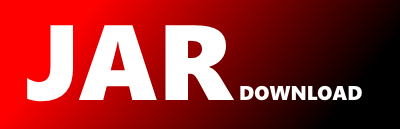
com.pulumi.azurenative.operationalinsights.kotlin.Cluster.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.operationalinsights.kotlin
import com.pulumi.azurenative.operationalinsights.kotlin.outputs.AssociatedWorkspaceResponse
import com.pulumi.azurenative.operationalinsights.kotlin.outputs.CapacityReservationPropertiesResponse
import com.pulumi.azurenative.operationalinsights.kotlin.outputs.ClusterSkuResponse
import com.pulumi.azurenative.operationalinsights.kotlin.outputs.IdentityResponse
import com.pulumi.azurenative.operationalinsights.kotlin.outputs.KeyVaultPropertiesResponse
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import com.pulumi.azurenative.operationalinsights.kotlin.outputs.AssociatedWorkspaceResponse.Companion.toKotlin as associatedWorkspaceResponseToKotlin
import com.pulumi.azurenative.operationalinsights.kotlin.outputs.CapacityReservationPropertiesResponse.Companion.toKotlin as capacityReservationPropertiesResponseToKotlin
import com.pulumi.azurenative.operationalinsights.kotlin.outputs.ClusterSkuResponse.Companion.toKotlin as clusterSkuResponseToKotlin
import com.pulumi.azurenative.operationalinsights.kotlin.outputs.IdentityResponse.Companion.toKotlin as identityResponseToKotlin
import com.pulumi.azurenative.operationalinsights.kotlin.outputs.KeyVaultPropertiesResponse.Companion.toKotlin as keyVaultPropertiesResponseToKotlin
/**
* Builder for [Cluster].
*/
@PulumiTagMarker
public class ClusterResourceBuilder internal constructor() {
public var name: String? = null
public var args: ClusterArgs = ClusterArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend ClusterArgsBuilder.() -> Unit) {
val builder = ClusterArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Cluster {
val builtJavaResource =
com.pulumi.azurenative.operationalinsights.Cluster(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Cluster(builtJavaResource)
}
}
/**
* The top level Log Analytics cluster resource container.
* Azure REST API version: 2021-06-01. Prior API version in Azure Native 1.x: 2020-10-01.
* Other available API versions: 2019-08-01-preview, 2020-08-01, 2022-10-01.
* ## Example Usage
* ### ClustersCreate
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var cluster = new AzureNative.OperationalInsights.Cluster("cluster", new()
* {
* ClusterName = "oiautorest6685",
* Location = "australiasoutheast",
* ResourceGroupName = "oiautorest6685",
* Sku = new AzureNative.OperationalInsights.Inputs.ClusterSkuArgs
* {
* Capacity = 1000,
* Name = AzureNative.OperationalInsights.ClusterSkuNameEnum.CapacityReservation,
* },
* Tags =
* {
* { "tag1", "val1" },
* },
* });
* });
* ```
* ```go
* package main
* import (
* operationalinsights "github.com/pulumi/pulumi-azure-native-sdk/operationalinsights/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := operationalinsights.NewCluster(ctx, "cluster", &operationalinsights.ClusterArgs{
* ClusterName: pulumi.String("oiautorest6685"),
* Location: pulumi.String("australiasoutheast"),
* ResourceGroupName: pulumi.String("oiautorest6685"),
* Sku: &operationalinsights.ClusterSkuArgs{
* Capacity: pulumi.Float64(1000),
* Name: pulumi.String(operationalinsights.ClusterSkuNameEnumCapacityReservation),
* },
* Tags: pulumi.StringMap{
* "tag1": pulumi.String("val1"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.operationalinsights.Cluster;
* import com.pulumi.azurenative.operationalinsights.ClusterArgs;
* import com.pulumi.azurenative.operationalinsights.inputs.ClusterSkuArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var cluster = new Cluster("cluster", ClusterArgs.builder()
* .clusterName("oiautorest6685")
* .location("australiasoutheast")
* .resourceGroupName("oiautorest6685")
* .sku(ClusterSkuArgs.builder()
* .capacity(1000)
* .name("CapacityReservation")
* .build())
* .tags(Map.of("tag1", "val1"))
* .build());
* }
* }
* ```
* ## Import
* An existing resource can be imported using its type token, name, and identifier, e.g.
* ```sh
* $ pulumi import azure-native:operationalinsights:Cluster oiautorest6685 /subscriptions/{subscriptionId}/resourcegroups/{resourceGroupName}/providers/Microsoft.OperationalInsights/clusters/{clusterName}
* ```
*/
public class Cluster internal constructor(
override val javaResource: com.pulumi.azurenative.operationalinsights.Cluster,
) : KotlinCustomResource(javaResource, ClusterMapper) {
/**
* The list of Log Analytics workspaces associated with the cluster
*/
public val associatedWorkspaces: Output>?
get() = javaResource.associatedWorkspaces().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
associatedWorkspaceResponseToKotlin(args0)
})
})
}).orElse(null)
})
/**
* The cluster's billing type.
*/
public val billingType: Output?
get() = javaResource.billingType().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Additional properties for capacity reservation
*/
public val capacityReservationProperties: Output?
get() = javaResource.capacityReservationProperties().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> capacityReservationPropertiesResponseToKotlin(args0) })
}).orElse(null)
})
/**
* The ID associated with the cluster.
*/
public val clusterId: Output
get() = javaResource.clusterId().applyValue({ args0 -> args0 })
/**
* The cluster creation time
*/
public val createdDate: Output
get() = javaResource.createdDate().applyValue({ args0 -> args0 })
/**
* The identity of the resource.
*/
public val identity: Output?
get() = javaResource.identity().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
identityResponseToKotlin(args0)
})
}).orElse(null)
})
/**
* Sets whether the cluster will support availability zones. This can be set as true only in regions where Azure Data Explorer support Availability Zones. This Property can not be modified after cluster creation. Default value is 'true' if region supports Availability Zones.
*/
public val isAvailabilityZonesEnabled: Output?
get() = javaResource.isAvailabilityZonesEnabled().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Configures whether cluster will use double encryption. This Property can not be modified after cluster creation. Default value is 'true'
*/
public val isDoubleEncryptionEnabled: Output?
get() = javaResource.isDoubleEncryptionEnabled().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The associated key properties.
*/
public val keyVaultProperties: Output?
get() = javaResource.keyVaultProperties().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> keyVaultPropertiesResponseToKotlin(args0) })
}).orElse(null)
})
/**
* The last time the cluster was updated.
*/
public val lastModifiedDate: Output
get() = javaResource.lastModifiedDate().applyValue({ args0 -> args0 })
/**
* The geo-location where the resource lives
*/
public val location: Output
get() = javaResource.location().applyValue({ args0 -> args0 })
/**
* The name of the resource
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* The provisioning state of the cluster.
*/
public val provisioningState: Output
get() = javaResource.provisioningState().applyValue({ args0 -> args0 })
/**
* The sku properties.
*/
public val sku: Output?
get() = javaResource.sku().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
clusterSkuResponseToKotlin(args0)
})
}).orElse(null)
})
/**
* Resource tags.
*/
public val tags: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy