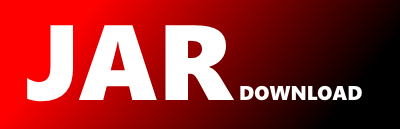
com.pulumi.azurenative.network.kotlin.outputs.ApplicationGatewayResponse.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.network.kotlin.outputs
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.collections.Map
/**
* Application gateway resource.
* @property authenticationCertificates Authentication certificates of the application gateway resource. For default limits, see [Application Gateway limits](https://docs.microsoft.com/azure/azure-subscription-service-limits#application-gateway-limits).
* @property autoscaleConfiguration Autoscale Configuration.
* @property backendAddressPools Backend address pool of the application gateway resource. For default limits, see [Application Gateway limits](https://docs.microsoft.com/azure/azure-subscription-service-limits#application-gateway-limits).
* @property backendHttpSettingsCollection Backend http settings of the application gateway resource. For default limits, see [Application Gateway limits](https://docs.microsoft.com/azure/azure-subscription-service-limits#application-gateway-limits).
* @property backendSettingsCollection Backend settings of the application gateway resource. For default limits, see [Application Gateway limits](https://docs.microsoft.com/azure/azure-subscription-service-limits#application-gateway-limits).
* @property customErrorConfigurations Custom error configurations of the application gateway resource.
* @property defaultPredefinedSslPolicy The default predefined SSL Policy applied on the application gateway resource.
* @property enableFips Whether FIPS is enabled on the application gateway resource.
* @property enableHttp2 Whether HTTP2 is enabled on the application gateway resource.
* @property etag A unique read-only string that changes whenever the resource is updated.
* @property firewallPolicy Reference to the FirewallPolicy resource.
* @property forceFirewallPolicyAssociation If true, associates a firewall policy with an application gateway regardless whether the policy differs from the WAF Config.
* @property frontendIPConfigurations Frontend IP addresses of the application gateway resource. For default limits, see [Application Gateway limits](https://docs.microsoft.com/azure/azure-subscription-service-limits#application-gateway-limits).
* @property frontendPorts Frontend ports of the application gateway resource. For default limits, see [Application Gateway limits](https://docs.microsoft.com/azure/azure-subscription-service-limits#application-gateway-limits).
* @property gatewayIPConfigurations Subnets of the application gateway resource. For default limits, see [Application Gateway limits](https://docs.microsoft.com/azure/azure-subscription-service-limits#application-gateway-limits).
* @property globalConfiguration Global Configuration.
* @property httpListeners Http listeners of the application gateway resource. For default limits, see [Application Gateway limits](https://docs.microsoft.com/azure/azure-subscription-service-limits#application-gateway-limits).
* @property id Resource ID.
* @property identity The identity of the application gateway, if configured.
* @property listeners Listeners of the application gateway resource. For default limits, see [Application Gateway limits](https://docs.microsoft.com/azure/azure-subscription-service-limits#application-gateway-limits).
* @property loadDistributionPolicies Load distribution policies of the application gateway resource.
* @property location Resource location.
* @property name Resource name.
* @property operationalState Operational state of the application gateway resource.
* @property privateEndpointConnections Private Endpoint connections on application gateway.
* @property privateLinkConfigurations PrivateLink configurations on application gateway.
* @property probes Probes of the application gateway resource.
* @property provisioningState The provisioning state of the application gateway resource.
* @property redirectConfigurations Redirect configurations of the application gateway resource. For default limits, see [Application Gateway limits](https://docs.microsoft.com/azure/azure-subscription-service-limits#application-gateway-limits).
* @property requestRoutingRules Request routing rules of the application gateway resource.
* @property resourceGuid The resource GUID property of the application gateway resource.
* @property rewriteRuleSets Rewrite rules for the application gateway resource.
* @property routingRules Routing rules of the application gateway resource.
* @property sku SKU of the application gateway resource.
* @property sslCertificates SSL certificates of the application gateway resource. For default limits, see [Application Gateway limits](https://docs.microsoft.com/azure/azure-subscription-service-limits#application-gateway-limits).
* @property sslPolicy SSL policy of the application gateway resource.
* @property sslProfiles SSL profiles of the application gateway resource. For default limits, see [Application Gateway limits](https://docs.microsoft.com/azure/azure-subscription-service-limits#application-gateway-limits).
* @property tags Resource tags.
* @property trustedClientCertificates Trusted client certificates of the application gateway resource. For default limits, see [Application Gateway limits](https://docs.microsoft.com/azure/azure-subscription-service-limits#application-gateway-limits).
* @property trustedRootCertificates Trusted Root certificates of the application gateway resource. For default limits, see [Application Gateway limits](https://docs.microsoft.com/azure/azure-subscription-service-limits#application-gateway-limits).
* @property type Resource type.
* @property urlPathMaps URL path map of the application gateway resource. For default limits, see [Application Gateway limits](https://docs.microsoft.com/azure/azure-subscription-service-limits#application-gateway-limits).
* @property webApplicationFirewallConfiguration Web application firewall configuration.
* @property zones A list of availability zones denoting where the resource needs to come from.
*/
public data class ApplicationGatewayResponse(
public val authenticationCertificates: List? =
null,
public val autoscaleConfiguration: ApplicationGatewayAutoscaleConfigurationResponse? = null,
public val backendAddressPools: List? = null,
public val backendHttpSettingsCollection: List? =
null,
public val backendSettingsCollection: List? = null,
public val customErrorConfigurations: List? = null,
public val defaultPredefinedSslPolicy: String,
public val enableFips: Boolean? = null,
public val enableHttp2: Boolean? = null,
public val etag: String,
public val firewallPolicy: SubResourceResponse? = null,
public val forceFirewallPolicyAssociation: Boolean? = null,
public val frontendIPConfigurations: List? =
null,
public val frontendPorts: List? = null,
public val gatewayIPConfigurations: List? = null,
public val globalConfiguration: ApplicationGatewayGlobalConfigurationResponse? = null,
public val httpListeners: List? = null,
public val id: String? = null,
public val identity: ManagedServiceIdentityResponse? = null,
public val listeners: List? = null,
public val loadDistributionPolicies: List? =
null,
public val location: String? = null,
public val name: String,
public val operationalState: String,
public val privateEndpointConnections: List,
public val privateLinkConfigurations: List? =
null,
public val probes: List? = null,
public val provisioningState: String,
public val redirectConfigurations: List? = null,
public val requestRoutingRules: List? = null,
public val resourceGuid: String,
public val rewriteRuleSets: List? = null,
public val routingRules: List? = null,
public val sku: ApplicationGatewaySkuResponse? = null,
public val sslCertificates: List? = null,
public val sslPolicy: ApplicationGatewaySslPolicyResponse? = null,
public val sslProfiles: List? = null,
public val tags: Map? = null,
public val trustedClientCertificates: List? =
null,
public val trustedRootCertificates: List? =
null,
public val type: String,
public val urlPathMaps: List? = null,
public val webApplicationFirewallConfiguration: ApplicationGatewayWebApplicationFirewallConfigurationResponse? = null,
public val zones: List? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.azurenative.network.outputs.ApplicationGatewayResponse): ApplicationGatewayResponse = ApplicationGatewayResponse(
authenticationCertificates = javaType.authenticationCertificates().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.network.kotlin.outputs.ApplicationGatewayAuthenticationCertificateResponse.Companion.toKotlin(args0)
})
}),
autoscaleConfiguration = javaType.autoscaleConfiguration().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.network.kotlin.outputs.ApplicationGatewayAutoscaleConfigurationResponse.Companion.toKotlin(args0)
})
}).orElse(null),
backendAddressPools = javaType.backendAddressPools().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.network.kotlin.outputs.ApplicationGatewayBackendAddressPoolResponse.Companion.toKotlin(args0)
})
}),
backendHttpSettingsCollection = javaType.backendHttpSettingsCollection().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.network.kotlin.outputs.ApplicationGatewayBackendHttpSettingsResponse.Companion.toKotlin(args0)
})
}),
backendSettingsCollection = javaType.backendSettingsCollection().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.network.kotlin.outputs.ApplicationGatewayBackendSettingsResponse.Companion.toKotlin(args0)
})
}),
customErrorConfigurations = javaType.customErrorConfigurations().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.network.kotlin.outputs.ApplicationGatewayCustomErrorResponse.Companion.toKotlin(args0)
})
}),
defaultPredefinedSslPolicy = javaType.defaultPredefinedSslPolicy(),
enableFips = javaType.enableFips().map({ args0 -> args0 }).orElse(null),
enableHttp2 = javaType.enableHttp2().map({ args0 -> args0 }).orElse(null),
etag = javaType.etag(),
firewallPolicy = javaType.firewallPolicy().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.network.kotlin.outputs.SubResourceResponse.Companion.toKotlin(args0)
})
}).orElse(null),
forceFirewallPolicyAssociation = javaType.forceFirewallPolicyAssociation().map({ args0 ->
args0
}).orElse(null),
frontendIPConfigurations = javaType.frontendIPConfigurations().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.network.kotlin.outputs.ApplicationGatewayFrontendIPConfigurationResponse.Companion.toKotlin(args0)
})
}),
frontendPorts = javaType.frontendPorts().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.network.kotlin.outputs.ApplicationGatewayFrontendPortResponse.Companion.toKotlin(args0)
})
}),
gatewayIPConfigurations = javaType.gatewayIPConfigurations().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.network.kotlin.outputs.ApplicationGatewayIPConfigurationResponse.Companion.toKotlin(args0)
})
}),
globalConfiguration = javaType.globalConfiguration().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.network.kotlin.outputs.ApplicationGatewayGlobalConfigurationResponse.Companion.toKotlin(args0)
})
}).orElse(null),
httpListeners = javaType.httpListeners().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.network.kotlin.outputs.ApplicationGatewayHttpListenerResponse.Companion.toKotlin(args0)
})
}),
id = javaType.id().map({ args0 -> args0 }).orElse(null),
identity = javaType.identity().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.network.kotlin.outputs.ManagedServiceIdentityResponse.Companion.toKotlin(args0)
})
}).orElse(null),
listeners = javaType.listeners().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.network.kotlin.outputs.ApplicationGatewayListenerResponse.Companion.toKotlin(args0)
})
}),
loadDistributionPolicies = javaType.loadDistributionPolicies().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.network.kotlin.outputs.ApplicationGatewayLoadDistributionPolicyResponse.Companion.toKotlin(args0)
})
}),
location = javaType.location().map({ args0 -> args0 }).orElse(null),
name = javaType.name(),
operationalState = javaType.operationalState(),
privateEndpointConnections = javaType.privateEndpointConnections().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.network.kotlin.outputs.ApplicationGatewayPrivateEndpointConnectionResponse.Companion.toKotlin(args0)
})
}),
privateLinkConfigurations = javaType.privateLinkConfigurations().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.network.kotlin.outputs.ApplicationGatewayPrivateLinkConfigurationResponse.Companion.toKotlin(args0)
})
}),
probes = javaType.probes().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.network.kotlin.outputs.ApplicationGatewayProbeResponse.Companion.toKotlin(args0)
})
}),
provisioningState = javaType.provisioningState(),
redirectConfigurations = javaType.redirectConfigurations().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.network.kotlin.outputs.ApplicationGatewayRedirectConfigurationResponse.Companion.toKotlin(args0)
})
}),
requestRoutingRules = javaType.requestRoutingRules().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.network.kotlin.outputs.ApplicationGatewayRequestRoutingRuleResponse.Companion.toKotlin(args0)
})
}),
resourceGuid = javaType.resourceGuid(),
rewriteRuleSets = javaType.rewriteRuleSets().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.network.kotlin.outputs.ApplicationGatewayRewriteRuleSetResponse.Companion.toKotlin(args0)
})
}),
routingRules = javaType.routingRules().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.network.kotlin.outputs.ApplicationGatewayRoutingRuleResponse.Companion.toKotlin(args0)
})
}),
sku = javaType.sku().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.network.kotlin.outputs.ApplicationGatewaySkuResponse.Companion.toKotlin(args0)
})
}).orElse(null),
sslCertificates = javaType.sslCertificates().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.network.kotlin.outputs.ApplicationGatewaySslCertificateResponse.Companion.toKotlin(args0)
})
}),
sslPolicy = javaType.sslPolicy().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.network.kotlin.outputs.ApplicationGatewaySslPolicyResponse.Companion.toKotlin(args0)
})
}).orElse(null),
sslProfiles = javaType.sslProfiles().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.network.kotlin.outputs.ApplicationGatewaySslProfileResponse.Companion.toKotlin(args0)
})
}),
tags = javaType.tags().map({ args0 -> args0.key.to(args0.value) }).toMap(),
trustedClientCertificates = javaType.trustedClientCertificates().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.network.kotlin.outputs.ApplicationGatewayTrustedClientCertificateResponse.Companion.toKotlin(args0)
})
}),
trustedRootCertificates = javaType.trustedRootCertificates().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.network.kotlin.outputs.ApplicationGatewayTrustedRootCertificateResponse.Companion.toKotlin(args0)
})
}),
type = javaType.type(),
urlPathMaps = javaType.urlPathMaps().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.network.kotlin.outputs.ApplicationGatewayUrlPathMapResponse.Companion.toKotlin(args0)
})
}),
webApplicationFirewallConfiguration = javaType.webApplicationFirewallConfiguration().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.network.kotlin.outputs.ApplicationGatewayWebApplicationFirewallConfigurationResponse.Companion.toKotlin(args0)
})
}).orElse(null),
zones = javaType.zones().map({ args0 -> args0 }),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy