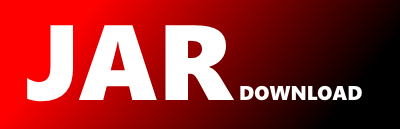
com.pulumi.azurenative.recoveryservices.kotlin.inputs.SkuArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.recoveryservices.kotlin.inputs
import com.pulumi.azurenative.recoveryservices.inputs.SkuArgs.builder
import com.pulumi.azurenative.recoveryservices.kotlin.enums.SkuName
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Identifies the unique system identifier for each Azure resource.
* @property capacity The sku capacity
* @property family The sku family
* @property name Name of SKU is RS0 (Recovery Services 0th version) and the tier is standard tier. They do not have affect on backend storage redundancy or any other vault settings. To manage storage redundancy, use the backupstorageconfig
* @property size The sku size
* @property tier The Sku tier.
*/
public data class SkuArgs(
public val capacity: Output? = null,
public val family: Output? = null,
public val name: Output>,
public val size: Output? = null,
public val tier: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.recoveryservices.inputs.SkuArgs =
com.pulumi.azurenative.recoveryservices.inputs.SkuArgs.builder()
.capacity(capacity?.applyValue({ args0 -> args0 }))
.family(family?.applyValue({ args0 -> args0 }))
.name(
name.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.size(size?.applyValue({ args0 -> args0 }))
.tier(tier?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [SkuArgs].
*/
@PulumiTagMarker
public class SkuArgsBuilder internal constructor() {
private var capacity: Output? = null
private var family: Output? = null
private var name: Output>? = null
private var size: Output? = null
private var tier: Output? = null
/**
* @param value The sku capacity
*/
@JvmName("fxmotkkyscwggsyu")
public suspend fun capacity(`value`: Output) {
this.capacity = value
}
/**
* @param value The sku family
*/
@JvmName("nkrougamjjmiksmc")
public suspend fun family(`value`: Output) {
this.family = value
}
/**
* @param value Name of SKU is RS0 (Recovery Services 0th version) and the tier is standard tier. They do not have affect on backend storage redundancy or any other vault settings. To manage storage redundancy, use the backupstorageconfig
*/
@JvmName("amwnbdyknadowdcd")
public suspend fun name(`value`: Output>) {
this.name = value
}
/**
* @param value The sku size
*/
@JvmName("vgifmmrteynkwdwm")
public suspend fun size(`value`: Output) {
this.size = value
}
/**
* @param value The Sku tier.
*/
@JvmName("neanvbxeathpxiru")
public suspend fun tier(`value`: Output) {
this.tier = value
}
/**
* @param value The sku capacity
*/
@JvmName("qnefmrquricrsroo")
public suspend fun capacity(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.capacity = mapped
}
/**
* @param value The sku family
*/
@JvmName("ogxhgwelppbkwtrn")
public suspend fun family(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.family = mapped
}
/**
* @param value Name of SKU is RS0 (Recovery Services 0th version) and the tier is standard tier. They do not have affect on backend storage redundancy or any other vault settings. To manage storage redundancy, use the backupstorageconfig
*/
@JvmName("kygyuwlfngwxacto")
public suspend fun name(`value`: Either) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value Name of SKU is RS0 (Recovery Services 0th version) and the tier is standard tier. They do not have affect on backend storage redundancy or any other vault settings. To manage storage redundancy, use the backupstorageconfig
*/
@JvmName("cvpangkfkpsqwxay")
public fun name(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value Name of SKU is RS0 (Recovery Services 0th version) and the tier is standard tier. They do not have affect on backend storage redundancy or any other vault settings. To manage storage redundancy, use the backupstorageconfig
*/
@JvmName("pkjjathqgiapmcgy")
public fun name(`value`: SkuName) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value The sku size
*/
@JvmName("jlcnocirfsntgdtj")
public suspend fun size(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.size = mapped
}
/**
* @param value The Sku tier.
*/
@JvmName("qycmqkynymwitmlg")
public suspend fun tier(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.tier = mapped
}
internal fun build(): SkuArgs = SkuArgs(
capacity = capacity,
family = family,
name = name ?: throw PulumiNullFieldException("name"),
size = size,
tier = tier,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy