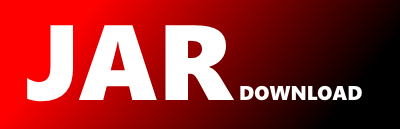
com.pulumi.azurenative.resources.kotlin.DeploymentStackAtSubscription.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.resources.kotlin
import com.pulumi.azurenative.resources.kotlin.outputs.DenySettingsResponse
import com.pulumi.azurenative.resources.kotlin.outputs.DeploymentStackPropertiesResponseActionOnUnmanage
import com.pulumi.azurenative.resources.kotlin.outputs.DeploymentStacksDebugSettingResponse
import com.pulumi.azurenative.resources.kotlin.outputs.DeploymentStacksParametersLinkResponse
import com.pulumi.azurenative.resources.kotlin.outputs.ErrorResponseResponse
import com.pulumi.azurenative.resources.kotlin.outputs.ManagedResourceReferenceResponse
import com.pulumi.azurenative.resources.kotlin.outputs.ResourceReferenceExtendedResponse
import com.pulumi.azurenative.resources.kotlin.outputs.ResourceReferenceResponse
import com.pulumi.azurenative.resources.kotlin.outputs.SystemDataResponse
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Any
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import com.pulumi.azurenative.resources.kotlin.outputs.DenySettingsResponse.Companion.toKotlin as denySettingsResponseToKotlin
import com.pulumi.azurenative.resources.kotlin.outputs.DeploymentStackPropertiesResponseActionOnUnmanage.Companion.toKotlin as deploymentStackPropertiesResponseActionOnUnmanageToKotlin
import com.pulumi.azurenative.resources.kotlin.outputs.DeploymentStacksDebugSettingResponse.Companion.toKotlin as deploymentStacksDebugSettingResponseToKotlin
import com.pulumi.azurenative.resources.kotlin.outputs.DeploymentStacksParametersLinkResponse.Companion.toKotlin as deploymentStacksParametersLinkResponseToKotlin
import com.pulumi.azurenative.resources.kotlin.outputs.ErrorResponseResponse.Companion.toKotlin as errorResponseResponseToKotlin
import com.pulumi.azurenative.resources.kotlin.outputs.ManagedResourceReferenceResponse.Companion.toKotlin as managedResourceReferenceResponseToKotlin
import com.pulumi.azurenative.resources.kotlin.outputs.ResourceReferenceExtendedResponse.Companion.toKotlin as resourceReferenceExtendedResponseToKotlin
import com.pulumi.azurenative.resources.kotlin.outputs.ResourceReferenceResponse.Companion.toKotlin as resourceReferenceResponseToKotlin
import com.pulumi.azurenative.resources.kotlin.outputs.SystemDataResponse.Companion.toKotlin as systemDataResponseToKotlin
/**
* Builder for [DeploymentStackAtSubscription].
*/
@PulumiTagMarker
public class DeploymentStackAtSubscriptionResourceBuilder internal constructor() {
public var name: String? = null
public var args: DeploymentStackAtSubscriptionArgs = DeploymentStackAtSubscriptionArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend DeploymentStackAtSubscriptionArgsBuilder.() -> Unit) {
val builder = DeploymentStackAtSubscriptionArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): DeploymentStackAtSubscription {
val builtJavaResource =
com.pulumi.azurenative.resources.DeploymentStackAtSubscription(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return DeploymentStackAtSubscription(builtJavaResource)
}
}
/**
* Deployment stack object.
* Azure REST API version: 2022-08-01-preview.
* Other available API versions: 2024-03-01.
* ## Example Usage
* ### DeploymentStacksCreateOrUpdate
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var deploymentStackAtSubscription = new AzureNative.Resources.DeploymentStackAtSubscription("deploymentStackAtSubscription", new()
* {
* ActionOnUnmanage = new AzureNative.Resources.Inputs.DeploymentStackPropertiesActionOnUnmanageArgs
* {
* ManagementGroups = AzureNative.Resources.DeploymentStacksDeleteDetachEnum.Detach,
* ResourceGroups = AzureNative.Resources.DeploymentStacksDeleteDetachEnum.Delete,
* Resources = AzureNative.Resources.DeploymentStacksDeleteDetachEnum.Delete,
* },
* DenySettings = new AzureNative.Resources.Inputs.DenySettingsArgs
* {
* ApplyToChildScopes = false,
* ExcludedActions = new[]
* {
* "action",
* },
* ExcludedPrincipals = new[]
* {
* "principal",
* },
* Mode = AzureNative.Resources.DenySettingsMode.DenyDelete,
* },
* DeploymentStackName = "simpleDeploymentStack",
* Location = "eastus",
* Parameters = new Dictionary
* {
* ["parameter1"] = new Dictionary
* {
* ["value"] = "a string",
* },
* },
* Tags =
* {
* { "tagkey", "tagVal" },
* },
* });
* });
* ```
* ```go
* package main
* import (
* resources "github.com/pulumi/pulumi-azure-native-sdk/resources/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := resources.NewDeploymentStackAtSubscription(ctx, "deploymentStackAtSubscription", &resources.DeploymentStackAtSubscriptionArgs{
* ActionOnUnmanage: &resources.DeploymentStackPropertiesActionOnUnmanageArgs{
* ManagementGroups: pulumi.String(resources.DeploymentStacksDeleteDetachEnumDetach),
* ResourceGroups: pulumi.String(resources.DeploymentStacksDeleteDetachEnumDelete),
* Resources: pulumi.String(resources.DeploymentStacksDeleteDetachEnumDelete),
* },
* DenySettings: &resources.DenySettingsArgs{
* ApplyToChildScopes: pulumi.Bool(false),
* ExcludedActions: pulumi.StringArray{
* pulumi.String("action"),
* },
* ExcludedPrincipals: pulumi.StringArray{
* pulumi.String("principal"),
* },
* Mode: pulumi.String(resources.DenySettingsModeDenyDelete),
* },
* DeploymentStackName: pulumi.String("simpleDeploymentStack"),
* Location: pulumi.String("eastus"),
* Parameters: pulumi.Any(map[string]interface{}{
* "parameter1": map[string]interface{}{
* "value": "a string",
* },
* }),
* Tags: pulumi.StringMap{
* "tagkey": pulumi.String("tagVal"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.resources.DeploymentStackAtSubscription;
* import com.pulumi.azurenative.resources.DeploymentStackAtSubscriptionArgs;
* import com.pulumi.azurenative.resources.inputs.DeploymentStackPropertiesActionOnUnmanageArgs;
* import com.pulumi.azurenative.resources.inputs.DenySettingsArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var deploymentStackAtSubscription = new DeploymentStackAtSubscription("deploymentStackAtSubscription", DeploymentStackAtSubscriptionArgs.builder()
* .actionOnUnmanage(DeploymentStackPropertiesActionOnUnmanageArgs.builder()
* .managementGroups("detach")
* .resourceGroups("delete")
* .resources("delete")
* .build())
* .denySettings(DenySettingsArgs.builder()
* .applyToChildScopes(false)
* .excludedActions("action")
* .excludedPrincipals("principal")
* .mode("denyDelete")
* .build())
* .deploymentStackName("simpleDeploymentStack")
* .location("eastus")
* .parameters(Map.of("parameter1", Map.of("value", "a string")))
* .tags(Map.of("tagkey", "tagVal"))
* .build());
* }
* }
* ```
* ## Import
* An existing resource can be imported using its type token, name, and identifier, e.g.
* ```sh
* $ pulumi import azure-native:resources:DeploymentStackAtSubscription simpleDeploymentStack /subscriptions/{subscriptionId}/providers/Microsoft.Resources/deploymentStacks/{deploymentStackName}
* ```
*/
public class DeploymentStackAtSubscription internal constructor(
override val javaResource: com.pulumi.azurenative.resources.DeploymentStackAtSubscription,
) : KotlinCustomResource(javaResource, DeploymentStackAtSubscriptionMapper) {
/**
* Defines the behavior of resources that are not managed immediately after the stack is updated.
*/
public val actionOnUnmanage: Output
get() = javaResource.actionOnUnmanage().applyValue({ args0 ->
args0.let({ args0 ->
deploymentStackPropertiesResponseActionOnUnmanageToKotlin(args0)
})
})
/**
* The debug setting of the deployment.
*/
public val debugSetting: Output?
get() = javaResource.debugSetting().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
deploymentStacksDebugSettingResponseToKotlin(args0)
})
}).orElse(null)
})
/**
* An array of resources that were deleted during the most recent update.
*/
public val deletedResources: Output>
get() = javaResource.deletedResources().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> resourceReferenceResponseToKotlin(args0) })
})
})
/**
* Defines how resources deployed by the stack are locked.
*/
public val denySettings: Output
get() = javaResource.denySettings().applyValue({ args0 ->
args0.let({ args0 ->
denySettingsResponseToKotlin(args0)
})
})
/**
* The resourceId of the deployment resource created by the deployment stack.
*/
public val deploymentId: Output
get() = javaResource.deploymentId().applyValue({ args0 -> args0 })
/**
* The scope at which the initial deployment should be created. If a scope is not specified, it will default to the scope of the deployment stack. Valid scopes are: management group (format: '/providers/Microsoft.Management/managementGroups/{managementGroupId}'), subscription (format: '/subscriptions/{subscriptionId}'), resource group (format: '/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}').
*/
public val deploymentScope: Output?
get() = javaResource.deploymentScope().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Deployment stack description.
*/
public val description: Output?
get() = javaResource.description().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* An array of resources that were detached during the most recent update.
*/
public val detachedResources: Output>
get() = javaResource.detachedResources().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> resourceReferenceResponseToKotlin(args0) })
})
})
/**
* The duration of the deployment stack update.
*/
public val duration: Output
get() = javaResource.duration().applyValue({ args0 -> args0 })
/**
* Common error response for all Azure Resource Manager APIs to return error details for failed operations. (This also follows the OData error response format.).
*/
public val error: Output?
get() = javaResource.error().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
errorResponseResponseToKotlin(args0)
})
}).orElse(null)
})
/**
* An array of resources that failed to reach goal state during the most recent update.
*/
public val failedResources: Output>
get() = javaResource.failedResources().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> resourceReferenceExtendedResponseToKotlin(args0) })
})
})
/**
* The location of the deployment stack. It cannot be changed after creation. It must be one of the supported Azure locations.
*/
public val location: Output?
get() = javaResource.location().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* Name of this resource.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* The outputs of the underlying deployment.
*/
public val outputs: Output
get() = javaResource.outputs().applyValue({ args0 -> args0 })
/**
* Name and value pairs that define the deployment parameters for the template. Use this element when providing the parameter values directly in the request, rather than linking to an existing parameter file. Use either the parametersLink property or the parameters property, but not both. It can be a JObject or a well formed JSON string.
*/
public val parameters: Output?
get() = javaResource.parameters().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The URI of parameters file. Use this element to link to an existing parameters file. Use either the parametersLink property or the parameters property, but not both.
*/
public val parametersLink: Output?
get() = javaResource.parametersLink().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> deploymentStacksParametersLinkResponseToKotlin(args0) })
}).orElse(null)
})
/**
* State of the deployment stack.
*/
public val provisioningState: Output
get() = javaResource.provisioningState().applyValue({ args0 -> args0 })
/**
* An array of resources currently managed by the deployment stack.
*/
public val resources: Output>
get() = javaResource.resources().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
managedResourceReferenceResponseToKotlin(args0)
})
})
})
/**
* Azure Resource Manager metadata containing createdBy and modifiedBy information.
*/
public val systemData: Output
get() = javaResource.systemData().applyValue({ args0 ->
args0.let({ args0 ->
systemDataResponseToKotlin(args0)
})
})
/**
* Deployment stack resource tags.
*/
public val tags: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy