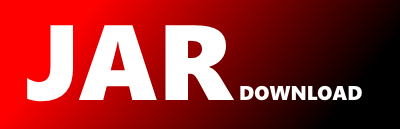
com.pulumi.azurenative.recoveryservices.kotlin.inputs.InnerHealthErrorArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.recoveryservices.kotlin.inputs
import com.pulumi.azurenative.recoveryservices.inputs.InnerHealthErrorArgs.builder
import com.pulumi.azurenative.recoveryservices.kotlin.enums.HealthErrorCustomerResolvability
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Implements InnerHealthError class. HealthError object has a list of InnerHealthErrors as child errors. InnerHealthError is used because this will prevent an infinite loop of structures when Hydra tries to auto-generate the contract. We are exposing the related health errors as inner health errors and all API consumers can utilize this in the same fashion as Exception -> InnerException.
* @property creationTimeUtc Error creation time (UTC).
* @property customerResolvability Value indicating whether the health error is customer resolvable.
* @property entityId ID of the entity.
* @property errorCategory Category of error.
* @property errorCode Error code.
* @property errorId The health error unique id.
* @property errorLevel Level of error.
* @property errorMessage Error message.
* @property errorSource Source of error.
* @property errorType Type of error.
* @property possibleCauses Possible causes of error.
* @property recommendedAction Recommended action to resolve error.
* @property recoveryProviderErrorMessage DRA error message.
* @property summaryMessage Summary message of the entity.
*/
public data class InnerHealthErrorArgs(
public val creationTimeUtc: Output? = null,
public val customerResolvability: Output>? =
null,
public val entityId: Output? = null,
public val errorCategory: Output? = null,
public val errorCode: Output? = null,
public val errorId: Output? = null,
public val errorLevel: Output? = null,
public val errorMessage: Output? = null,
public val errorSource: Output? = null,
public val errorType: Output? = null,
public val possibleCauses: Output? = null,
public val recommendedAction: Output? = null,
public val recoveryProviderErrorMessage: Output? = null,
public val summaryMessage: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.recoveryservices.inputs.InnerHealthErrorArgs =
com.pulumi.azurenative.recoveryservices.inputs.InnerHealthErrorArgs.builder()
.creationTimeUtc(creationTimeUtc?.applyValue({ args0 -> args0 }))
.customerResolvability(
customerResolvability?.applyValue({ args0 ->
args0.transform({ args0 ->
args0
}, { args0 -> args0.let({ args0 -> args0.toJava() }) })
}),
)
.entityId(entityId?.applyValue({ args0 -> args0 }))
.errorCategory(errorCategory?.applyValue({ args0 -> args0 }))
.errorCode(errorCode?.applyValue({ args0 -> args0 }))
.errorId(errorId?.applyValue({ args0 -> args0 }))
.errorLevel(errorLevel?.applyValue({ args0 -> args0 }))
.errorMessage(errorMessage?.applyValue({ args0 -> args0 }))
.errorSource(errorSource?.applyValue({ args0 -> args0 }))
.errorType(errorType?.applyValue({ args0 -> args0 }))
.possibleCauses(possibleCauses?.applyValue({ args0 -> args0 }))
.recommendedAction(recommendedAction?.applyValue({ args0 -> args0 }))
.recoveryProviderErrorMessage(recoveryProviderErrorMessage?.applyValue({ args0 -> args0 }))
.summaryMessage(summaryMessage?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [InnerHealthErrorArgs].
*/
@PulumiTagMarker
public class InnerHealthErrorArgsBuilder internal constructor() {
private var creationTimeUtc: Output? = null
private var customerResolvability: Output>? =
null
private var entityId: Output? = null
private var errorCategory: Output? = null
private var errorCode: Output? = null
private var errorId: Output? = null
private var errorLevel: Output? = null
private var errorMessage: Output? = null
private var errorSource: Output? = null
private var errorType: Output? = null
private var possibleCauses: Output? = null
private var recommendedAction: Output? = null
private var recoveryProviderErrorMessage: Output? = null
private var summaryMessage: Output? = null
/**
* @param value Error creation time (UTC).
*/
@JvmName("nojcymwkhnsqvesw")
public suspend fun creationTimeUtc(`value`: Output) {
this.creationTimeUtc = value
}
/**
* @param value Value indicating whether the health error is customer resolvable.
*/
@JvmName("dcfbkemtgalpcynj")
public suspend fun customerResolvability(`value`: Output>) {
this.customerResolvability = value
}
/**
* @param value ID of the entity.
*/
@JvmName("xrvbdlsomiapghyg")
public suspend fun entityId(`value`: Output) {
this.entityId = value
}
/**
* @param value Category of error.
*/
@JvmName("hkhndjufmolvqkde")
public suspend fun errorCategory(`value`: Output) {
this.errorCategory = value
}
/**
* @param value Error code.
*/
@JvmName("nvtkxlmgbcsgbmbs")
public suspend fun errorCode(`value`: Output) {
this.errorCode = value
}
/**
* @param value The health error unique id.
*/
@JvmName("lwgjjfdiwmialhsn")
public suspend fun errorId(`value`: Output) {
this.errorId = value
}
/**
* @param value Level of error.
*/
@JvmName("climtmvlcuaxvogd")
public suspend fun errorLevel(`value`: Output) {
this.errorLevel = value
}
/**
* @param value Error message.
*/
@JvmName("adclenitubwjgwog")
public suspend fun errorMessage(`value`: Output) {
this.errorMessage = value
}
/**
* @param value Source of error.
*/
@JvmName("rhxcnevrnyxivccy")
public suspend fun errorSource(`value`: Output) {
this.errorSource = value
}
/**
* @param value Type of error.
*/
@JvmName("rwhfwhglenswqddp")
public suspend fun errorType(`value`: Output) {
this.errorType = value
}
/**
* @param value Possible causes of error.
*/
@JvmName("icdknhgncyknrhos")
public suspend fun possibleCauses(`value`: Output) {
this.possibleCauses = value
}
/**
* @param value Recommended action to resolve error.
*/
@JvmName("gfnnbnrbgaqnfvmi")
public suspend fun recommendedAction(`value`: Output) {
this.recommendedAction = value
}
/**
* @param value DRA error message.
*/
@JvmName("pkdvidsggecnjbmy")
public suspend fun recoveryProviderErrorMessage(`value`: Output) {
this.recoveryProviderErrorMessage = value
}
/**
* @param value Summary message of the entity.
*/
@JvmName("uqmxgtmtjawiksvy")
public suspend fun summaryMessage(`value`: Output) {
this.summaryMessage = value
}
/**
* @param value Error creation time (UTC).
*/
@JvmName("ttvwfhjrahvindnv")
public suspend fun creationTimeUtc(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.creationTimeUtc = mapped
}
/**
* @param value Value indicating whether the health error is customer resolvable.
*/
@JvmName("dpfmtwbvasjarckq")
public suspend fun customerResolvability(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.customerResolvability = mapped
}
/**
* @param value Value indicating whether the health error is customer resolvable.
*/
@JvmName("askanhmnuyyncexs")
public fun customerResolvability(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.customerResolvability = mapped
}
/**
* @param value Value indicating whether the health error is customer resolvable.
*/
@JvmName("hrmrawpitctejmsg")
public fun customerResolvability(`value`: HealthErrorCustomerResolvability) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.customerResolvability = mapped
}
/**
* @param value ID of the entity.
*/
@JvmName("ylrwsfnsxaylvvpw")
public suspend fun entityId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.entityId = mapped
}
/**
* @param value Category of error.
*/
@JvmName("tkicetgfxiipvuuq")
public suspend fun errorCategory(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.errorCategory = mapped
}
/**
* @param value Error code.
*/
@JvmName("xovusqwfhhwaxtak")
public suspend fun errorCode(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.errorCode = mapped
}
/**
* @param value The health error unique id.
*/
@JvmName("tqavvgrdrswknapw")
public suspend fun errorId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.errorId = mapped
}
/**
* @param value Level of error.
*/
@JvmName("ckghlvvfgtiakidc")
public suspend fun errorLevel(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.errorLevel = mapped
}
/**
* @param value Error message.
*/
@JvmName("jufyvdakhomiasnu")
public suspend fun errorMessage(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.errorMessage = mapped
}
/**
* @param value Source of error.
*/
@JvmName("alsekoxqhasyaocw")
public suspend fun errorSource(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.errorSource = mapped
}
/**
* @param value Type of error.
*/
@JvmName("sctrjfitxtvouqph")
public suspend fun errorType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.errorType = mapped
}
/**
* @param value Possible causes of error.
*/
@JvmName("jducmkosqsliwgui")
public suspend fun possibleCauses(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.possibleCauses = mapped
}
/**
* @param value Recommended action to resolve error.
*/
@JvmName("klwtfkeqqyouvvok")
public suspend fun recommendedAction(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.recommendedAction = mapped
}
/**
* @param value DRA error message.
*/
@JvmName("bqaigsqsrklffptg")
public suspend fun recoveryProviderErrorMessage(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.recoveryProviderErrorMessage = mapped
}
/**
* @param value Summary message of the entity.
*/
@JvmName("rxpltrqbkfabxtyi")
public suspend fun summaryMessage(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.summaryMessage = mapped
}
internal fun build(): InnerHealthErrorArgs = InnerHealthErrorArgs(
creationTimeUtc = creationTimeUtc,
customerResolvability = customerResolvability,
entityId = entityId,
errorCategory = errorCategory,
errorCode = errorCode,
errorId = errorId,
errorLevel = errorLevel,
errorMessage = errorMessage,
errorSource = errorSource,
errorType = errorType,
possibleCauses = possibleCauses,
recommendedAction = recommendedAction,
recoveryProviderErrorMessage = recoveryProviderErrorMessage,
summaryMessage = summaryMessage,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy