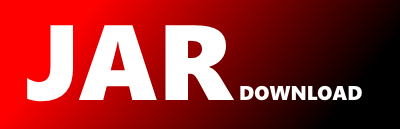
com.pulumi.azurenative.peering.kotlin.PeeringArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.peering.kotlin
import com.pulumi.azurenative.peering.PeeringArgs.builder
import com.pulumi.azurenative.peering.kotlin.enums.Kind
import com.pulumi.azurenative.peering.kotlin.inputs.PeeringPropertiesDirectArgs
import com.pulumi.azurenative.peering.kotlin.inputs.PeeringPropertiesDirectArgsBuilder
import com.pulumi.azurenative.peering.kotlin.inputs.PeeringPropertiesExchangeArgs
import com.pulumi.azurenative.peering.kotlin.inputs.PeeringPropertiesExchangeArgsBuilder
import com.pulumi.azurenative.peering.kotlin.inputs.PeeringSkuArgs
import com.pulumi.azurenative.peering.kotlin.inputs.PeeringSkuArgsBuilder
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* Peering is a logical representation of a set of connections to the Microsoft Cloud Edge at a location.
* Azure REST API version: 2022-10-01. Prior API version in Azure Native 1.x: 2021-01-01.
* ## Example Usage
* ### Create a direct peering
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var peering = new AzureNative.Peering.Peering("peering", new()
* {
* Direct = new AzureNative.Peering.Inputs.PeeringPropertiesDirectArgs
* {
* Connections = new[]
* {
* new AzureNative.Peering.Inputs.DirectConnectionArgs
* {
* BandwidthInMbps = 10000,
* BgpSession = new AzureNative.Peering.Inputs.BgpSessionArgs
* {
* MaxPrefixesAdvertisedV4 = 1000,
* MaxPrefixesAdvertisedV6 = 100,
* Md5AuthenticationKey = "test-md5-auth-key",
* SessionPrefixV4 = "192.168.0.0/31",
* SessionPrefixV6 = "fd00::0/127",
* },
* ConnectionIdentifier = "5F4CB5C7-6B43-4444-9338-9ABC72606C16",
* PeeringDBFacilityId = 99999,
* SessionAddressProvider = AzureNative.Peering.SessionAddressProvider.Peer,
* UseForPeeringService = false,
* },
* new AzureNative.Peering.Inputs.DirectConnectionArgs
* {
* BandwidthInMbps = 10000,
* ConnectionIdentifier = "8AB00818-D533-4504-A25A-03A17F61201C",
* PeeringDBFacilityId = 99999,
* SessionAddressProvider = AzureNative.Peering.SessionAddressProvider.Microsoft,
* UseForPeeringService = true,
* },
* },
* DirectPeeringType = AzureNative.Peering.DirectPeeringType.Edge,
* PeerAsn = new AzureNative.Peering.Inputs.SubResourceArgs
* {
* Id = "/subscriptions/subId/providers/Microsoft.Peering/peerAsns/myAsn1",
* },
* },
* Kind = AzureNative.Peering.Kind.Direct,
* Location = "eastus",
* PeeringLocation = "peeringLocation0",
* PeeringName = "peeringName",
* ResourceGroupName = "rgName",
* Sku = new AzureNative.Peering.Inputs.PeeringSkuArgs
* {
* Name = "Basic_Direct_Free",
* },
* });
* });
* ```
* ```go
* package main
* import (
* peering "github.com/pulumi/pulumi-azure-native-sdk/peering/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := peering.NewPeering(ctx, "peering", &peering.PeeringArgs{
* Direct: &peering.PeeringPropertiesDirectArgs{
* Connections: peering.DirectConnectionArray{
* &peering.DirectConnectionArgs{
* BandwidthInMbps: pulumi.Int(10000),
* BgpSession: &peering.BgpSessionArgs{
* MaxPrefixesAdvertisedV4: pulumi.Int(1000),
* MaxPrefixesAdvertisedV6: pulumi.Int(100),
* Md5AuthenticationKey: pulumi.String("test-md5-auth-key"),
* SessionPrefixV4: pulumi.String("192.168.0.0/31"),
* SessionPrefixV6: pulumi.String("fd00::0/127"),
* },
* ConnectionIdentifier: pulumi.String("5F4CB5C7-6B43-4444-9338-9ABC72606C16"),
* PeeringDBFacilityId: pulumi.Int(99999),
* SessionAddressProvider: pulumi.String(peering.SessionAddressProviderPeer),
* UseForPeeringService: pulumi.Bool(false),
* },
* &peering.DirectConnectionArgs{
* BandwidthInMbps: pulumi.Int(10000),
* ConnectionIdentifier: pulumi.String("8AB00818-D533-4504-A25A-03A17F61201C"),
* PeeringDBFacilityId: pulumi.Int(99999),
* SessionAddressProvider: pulumi.String(peering.SessionAddressProviderMicrosoft),
* UseForPeeringService: pulumi.Bool(true),
* },
* },
* DirectPeeringType: pulumi.String(peering.DirectPeeringTypeEdge),
* PeerAsn: &peering.SubResourceArgs{
* Id: pulumi.String("/subscriptions/subId/providers/Microsoft.Peering/peerAsns/myAsn1"),
* },
* },
* Kind: pulumi.String(peering.KindDirect),
* Location: pulumi.String("eastus"),
* PeeringLocation: pulumi.String("peeringLocation0"),
* PeeringName: pulumi.String("peeringName"),
* ResourceGroupName: pulumi.String("rgName"),
* Sku: &peering.PeeringSkuArgs{
* Name: pulumi.String("Basic_Direct_Free"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.peering.Peering;
* import com.pulumi.azurenative.peering.PeeringArgs;
* import com.pulumi.azurenative.peering.inputs.PeeringPropertiesDirectArgs;
* import com.pulumi.azurenative.peering.inputs.SubResourceArgs;
* import com.pulumi.azurenative.peering.inputs.PeeringSkuArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var peering = new Peering("peering", PeeringArgs.builder()
* .direct(PeeringPropertiesDirectArgs.builder()
* .connections(
* DirectConnectionArgs.builder()
* .bandwidthInMbps(10000)
* .bgpSession(BgpSessionArgs.builder()
* .maxPrefixesAdvertisedV4(1000)
* .maxPrefixesAdvertisedV6(100)
* .md5AuthenticationKey("test-md5-auth-key")
* .sessionPrefixV4("192.168.0.0/31")
* .sessionPrefixV6("fd00::0/127")
* .build())
* .connectionIdentifier("5F4CB5C7-6B43-4444-9338-9ABC72606C16")
* .peeringDBFacilityId(99999)
* .sessionAddressProvider("Peer")
* .useForPeeringService(false)
* .build(),
* DirectConnectionArgs.builder()
* .bandwidthInMbps(10000)
* .connectionIdentifier("8AB00818-D533-4504-A25A-03A17F61201C")
* .peeringDBFacilityId(99999)
* .sessionAddressProvider("Microsoft")
* .useForPeeringService(true)
* .build())
* .directPeeringType("Edge")
* .peerAsn(SubResourceArgs.builder()
* .id("/subscriptions/subId/providers/Microsoft.Peering/peerAsns/myAsn1")
* .build())
* .build())
* .kind("Direct")
* .location("eastus")
* .peeringLocation("peeringLocation0")
* .peeringName("peeringName")
* .resourceGroupName("rgName")
* .sku(PeeringSkuArgs.builder()
* .name("Basic_Direct_Free")
* .build())
* .build());
* }
* }
* ```
* ### Create a peering with exchange route server
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var peering = new AzureNative.Peering.Peering("peering", new()
* {
* Direct = new AzureNative.Peering.Inputs.PeeringPropertiesDirectArgs
* {
* Connections = new[]
* {
* new AzureNative.Peering.Inputs.DirectConnectionArgs
* {
* BandwidthInMbps = 10000,
* BgpSession = new AzureNative.Peering.Inputs.BgpSessionArgs
* {
* MaxPrefixesAdvertisedV4 = 1000,
* MaxPrefixesAdvertisedV6 = 100,
* MicrosoftSessionIPv4Address = "192.168.0.123",
* PeerSessionIPv4Address = "192.168.0.234",
* SessionPrefixV4 = "192.168.0.0/24",
* },
* ConnectionIdentifier = "5F4CB5C7-6B43-4444-9338-9ABC72606C16",
* PeeringDBFacilityId = 99999,
* SessionAddressProvider = AzureNative.Peering.SessionAddressProvider.Peer,
* UseForPeeringService = true,
* },
* },
* DirectPeeringType = AzureNative.Peering.DirectPeeringType.IxRs,
* PeerAsn = new AzureNative.Peering.Inputs.SubResourceArgs
* {
* Id = "/subscriptions/subId/providers/Microsoft.Peering/peerAsns/myAsn1",
* },
* },
* Kind = AzureNative.Peering.Kind.Direct,
* Location = "eastus",
* PeeringLocation = "peeringLocation0",
* PeeringName = "peeringName",
* ResourceGroupName = "rgName",
* Sku = new AzureNative.Peering.Inputs.PeeringSkuArgs
* {
* Name = "Premium_Direct_Free",
* },
* });
* });
* ```
* ```go
* package main
* import (
* peering "github.com/pulumi/pulumi-azure-native-sdk/peering/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := peering.NewPeering(ctx, "peering", &peering.PeeringArgs{
* Direct: &peering.PeeringPropertiesDirectArgs{
* Connections: peering.DirectConnectionArray{
* &peering.DirectConnectionArgs{
* BandwidthInMbps: pulumi.Int(10000),
* BgpSession: &peering.BgpSessionArgs{
* MaxPrefixesAdvertisedV4: pulumi.Int(1000),
* MaxPrefixesAdvertisedV6: pulumi.Int(100),
* MicrosoftSessionIPv4Address: pulumi.String("192.168.0.123"),
* PeerSessionIPv4Address: pulumi.String("192.168.0.234"),
* SessionPrefixV4: pulumi.String("192.168.0.0/24"),
* },
* ConnectionIdentifier: pulumi.String("5F4CB5C7-6B43-4444-9338-9ABC72606C16"),
* PeeringDBFacilityId: pulumi.Int(99999),
* SessionAddressProvider: pulumi.String(peering.SessionAddressProviderPeer),
* UseForPeeringService: pulumi.Bool(true),
* },
* },
* DirectPeeringType: pulumi.String(peering.DirectPeeringTypeIxRs),
* PeerAsn: &peering.SubResourceArgs{
* Id: pulumi.String("/subscriptions/subId/providers/Microsoft.Peering/peerAsns/myAsn1"),
* },
* },
* Kind: pulumi.String(peering.KindDirect),
* Location: pulumi.String("eastus"),
* PeeringLocation: pulumi.String("peeringLocation0"),
* PeeringName: pulumi.String("peeringName"),
* ResourceGroupName: pulumi.String("rgName"),
* Sku: &peering.PeeringSkuArgs{
* Name: pulumi.String("Premium_Direct_Free"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.peering.Peering;
* import com.pulumi.azurenative.peering.PeeringArgs;
* import com.pulumi.azurenative.peering.inputs.PeeringPropertiesDirectArgs;
* import com.pulumi.azurenative.peering.inputs.SubResourceArgs;
* import com.pulumi.azurenative.peering.inputs.PeeringSkuArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var peering = new Peering("peering", PeeringArgs.builder()
* .direct(PeeringPropertiesDirectArgs.builder()
* .connections(DirectConnectionArgs.builder()
* .bandwidthInMbps(10000)
* .bgpSession(BgpSessionArgs.builder()
* .maxPrefixesAdvertisedV4(1000)
* .maxPrefixesAdvertisedV6(100)
* .microsoftSessionIPv4Address("192.168.0.123")
* .peerSessionIPv4Address("192.168.0.234")
* .sessionPrefixV4("192.168.0.0/24")
* .build())
* .connectionIdentifier("5F4CB5C7-6B43-4444-9338-9ABC72606C16")
* .peeringDBFacilityId(99999)
* .sessionAddressProvider("Peer")
* .useForPeeringService(true)
* .build())
* .directPeeringType("IxRs")
* .peerAsn(SubResourceArgs.builder()
* .id("/subscriptions/subId/providers/Microsoft.Peering/peerAsns/myAsn1")
* .build())
* .build())
* .kind("Direct")
* .location("eastus")
* .peeringLocation("peeringLocation0")
* .peeringName("peeringName")
* .resourceGroupName("rgName")
* .sku(PeeringSkuArgs.builder()
* .name("Premium_Direct_Free")
* .build())
* .build());
* }
* }
* ```
* ### Create an exchange peering
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var peering = new AzureNative.Peering.Peering("peering", new()
* {
* Exchange = new AzureNative.Peering.Inputs.PeeringPropertiesExchangeArgs
* {
* Connections = new[]
* {
* new AzureNative.Peering.Inputs.ExchangeConnectionArgs
* {
* BgpSession = new AzureNative.Peering.Inputs.BgpSessionArgs
* {
* MaxPrefixesAdvertisedV4 = 1000,
* MaxPrefixesAdvertisedV6 = 100,
* Md5AuthenticationKey = "test-md5-auth-key",
* PeerSessionIPv4Address = "192.168.2.1",
* PeerSessionIPv6Address = "fd00::1",
* },
* ConnectionIdentifier = "CE495334-0E94-4E51-8164-8116D6CD284D",
* PeeringDBFacilityId = 99999,
* },
* new AzureNative.Peering.Inputs.ExchangeConnectionArgs
* {
* BgpSession = new AzureNative.Peering.Inputs.BgpSessionArgs
* {
* MaxPrefixesAdvertisedV4 = 1000,
* MaxPrefixesAdvertisedV6 = 100,
* Md5AuthenticationKey = "test-md5-auth-key",
* PeerSessionIPv4Address = "192.168.2.2",
* PeerSessionIPv6Address = "fd00::2",
* },
* ConnectionIdentifier = "CDD8E673-CB07-47E6-84DE-3739F778762B",
* PeeringDBFacilityId = 99999,
* },
* },
* PeerAsn = new AzureNative.Peering.Inputs.SubResourceArgs
* {
* Id = "/subscriptions/subId/providers/Microsoft.Peering/peerAsns/myAsn1",
* },
* },
* Kind = AzureNative.Peering.Kind.Exchange,
* Location = "eastus",
* PeeringLocation = "peeringLocation0",
* PeeringName = "peeringName",
* ResourceGroupName = "rgName",
* Sku = new AzureNative.Peering.Inputs.PeeringSkuArgs
* {
* Name = "Basic_Exchange_Free",
* },
* });
* });
* ```
* ```go
* package main
* import (
* peering "github.com/pulumi/pulumi-azure-native-sdk/peering/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := peering.NewPeering(ctx, "peering", &peering.PeeringArgs{
* Exchange: &peering.PeeringPropertiesExchangeArgs{
* Connections: peering.ExchangeConnectionArray{
* &peering.ExchangeConnectionArgs{
* BgpSession: &peering.BgpSessionArgs{
* MaxPrefixesAdvertisedV4: pulumi.Int(1000),
* MaxPrefixesAdvertisedV6: pulumi.Int(100),
* Md5AuthenticationKey: pulumi.String("test-md5-auth-key"),
* PeerSessionIPv4Address: pulumi.String("192.168.2.1"),
* PeerSessionIPv6Address: pulumi.String("fd00::1"),
* },
* ConnectionIdentifier: pulumi.String("CE495334-0E94-4E51-8164-8116D6CD284D"),
* PeeringDBFacilityId: pulumi.Int(99999),
* },
* &peering.ExchangeConnectionArgs{
* BgpSession: &peering.BgpSessionArgs{
* MaxPrefixesAdvertisedV4: pulumi.Int(1000),
* MaxPrefixesAdvertisedV6: pulumi.Int(100),
* Md5AuthenticationKey: pulumi.String("test-md5-auth-key"),
* PeerSessionIPv4Address: pulumi.String("192.168.2.2"),
* PeerSessionIPv6Address: pulumi.String("fd00::2"),
* },
* ConnectionIdentifier: pulumi.String("CDD8E673-CB07-47E6-84DE-3739F778762B"),
* PeeringDBFacilityId: pulumi.Int(99999),
* },
* },
* PeerAsn: &peering.SubResourceArgs{
* Id: pulumi.String("/subscriptions/subId/providers/Microsoft.Peering/peerAsns/myAsn1"),
* },
* },
* Kind: pulumi.String(peering.KindExchange),
* Location: pulumi.String("eastus"),
* PeeringLocation: pulumi.String("peeringLocation0"),
* PeeringName: pulumi.String("peeringName"),
* ResourceGroupName: pulumi.String("rgName"),
* Sku: &peering.PeeringSkuArgs{
* Name: pulumi.String("Basic_Exchange_Free"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.peering.Peering;
* import com.pulumi.azurenative.peering.PeeringArgs;
* import com.pulumi.azurenative.peering.inputs.PeeringPropertiesExchangeArgs;
* import com.pulumi.azurenative.peering.inputs.SubResourceArgs;
* import com.pulumi.azurenative.peering.inputs.PeeringSkuArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var peering = new Peering("peering", PeeringArgs.builder()
* .exchange(PeeringPropertiesExchangeArgs.builder()
* .connections(
* ExchangeConnectionArgs.builder()
* .bgpSession(BgpSessionArgs.builder()
* .maxPrefixesAdvertisedV4(1000)
* .maxPrefixesAdvertisedV6(100)
* .md5AuthenticationKey("test-md5-auth-key")
* .peerSessionIPv4Address("192.168.2.1")
* .peerSessionIPv6Address("fd00::1")
* .build())
* .connectionIdentifier("CE495334-0E94-4E51-8164-8116D6CD284D")
* .peeringDBFacilityId(99999)
* .build(),
* ExchangeConnectionArgs.builder()
* .bgpSession(BgpSessionArgs.builder()
* .maxPrefixesAdvertisedV4(1000)
* .maxPrefixesAdvertisedV6(100)
* .md5AuthenticationKey("test-md5-auth-key")
* .peerSessionIPv4Address("192.168.2.2")
* .peerSessionIPv6Address("fd00::2")
* .build())
* .connectionIdentifier("CDD8E673-CB07-47E6-84DE-3739F778762B")
* .peeringDBFacilityId(99999)
* .build())
* .peerAsn(SubResourceArgs.builder()
* .id("/subscriptions/subId/providers/Microsoft.Peering/peerAsns/myAsn1")
* .build())
* .build())
* .kind("Exchange")
* .location("eastus")
* .peeringLocation("peeringLocation0")
* .peeringName("peeringName")
* .resourceGroupName("rgName")
* .sku(PeeringSkuArgs.builder()
* .name("Basic_Exchange_Free")
* .build())
* .build());
* }
* }
* ```
* ## Import
* An existing resource can be imported using its type token, name, and identifier, e.g.
* ```sh
* $ pulumi import azure-native:peering:Peering peeringName /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Peering/peerings/{peeringName}
* ```
* @property direct The properties that define a direct peering.
* @property exchange The properties that define an exchange peering.
* @property kind The kind of the peering.
* @property location The location of the resource.
* @property peeringLocation The location of the peering.
* @property peeringName The name of the peering.
* @property resourceGroupName The name of the resource group.
* @property sku The SKU that defines the tier and kind of the peering.
* @property tags The resource tags.
*/
public data class PeeringArgs(
public val direct: Output? = null,
public val exchange: Output? = null,
public val kind: Output>? = null,
public val location: Output? = null,
public val peeringLocation: Output? = null,
public val peeringName: Output? = null,
public val resourceGroupName: Output? = null,
public val sku: Output? = null,
public val tags: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy