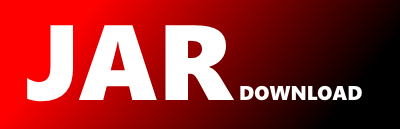
com.pulumi.azurenative.redhatopenshift.kotlin.OpenShiftCluster.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.redhatopenshift.kotlin
import com.pulumi.azurenative.redhatopenshift.kotlin.outputs.APIServerProfileResponse
import com.pulumi.azurenative.redhatopenshift.kotlin.outputs.ClusterProfileResponse
import com.pulumi.azurenative.redhatopenshift.kotlin.outputs.ConsoleProfileResponse
import com.pulumi.azurenative.redhatopenshift.kotlin.outputs.IngressProfileResponse
import com.pulumi.azurenative.redhatopenshift.kotlin.outputs.MasterProfileResponse
import com.pulumi.azurenative.redhatopenshift.kotlin.outputs.NetworkProfileResponse
import com.pulumi.azurenative.redhatopenshift.kotlin.outputs.ServicePrincipalProfileResponse
import com.pulumi.azurenative.redhatopenshift.kotlin.outputs.SystemDataResponse
import com.pulumi.azurenative.redhatopenshift.kotlin.outputs.WorkerProfileResponse
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import com.pulumi.azurenative.redhatopenshift.kotlin.outputs.APIServerProfileResponse.Companion.toKotlin as aPIServerProfileResponseToKotlin
import com.pulumi.azurenative.redhatopenshift.kotlin.outputs.ClusterProfileResponse.Companion.toKotlin as clusterProfileResponseToKotlin
import com.pulumi.azurenative.redhatopenshift.kotlin.outputs.ConsoleProfileResponse.Companion.toKotlin as consoleProfileResponseToKotlin
import com.pulumi.azurenative.redhatopenshift.kotlin.outputs.IngressProfileResponse.Companion.toKotlin as ingressProfileResponseToKotlin
import com.pulumi.azurenative.redhatopenshift.kotlin.outputs.MasterProfileResponse.Companion.toKotlin as masterProfileResponseToKotlin
import com.pulumi.azurenative.redhatopenshift.kotlin.outputs.NetworkProfileResponse.Companion.toKotlin as networkProfileResponseToKotlin
import com.pulumi.azurenative.redhatopenshift.kotlin.outputs.ServicePrincipalProfileResponse.Companion.toKotlin as servicePrincipalProfileResponseToKotlin
import com.pulumi.azurenative.redhatopenshift.kotlin.outputs.SystemDataResponse.Companion.toKotlin as systemDataResponseToKotlin
import com.pulumi.azurenative.redhatopenshift.kotlin.outputs.WorkerProfileResponse.Companion.toKotlin as workerProfileResponseToKotlin
/**
* Builder for [OpenShiftCluster].
*/
@PulumiTagMarker
public class OpenShiftClusterResourceBuilder internal constructor() {
public var name: String? = null
public var args: OpenShiftClusterArgs = OpenShiftClusterArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend OpenShiftClusterArgsBuilder.() -> Unit) {
val builder = OpenShiftClusterArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): OpenShiftCluster {
val builtJavaResource =
com.pulumi.azurenative.redhatopenshift.OpenShiftCluster(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return OpenShiftCluster(builtJavaResource)
}
}
/**
* OpenShiftCluster represents an Azure Red Hat OpenShift cluster.
* Azure REST API version: 2022-09-04. Prior API version in Azure Native 1.x: 2020-04-30.
* Other available API versions: 2023-04-01, 2023-07-01-preview, 2023-09-04, 2023-11-22.
* ## Example Usage
* ### Creates or updates a OpenShift cluster with the specified subscription, resource group and resource name.
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var openShiftCluster = new AzureNative.RedHatOpenShift.OpenShiftCluster("openShiftCluster", new()
* {
* ApiserverProfile = new AzureNative.RedHatOpenShift.Inputs.APIServerProfileArgs
* {
* Visibility = AzureNative.RedHatOpenShift.Visibility.Public,
* },
* ClusterProfile = new AzureNative.RedHatOpenShift.Inputs.ClusterProfileArgs
* {
* Domain = "cluster.location.aroapp.io",
* FipsValidatedModules = AzureNative.RedHatOpenShift.FipsValidatedModules.Enabled,
* PullSecret = "{\"auths\":{\"registry.connect.redhat.com\":{\"auth\":\"\"},\"registry.redhat.io\":{\"auth\":\"\"}}}",
* ResourceGroupId = "/subscriptions/subscriptionId/resourceGroups/clusterResourceGroup",
* },
* ConsoleProfile = null,
* IngressProfiles = new[]
* {
* new AzureNative.RedHatOpenShift.Inputs.IngressProfileArgs
* {
* Name = "default",
* Visibility = AzureNative.RedHatOpenShift.Visibility.Public,
* },
* },
* Location = "location",
* MasterProfile = new AzureNative.RedHatOpenShift.Inputs.MasterProfileArgs
* {
* EncryptionAtHost = AzureNative.RedHatOpenShift.EncryptionAtHost.Enabled,
* SubnetId = "/subscriptions/subscriptionId/resourceGroups/vnetResourceGroup/providers/Microsoft.Network/virtualNetworks/vnet/subnets/master",
* VmSize = "Standard_D8s_v3",
* },
* NetworkProfile = new AzureNative.RedHatOpenShift.Inputs.NetworkProfileArgs
* {
* PodCidr = "10.128.0.0/14",
* ServiceCidr = "172.30.0.0/16",
* },
* ResourceGroupName = "resourceGroup",
* ResourceName = "resourceName",
* ServicePrincipalProfile = new AzureNative.RedHatOpenShift.Inputs.ServicePrincipalProfileArgs
* {
* ClientId = "clientId",
* ClientSecret = "clientSecret",
* },
* Tags =
* {
* { "key", "value" },
* },
* WorkerProfiles = new[]
* {
* new AzureNative.RedHatOpenShift.Inputs.WorkerProfileArgs
* {
* Count = 3,
* DiskSizeGB = 128,
* Name = "worker",
* SubnetId = "/subscriptions/subscriptionId/resourceGroups/vnetResourceGroup/providers/Microsoft.Network/virtualNetworks/vnet/subnets/worker",
* VmSize = "Standard_D2s_v3",
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* redhatopenshift "github.com/pulumi/pulumi-azure-native-sdk/redhatopenshift/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := redhatopenshift.NewOpenShiftCluster(ctx, "openShiftCluster", &redhatopenshift.OpenShiftClusterArgs{
* ApiserverProfile: &redhatopenshift.APIServerProfileArgs{
* Visibility: pulumi.String(redhatopenshift.VisibilityPublic),
* },
* ClusterProfile: &redhatopenshift.ClusterProfileArgs{
* Domain: pulumi.String("cluster.location.aroapp.io"),
* FipsValidatedModules: pulumi.String(redhatopenshift.FipsValidatedModulesEnabled),
* PullSecret: pulumi.String("{\"auths\":{\"registry.connect.redhat.com\":{\"auth\":\"\"},\"registry.redhat.io\":{\"auth\":\"\"}}}"),
* ResourceGroupId: pulumi.String("/subscriptions/subscriptionId/resourceGroups/clusterResourceGroup"),
* },
* ConsoleProfile: nil,
* IngressProfiles: redhatopenshift.IngressProfileArray{
* &redhatopenshift.IngressProfileArgs{
* Name: pulumi.String("default"),
* Visibility: pulumi.String(redhatopenshift.VisibilityPublic),
* },
* },
* Location: pulumi.String("location"),
* MasterProfile: &redhatopenshift.MasterProfileArgs{
* EncryptionAtHost: pulumi.String(redhatopenshift.EncryptionAtHostEnabled),
* SubnetId: pulumi.String("/subscriptions/subscriptionId/resourceGroups/vnetResourceGroup/providers/Microsoft.Network/virtualNetworks/vnet/subnets/master"),
* VmSize: pulumi.String("Standard_D8s_v3"),
* },
* NetworkProfile: &redhatopenshift.NetworkProfileArgs{
* PodCidr: pulumi.String("10.128.0.0/14"),
* ServiceCidr: pulumi.String("172.30.0.0/16"),
* },
* ResourceGroupName: pulumi.String("resourceGroup"),
* ResourceName: pulumi.String("resourceName"),
* ServicePrincipalProfile: &redhatopenshift.ServicePrincipalProfileArgs{
* ClientId: pulumi.String("clientId"),
* ClientSecret: pulumi.String("clientSecret"),
* },
* Tags: pulumi.StringMap{
* "key": pulumi.String("value"),
* },
* WorkerProfiles: redhatopenshift.WorkerProfileArray{
* &redhatopenshift.WorkerProfileArgs{
* Count: pulumi.Int(3),
* DiskSizeGB: pulumi.Int(128),
* Name: pulumi.String("worker"),
* SubnetId: pulumi.String("/subscriptions/subscriptionId/resourceGroups/vnetResourceGroup/providers/Microsoft.Network/virtualNetworks/vnet/subnets/worker"),
* VmSize: pulumi.String("Standard_D2s_v3"),
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.redhatopenshift.OpenShiftCluster;
* import com.pulumi.azurenative.redhatopenshift.OpenShiftClusterArgs;
* import com.pulumi.azurenative.redhatopenshift.inputs.APIServerProfileArgs;
* import com.pulumi.azurenative.redhatopenshift.inputs.ClusterProfileArgs;
* import com.pulumi.azurenative.redhatopenshift.inputs.ConsoleProfileArgs;
* import com.pulumi.azurenative.redhatopenshift.inputs.IngressProfileArgs;
* import com.pulumi.azurenative.redhatopenshift.inputs.MasterProfileArgs;
* import com.pulumi.azurenative.redhatopenshift.inputs.NetworkProfileArgs;
* import com.pulumi.azurenative.redhatopenshift.inputs.ServicePrincipalProfileArgs;
* import com.pulumi.azurenative.redhatopenshift.inputs.WorkerProfileArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var openShiftCluster = new OpenShiftCluster("openShiftCluster", OpenShiftClusterArgs.builder()
* .apiserverProfile(APIServerProfileArgs.builder()
* .visibility("Public")
* .build())
* .clusterProfile(ClusterProfileArgs.builder()
* .domain("cluster.location.aroapp.io")
* .fipsValidatedModules("Enabled")
* .pullSecret("{\"auths\":{\"registry.connect.redhat.com\":{\"auth\":\"\"},\"registry.redhat.io\":{\"auth\":\"\"}}}")
* .resourceGroupId("/subscriptions/subscriptionId/resourceGroups/clusterResourceGroup")
* .build())
* .consoleProfile()
* .ingressProfiles(IngressProfileArgs.builder()
* .name("default")
* .visibility("Public")
* .build())
* .location("location")
* .masterProfile(MasterProfileArgs.builder()
* .encryptionAtHost("Enabled")
* .subnetId("/subscriptions/subscriptionId/resourceGroups/vnetResourceGroup/providers/Microsoft.Network/virtualNetworks/vnet/subnets/master")
* .vmSize("Standard_D8s_v3")
* .build())
* .networkProfile(NetworkProfileArgs.builder()
* .podCidr("10.128.0.0/14")
* .serviceCidr("172.30.0.0/16")
* .build())
* .resourceGroupName("resourceGroup")
* .resourceName("resourceName")
* .servicePrincipalProfile(ServicePrincipalProfileArgs.builder()
* .clientId("clientId")
* .clientSecret("clientSecret")
* .build())
* .tags(Map.of("key", "value"))
* .workerProfiles(WorkerProfileArgs.builder()
* .count(3)
* .diskSizeGB(128)
* .name("worker")
* .subnetId("/subscriptions/subscriptionId/resourceGroups/vnetResourceGroup/providers/Microsoft.Network/virtualNetworks/vnet/subnets/worker")
* .vmSize("Standard_D2s_v3")
* .build())
* .build());
* }
* }
* ```
* ## Import
* An existing resource can be imported using its type token, name, and identifier, e.g.
* ```sh
* $ pulumi import azure-native:redhatopenshift:OpenShiftCluster resourceName /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.RedHatOpenShift/openShiftClusters/{resourceName}
* ```
*/
public class OpenShiftCluster internal constructor(
override val javaResource: com.pulumi.azurenative.redhatopenshift.OpenShiftCluster,
) : KotlinCustomResource(javaResource, OpenShiftClusterMapper) {
/**
* The cluster API server profile.
*/
public val apiserverProfile: Output?
get() = javaResource.apiserverProfile().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> aPIServerProfileResponseToKotlin(args0) })
}).orElse(null)
})
/**
* The cluster profile.
*/
public val clusterProfile: Output?
get() = javaResource.clusterProfile().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> clusterProfileResponseToKotlin(args0) })
}).orElse(null)
})
/**
* The console profile.
*/
public val consoleProfile: Output?
get() = javaResource.consoleProfile().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> consoleProfileResponseToKotlin(args0) })
}).orElse(null)
})
/**
* The cluster ingress profiles.
*/
public val ingressProfiles: Output>?
get() = javaResource.ingressProfiles().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
ingressProfileResponseToKotlin(args0)
})
})
}).orElse(null)
})
/**
* The geo-location where the resource lives
*/
public val location: Output
get() = javaResource.location().applyValue({ args0 -> args0 })
/**
* The cluster master profile.
*/
public val masterProfile: Output?
get() = javaResource.masterProfile().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> masterProfileResponseToKotlin(args0) })
}).orElse(null)
})
/**
* The name of the resource
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* The cluster network profile.
*/
public val networkProfile: Output?
get() = javaResource.networkProfile().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> networkProfileResponseToKotlin(args0) })
}).orElse(null)
})
/**
* The cluster provisioning state.
*/
public val provisioningState: Output?
get() = javaResource.provisioningState().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The cluster service principal profile.
*/
public val servicePrincipalProfile: Output?
get() = javaResource.servicePrincipalProfile().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> servicePrincipalProfileResponseToKotlin(args0) })
}).orElse(null)
})
/**
* Azure Resource Manager metadata containing createdBy and modifiedBy information.
*/
public val systemData: Output
get() = javaResource.systemData().applyValue({ args0 ->
args0.let({ args0 ->
systemDataResponseToKotlin(args0)
})
})
/**
* Resource tags.
*/
public val tags: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy