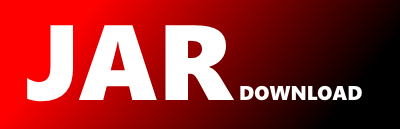
com.pulumi.azurenative.scom.kotlin.inputs.AzureHybridBenefitPropertiesArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.scom.kotlin.inputs
import com.pulumi.azurenative.scom.inputs.AzureHybridBenefitPropertiesArgs.builder
import com.pulumi.azurenative.scom.kotlin.enums.HybridLicenseType
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* The properties to maximize savings by using Azure Hybrid Benefit
* @property scomLicenseType SCOM license type. Maximize savings by using license you already own
* @property sqlServerLicenseType SQL Server license type. Maximize savings by using Azure Hybrid Benefit for SQL Server with license you already own
* @property windowsServerLicenseType Specifies that the image or disk that is being used was licensed on-premises.
For more information, see [Azure Hybrid Use Benefit for Windows Server](https://docs.microsoft.com/azure/virtual-machines/virtual-machines-windows-hybrid-use-benefit-licensing?toc=%2fazure%2fvirtual-machines%2fwindows%2ftoc.json)
*/
public data class AzureHybridBenefitPropertiesArgs(
public val scomLicenseType: Output>? = null,
public val sqlServerLicenseType: Output>? = null,
public val windowsServerLicenseType: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.scom.inputs.AzureHybridBenefitPropertiesArgs =
com.pulumi.azurenative.scom.inputs.AzureHybridBenefitPropertiesArgs.builder()
.scomLicenseType(
scomLicenseType?.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.sqlServerLicenseType(
sqlServerLicenseType?.applyValue({ args0 ->
args0.transform(
{ args0 -> args0 },
{ args0 -> args0.let({ args0 -> args0.toJava() }) },
)
}),
)
.windowsServerLicenseType(
windowsServerLicenseType?.applyValue({ args0 ->
args0.transform({ args0 ->
args0
}, { args0 -> args0.let({ args0 -> args0.toJava() }) })
}),
).build()
}
/**
* Builder for [AzureHybridBenefitPropertiesArgs].
*/
@PulumiTagMarker
public class AzureHybridBenefitPropertiesArgsBuilder internal constructor() {
private var scomLicenseType: Output>? = null
private var sqlServerLicenseType: Output>? = null
private var windowsServerLicenseType: Output>? = null
/**
* @param value SCOM license type. Maximize savings by using license you already own
*/
@JvmName("fxemjkcwtdgeyaaq")
public suspend fun scomLicenseType(`value`: Output>) {
this.scomLicenseType = value
}
/**
* @param value SQL Server license type. Maximize savings by using Azure Hybrid Benefit for SQL Server with license you already own
*/
@JvmName("cnlwcmdfixooxvwj")
public suspend fun sqlServerLicenseType(`value`: Output>) {
this.sqlServerLicenseType = value
}
/**
* @param value Specifies that the image or disk that is being used was licensed on-premises.
For more information, see [Azure Hybrid Use Benefit for Windows Server](https://docs.microsoft.com/azure/virtual-machines/virtual-machines-windows-hybrid-use-benefit-licensing?toc=%2fazure%2fvirtual-machines%2fwindows%2ftoc.json)
*/
@JvmName("tkccaiqqxjakvvaq")
public suspend fun windowsServerLicenseType(`value`: Output>) {
this.windowsServerLicenseType = value
}
/**
* @param value SCOM license type. Maximize savings by using license you already own
*/
@JvmName("xoslspjdexpclbpj")
public suspend fun scomLicenseType(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.scomLicenseType = mapped
}
/**
* @param value SCOM license type. Maximize savings by using license you already own
*/
@JvmName("vfwlmvkoobokcsux")
public fun scomLicenseType(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.scomLicenseType = mapped
}
/**
* @param value SCOM license type. Maximize savings by using license you already own
*/
@JvmName("uwgommgjgjmdcnwx")
public fun scomLicenseType(`value`: HybridLicenseType) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.scomLicenseType = mapped
}
/**
* @param value SQL Server license type. Maximize savings by using Azure Hybrid Benefit for SQL Server with license you already own
*/
@JvmName("bxmncobbxggqptgo")
public suspend fun sqlServerLicenseType(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sqlServerLicenseType = mapped
}
/**
* @param value SQL Server license type. Maximize savings by using Azure Hybrid Benefit for SQL Server with license you already own
*/
@JvmName("iqftputdpfjwwotv")
public fun sqlServerLicenseType(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.sqlServerLicenseType = mapped
}
/**
* @param value SQL Server license type. Maximize savings by using Azure Hybrid Benefit for SQL Server with license you already own
*/
@JvmName("xrbainbjivbvdrnv")
public fun sqlServerLicenseType(`value`: HybridLicenseType) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.sqlServerLicenseType = mapped
}
/**
* @param value Specifies that the image or disk that is being used was licensed on-premises.
For more information, see [Azure Hybrid Use Benefit for Windows Server](https://docs.microsoft.com/azure/virtual-machines/virtual-machines-windows-hybrid-use-benefit-licensing?toc=%2fazure%2fvirtual-machines%2fwindows%2ftoc.json)
*/
@JvmName("irwrefpxqlgtsvlj")
public suspend fun windowsServerLicenseType(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.windowsServerLicenseType = mapped
}
/**
* @param value Specifies that the image or disk that is being used was licensed on-premises.
For more information, see [Azure Hybrid Use Benefit for Windows Server](https://docs.microsoft.com/azure/virtual-machines/virtual-machines-windows-hybrid-use-benefit-licensing?toc=%2fazure%2fvirtual-machines%2fwindows%2ftoc.json)
*/
@JvmName("fxqpvisnxusmimvv")
public fun windowsServerLicenseType(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.windowsServerLicenseType = mapped
}
/**
* @param value Specifies that the image or disk that is being used was licensed on-premises.
For more information, see [Azure Hybrid Use Benefit for Windows Server](https://docs.microsoft.com/azure/virtual-machines/virtual-machines-windows-hybrid-use-benefit-licensing?toc=%2fazure%2fvirtual-machines%2fwindows%2ftoc.json)
*/
@JvmName("gotdaewfpxpxxuts")
public fun windowsServerLicenseType(`value`: HybridLicenseType) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.windowsServerLicenseType = mapped
}
internal fun build(): AzureHybridBenefitPropertiesArgs = AzureHybridBenefitPropertiesArgs(
scomLicenseType = scomLicenseType,
sqlServerLicenseType = sqlServerLicenseType,
windowsServerLicenseType = windowsServerLicenseType,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy