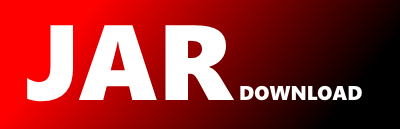
com.pulumi.azurenative.recoveryservices.kotlin.inputs.SharedDiskReplicationItemPropertiesArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.recoveryservices.kotlin.inputs
import com.pulumi.azurenative.recoveryservices.inputs.SharedDiskReplicationItemPropertiesArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Shared Disk Replication item custom data details.
* @property activeLocation The Current active location of the PE.
* @property allowedOperations The allowed operations on the Replication protected item.
* @property currentScenario The current scenario.
* @property healthErrors List of health errors.
* @property protectionState The protection state of shared disk.
* @property replicationHealth The consolidated protection health for the VM taking any issues with SRS as well as all the replication units associated with the VM's replication group into account. This is a string representation of the ProtectionHealth enumeration.
* @property sharedDiskProviderSpecificDetails The Replication provider custom settings.
* @property testFailoverState The tfo state of shared disk.
*/
public data class SharedDiskReplicationItemPropertiesArgs(
public val activeLocation: Output? = null,
public val allowedOperations: Output>? = null,
public val currentScenario: Output? = null,
public val healthErrors: Output>? = null,
public val protectionState: Output? = null,
public val replicationHealth: Output? = null,
public val sharedDiskProviderSpecificDetails: Output? = null,
public val testFailoverState: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.recoveryservices.inputs.SharedDiskReplicationItemPropertiesArgs =
com.pulumi.azurenative.recoveryservices.inputs.SharedDiskReplicationItemPropertiesArgs.builder()
.activeLocation(activeLocation?.applyValue({ args0 -> args0 }))
.allowedOperations(allowedOperations?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.currentScenario(currentScenario?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.healthErrors(
healthErrors?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.protectionState(protectionState?.applyValue({ args0 -> args0 }))
.replicationHealth(replicationHealth?.applyValue({ args0 -> args0 }))
.sharedDiskProviderSpecificDetails(
sharedDiskProviderSpecificDetails?.applyValue({ args0 ->
args0.let({ args0 -> args0.toJava() })
}),
)
.testFailoverState(testFailoverState?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [SharedDiskReplicationItemPropertiesArgs].
*/
@PulumiTagMarker
public class SharedDiskReplicationItemPropertiesArgsBuilder internal constructor() {
private var activeLocation: Output? = null
private var allowedOperations: Output>? = null
private var currentScenario: Output? = null
private var healthErrors: Output>? = null
private var protectionState: Output? = null
private var replicationHealth: Output? = null
private var sharedDiskProviderSpecificDetails: Output? = null
private var testFailoverState: Output? = null
/**
* @param value The Current active location of the PE.
*/
@JvmName("rgunbcpoelyngcpt")
public suspend fun activeLocation(`value`: Output) {
this.activeLocation = value
}
/**
* @param value The allowed operations on the Replication protected item.
*/
@JvmName("rkymswddxjqxkwis")
public suspend fun allowedOperations(`value`: Output>) {
this.allowedOperations = value
}
@JvmName("ltihueivipqkaicy")
public suspend fun allowedOperations(vararg values: Output) {
this.allowedOperations = Output.all(values.asList())
}
/**
* @param values The allowed operations on the Replication protected item.
*/
@JvmName("eeesucbwniwexatx")
public suspend fun allowedOperations(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy