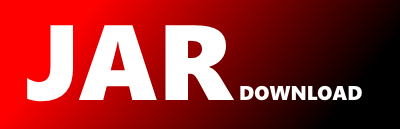
com.pulumi.azurenative.recoveryservices.kotlin.inputs.VaultPropertiesArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.recoveryservices.kotlin.inputs
import com.pulumi.azurenative.recoveryservices.inputs.VaultPropertiesArgs.builder
import com.pulumi.azurenative.recoveryservices.kotlin.enums.PublicNetworkAccess
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Properties of the vault.
* @property encryption Customer Managed Key details of the resource.
* @property monitoringSettings Monitoring Settings of the vault
* @property publicNetworkAccess property to enable or disable resource provider inbound network traffic from public clients
* @property restoreSettings Restore Settings of the vault
* @property securitySettings Security Settings of the vault
*/
public data class VaultPropertiesArgs(
public val encryption: Output? = null,
public val monitoringSettings: Output? = null,
public val publicNetworkAccess: Output>? = null,
public val restoreSettings: Output? = null,
public val securitySettings: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.recoveryservices.inputs.VaultPropertiesArgs =
com.pulumi.azurenative.recoveryservices.inputs.VaultPropertiesArgs.builder()
.encryption(encryption?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.monitoringSettings(
monitoringSettings?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.publicNetworkAccess(
publicNetworkAccess?.applyValue({ args0 ->
args0.transform(
{ args0 -> args0 },
{ args0 -> args0.let({ args0 -> args0.toJava() }) },
)
}),
)
.restoreSettings(restoreSettings?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.securitySettings(
securitySettings?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [VaultPropertiesArgs].
*/
@PulumiTagMarker
public class VaultPropertiesArgsBuilder internal constructor() {
private var encryption: Output? = null
private var monitoringSettings: Output? = null
private var publicNetworkAccess: Output>? = null
private var restoreSettings: Output? = null
private var securitySettings: Output? = null
/**
* @param value Customer Managed Key details of the resource.
*/
@JvmName("ftxmhmtvjpgwqhgq")
public suspend fun encryption(`value`: Output) {
this.encryption = value
}
/**
* @param value Monitoring Settings of the vault
*/
@JvmName("camlvselvuxjiaih")
public suspend fun monitoringSettings(`value`: Output) {
this.monitoringSettings = value
}
/**
* @param value property to enable or disable resource provider inbound network traffic from public clients
*/
@JvmName("foejsrgmcgiqkini")
public suspend fun publicNetworkAccess(`value`: Output>) {
this.publicNetworkAccess = value
}
/**
* @param value Restore Settings of the vault
*/
@JvmName("wnxnwxbsrhrlbsos")
public suspend fun restoreSettings(`value`: Output) {
this.restoreSettings = value
}
/**
* @param value Security Settings of the vault
*/
@JvmName("unhcymyvgvhcarbm")
public suspend fun securitySettings(`value`: Output) {
this.securitySettings = value
}
/**
* @param value Customer Managed Key details of the resource.
*/
@JvmName("vteoytvxpjrqbfvr")
public suspend fun encryption(`value`: VaultPropertiesEncryptionArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.encryption = mapped
}
/**
* @param argument Customer Managed Key details of the resource.
*/
@JvmName("eiigxryvoercljxh")
public suspend fun encryption(argument: suspend VaultPropertiesEncryptionArgsBuilder.() -> Unit) {
val toBeMapped = VaultPropertiesEncryptionArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.encryption = mapped
}
/**
* @param value Monitoring Settings of the vault
*/
@JvmName("pywihxvdijcrhowx")
public suspend fun monitoringSettings(`value`: MonitoringSettingsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.monitoringSettings = mapped
}
/**
* @param argument Monitoring Settings of the vault
*/
@JvmName("ahluodtmgqrlwjri")
public suspend fun monitoringSettings(argument: suspend MonitoringSettingsArgsBuilder.() -> Unit) {
val toBeMapped = MonitoringSettingsArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.monitoringSettings = mapped
}
/**
* @param value property to enable or disable resource provider inbound network traffic from public clients
*/
@JvmName("gtonfebtolnuatlx")
public suspend fun publicNetworkAccess(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.publicNetworkAccess = mapped
}
/**
* @param value property to enable or disable resource provider inbound network traffic from public clients
*/
@JvmName("appwgmtbfrlvxbqf")
public fun publicNetworkAccess(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.publicNetworkAccess = mapped
}
/**
* @param value property to enable or disable resource provider inbound network traffic from public clients
*/
@JvmName("gsmjrutdpdtnohle")
public fun publicNetworkAccess(`value`: PublicNetworkAccess) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.publicNetworkAccess = mapped
}
/**
* @param value Restore Settings of the vault
*/
@JvmName("pbjsivjulkbesggt")
public suspend fun restoreSettings(`value`: RestoreSettingsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.restoreSettings = mapped
}
/**
* @param argument Restore Settings of the vault
*/
@JvmName("rkmofvxyygaquvwi")
public suspend fun restoreSettings(argument: suspend RestoreSettingsArgsBuilder.() -> Unit) {
val toBeMapped = RestoreSettingsArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.restoreSettings = mapped
}
/**
* @param value Security Settings of the vault
*/
@JvmName("lfjcnkgablmtyxxv")
public suspend fun securitySettings(`value`: SecuritySettingsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.securitySettings = mapped
}
/**
* @param argument Security Settings of the vault
*/
@JvmName("sisbqsvhwfpfxmrq")
public suspend fun securitySettings(argument: suspend SecuritySettingsArgsBuilder.() -> Unit) {
val toBeMapped = SecuritySettingsArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.securitySettings = mapped
}
internal fun build(): VaultPropertiesArgs = VaultPropertiesArgs(
encryption = encryption,
monitoringSettings = monitoringSettings,
publicNetworkAccess = publicNetworkAccess,
restoreSettings = restoreSettings,
securitySettings = securitySettings,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy