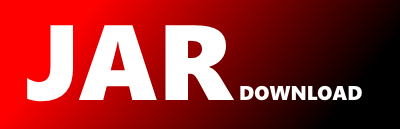
com.pulumi.azurenative.saas.kotlin.SaasSubscriptionLevelArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.saas.kotlin
import com.pulumi.azurenative.saas.SaasSubscriptionLevelArgs.builder
import com.pulumi.azurenative.saas.kotlin.inputs.SaasCreationPropertiesArgs
import com.pulumi.azurenative.saas.kotlin.inputs.SaasCreationPropertiesArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* SaaS REST API resource definition.
* Azure REST API version: 2018-03-01-beta. Prior API version in Azure Native 1.x: 2018-03-01-beta.
* ## Example Usage
* ### Create subscription level SaaS resource
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var saasSubscriptionLevel = new AzureNative.SaaS.SaasSubscriptionLevel("saasSubscriptionLevel", new()
* {
* Location = "global",
* Name = "MyContosoSubscription",
* Properties = new AzureNative.SaaS.Inputs.SaasCreationPropertiesArgs
* {
* OfferId = "contosoOffer",
* PaymentChannelMetadata =
* {
* { "AzureSubscriptionId", "155af98a-3205-47e7-883b-a2ab9db9f88d" },
* },
* PaymentChannelType = AzureNative.SaaS.PaymentChannelType.SubscriptionDelegated,
* PublisherId = "microsoft-contoso",
* SaasResourceName = "MyContosoSubscription",
* SkuId = "free",
* TermId = "hjdtn7tfnxcy",
* },
* ResourceGroupName = "my-saas-rg",
* ResourceName = "MyContosoSubscription",
* });
* });
* ```
* ```go
* package main
* import (
* saas "github.com/pulumi/pulumi-azure-native-sdk/saas/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := saas.NewSaasSubscriptionLevel(ctx, "saasSubscriptionLevel", &saas.SaasSubscriptionLevelArgs{
* Location: pulumi.String("global"),
* Name: pulumi.String("MyContosoSubscription"),
* Properties: &saas.SaasCreationPropertiesArgs{
* OfferId: pulumi.String("contosoOffer"),
* PaymentChannelMetadata: pulumi.StringMap{
* "AzureSubscriptionId": pulumi.String("155af98a-3205-47e7-883b-a2ab9db9f88d"),
* },
* PaymentChannelType: pulumi.String(saas.PaymentChannelTypeSubscriptionDelegated),
* PublisherId: pulumi.String("microsoft-contoso"),
* SaasResourceName: pulumi.String("MyContosoSubscription"),
* SkuId: pulumi.String("free"),
* TermId: pulumi.String("hjdtn7tfnxcy"),
* },
* ResourceGroupName: pulumi.String("my-saas-rg"),
* ResourceName: pulumi.String("MyContosoSubscription"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.saas.SaasSubscriptionLevel;
* import com.pulumi.azurenative.saas.SaasSubscriptionLevelArgs;
* import com.pulumi.azurenative.saas.inputs.SaasCreationPropertiesArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var saasSubscriptionLevel = new SaasSubscriptionLevel("saasSubscriptionLevel", SaasSubscriptionLevelArgs.builder()
* .location("global")
* .name("MyContosoSubscription")
* .properties(SaasCreationPropertiesArgs.builder()
* .offerId("contosoOffer")
* .paymentChannelMetadata(Map.of("AzureSubscriptionId", "155af98a-3205-47e7-883b-a2ab9db9f88d"))
* .paymentChannelType("SubscriptionDelegated")
* .publisherId("microsoft-contoso")
* .saasResourceName("MyContosoSubscription")
* .skuId("free")
* .termId("hjdtn7tfnxcy")
* .build())
* .resourceGroupName("my-saas-rg")
* .resourceName("MyContosoSubscription")
* .build());
* }
* }
* ```
* ## Import
* An existing resource can be imported using its type token, name, and identifier, e.g.
* ```sh
* $ pulumi import azure-native:saas:SaasSubscriptionLevel MyContosoSubscription /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.SaaS/resources/{resourceName}
* ```
* @property location Resource location. Only value allowed for SaaS is 'global'
* @property name The resource name
* @property properties Properties of the SaaS resource that are relevant for creation.
* @property resourceGroupName The name of the resource group.
* @property resourceName The name of the resource.
* @property tags the resource tags.
*/
public data class SaasSubscriptionLevelArgs(
public val location: Output? = null,
public val name: Output? = null,
public val properties: Output? = null,
public val resourceGroupName: Output? = null,
public val resourceName: Output? = null,
public val tags: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy