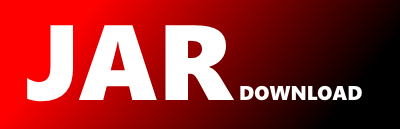
com.pulumi.azurenative.scheduler.kotlin.inputs.JobRecurrenceArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.scheduler.kotlin.inputs
import com.pulumi.azurenative.scheduler.inputs.JobRecurrenceArgs.builder
import com.pulumi.azurenative.scheduler.kotlin.enums.RecurrenceFrequency
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property count Gets or sets the maximum number of times that the job should run.
* @property endTime Gets or sets the time at which the job will complete.
* @property frequency Gets or sets the frequency of recurrence (second, minute, hour, day, week, month).
* @property interval Gets or sets the interval between retries.
* @property schedule
*/
public data class JobRecurrenceArgs(
public val count: Output? = null,
public val endTime: Output? = null,
public val frequency: Output? = null,
public val interval: Output? = null,
public val schedule: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.scheduler.inputs.JobRecurrenceArgs =
com.pulumi.azurenative.scheduler.inputs.JobRecurrenceArgs.builder()
.count(count?.applyValue({ args0 -> args0 }))
.endTime(endTime?.applyValue({ args0 -> args0 }))
.frequency(frequency?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.interval(interval?.applyValue({ args0 -> args0 }))
.schedule(schedule?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [JobRecurrenceArgs].
*/
@PulumiTagMarker
public class JobRecurrenceArgsBuilder internal constructor() {
private var count: Output? = null
private var endTime: Output? = null
private var frequency: Output? = null
private var interval: Output? = null
private var schedule: Output? = null
/**
* @param value Gets or sets the maximum number of times that the job should run.
*/
@JvmName("hyiararawsapwiww")
public suspend fun count(`value`: Output) {
this.count = value
}
/**
* @param value Gets or sets the time at which the job will complete.
*/
@JvmName("hpvjkbqcbwpvudeo")
public suspend fun endTime(`value`: Output) {
this.endTime = value
}
/**
* @param value Gets or sets the frequency of recurrence (second, minute, hour, day, week, month).
*/
@JvmName("mibysfmlwhhaqtsm")
public suspend fun frequency(`value`: Output) {
this.frequency = value
}
/**
* @param value Gets or sets the interval between retries.
*/
@JvmName("puaildudagtxautx")
public suspend fun interval(`value`: Output) {
this.interval = value
}
/**
* @param value
*/
@JvmName("fslwiaaqjjaulfdq")
public suspend fun schedule(`value`: Output) {
this.schedule = value
}
/**
* @param value Gets or sets the maximum number of times that the job should run.
*/
@JvmName("cjyykmspbbncxahp")
public suspend fun count(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.count = mapped
}
/**
* @param value Gets or sets the time at which the job will complete.
*/
@JvmName("psjtwoapgiukwdur")
public suspend fun endTime(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.endTime = mapped
}
/**
* @param value Gets or sets the frequency of recurrence (second, minute, hour, day, week, month).
*/
@JvmName("ytmekmanqqfujbqi")
public suspend fun frequency(`value`: RecurrenceFrequency?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.frequency = mapped
}
/**
* @param value Gets or sets the interval between retries.
*/
@JvmName("lofwdpdfojaqsmlx")
public suspend fun interval(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.interval = mapped
}
/**
* @param value
*/
@JvmName("cpgpwxlyioxbpfjf")
public suspend fun schedule(`value`: JobRecurrenceScheduleArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.schedule = mapped
}
/**
* @param argument
*/
@JvmName("ugfmpdvmjbqexnwk")
public suspend fun schedule(argument: suspend JobRecurrenceScheduleArgsBuilder.() -> Unit) {
val toBeMapped = JobRecurrenceScheduleArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.schedule = mapped
}
internal fun build(): JobRecurrenceArgs = JobRecurrenceArgs(
count = count,
endTime = endTime,
frequency = frequency,
interval = interval,
schedule = schedule,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy