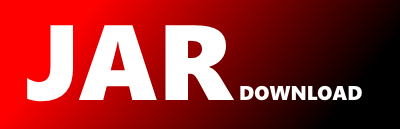
com.pulumi.azurenative.networkcloud.kotlin.ClusterArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.networkcloud.kotlin
import com.pulumi.azurenative.networkcloud.ClusterArgs.builder
import com.pulumi.azurenative.networkcloud.kotlin.enums.ClusterType
import com.pulumi.azurenative.networkcloud.kotlin.inputs.ClusterSecretArchiveArgs
import com.pulumi.azurenative.networkcloud.kotlin.inputs.ClusterSecretArchiveArgsBuilder
import com.pulumi.azurenative.networkcloud.kotlin.inputs.ClusterUpdateStrategyArgs
import com.pulumi.azurenative.networkcloud.kotlin.inputs.ClusterUpdateStrategyArgsBuilder
import com.pulumi.azurenative.networkcloud.kotlin.inputs.ExtendedLocationArgs
import com.pulumi.azurenative.networkcloud.kotlin.inputs.ExtendedLocationArgsBuilder
import com.pulumi.azurenative.networkcloud.kotlin.inputs.ManagedResourceGroupConfigurationArgs
import com.pulumi.azurenative.networkcloud.kotlin.inputs.ManagedResourceGroupConfigurationArgsBuilder
import com.pulumi.azurenative.networkcloud.kotlin.inputs.RackDefinitionArgs
import com.pulumi.azurenative.networkcloud.kotlin.inputs.RackDefinitionArgsBuilder
import com.pulumi.azurenative.networkcloud.kotlin.inputs.RuntimeProtectionConfigurationArgs
import com.pulumi.azurenative.networkcloud.kotlin.inputs.RuntimeProtectionConfigurationArgsBuilder
import com.pulumi.azurenative.networkcloud.kotlin.inputs.ServicePrincipalInformationArgs
import com.pulumi.azurenative.networkcloud.kotlin.inputs.ServicePrincipalInformationArgsBuilder
import com.pulumi.azurenative.networkcloud.kotlin.inputs.ValidationThresholdArgs
import com.pulumi.azurenative.networkcloud.kotlin.inputs.ValidationThresholdArgsBuilder
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
*
* Azure REST API version: 2023-10-01-preview. Prior API version in Azure Native 1.x: 2022-12-12-preview.
* Other available API versions: 2023-07-01, 2024-06-01-preview.
* ## Example Usage
* ### Create or update cluster
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var cluster = new AzureNative.NetworkCloud.Cluster("cluster", new()
* {
* AggregatorOrSingleRackDefinition = new AzureNative.NetworkCloud.Inputs.RackDefinitionArgs
* {
* BareMetalMachineConfigurationData = new[]
* {
* new AzureNative.NetworkCloud.Inputs.BareMetalMachineConfigurationDataArgs
* {
* BmcCredentials = new AzureNative.NetworkCloud.Inputs.AdministrativeCredentialsArgs
* {
* Password = "{password}",
* Username = "username",
* },
* BmcMacAddress = "AA:BB:CC:DD:EE:FF",
* BootMacAddress = "00:BB:CC:DD:EE:FF",
* MachineDetails = "extraDetails",
* MachineName = "bmmName1",
* RackSlot = 1,
* SerialNumber = "BM1219XXX",
* },
* new AzureNative.NetworkCloud.Inputs.BareMetalMachineConfigurationDataArgs
* {
* BmcCredentials = new AzureNative.NetworkCloud.Inputs.AdministrativeCredentialsArgs
* {
* Password = "{password}",
* Username = "username",
* },
* BmcMacAddress = "AA:BB:CC:DD:EE:00",
* BootMacAddress = "00:BB:CC:DD:EE:00",
* MachineDetails = "extraDetails",
* MachineName = "bmmName2",
* RackSlot = 2,
* SerialNumber = "BM1219YYY",
* },
* },
* NetworkRackId = "/subscriptions/123e4567-e89b-12d3-a456-426655440000/resourceGroups/resourceGroupName/providers/Microsoft.ManagedNetworkFabric/networkRacks/networkRackName",
* RackLocation = "Foo Datacenter, Floor 3, Aisle 9, Rack 2",
* RackSerialNumber = "AA1234",
* RackSkuId = "/subscriptions/123e4567-e89b-12d3-a456-426655440000/providers/Microsoft.NetworkCloud/rackSkus/rackSkuName",
* StorageApplianceConfigurationData = new[]
* {
* new AzureNative.NetworkCloud.Inputs.StorageApplianceConfigurationDataArgs
* {
* AdminCredentials = new AzureNative.NetworkCloud.Inputs.AdministrativeCredentialsArgs
* {
* Password = "{password}",
* Username = "username",
* },
* RackSlot = 1,
* SerialNumber = "BM1219XXX",
* StorageApplianceName = "vmName",
* },
* },
* },
* AnalyticsWorkspaceId = "/subscriptions/123e4567-e89b-12d3-a456-426655440000/resourceGroups/resourceGroupName/providers/microsoft.operationalInsights/workspaces/logAnalyticsWorkspaceName",
* ClusterLocation = "Foo Street, 3rd Floor, row 9",
* ClusterName = "clusterName",
* ClusterServicePrincipal = new AzureNative.NetworkCloud.Inputs.ServicePrincipalInformationArgs
* {
* ApplicationId = "12345678-1234-1234-1234-123456789012",
* Password = "{password}",
* PrincipalId = "00000008-0004-0004-0004-000000000012",
* TenantId = "80000000-4000-4000-4000-120000000000",
* },
* ClusterType = AzureNative.NetworkCloud.ClusterType.SingleRack,
* ClusterVersion = "1.0.0",
* ComputeDeploymentThreshold = new AzureNative.NetworkCloud.Inputs.ValidationThresholdArgs
* {
* Grouping = AzureNative.NetworkCloud.ValidationThresholdGrouping.PerCluster,
* Type = AzureNative.NetworkCloud.ValidationThresholdType.PercentSuccess,
* Value = 90,
* },
* ComputeRackDefinitions = new[]
* {
* new AzureNative.NetworkCloud.Inputs.RackDefinitionArgs
* {
* BareMetalMachineConfigurationData = new[]
* {
* new AzureNative.NetworkCloud.Inputs.BareMetalMachineConfigurationDataArgs
* {
* BmcCredentials = new AzureNative.NetworkCloud.Inputs.AdministrativeCredentialsArgs
* {
* Password = "{password}",
* Username = "username",
* },
* BmcMacAddress = "AA:BB:CC:DD:EE:FF",
* BootMacAddress = "00:BB:CC:DD:EE:FF",
* MachineDetails = "extraDetails",
* MachineName = "bmmName1",
* RackSlot = 1,
* SerialNumber = "BM1219XXX",
* },
* new AzureNative.NetworkCloud.Inputs.BareMetalMachineConfigurationDataArgs
* {
* BmcCredentials = new AzureNative.NetworkCloud.Inputs.AdministrativeCredentialsArgs
* {
* Password = "{password}",
* Username = "username",
* },
* BmcMacAddress = "AA:BB:CC:DD:EE:00",
* BootMacAddress = "00:BB:CC:DD:EE:00",
* MachineDetails = "extraDetails",
* MachineName = "bmmName2",
* RackSlot = 2,
* SerialNumber = "BM1219YYY",
* },
* },
* NetworkRackId = "/subscriptions/123e4567-e89b-12d3-a456-426655440000/resourceGroups/resourceGroupName/providers/Microsoft.ManagedNetworkFabric/networkRacks/networkRackName",
* RackLocation = "Foo Datacenter, Floor 3, Aisle 9, Rack 2",
* RackSerialNumber = "AA1234",
* RackSkuId = "/subscriptions/123e4567-e89b-12d3-a456-426655440000/providers/Microsoft.NetworkCloud/rackSkus/rackSkuName",
* StorageApplianceConfigurationData = new[]
* {
* new AzureNative.NetworkCloud.Inputs.StorageApplianceConfigurationDataArgs
* {
* AdminCredentials = new AzureNative.NetworkCloud.Inputs.AdministrativeCredentialsArgs
* {
* Password = "{password}",
* Username = "username",
* },
* RackSlot = 1,
* SerialNumber = "BM1219XXX",
* StorageApplianceName = "vmName",
* },
* },
* },
* },
* ExtendedLocation = new AzureNative.NetworkCloud.Inputs.ExtendedLocationArgs
* {
* Name = "/subscriptions/123e4567-e89b-12d3-a456-426655440000/resourceGroups/resourceGroupName/providers/Microsoft.ExtendedLocation/customLocations/clusterManagerExtendedLocationName",
* Type = "CustomLocation",
* },
* Location = "location",
* ManagedResourceGroupConfiguration = new AzureNative.NetworkCloud.Inputs.ManagedResourceGroupConfigurationArgs
* {
* Location = "East US",
* Name = "my-managed-rg",
* },
* NetworkFabricId = "/subscriptions/123e4567-e89b-12d3-a456-426655440000/resourceGroups/resourceGroupName/providers/Microsoft.ManagedNetworkFabric/networkFabrics/fabricName",
* ResourceGroupName = "resourceGroupName",
* RuntimeProtectionConfiguration = new AzureNative.NetworkCloud.Inputs.RuntimeProtectionConfigurationArgs
* {
* EnforcementLevel = AzureNative.NetworkCloud.RuntimeProtectionEnforcementLevel.OnDemand,
* },
* SecretArchive = new AzureNative.NetworkCloud.Inputs.ClusterSecretArchiveArgs
* {
* KeyVaultId = "/subscriptions/123e4567-e89b-12d3-a456-426655440000/resourceGroups/resourceGroupName/providers/Microsoft.KeyVault/vaults/keyVaultName",
* UseKeyVault = AzureNative.NetworkCloud.ClusterSecretArchiveEnabled.True,
* },
* Tags =
* {
* { "key1", "myvalue1" },
* { "key2", "myvalue2" },
* },
* UpdateStrategy = new AzureNative.NetworkCloud.Inputs.ClusterUpdateStrategyArgs
* {
* MaxUnavailable = 4,
* StrategyType = AzureNative.NetworkCloud.ClusterUpdateStrategyType.Rack,
* ThresholdType = AzureNative.NetworkCloud.ValidationThresholdType.CountSuccess,
* ThresholdValue = 4,
* WaitTimeMinutes = 10,
* },
* });
* });
* ```
* ```go
* package main
* import (
* networkcloud "github.com/pulumi/pulumi-azure-native-sdk/networkcloud/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := networkcloud.NewCluster(ctx, "cluster", &networkcloud.ClusterArgs{
* AggregatorOrSingleRackDefinition: &networkcloud.RackDefinitionArgs{
* BareMetalMachineConfigurationData: networkcloud.BareMetalMachineConfigurationDataArray{
* &networkcloud.BareMetalMachineConfigurationDataArgs{
* BmcCredentials: &networkcloud.AdministrativeCredentialsArgs{
* Password: pulumi.String("{password}"),
* Username: pulumi.String("username"),
* },
* BmcMacAddress: pulumi.String("AA:BB:CC:DD:EE:FF"),
* BootMacAddress: pulumi.String("00:BB:CC:DD:EE:FF"),
* MachineDetails: pulumi.String("extraDetails"),
* MachineName: pulumi.String("bmmName1"),
* RackSlot: pulumi.Float64(1),
* SerialNumber: pulumi.String("BM1219XXX"),
* },
* &networkcloud.BareMetalMachineConfigurationDataArgs{
* BmcCredentials: &networkcloud.AdministrativeCredentialsArgs{
* Password: pulumi.String("{password}"),
* Username: pulumi.String("username"),
* },
* BmcMacAddress: pulumi.String("AA:BB:CC:DD:EE:00"),
* BootMacAddress: pulumi.String("00:BB:CC:DD:EE:00"),
* MachineDetails: pulumi.String("extraDetails"),
* MachineName: pulumi.String("bmmName2"),
* RackSlot: pulumi.Float64(2),
* SerialNumber: pulumi.String("BM1219YYY"),
* },
* },
* NetworkRackId: pulumi.String("/subscriptions/123e4567-e89b-12d3-a456-426655440000/resourceGroups/resourceGroupName/providers/Microsoft.ManagedNetworkFabric/networkRacks/networkRackName"),
* RackLocation: pulumi.String("Foo Datacenter, Floor 3, Aisle 9, Rack 2"),
* RackSerialNumber: pulumi.String("AA1234"),
* RackSkuId: pulumi.String("/subscriptions/123e4567-e89b-12d3-a456-426655440000/providers/Microsoft.NetworkCloud/rackSkus/rackSkuName"),
* StorageApplianceConfigurationData: networkcloud.StorageApplianceConfigurationDataArray{
* &networkcloud.StorageApplianceConfigurationDataArgs{
* AdminCredentials: &networkcloud.AdministrativeCredentialsArgs{
* Password: pulumi.String("{password}"),
* Username: pulumi.String("username"),
* },
* RackSlot: pulumi.Float64(1),
* SerialNumber: pulumi.String("BM1219XXX"),
* StorageApplianceName: pulumi.String("vmName"),
* },
* },
* },
* AnalyticsWorkspaceId: pulumi.String("/subscriptions/123e4567-e89b-12d3-a456-426655440000/resourceGroups/resourceGroupName/providers/microsoft.operationalInsights/workspaces/logAnalyticsWorkspaceName"),
* ClusterLocation: pulumi.String("Foo Street, 3rd Floor, row 9"),
* ClusterName: pulumi.String("clusterName"),
* ClusterServicePrincipal: &networkcloud.ServicePrincipalInformationArgs{
* ApplicationId: pulumi.String("12345678-1234-1234-1234-123456789012"),
* Password: pulumi.String("{password}"),
* PrincipalId: pulumi.String("00000008-0004-0004-0004-000000000012"),
* TenantId: pulumi.String("80000000-4000-4000-4000-120000000000"),
* },
* ClusterType: pulumi.String(networkcloud.ClusterTypeSingleRack),
* ClusterVersion: pulumi.String("1.0.0"),
* ComputeDeploymentThreshold: &networkcloud.ValidationThresholdArgs{
* Grouping: pulumi.String(networkcloud.ValidationThresholdGroupingPerCluster),
* Type: pulumi.String(networkcloud.ValidationThresholdTypePercentSuccess),
* Value: pulumi.Float64(90),
* },
* ComputeRackDefinitions: networkcloud.RackDefinitionArray{
* &networkcloud.RackDefinitionArgs{
* BareMetalMachineConfigurationData: networkcloud.BareMetalMachineConfigurationDataArray{
* &networkcloud.BareMetalMachineConfigurationDataArgs{
* BmcCredentials: &networkcloud.AdministrativeCredentialsArgs{
* Password: pulumi.String("{password}"),
* Username: pulumi.String("username"),
* },
* BmcMacAddress: pulumi.String("AA:BB:CC:DD:EE:FF"),
* BootMacAddress: pulumi.String("00:BB:CC:DD:EE:FF"),
* MachineDetails: pulumi.String("extraDetails"),
* MachineName: pulumi.String("bmmName1"),
* RackSlot: pulumi.Float64(1),
* SerialNumber: pulumi.String("BM1219XXX"),
* },
* &networkcloud.BareMetalMachineConfigurationDataArgs{
* BmcCredentials: &networkcloud.AdministrativeCredentialsArgs{
* Password: pulumi.String("{password}"),
* Username: pulumi.String("username"),
* },
* BmcMacAddress: pulumi.String("AA:BB:CC:DD:EE:00"),
* BootMacAddress: pulumi.String("00:BB:CC:DD:EE:00"),
* MachineDetails: pulumi.String("extraDetails"),
* MachineName: pulumi.String("bmmName2"),
* RackSlot: pulumi.Float64(2),
* SerialNumber: pulumi.String("BM1219YYY"),
* },
* },
* NetworkRackId: pulumi.String("/subscriptions/123e4567-e89b-12d3-a456-426655440000/resourceGroups/resourceGroupName/providers/Microsoft.ManagedNetworkFabric/networkRacks/networkRackName"),
* RackLocation: pulumi.String("Foo Datacenter, Floor 3, Aisle 9, Rack 2"),
* RackSerialNumber: pulumi.String("AA1234"),
* RackSkuId: pulumi.String("/subscriptions/123e4567-e89b-12d3-a456-426655440000/providers/Microsoft.NetworkCloud/rackSkus/rackSkuName"),
* StorageApplianceConfigurationData: networkcloud.StorageApplianceConfigurationDataArray{
* &networkcloud.StorageApplianceConfigurationDataArgs{
* AdminCredentials: &networkcloud.AdministrativeCredentialsArgs{
* Password: pulumi.String("{password}"),
* Username: pulumi.String("username"),
* },
* RackSlot: pulumi.Float64(1),
* SerialNumber: pulumi.String("BM1219XXX"),
* StorageApplianceName: pulumi.String("vmName"),
* },
* },
* },
* },
* ExtendedLocation: &networkcloud.ExtendedLocationArgs{
* Name: pulumi.String("/subscriptions/123e4567-e89b-12d3-a456-426655440000/resourceGroups/resourceGroupName/providers/Microsoft.ExtendedLocation/customLocations/clusterManagerExtendedLocationName"),
* Type: pulumi.String("CustomLocation"),
* },
* Location: pulumi.String("location"),
* ManagedResourceGroupConfiguration: &networkcloud.ManagedResourceGroupConfigurationArgs{
* Location: pulumi.String("East US"),
* Name: pulumi.String("my-managed-rg"),
* },
* NetworkFabricId: pulumi.String("/subscriptions/123e4567-e89b-12d3-a456-426655440000/resourceGroups/resourceGroupName/providers/Microsoft.ManagedNetworkFabric/networkFabrics/fabricName"),
* ResourceGroupName: pulumi.String("resourceGroupName"),
* RuntimeProtectionConfiguration: &networkcloud.RuntimeProtectionConfigurationArgs{
* EnforcementLevel: pulumi.String(networkcloud.RuntimeProtectionEnforcementLevelOnDemand),
* },
* SecretArchive: &networkcloud.ClusterSecretArchiveArgs{
* KeyVaultId: pulumi.String("/subscriptions/123e4567-e89b-12d3-a456-426655440000/resourceGroups/resourceGroupName/providers/Microsoft.KeyVault/vaults/keyVaultName"),
* UseKeyVault: pulumi.String(networkcloud.ClusterSecretArchiveEnabledTrue),
* },
* Tags: pulumi.StringMap{
* "key1": pulumi.String("myvalue1"),
* "key2": pulumi.String("myvalue2"),
* },
* UpdateStrategy: &networkcloud.ClusterUpdateStrategyArgs{
* MaxUnavailable: pulumi.Float64(4),
* StrategyType: pulumi.String(networkcloud.ClusterUpdateStrategyTypeRack),
* ThresholdType: pulumi.String(networkcloud.ValidationThresholdTypeCountSuccess),
* ThresholdValue: pulumi.Float64(4),
* WaitTimeMinutes: pulumi.Float64(10),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.networkcloud.Cluster;
* import com.pulumi.azurenative.networkcloud.ClusterArgs;
* import com.pulumi.azurenative.networkcloud.inputs.RackDefinitionArgs;
* import com.pulumi.azurenative.networkcloud.inputs.ServicePrincipalInformationArgs;
* import com.pulumi.azurenative.networkcloud.inputs.ValidationThresholdArgs;
* import com.pulumi.azurenative.networkcloud.inputs.ExtendedLocationArgs;
* import com.pulumi.azurenative.networkcloud.inputs.ManagedResourceGroupConfigurationArgs;
* import com.pulumi.azurenative.networkcloud.inputs.RuntimeProtectionConfigurationArgs;
* import com.pulumi.azurenative.networkcloud.inputs.ClusterSecretArchiveArgs;
* import com.pulumi.azurenative.networkcloud.inputs.ClusterUpdateStrategyArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var cluster = new Cluster("cluster", ClusterArgs.builder()
* .aggregatorOrSingleRackDefinition(RackDefinitionArgs.builder()
* .bareMetalMachineConfigurationData(
* BareMetalMachineConfigurationDataArgs.builder()
* .bmcCredentials(AdministrativeCredentialsArgs.builder()
* .password("{password}")
* .username("username")
* .build())
* .bmcMacAddress("AA:BB:CC:DD:EE:FF")
* .bootMacAddress("00:BB:CC:DD:EE:FF")
* .machineDetails("extraDetails")
* .machineName("bmmName1")
* .rackSlot(1)
* .serialNumber("BM1219XXX")
* .build(),
* BareMetalMachineConfigurationDataArgs.builder()
* .bmcCredentials(AdministrativeCredentialsArgs.builder()
* .password("{password}")
* .username("username")
* .build())
* .bmcMacAddress("AA:BB:CC:DD:EE:00")
* .bootMacAddress("00:BB:CC:DD:EE:00")
* .machineDetails("extraDetails")
* .machineName("bmmName2")
* .rackSlot(2)
* .serialNumber("BM1219YYY")
* .build())
* .networkRackId("/subscriptions/123e4567-e89b-12d3-a456-426655440000/resourceGroups/resourceGroupName/providers/Microsoft.ManagedNetworkFabric/networkRacks/networkRackName")
* .rackLocation("Foo Datacenter, Floor 3, Aisle 9, Rack 2")
* .rackSerialNumber("AA1234")
* .rackSkuId("/subscriptions/123e4567-e89b-12d3-a456-426655440000/providers/Microsoft.NetworkCloud/rackSkus/rackSkuName")
* .storageApplianceConfigurationData(StorageApplianceConfigurationDataArgs.builder()
* .adminCredentials(AdministrativeCredentialsArgs.builder()
* .password("{password}")
* .username("username")
* .build())
* .rackSlot(1)
* .serialNumber("BM1219XXX")
* .storageApplianceName("vmName")
* .build())
* .build())
* .analyticsWorkspaceId("/subscriptions/123e4567-e89b-12d3-a456-426655440000/resourceGroups/resourceGroupName/providers/microsoft.operationalInsights/workspaces/logAnalyticsWorkspaceName")
* .clusterLocation("Foo Street, 3rd Floor, row 9")
* .clusterName("clusterName")
* .clusterServicePrincipal(ServicePrincipalInformationArgs.builder()
* .applicationId("12345678-1234-1234-1234-123456789012")
* .password("{password}")
* .principalId("00000008-0004-0004-0004-000000000012")
* .tenantId("80000000-4000-4000-4000-120000000000")
* .build())
* .clusterType("SingleRack")
* .clusterVersion("1.0.0")
* .computeDeploymentThreshold(ValidationThresholdArgs.builder()
* .grouping("PerCluster")
* .type("PercentSuccess")
* .value(90)
* .build())
* .computeRackDefinitions(RackDefinitionArgs.builder()
* .bareMetalMachineConfigurationData(
* BareMetalMachineConfigurationDataArgs.builder()
* .bmcCredentials(AdministrativeCredentialsArgs.builder()
* .password("{password}")
* .username("username")
* .build())
* .bmcMacAddress("AA:BB:CC:DD:EE:FF")
* .bootMacAddress("00:BB:CC:DD:EE:FF")
* .machineDetails("extraDetails")
* .machineName("bmmName1")
* .rackSlot(1)
* .serialNumber("BM1219XXX")
* .build(),
* BareMetalMachineConfigurationDataArgs.builder()
* .bmcCredentials(AdministrativeCredentialsArgs.builder()
* .password("{password}")
* .username("username")
* .build())
* .bmcMacAddress("AA:BB:CC:DD:EE:00")
* .bootMacAddress("00:BB:CC:DD:EE:00")
* .machineDetails("extraDetails")
* .machineName("bmmName2")
* .rackSlot(2)
* .serialNumber("BM1219YYY")
* .build())
* .networkRackId("/subscriptions/123e4567-e89b-12d3-a456-426655440000/resourceGroups/resourceGroupName/providers/Microsoft.ManagedNetworkFabric/networkRacks/networkRackName")
* .rackLocation("Foo Datacenter, Floor 3, Aisle 9, Rack 2")
* .rackSerialNumber("AA1234")
* .rackSkuId("/subscriptions/123e4567-e89b-12d3-a456-426655440000/providers/Microsoft.NetworkCloud/rackSkus/rackSkuName")
* .storageApplianceConfigurationData(StorageApplianceConfigurationDataArgs.builder()
* .adminCredentials(AdministrativeCredentialsArgs.builder()
* .password("{password}")
* .username("username")
* .build())
* .rackSlot(1)
* .serialNumber("BM1219XXX")
* .storageApplianceName("vmName")
* .build())
* .build())
* .extendedLocation(ExtendedLocationArgs.builder()
* .name("/subscriptions/123e4567-e89b-12d3-a456-426655440000/resourceGroups/resourceGroupName/providers/Microsoft.ExtendedLocation/customLocations/clusterManagerExtendedLocationName")
* .type("CustomLocation")
* .build())
* .location("location")
* .managedResourceGroupConfiguration(ManagedResourceGroupConfigurationArgs.builder()
* .location("East US")
* .name("my-managed-rg")
* .build())
* .networkFabricId("/subscriptions/123e4567-e89b-12d3-a456-426655440000/resourceGroups/resourceGroupName/providers/Microsoft.ManagedNetworkFabric/networkFabrics/fabricName")
* .resourceGroupName("resourceGroupName")
* .runtimeProtectionConfiguration(RuntimeProtectionConfigurationArgs.builder()
* .enforcementLevel("OnDemand")
* .build())
* .secretArchive(ClusterSecretArchiveArgs.builder()
* .keyVaultId("/subscriptions/123e4567-e89b-12d3-a456-426655440000/resourceGroups/resourceGroupName/providers/Microsoft.KeyVault/vaults/keyVaultName")
* .useKeyVault("True")
* .build())
* .tags(Map.ofEntries(
* Map.entry("key1", "myvalue1"),
* Map.entry("key2", "myvalue2")
* ))
* .updateStrategy(ClusterUpdateStrategyArgs.builder()
* .maxUnavailable(4)
* .strategyType("Rack")
* .thresholdType("CountSuccess")
* .thresholdValue(4)
* .waitTimeMinutes(10)
* .build())
* .build());
* }
* }
* ```
* ## Import
* An existing resource can be imported using its type token, name, and identifier, e.g.
* ```sh
* $ pulumi import azure-native:networkcloud:Cluster clusterName /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetworkCloud/clusters/{clusterName}
* ```
* @property aggregatorOrSingleRackDefinition The rack definition that is intended to reflect only a single rack in a single rack cluster, or an aggregator rack in a multi-rack cluster.
* @property analyticsWorkspaceId The resource ID of the Log Analytics Workspace that will be used for storing relevant logs.
* @property clusterLocation The customer-provided location information to identify where the cluster resides.
* @property clusterName The name of the cluster.
* @property clusterServicePrincipal The service principal to be used by the cluster during Arc Appliance installation.
* @property clusterType The type of rack configuration for the cluster.
* @property clusterVersion The current runtime version of the cluster.
* @property computeDeploymentThreshold The validation threshold indicating the allowable failures of compute machines during environment validation and deployment.
* @property computeRackDefinitions The list of rack definitions for the compute racks in a multi-rack
* cluster, or an empty list in a single-rack cluster.
* @property extendedLocation The extended location of the cluster manager associated with the cluster.
* @property location The geo-location where the resource lives
* @property managedResourceGroupConfiguration The configuration of the managed resource group associated with the resource.
* @property networkFabricId The resource ID of the Network Fabric associated with the cluster.
* @property resourceGroupName The name of the resource group. The name is case insensitive.
* @property runtimeProtectionConfiguration The settings for cluster runtime protection.
* @property secretArchive The configuration for use of a key vault to store secrets for later retrieval by the operator.
* @property tags Resource tags.
* @property updateStrategy The strategy for updating the cluster.
*/
public data class ClusterArgs(
public val aggregatorOrSingleRackDefinition: Output? = null,
public val analyticsWorkspaceId: Output? = null,
public val clusterLocation: Output? = null,
public val clusterName: Output? = null,
public val clusterServicePrincipal: Output? = null,
public val clusterType: Output>? = null,
public val clusterVersion: Output? = null,
public val computeDeploymentThreshold: Output? = null,
public val computeRackDefinitions: Output>? = null,
public val extendedLocation: Output? = null,
public val location: Output? = null,
public val managedResourceGroupConfiguration: Output? =
null,
public val networkFabricId: Output? = null,
public val resourceGroupName: Output? = null,
public val runtimeProtectionConfiguration: Output? = null,
public val secretArchive: Output? = null,
public val tags: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy