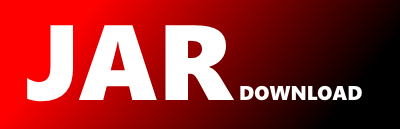
com.pulumi.azurenative.offazurespringboot.kotlin.OffazurespringbootFunctions.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.offazurespringboot.kotlin
import com.pulumi.azurenative.offazurespringboot.OffazurespringbootFunctions.getSpringbootappPlain
import com.pulumi.azurenative.offazurespringboot.OffazurespringbootFunctions.getSpringbootserverPlain
import com.pulumi.azurenative.offazurespringboot.OffazurespringbootFunctions.getSpringbootsitePlain
import com.pulumi.azurenative.offazurespringboot.kotlin.inputs.GetSpringbootappPlainArgs
import com.pulumi.azurenative.offazurespringboot.kotlin.inputs.GetSpringbootappPlainArgsBuilder
import com.pulumi.azurenative.offazurespringboot.kotlin.inputs.GetSpringbootserverPlainArgs
import com.pulumi.azurenative.offazurespringboot.kotlin.inputs.GetSpringbootserverPlainArgsBuilder
import com.pulumi.azurenative.offazurespringboot.kotlin.inputs.GetSpringbootsitePlainArgs
import com.pulumi.azurenative.offazurespringboot.kotlin.inputs.GetSpringbootsitePlainArgsBuilder
import com.pulumi.azurenative.offazurespringboot.kotlin.outputs.GetSpringbootappResult
import com.pulumi.azurenative.offazurespringboot.kotlin.outputs.GetSpringbootserverResult
import com.pulumi.azurenative.offazurespringboot.kotlin.outputs.GetSpringbootsiteResult
import kotlinx.coroutines.future.await
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import com.pulumi.azurenative.offazurespringboot.kotlin.outputs.GetSpringbootappResult.Companion.toKotlin as getSpringbootappResultToKotlin
import com.pulumi.azurenative.offazurespringboot.kotlin.outputs.GetSpringbootserverResult.Companion.toKotlin as getSpringbootserverResultToKotlin
import com.pulumi.azurenative.offazurespringboot.kotlin.outputs.GetSpringbootsiteResult.Companion.toKotlin as getSpringbootsiteResultToKotlin
public object OffazurespringbootFunctions {
/**
* Get a springbootapps resource.
* Azure REST API version: 2024-04-01-preview.
* @param argument null
* @return The springbootapps envelope resource definition.
*/
public suspend fun getSpringbootapp(argument: GetSpringbootappPlainArgs): GetSpringbootappResult =
getSpringbootappResultToKotlin(getSpringbootappPlain(argument.toJava()).await())
/**
* @see [getSpringbootapp].
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param siteName The springbootsites name.
* @param springbootappsName The springbootapps name.
* @return The springbootapps envelope resource definition.
*/
public suspend fun getSpringbootapp(
resourceGroupName: String,
siteName: String,
springbootappsName: String,
): GetSpringbootappResult {
val argument = GetSpringbootappPlainArgs(
resourceGroupName = resourceGroupName,
siteName = siteName,
springbootappsName = springbootappsName,
)
return getSpringbootappResultToKotlin(getSpringbootappPlain(argument.toJava()).await())
}
/**
* @see [getSpringbootapp].
* @param argument Builder for [com.pulumi.azurenative.offazurespringboot.kotlin.inputs.GetSpringbootappPlainArgs].
* @return The springbootapps envelope resource definition.
*/
public suspend fun getSpringbootapp(argument: suspend GetSpringbootappPlainArgsBuilder.() -> Unit): GetSpringbootappResult {
val builder = GetSpringbootappPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getSpringbootappResultToKotlin(getSpringbootappPlain(builtArgument.toJava()).await())
}
/**
* List springbootservers resource.
* Azure REST API version: 2023-01-01-preview.
* @param argument null
* @return The springbootservers envelope resource definition.
*/
public suspend fun getSpringbootserver(argument: GetSpringbootserverPlainArgs): GetSpringbootserverResult =
getSpringbootserverResultToKotlin(getSpringbootserverPlain(argument.toJava()).await())
/**
* @see [getSpringbootserver].
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param siteName The springbootsites name.
* @param springbootserversName The springbootservers name.
* @return The springbootservers envelope resource definition.
*/
public suspend fun getSpringbootserver(
resourceGroupName: String,
siteName: String,
springbootserversName: String,
): GetSpringbootserverResult {
val argument = GetSpringbootserverPlainArgs(
resourceGroupName = resourceGroupName,
siteName = siteName,
springbootserversName = springbootserversName,
)
return getSpringbootserverResultToKotlin(getSpringbootserverPlain(argument.toJava()).await())
}
/**
* @see [getSpringbootserver].
* @param argument Builder for [com.pulumi.azurenative.offazurespringboot.kotlin.inputs.GetSpringbootserverPlainArgs].
* @return The springbootservers envelope resource definition.
*/
public suspend fun getSpringbootserver(argument: suspend GetSpringbootserverPlainArgsBuilder.() -> Unit): GetSpringbootserverResult {
val builder = GetSpringbootserverPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getSpringbootserverResultToKotlin(getSpringbootserverPlain(builtArgument.toJava()).await())
}
/**
* Get a springbootsites resource.
* Azure REST API version: 2023-01-01-preview.
* @param argument null
* @return The springbootsites envelope resource definition.
*/
public suspend fun getSpringbootsite(argument: GetSpringbootsitePlainArgs): GetSpringbootsiteResult =
getSpringbootsiteResultToKotlin(getSpringbootsitePlain(argument.toJava()).await())
/**
* @see [getSpringbootsite].
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param springbootsitesName The springbootsites name.
* @return The springbootsites envelope resource definition.
*/
public suspend fun getSpringbootsite(resourceGroupName: String, springbootsitesName: String): GetSpringbootsiteResult {
val argument = GetSpringbootsitePlainArgs(
resourceGroupName = resourceGroupName,
springbootsitesName = springbootsitesName,
)
return getSpringbootsiteResultToKotlin(getSpringbootsitePlain(argument.toJava()).await())
}
/**
* @see [getSpringbootsite].
* @param argument Builder for [com.pulumi.azurenative.offazurespringboot.kotlin.inputs.GetSpringbootsitePlainArgs].
* @return The springbootsites envelope resource definition.
*/
public suspend fun getSpringbootsite(argument: suspend GetSpringbootsitePlainArgsBuilder.() -> Unit): GetSpringbootsiteResult {
val builder = GetSpringbootsitePlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getSpringbootsiteResultToKotlin(getSpringbootsitePlain(builtArgument.toJava()).await())
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy