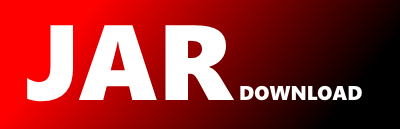
com.pulumi.azurenative.offazurespringboot.kotlin.outputs.SpringbootappsPropertiesResponse.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.offazurespringboot.kotlin.outputs
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.collections.Map
/**
* The springbootapps resource definition.
* @property appName The name of SpringBootApp.
* @property appPort The application port.
* @property appType The application type, whether it is a SpringBoot app.
* @property applicationConfigurations The application configuration file list.
* @property artifactName The artifact name of SpringBootApp.
* @property bindingPorts The application binding port list.
* @property buildJdkVersion The jdk version in build.
* @property certificates The certificate file list.
* @property checksum The checksum of jar file.
* @property connectionStrings The connection string list.
* @property dependencies The dependency list.
* @property environments The environment variable list.
* @property errors The list of errors.
* @property instanceCount The total instance count the app deployed.
* @property instances The breakdown info for app instances on all the servers
* @property jarFileLocation The jar file location on the server.
* @property jvmMemoryInMB The jvm heap memory allocated.
* @property jvmOptions The jvm options.
* @property labels Resource labels
* @property lastModifiedTime Time when this springbootapps jar file was last modified.
* @property lastUpdatedTime Time when this springbootapps instance was last refreshed.
* @property machineArmIds The machine ARM id list the app belongs to.
* @property miscs The other types of date collected.
* @property provisioningState The resource provisioning state.
* @property runtimeJdkVersion The jdk version installed on server
* @property servers The server list the app installed
* @property springBootVersion The spring boot version.
* @property staticContentLocations The static content location list.
*/
public data class SpringbootappsPropertiesResponse(
public val appName: String? = null,
public val appPort: Int? = null,
public val appType: String? = null,
public val applicationConfigurations: List? = null,
public val artifactName: String? = null,
public val bindingPorts: List? = null,
public val buildJdkVersion: String? = null,
public val certificates: List? = null,
public val checksum: String? = null,
public val connectionStrings: List? = null,
public val dependencies: List? = null,
public val environments: List? = null,
public val errors: List? = null,
public val instanceCount: Int? = null,
public val instances: List? = null,
public val jarFileLocation: String? = null,
public val jvmMemoryInMB: Int? = null,
public val jvmOptions: List? = null,
public val labels: Map? = null,
public val lastModifiedTime: String? = null,
public val lastUpdatedTime: String? = null,
public val machineArmIds: List? = null,
public val miscs: List? = null,
public val provisioningState: String,
public val runtimeJdkVersion: String? = null,
public val servers: List? = null,
public val springBootVersion: String? = null,
public val staticContentLocations: List? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.azurenative.offazurespringboot.outputs.SpringbootappsPropertiesResponse): SpringbootappsPropertiesResponse = SpringbootappsPropertiesResponse(
appName = javaType.appName().map({ args0 -> args0 }).orElse(null),
appPort = javaType.appPort().map({ args0 -> args0 }).orElse(null),
appType = javaType.appType().map({ args0 -> args0 }).orElse(null),
applicationConfigurations = javaType.applicationConfigurations().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.offazurespringboot.kotlin.outputs.SpringbootappsPropertiesResponseApplicationConfigurations.Companion.toKotlin(args0)
})
}),
artifactName = javaType.artifactName().map({ args0 -> args0 }).orElse(null),
bindingPorts = javaType.bindingPorts().map({ args0 -> args0 }),
buildJdkVersion = javaType.buildJdkVersion().map({ args0 -> args0 }).orElse(null),
certificates = javaType.certificates().map({ args0 -> args0 }),
checksum = javaType.checksum().map({ args0 -> args0 }).orElse(null),
connectionStrings = javaType.connectionStrings().map({ args0 -> args0 }),
dependencies = javaType.dependencies().map({ args0 -> args0 }),
environments = javaType.environments().map({ args0 -> args0 }),
errors = javaType.errors().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.offazurespringboot.kotlin.outputs.ErrorResponse.Companion.toKotlin(args0)
})
}),
instanceCount = javaType.instanceCount().map({ args0 -> args0 }).orElse(null),
instances = javaType.instances().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.offazurespringboot.kotlin.outputs.SpringbootappsPropertiesResponseInstances.Companion.toKotlin(args0)
})
}),
jarFileLocation = javaType.jarFileLocation().map({ args0 -> args0 }).orElse(null),
jvmMemoryInMB = javaType.jvmMemoryInMB().map({ args0 -> args0 }).orElse(null),
jvmOptions = javaType.jvmOptions().map({ args0 -> args0 }),
labels = javaType.labels().map({ args0 -> args0.key.to(args0.value) }).toMap(),
lastModifiedTime = javaType.lastModifiedTime().map({ args0 -> args0 }).orElse(null),
lastUpdatedTime = javaType.lastUpdatedTime().map({ args0 -> args0 }).orElse(null),
machineArmIds = javaType.machineArmIds().map({ args0 -> args0 }),
miscs = javaType.miscs().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.offazurespringboot.kotlin.outputs.SpringbootappsPropertiesResponseMiscs.Companion.toKotlin(args0)
})
}),
provisioningState = javaType.provisioningState(),
runtimeJdkVersion = javaType.runtimeJdkVersion().map({ args0 -> args0 }).orElse(null),
servers = javaType.servers().map({ args0 -> args0 }),
springBootVersion = javaType.springBootVersion().map({ args0 -> args0 }).orElse(null),
staticContentLocations = javaType.staticContentLocations().map({ args0 -> args0 }),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy