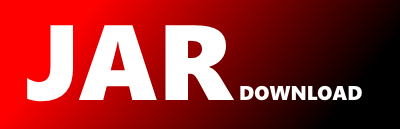
com.pulumi.azurenative.scheduler.kotlin.inputs.JobActionArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.scheduler.kotlin.inputs
import com.pulumi.azurenative.scheduler.inputs.JobActionArgs.builder
import com.pulumi.azurenative.scheduler.kotlin.enums.JobActionType
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property errorAction Gets or sets the error action.
* @property queueMessage Gets or sets the storage queue message.
* @property request Gets or sets the http requests.
* @property retryPolicy Gets or sets the retry policy.
* @property serviceBusQueueMessage Gets or sets the service bus queue message.
* @property serviceBusTopicMessage Gets or sets the service bus topic message.
* @property type Gets or sets the job action type.
*/
public data class JobActionArgs(
public val errorAction: Output? = null,
public val queueMessage: Output? = null,
public val request: Output? = null,
public val retryPolicy: Output? = null,
public val serviceBusQueueMessage: Output? = null,
public val serviceBusTopicMessage: Output? = null,
public val type: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.scheduler.inputs.JobActionArgs =
com.pulumi.azurenative.scheduler.inputs.JobActionArgs.builder()
.errorAction(errorAction?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.queueMessage(queueMessage?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.request(request?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.retryPolicy(retryPolicy?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.serviceBusQueueMessage(
serviceBusQueueMessage?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.serviceBusTopicMessage(
serviceBusTopicMessage?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.type(type?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [JobActionArgs].
*/
@PulumiTagMarker
public class JobActionArgsBuilder internal constructor() {
private var errorAction: Output? = null
private var queueMessage: Output? = null
private var request: Output? = null
private var retryPolicy: Output? = null
private var serviceBusQueueMessage: Output? = null
private var serviceBusTopicMessage: Output? = null
private var type: Output? = null
/**
* @param value Gets or sets the error action.
*/
@JvmName("eqieuqplmsbcebtf")
public suspend fun errorAction(`value`: Output) {
this.errorAction = value
}
/**
* @param value Gets or sets the storage queue message.
*/
@JvmName("nmemevfudgredvoa")
public suspend fun queueMessage(`value`: Output) {
this.queueMessage = value
}
/**
* @param value Gets or sets the http requests.
*/
@JvmName("xirytfvwxccqpwtm")
public suspend fun request(`value`: Output) {
this.request = value
}
/**
* @param value Gets or sets the retry policy.
*/
@JvmName("nyayjhiarwalvlni")
public suspend fun retryPolicy(`value`: Output) {
this.retryPolicy = value
}
/**
* @param value Gets or sets the service bus queue message.
*/
@JvmName("abtktgatemwgxeqa")
public suspend fun serviceBusQueueMessage(`value`: Output) {
this.serviceBusQueueMessage = value
}
/**
* @param value Gets or sets the service bus topic message.
*/
@JvmName("dghpfmpgyllwjvqb")
public suspend fun serviceBusTopicMessage(`value`: Output) {
this.serviceBusTopicMessage = value
}
/**
* @param value Gets or sets the job action type.
*/
@JvmName("gcrqxvoxudbmyiew")
public suspend fun type(`value`: Output) {
this.type = value
}
/**
* @param value Gets or sets the error action.
*/
@JvmName("qamrkoxxiloxfnal")
public suspend fun errorAction(`value`: JobErrorActionArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.errorAction = mapped
}
/**
* @param argument Gets or sets the error action.
*/
@JvmName("eyltxrtdvujgptwi")
public suspend fun errorAction(argument: suspend JobErrorActionArgsBuilder.() -> Unit) {
val toBeMapped = JobErrorActionArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.errorAction = mapped
}
/**
* @param value Gets or sets the storage queue message.
*/
@JvmName("vwxsurjokrxixysc")
public suspend fun queueMessage(`value`: StorageQueueMessageArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.queueMessage = mapped
}
/**
* @param argument Gets or sets the storage queue message.
*/
@JvmName("wqqlcstsrpdiducm")
public suspend fun queueMessage(argument: suspend StorageQueueMessageArgsBuilder.() -> Unit) {
val toBeMapped = StorageQueueMessageArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.queueMessage = mapped
}
/**
* @param value Gets or sets the http requests.
*/
@JvmName("jnkxurvdxqarwpeu")
public suspend fun request(`value`: HttpRequestArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.request = mapped
}
/**
* @param argument Gets or sets the http requests.
*/
@JvmName("nwqyyrdwdgfsqpss")
public suspend fun request(argument: suspend HttpRequestArgsBuilder.() -> Unit) {
val toBeMapped = HttpRequestArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.request = mapped
}
/**
* @param value Gets or sets the retry policy.
*/
@JvmName("krkoyxjqubkuyajx")
public suspend fun retryPolicy(`value`: RetryPolicyArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.retryPolicy = mapped
}
/**
* @param argument Gets or sets the retry policy.
*/
@JvmName("xbopsbkicllagadc")
public suspend fun retryPolicy(argument: suspend RetryPolicyArgsBuilder.() -> Unit) {
val toBeMapped = RetryPolicyArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.retryPolicy = mapped
}
/**
* @param value Gets or sets the service bus queue message.
*/
@JvmName("guuuqwxleukyfqta")
public suspend fun serviceBusQueueMessage(`value`: ServiceBusQueueMessageArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.serviceBusQueueMessage = mapped
}
/**
* @param argument Gets or sets the service bus queue message.
*/
@JvmName("rqatkdtlgbqkobat")
public suspend fun serviceBusQueueMessage(argument: suspend ServiceBusQueueMessageArgsBuilder.() -> Unit) {
val toBeMapped = ServiceBusQueueMessageArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.serviceBusQueueMessage = mapped
}
/**
* @param value Gets or sets the service bus topic message.
*/
@JvmName("xdwigkmdvcywwoil")
public suspend fun serviceBusTopicMessage(`value`: ServiceBusTopicMessageArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.serviceBusTopicMessage = mapped
}
/**
* @param argument Gets or sets the service bus topic message.
*/
@JvmName("etylemfudrdgygdk")
public suspend fun serviceBusTopicMessage(argument: suspend ServiceBusTopicMessageArgsBuilder.() -> Unit) {
val toBeMapped = ServiceBusTopicMessageArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.serviceBusTopicMessage = mapped
}
/**
* @param value Gets or sets the job action type.
*/
@JvmName("mhspdseirehhgdys")
public suspend fun type(`value`: JobActionType?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.type = mapped
}
internal fun build(): JobActionArgs = JobActionArgs(
errorAction = errorAction,
queueMessage = queueMessage,
request = request,
retryPolicy = retryPolicy,
serviceBusQueueMessage = serviceBusQueueMessage,
serviceBusTopicMessage = serviceBusTopicMessage,
type = type,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy