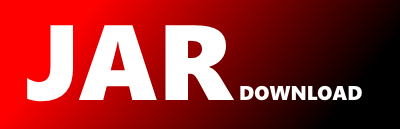
com.pulumi.azurenative.relay.kotlin.PrivateEndpointConnectionArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.relay.kotlin
import com.pulumi.azurenative.relay.PrivateEndpointConnectionArgs.builder
import com.pulumi.azurenative.relay.kotlin.enums.EndPointProvisioningState
import com.pulumi.azurenative.relay.kotlin.inputs.ConnectionStateArgs
import com.pulumi.azurenative.relay.kotlin.inputs.ConnectionStateArgsBuilder
import com.pulumi.azurenative.relay.kotlin.inputs.PrivateEndpointArgs
import com.pulumi.azurenative.relay.kotlin.inputs.PrivateEndpointArgsBuilder
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Properties of the PrivateEndpointConnection.
* Azure REST API version: 2021-11-01. Prior API version in Azure Native 1.x: 2018-01-01-preview.
* Other available API versions: 2018-01-01-preview.
* ## Example Usage
* ### NameSpacePrivateEndPointConnectionCreate
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var privateEndpointConnection = new AzureNative.Relay.PrivateEndpointConnection("privateEndpointConnection", new()
* {
* NamespaceName = "example-RelayNamespace-5849",
* PrivateEndpoint = new AzureNative.Relay.Inputs.PrivateEndpointArgs
* {
* Id = "/subscriptions/ffffffff-ffff-ffff-ffff-ffffffffffff/resourceGroups/resourcegroup/providers/Microsoft.Network/privateEndpoints/ali-relay-pve-1",
* },
* PrivateEndpointConnectionName = "{privateEndpointConnection name}",
* PrivateLinkServiceConnectionState = new AzureNative.Relay.Inputs.ConnectionStateArgs
* {
* Description = "You may pass",
* Status = AzureNative.Relay.PrivateLinkConnectionStatus.Approved,
* },
* ResourceGroupName = "resourcegroup",
* });
* });
* ```
* ```go
* package main
* import (
* relay "github.com/pulumi/pulumi-azure-native-sdk/relay/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := relay.NewPrivateEndpointConnection(ctx, "privateEndpointConnection", &relay.PrivateEndpointConnectionArgs{
* NamespaceName: pulumi.String("example-RelayNamespace-5849"),
* PrivateEndpoint: &relay.PrivateEndpointArgs{
* Id: pulumi.String("/subscriptions/ffffffff-ffff-ffff-ffff-ffffffffffff/resourceGroups/resourcegroup/providers/Microsoft.Network/privateEndpoints/ali-relay-pve-1"),
* },
* PrivateEndpointConnectionName: pulumi.String("{privateEndpointConnection name}"),
* PrivateLinkServiceConnectionState: &relay.ConnectionStateArgs{
* Description: pulumi.String("You may pass"),
* Status: pulumi.String(relay.PrivateLinkConnectionStatusApproved),
* },
* ResourceGroupName: pulumi.String("resourcegroup"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.relay.PrivateEndpointConnection;
* import com.pulumi.azurenative.relay.PrivateEndpointConnectionArgs;
* import com.pulumi.azurenative.relay.inputs.PrivateEndpointArgs;
* import com.pulumi.azurenative.relay.inputs.ConnectionStateArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var privateEndpointConnection = new PrivateEndpointConnection("privateEndpointConnection", PrivateEndpointConnectionArgs.builder()
* .namespaceName("example-RelayNamespace-5849")
* .privateEndpoint(PrivateEndpointArgs.builder()
* .id("/subscriptions/ffffffff-ffff-ffff-ffff-ffffffffffff/resourceGroups/resourcegroup/providers/Microsoft.Network/privateEndpoints/ali-relay-pve-1")
* .build())
* .privateEndpointConnectionName("{privateEndpointConnection name}")
* .privateLinkServiceConnectionState(ConnectionStateArgs.builder()
* .description("You may pass")
* .status("Approved")
* .build())
* .resourceGroupName("resourcegroup")
* .build());
* }
* }
* ```
* ## Import
* An existing resource can be imported using its type token, name, and identifier, e.g.
* ```sh
* $ pulumi import azure-native:relay:PrivateEndpointConnection {privateEndpointConnection name} /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Relay/namespaces/{namespaceName}/privateEndpointConnections/{privateEndpointConnectionName}
* ```
* @property namespaceName The namespace name
* @property privateEndpoint The Private Endpoint resource for this Connection.
* @property privateEndpointConnectionName The PrivateEndpointConnection name
* @property privateLinkServiceConnectionState Details about the state of the connection.
* @property provisioningState Provisioning state of the Private Endpoint Connection.
* @property resourceGroupName Name of the Resource group within the Azure subscription.
*/
public data class PrivateEndpointConnectionArgs(
public val namespaceName: Output? = null,
public val privateEndpoint: Output? = null,
public val privateEndpointConnectionName: Output? = null,
public val privateLinkServiceConnectionState: Output? = null,
public val provisioningState: Output>? = null,
public val resourceGroupName: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.relay.PrivateEndpointConnectionArgs =
com.pulumi.azurenative.relay.PrivateEndpointConnectionArgs.builder()
.namespaceName(namespaceName?.applyValue({ args0 -> args0 }))
.privateEndpoint(privateEndpoint?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.privateEndpointConnectionName(privateEndpointConnectionName?.applyValue({ args0 -> args0 }))
.privateLinkServiceConnectionState(
privateLinkServiceConnectionState?.applyValue({ args0 ->
args0.let({ args0 -> args0.toJava() })
}),
)
.provisioningState(
provisioningState?.applyValue({ args0 ->
args0.transform(
{ args0 -> args0 },
{ args0 -> args0.let({ args0 -> args0.toJava() }) },
)
}),
)
.resourceGroupName(resourceGroupName?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [PrivateEndpointConnectionArgs].
*/
@PulumiTagMarker
public class PrivateEndpointConnectionArgsBuilder internal constructor() {
private var namespaceName: Output? = null
private var privateEndpoint: Output? = null
private var privateEndpointConnectionName: Output? = null
private var privateLinkServiceConnectionState: Output? = null
private var provisioningState: Output>? = null
private var resourceGroupName: Output? = null
/**
* @param value The namespace name
*/
@JvmName("wresgucawjfyxplv")
public suspend fun namespaceName(`value`: Output) {
this.namespaceName = value
}
/**
* @param value The Private Endpoint resource for this Connection.
*/
@JvmName("jyhsnibuiakotgar")
public suspend fun privateEndpoint(`value`: Output) {
this.privateEndpoint = value
}
/**
* @param value The PrivateEndpointConnection name
*/
@JvmName("gbopxmlgwhssylnu")
public suspend fun privateEndpointConnectionName(`value`: Output) {
this.privateEndpointConnectionName = value
}
/**
* @param value Details about the state of the connection.
*/
@JvmName("xpowrldghikjjvxg")
public suspend fun privateLinkServiceConnectionState(`value`: Output) {
this.privateLinkServiceConnectionState = value
}
/**
* @param value Provisioning state of the Private Endpoint Connection.
*/
@JvmName("aamikavyeeinqnmr")
public suspend fun provisioningState(`value`: Output>) {
this.provisioningState = value
}
/**
* @param value Name of the Resource group within the Azure subscription.
*/
@JvmName("pgdewfpavpirwfos")
public suspend fun resourceGroupName(`value`: Output) {
this.resourceGroupName = value
}
/**
* @param value The namespace name
*/
@JvmName("qedlnsmytqmtrjji")
public suspend fun namespaceName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.namespaceName = mapped
}
/**
* @param value The Private Endpoint resource for this Connection.
*/
@JvmName("yikkirsfvyujfetc")
public suspend fun privateEndpoint(`value`: PrivateEndpointArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.privateEndpoint = mapped
}
/**
* @param argument The Private Endpoint resource for this Connection.
*/
@JvmName("gfputagljibfissl")
public suspend fun privateEndpoint(argument: suspend PrivateEndpointArgsBuilder.() -> Unit) {
val toBeMapped = PrivateEndpointArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.privateEndpoint = mapped
}
/**
* @param value The PrivateEndpointConnection name
*/
@JvmName("qlxywqabigeokbqe")
public suspend fun privateEndpointConnectionName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.privateEndpointConnectionName = mapped
}
/**
* @param value Details about the state of the connection.
*/
@JvmName("rtadcvnvjwtbwbdx")
public suspend fun privateLinkServiceConnectionState(`value`: ConnectionStateArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.privateLinkServiceConnectionState = mapped
}
/**
* @param argument Details about the state of the connection.
*/
@JvmName("pynghnkxoqwcpavc")
public suspend fun privateLinkServiceConnectionState(argument: suspend ConnectionStateArgsBuilder.() -> Unit) {
val toBeMapped = ConnectionStateArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.privateLinkServiceConnectionState = mapped
}
/**
* @param value Provisioning state of the Private Endpoint Connection.
*/
@JvmName("xtydjbughrtvqqpv")
public suspend fun provisioningState(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.provisioningState = mapped
}
/**
* @param value Provisioning state of the Private Endpoint Connection.
*/
@JvmName("tfuxkhounsmbffpo")
public fun provisioningState(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.provisioningState = mapped
}
/**
* @param value Provisioning state of the Private Endpoint Connection.
*/
@JvmName("usxlxsrvcjyiumdd")
public fun provisioningState(`value`: EndPointProvisioningState) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.provisioningState = mapped
}
/**
* @param value Name of the Resource group within the Azure subscription.
*/
@JvmName("ajuxmhqvjgklbgbk")
public suspend fun resourceGroupName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.resourceGroupName = mapped
}
internal fun build(): PrivateEndpointConnectionArgs = PrivateEndpointConnectionArgs(
namespaceName = namespaceName,
privateEndpoint = privateEndpoint,
privateEndpointConnectionName = privateEndpointConnectionName,
privateLinkServiceConnectionState = privateLinkServiceConnectionState,
provisioningState = provisioningState,
resourceGroupName = resourceGroupName,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy