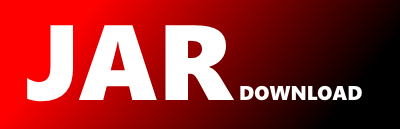
com.pulumi.azurenative.peering.kotlin.inputs.BgpSessionArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.peering.kotlin.inputs
import com.pulumi.azurenative.peering.inputs.BgpSessionArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* The properties that define a BGP session.
* @property maxPrefixesAdvertisedV4 The maximum number of prefixes advertised over the IPv4 session.
* @property maxPrefixesAdvertisedV6 The maximum number of prefixes advertised over the IPv6 session.
* @property md5AuthenticationKey The MD5 authentication key of the session.
* @property microsoftSessionIPv4Address The IPv4 session address on Microsoft's end.
* @property microsoftSessionIPv6Address The IPv6 session address on Microsoft's end.
* @property peerSessionIPv4Address The IPv4 session address on peer's end.
* @property peerSessionIPv6Address The IPv6 session address on peer's end.
* @property sessionPrefixV4 The IPv4 prefix that contains both ends' IPv4 addresses.
* @property sessionPrefixV6 The IPv6 prefix that contains both ends' IPv6 addresses.
*/
public data class BgpSessionArgs(
public val maxPrefixesAdvertisedV4: Output? = null,
public val maxPrefixesAdvertisedV6: Output? = null,
public val md5AuthenticationKey: Output? = null,
public val microsoftSessionIPv4Address: Output? = null,
public val microsoftSessionIPv6Address: Output? = null,
public val peerSessionIPv4Address: Output? = null,
public val peerSessionIPv6Address: Output? = null,
public val sessionPrefixV4: Output? = null,
public val sessionPrefixV6: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.peering.inputs.BgpSessionArgs =
com.pulumi.azurenative.peering.inputs.BgpSessionArgs.builder()
.maxPrefixesAdvertisedV4(maxPrefixesAdvertisedV4?.applyValue({ args0 -> args0 }))
.maxPrefixesAdvertisedV6(maxPrefixesAdvertisedV6?.applyValue({ args0 -> args0 }))
.md5AuthenticationKey(md5AuthenticationKey?.applyValue({ args0 -> args0 }))
.microsoftSessionIPv4Address(microsoftSessionIPv4Address?.applyValue({ args0 -> args0 }))
.microsoftSessionIPv6Address(microsoftSessionIPv6Address?.applyValue({ args0 -> args0 }))
.peerSessionIPv4Address(peerSessionIPv4Address?.applyValue({ args0 -> args0 }))
.peerSessionIPv6Address(peerSessionIPv6Address?.applyValue({ args0 -> args0 }))
.sessionPrefixV4(sessionPrefixV4?.applyValue({ args0 -> args0 }))
.sessionPrefixV6(sessionPrefixV6?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [BgpSessionArgs].
*/
@PulumiTagMarker
public class BgpSessionArgsBuilder internal constructor() {
private var maxPrefixesAdvertisedV4: Output? = null
private var maxPrefixesAdvertisedV6: Output? = null
private var md5AuthenticationKey: Output? = null
private var microsoftSessionIPv4Address: Output? = null
private var microsoftSessionIPv6Address: Output? = null
private var peerSessionIPv4Address: Output? = null
private var peerSessionIPv6Address: Output? = null
private var sessionPrefixV4: Output? = null
private var sessionPrefixV6: Output? = null
/**
* @param value The maximum number of prefixes advertised over the IPv4 session.
*/
@JvmName("jahppiuvopmbduna")
public suspend fun maxPrefixesAdvertisedV4(`value`: Output) {
this.maxPrefixesAdvertisedV4 = value
}
/**
* @param value The maximum number of prefixes advertised over the IPv6 session.
*/
@JvmName("swywvnwnsbsrgfqk")
public suspend fun maxPrefixesAdvertisedV6(`value`: Output) {
this.maxPrefixesAdvertisedV6 = value
}
/**
* @param value The MD5 authentication key of the session.
*/
@JvmName("yabakmfigfocfqhf")
public suspend fun md5AuthenticationKey(`value`: Output) {
this.md5AuthenticationKey = value
}
/**
* @param value The IPv4 session address on Microsoft's end.
*/
@JvmName("uxloqjgvnbhgbpca")
public suspend fun microsoftSessionIPv4Address(`value`: Output) {
this.microsoftSessionIPv4Address = value
}
/**
* @param value The IPv6 session address on Microsoft's end.
*/
@JvmName("rwlkbsimsficjqtr")
public suspend fun microsoftSessionIPv6Address(`value`: Output) {
this.microsoftSessionIPv6Address = value
}
/**
* @param value The IPv4 session address on peer's end.
*/
@JvmName("xvmwipahwnukwpvp")
public suspend fun peerSessionIPv4Address(`value`: Output) {
this.peerSessionIPv4Address = value
}
/**
* @param value The IPv6 session address on peer's end.
*/
@JvmName("vhsrwnqdlihhidpd")
public suspend fun peerSessionIPv6Address(`value`: Output) {
this.peerSessionIPv6Address = value
}
/**
* @param value The IPv4 prefix that contains both ends' IPv4 addresses.
*/
@JvmName("dhthbechgjvnbhxx")
public suspend fun sessionPrefixV4(`value`: Output) {
this.sessionPrefixV4 = value
}
/**
* @param value The IPv6 prefix that contains both ends' IPv6 addresses.
*/
@JvmName("ggwmuhbvwhcmohql")
public suspend fun sessionPrefixV6(`value`: Output) {
this.sessionPrefixV6 = value
}
/**
* @param value The maximum number of prefixes advertised over the IPv4 session.
*/
@JvmName("mnytkeprdwkbnabu")
public suspend fun maxPrefixesAdvertisedV4(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxPrefixesAdvertisedV4 = mapped
}
/**
* @param value The maximum number of prefixes advertised over the IPv6 session.
*/
@JvmName("qjcfoylptgitqyfq")
public suspend fun maxPrefixesAdvertisedV6(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxPrefixesAdvertisedV6 = mapped
}
/**
* @param value The MD5 authentication key of the session.
*/
@JvmName("gwqrqpptencvbxak")
public suspend fun md5AuthenticationKey(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.md5AuthenticationKey = mapped
}
/**
* @param value The IPv4 session address on Microsoft's end.
*/
@JvmName("etsvvbiwrvprjygx")
public suspend fun microsoftSessionIPv4Address(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.microsoftSessionIPv4Address = mapped
}
/**
* @param value The IPv6 session address on Microsoft's end.
*/
@JvmName("brcdloqxibjyofdo")
public suspend fun microsoftSessionIPv6Address(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.microsoftSessionIPv6Address = mapped
}
/**
* @param value The IPv4 session address on peer's end.
*/
@JvmName("thfcwbfribvmomqd")
public suspend fun peerSessionIPv4Address(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.peerSessionIPv4Address = mapped
}
/**
* @param value The IPv6 session address on peer's end.
*/
@JvmName("ryprddicweyrxvdb")
public suspend fun peerSessionIPv6Address(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.peerSessionIPv6Address = mapped
}
/**
* @param value The IPv4 prefix that contains both ends' IPv4 addresses.
*/
@JvmName("lqfqfxoykcgyidfm")
public suspend fun sessionPrefixV4(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sessionPrefixV4 = mapped
}
/**
* @param value The IPv6 prefix that contains both ends' IPv6 addresses.
*/
@JvmName("oexdljvuulhyehmb")
public suspend fun sessionPrefixV6(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sessionPrefixV6 = mapped
}
internal fun build(): BgpSessionArgs = BgpSessionArgs(
maxPrefixesAdvertisedV4 = maxPrefixesAdvertisedV4,
maxPrefixesAdvertisedV6 = maxPrefixesAdvertisedV6,
md5AuthenticationKey = md5AuthenticationKey,
microsoftSessionIPv4Address = microsoftSessionIPv4Address,
microsoftSessionIPv6Address = microsoftSessionIPv6Address,
peerSessionIPv4Address = peerSessionIPv4Address,
peerSessionIPv6Address = peerSessionIPv6Address,
sessionPrefixV4 = sessionPrefixV4,
sessionPrefixV6 = sessionPrefixV6,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy