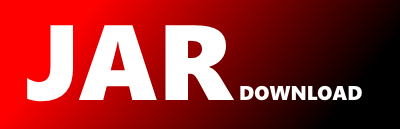
com.pulumi.azurenative.openenergyplatform.kotlin.OpenenergyplatformFunctions.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.openenergyplatform.kotlin
import com.pulumi.azurenative.openenergyplatform.OpenenergyplatformFunctions.getEnergyServicePlain
import com.pulumi.azurenative.openenergyplatform.OpenenergyplatformFunctions.listEnergyServicePartitionsPlain
import com.pulumi.azurenative.openenergyplatform.kotlin.inputs.GetEnergyServicePlainArgs
import com.pulumi.azurenative.openenergyplatform.kotlin.inputs.GetEnergyServicePlainArgsBuilder
import com.pulumi.azurenative.openenergyplatform.kotlin.inputs.ListEnergyServicePartitionsPlainArgs
import com.pulumi.azurenative.openenergyplatform.kotlin.inputs.ListEnergyServicePartitionsPlainArgsBuilder
import com.pulumi.azurenative.openenergyplatform.kotlin.outputs.GetEnergyServiceResult
import com.pulumi.azurenative.openenergyplatform.kotlin.outputs.ListEnergyServicePartitionsResult
import kotlinx.coroutines.future.await
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import com.pulumi.azurenative.openenergyplatform.kotlin.outputs.GetEnergyServiceResult.Companion.toKotlin as getEnergyServiceResultToKotlin
import com.pulumi.azurenative.openenergyplatform.kotlin.outputs.ListEnergyServicePartitionsResult.Companion.toKotlin as listEnergyServicePartitionsResultToKotlin
public object OpenenergyplatformFunctions {
/**
* Returns oep resource for a given name.
* Azure REST API version: 2022-04-04-preview.
* @param argument null
* @return null
*/
public suspend fun getEnergyService(argument: GetEnergyServicePlainArgs): GetEnergyServiceResult =
getEnergyServiceResultToKotlin(getEnergyServicePlain(argument.toJava()).await())
/**
* @see [getEnergyService].
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param resourceName The resource name.
* @return null
*/
public suspend fun getEnergyService(resourceGroupName: String, resourceName: String): GetEnergyServiceResult {
val argument = GetEnergyServicePlainArgs(
resourceGroupName = resourceGroupName,
resourceName = resourceName,
)
return getEnergyServiceResultToKotlin(getEnergyServicePlain(argument.toJava()).await())
}
/**
* @see [getEnergyService].
* @param argument Builder for [com.pulumi.azurenative.openenergyplatform.kotlin.inputs.GetEnergyServicePlainArgs].
* @return null
*/
public suspend fun getEnergyService(argument: suspend GetEnergyServicePlainArgsBuilder.() -> Unit): GetEnergyServiceResult {
val builder = GetEnergyServicePlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getEnergyServiceResultToKotlin(getEnergyServicePlain(builtArgument.toJava()).await())
}
/**
* Method that gets called when list of partitions is requested.
* Azure REST API version: 2022-04-04-preview.
* @param argument null
* @return List of data partitions.
*/
public suspend fun listEnergyServicePartitions(argument: ListEnergyServicePartitionsPlainArgs): ListEnergyServicePartitionsResult =
listEnergyServicePartitionsResultToKotlin(listEnergyServicePartitionsPlain(argument.toJava()).await())
/**
* @see [listEnergyServicePartitions].
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param resourceName The resource name.
* @return List of data partitions.
*/
public suspend fun listEnergyServicePartitions(resourceGroupName: String, resourceName: String): ListEnergyServicePartitionsResult {
val argument = ListEnergyServicePartitionsPlainArgs(
resourceGroupName = resourceGroupName,
resourceName = resourceName,
)
return listEnergyServicePartitionsResultToKotlin(listEnergyServicePartitionsPlain(argument.toJava()).await())
}
/**
* @see [listEnergyServicePartitions].
* @param argument Builder for [com.pulumi.azurenative.openenergyplatform.kotlin.inputs.ListEnergyServicePartitionsPlainArgs].
* @return List of data partitions.
*/
public suspend fun listEnergyServicePartitions(argument: suspend ListEnergyServicePartitionsPlainArgsBuilder.() -> Unit): ListEnergyServicePartitionsResult {
val builder = ListEnergyServicePartitionsPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return listEnergyServicePartitionsResultToKotlin(listEnergyServicePartitionsPlain(builtArgument.toJava()).await())
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy