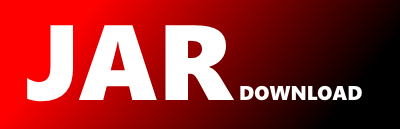
com.pulumi.azurenative.network.kotlin.inputs.RulesEngineActionArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.network.kotlin.inputs
import com.pulumi.azurenative.network.inputs.RulesEngineActionArgs.builder
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* One or more actions that will execute, modifying the request and/or response.
* @property requestHeaderActions A list of header actions to apply from the request from AFD to the origin.
* @property responseHeaderActions A list of header actions to apply from the response from AFD to the client.
* @property routeConfigurationOverride Override the route configuration.
*/
public data class RulesEngineActionArgs(
public val requestHeaderActions: Output>? = null,
public val responseHeaderActions: Output>? = null,
public val routeConfigurationOverride: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.network.inputs.RulesEngineActionArgs =
com.pulumi.azurenative.network.inputs.RulesEngineActionArgs.builder()
.requestHeaderActions(
requestHeaderActions?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.responseHeaderActions(
responseHeaderActions?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.routeConfigurationOverride(
routeConfigurationOverride?.applyValue({ args0 ->
args0.transform({ args0 -> args0.let({ args0 -> args0.toJava() }) }, { args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
).build()
}
/**
* Builder for [RulesEngineActionArgs].
*/
@PulumiTagMarker
public class RulesEngineActionArgsBuilder internal constructor() {
private var requestHeaderActions: Output>? = null
private var responseHeaderActions: Output>? = null
private var routeConfigurationOverride:
Output>? = null
/**
* @param value A list of header actions to apply from the request from AFD to the origin.
*/
@JvmName("pxuptdmjsvrrouag")
public suspend fun requestHeaderActions(`value`: Output>) {
this.requestHeaderActions = value
}
@JvmName("peypurnmtyxfacdb")
public suspend fun requestHeaderActions(vararg values: Output) {
this.requestHeaderActions = Output.all(values.asList())
}
/**
* @param values A list of header actions to apply from the request from AFD to the origin.
*/
@JvmName("absuaapddmbxhoxk")
public suspend fun requestHeaderActions(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy