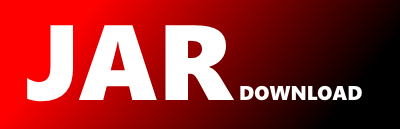
com.pulumi.azurenative.network.kotlin.outputs.GetFirewallPolicyResult.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.network.kotlin.outputs
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.collections.Map
/**
* FirewallPolicy Resource.
* @property basePolicy The parent firewall policy from which rules are inherited.
* @property childPolicies List of references to Child Firewall Policies.
* @property dnsSettings DNS Proxy Settings definition.
* @property etag A unique read-only string that changes whenever the resource is updated.
* @property explicitProxy Explicit Proxy Settings definition.
* @property firewalls List of references to Azure Firewalls that this Firewall Policy is associated with.
* @property id Resource ID.
* @property identity The identity of the firewall policy.
* @property insights Insights on Firewall Policy.
* @property intrusionDetection The configuration for Intrusion detection.
* @property location Resource location.
* @property name Resource name.
* @property provisioningState The provisioning state of the firewall policy resource.
* @property ruleCollectionGroups List of references to FirewallPolicyRuleCollectionGroups.
* @property sku The Firewall Policy SKU.
* @property snat The private IP addresses/IP ranges to which traffic will not be SNAT.
* @property sql SQL Settings definition.
* @property tags Resource tags.
* @property threatIntelMode The operation mode for Threat Intelligence.
* @property threatIntelWhitelist ThreatIntel Whitelist for Firewall Policy.
* @property transportSecurity TLS Configuration definition.
* @property type Resource type.
*/
public data class GetFirewallPolicyResult(
public val basePolicy: SubResourceResponse? = null,
public val childPolicies: List,
public val dnsSettings: DnsSettingsResponse? = null,
public val etag: String,
public val explicitProxy: ExplicitProxyResponse? = null,
public val firewalls: List,
public val id: String? = null,
public val identity: ManagedServiceIdentityResponse? = null,
public val insights: FirewallPolicyInsightsResponse? = null,
public val intrusionDetection: FirewallPolicyIntrusionDetectionResponse? = null,
public val location: String? = null,
public val name: String,
public val provisioningState: String,
public val ruleCollectionGroups: List,
public val sku: FirewallPolicySkuResponse? = null,
public val snat: FirewallPolicySNATResponse? = null,
public val sql: FirewallPolicySQLResponse? = null,
public val tags: Map? = null,
public val threatIntelMode: String? = null,
public val threatIntelWhitelist: FirewallPolicyThreatIntelWhitelistResponse? = null,
public val transportSecurity: FirewallPolicyTransportSecurityResponse? = null,
public val type: String,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.azurenative.network.outputs.GetFirewallPolicyResult): GetFirewallPolicyResult = GetFirewallPolicyResult(
basePolicy = javaType.basePolicy().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.network.kotlin.outputs.SubResourceResponse.Companion.toKotlin(args0)
})
}).orElse(null),
childPolicies = javaType.childPolicies().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.network.kotlin.outputs.SubResourceResponse.Companion.toKotlin(args0)
})
}),
dnsSettings = javaType.dnsSettings().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.network.kotlin.outputs.DnsSettingsResponse.Companion.toKotlin(args0)
})
}).orElse(null),
etag = javaType.etag(),
explicitProxy = javaType.explicitProxy().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.network.kotlin.outputs.ExplicitProxyResponse.Companion.toKotlin(args0)
})
}).orElse(null),
firewalls = javaType.firewalls().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.network.kotlin.outputs.SubResourceResponse.Companion.toKotlin(args0)
})
}),
id = javaType.id().map({ args0 -> args0 }).orElse(null),
identity = javaType.identity().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.network.kotlin.outputs.ManagedServiceIdentityResponse.Companion.toKotlin(args0)
})
}).orElse(null),
insights = javaType.insights().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.network.kotlin.outputs.FirewallPolicyInsightsResponse.Companion.toKotlin(args0)
})
}).orElse(null),
intrusionDetection = javaType.intrusionDetection().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.network.kotlin.outputs.FirewallPolicyIntrusionDetectionResponse.Companion.toKotlin(args0)
})
}).orElse(null),
location = javaType.location().map({ args0 -> args0 }).orElse(null),
name = javaType.name(),
provisioningState = javaType.provisioningState(),
ruleCollectionGroups = javaType.ruleCollectionGroups().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.network.kotlin.outputs.SubResourceResponse.Companion.toKotlin(args0)
})
}),
sku = javaType.sku().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.network.kotlin.outputs.FirewallPolicySkuResponse.Companion.toKotlin(args0)
})
}).orElse(null),
snat = javaType.snat().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.network.kotlin.outputs.FirewallPolicySNATResponse.Companion.toKotlin(args0)
})
}).orElse(null),
sql = javaType.sql().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.network.kotlin.outputs.FirewallPolicySQLResponse.Companion.toKotlin(args0)
})
}).orElse(null),
tags = javaType.tags().map({ args0 -> args0.key.to(args0.value) }).toMap(),
threatIntelMode = javaType.threatIntelMode().map({ args0 -> args0 }).orElse(null),
threatIntelWhitelist = javaType.threatIntelWhitelist().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.network.kotlin.outputs.FirewallPolicyThreatIntelWhitelistResponse.Companion.toKotlin(args0)
})
}).orElse(null),
transportSecurity = javaType.transportSecurity().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.network.kotlin.outputs.FirewallPolicyTransportSecurityResponse.Companion.toKotlin(args0)
})
}).orElse(null),
type = javaType.type(),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy