**
* @param value The resource id of theRouteMap associated with this RoutingConfiguration for outbound advertised routes.
*/
@JvmName("nvairtrruiyqcqcq")
public suspend fun outboundRouteMap(`value`: Output) {
this.outboundRouteMap = value
}
/**
* @param value The list of RouteTables to advertise the routes to.
*/
@JvmName("rfbfdffjhmpsdrxg")
public suspend fun propagatedRouteTables(`value`: Output) {
this.propagatedRouteTables = value
}
/**
* @param value List of routes that control routing from VirtualHub into a virtual network connection.
*/
@JvmName("ujhnewubtdgqiiif")
public suspend fun vnetRoutes(`value`: Output) {
this.vnetRoutes = value
}
/**
* @param value The resource id RouteTable associated with this RoutingConfiguration.
*/
@JvmName("lskwhoviaoskfbsj")
public suspend fun associatedRouteTable(`value`: SubResourceArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.associatedRouteTable = mapped
}
/**
* @param argument The resource id RouteTable associated with this RoutingConfiguration.
*/
@JvmName("kjamwkdftmbkpuyp")
public suspend fun associatedRouteTable(argument: suspend SubResourceArgsBuilder.() -> Unit) {
val toBeMapped = SubResourceArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.associatedRouteTable = mapped
}
/**
* @param value The resource id of the RouteMap associated with this RoutingConfiguration for inbound learned routes.
*/
@JvmName("enwrmjuqxfwkykjf")
public suspend fun inboundRouteMap(`value`: SubResourceArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.inboundRouteMap = mapped
}
/**
* @param argument The resource id of the RouteMap associated with this RoutingConfiguration for inbound learned routes.
*/
@JvmName("xhpqwjoqqyijtfup")
public suspend fun inboundRouteMap(argument: suspend SubResourceArgsBuilder.() -> Unit) {
val toBeMapped = SubResourceArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.inboundRouteMap = mapped
}
/**
* @param value The resource id of theRouteMap associated with this RoutingConfiguration for outbound advertised routes.
*/
@JvmName("tuiubdvwgbunjjab")
public suspend fun outboundRouteMap(`value`: SubResourceArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.outboundRouteMap = mapped
}
/**
* @param argument The resource id of theRouteMap associated with this RoutingConfiguration for outbound advertised routes.
*/
@JvmName("ebtjohurrevrmibo")
public suspend fun outboundRouteMap(argument: suspend SubResourceArgsBuilder.() -> Unit) {
val toBeMapped = SubResourceArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.outboundRouteMap = mapped
}
/**
* @param value The list of RouteTables to advertise the routes to.
*/
@JvmName("vgrlkvocwkcmdaks")
public suspend fun propagatedRouteTables(`value`: PropagatedRouteTableArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.propagatedRouteTables = mapped
}
/**
* @param argument The list of RouteTables to advertise the routes to.
*/
@JvmName("lsfqfkoatrpaebsr")
public suspend fun propagatedRouteTables(argument: suspend PropagatedRouteTableArgsBuilder.() -> Unit) {
val toBeMapped = PropagatedRouteTableArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.propagatedRouteTables = mapped
}
/**
* @param value List of routes that control routing from VirtualHub into a virtual network connection.
*/
@JvmName("tennkneetadalboo")
public suspend fun vnetRoutes(`value`: VnetRouteArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.vnetRoutes = mapped
}
/**
* @param argument List of routes that control routing from VirtualHub into a virtual network connection.
*/
@JvmName("fdnciyxtunqlljpb")
public suspend fun vnetRoutes(argument: suspend VnetRouteArgsBuilder.() -> Unit) {
val toBeMapped = VnetRouteArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.vnetRoutes = mapped
}
internal fun build(): RoutingConfigurationArgs = RoutingConfigurationArgs(
associatedRouteTable = associatedRouteTable,
inboundRouteMap = inboundRouteMap,
outboundRouteMap = outboundRouteMap,
propagatedRouteTables = propagatedRouteTables,
vnetRoutes = vnetRoutes,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy