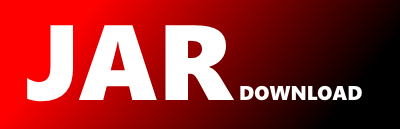
com.pulumi.azurenative.scvmm.kotlin.inputs.NetworkInterfacesArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.scvmm.kotlin.inputs
import com.pulumi.azurenative.scvmm.inputs.NetworkInterfacesArgs.builder
import com.pulumi.azurenative.scvmm.kotlin.enums.AllocationMethod
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Network Interface model
* @property ipv4AddressType Gets or sets the ipv4 address type.
* @property ipv6AddressType Gets or sets the ipv6 address type.
* @property macAddress Gets or sets the nic MAC address.
* @property macAddressType Gets or sets the mac address type.
* @property name Gets or sets the name of the network interface.
* @property nicId Gets or sets the nic id.
* @property virtualNetworkId Gets or sets the ARM Id of the Microsoft.ScVmm/virtualNetwork resource to connect the nic.
*/
public data class NetworkInterfacesArgs(
public val ipv4AddressType: Output>? = null,
public val ipv6AddressType: Output>? = null,
public val macAddress: Output? = null,
public val macAddressType: Output>? = null,
public val name: Output? = null,
public val nicId: Output? = null,
public val virtualNetworkId: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.scvmm.inputs.NetworkInterfacesArgs =
com.pulumi.azurenative.scvmm.inputs.NetworkInterfacesArgs.builder()
.ipv4AddressType(
ipv4AddressType?.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.ipv6AddressType(
ipv6AddressType?.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.macAddress(macAddress?.applyValue({ args0 -> args0 }))
.macAddressType(
macAddressType?.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.name(name?.applyValue({ args0 -> args0 }))
.nicId(nicId?.applyValue({ args0 -> args0 }))
.virtualNetworkId(virtualNetworkId?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [NetworkInterfacesArgs].
*/
@PulumiTagMarker
public class NetworkInterfacesArgsBuilder internal constructor() {
private var ipv4AddressType: Output>? = null
private var ipv6AddressType: Output>? = null
private var macAddress: Output? = null
private var macAddressType: Output>? = null
private var name: Output? = null
private var nicId: Output? = null
private var virtualNetworkId: Output? = null
/**
* @param value Gets or sets the ipv4 address type.
*/
@JvmName("msgulmlskvdeeecx")
public suspend fun ipv4AddressType(`value`: Output>) {
this.ipv4AddressType = value
}
/**
* @param value Gets or sets the ipv6 address type.
*/
@JvmName("kfchgnikelumpjst")
public suspend fun ipv6AddressType(`value`: Output>) {
this.ipv6AddressType = value
}
/**
* @param value Gets or sets the nic MAC address.
*/
@JvmName("cuwkeqklhuqlbhde")
public suspend fun macAddress(`value`: Output) {
this.macAddress = value
}
/**
* @param value Gets or sets the mac address type.
*/
@JvmName("tisjcfyglyuhrnrs")
public suspend fun macAddressType(`value`: Output>) {
this.macAddressType = value
}
/**
* @param value Gets or sets the name of the network interface.
*/
@JvmName("otalvwbaqqaedgft")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value Gets or sets the nic id.
*/
@JvmName("hylstruwfpkmuocg")
public suspend fun nicId(`value`: Output) {
this.nicId = value
}
/**
* @param value Gets or sets the ARM Id of the Microsoft.ScVmm/virtualNetwork resource to connect the nic.
*/
@JvmName("msuqkxbiplttyffj")
public suspend fun virtualNetworkId(`value`: Output) {
this.virtualNetworkId = value
}
/**
* @param value Gets or sets the ipv4 address type.
*/
@JvmName("qpbvrshhbdmjolpb")
public suspend fun ipv4AddressType(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.ipv4AddressType = mapped
}
/**
* @param value Gets or sets the ipv4 address type.
*/
@JvmName("agfutnxvftpyqore")
public fun ipv4AddressType(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.ipv4AddressType = mapped
}
/**
* @param value Gets or sets the ipv4 address type.
*/
@JvmName("fpgsyistokgmghvo")
public fun ipv4AddressType(`value`: AllocationMethod) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.ipv4AddressType = mapped
}
/**
* @param value Gets or sets the ipv6 address type.
*/
@JvmName("osyogwrvsedbypqp")
public suspend fun ipv6AddressType(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.ipv6AddressType = mapped
}
/**
* @param value Gets or sets the ipv6 address type.
*/
@JvmName("ilterixpofkgxvxk")
public fun ipv6AddressType(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.ipv6AddressType = mapped
}
/**
* @param value Gets or sets the ipv6 address type.
*/
@JvmName("sghhtjwkbkhdpqku")
public fun ipv6AddressType(`value`: AllocationMethod) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.ipv6AddressType = mapped
}
/**
* @param value Gets or sets the nic MAC address.
*/
@JvmName("alpkoqucqvxwocht")
public suspend fun macAddress(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.macAddress = mapped
}
/**
* @param value Gets or sets the mac address type.
*/
@JvmName("nubksaorbnsexhut")
public suspend fun macAddressType(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.macAddressType = mapped
}
/**
* @param value Gets or sets the mac address type.
*/
@JvmName("dkhamflldguowmim")
public fun macAddressType(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.macAddressType = mapped
}
/**
* @param value Gets or sets the mac address type.
*/
@JvmName("ucegikbjipbuovay")
public fun macAddressType(`value`: AllocationMethod) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.macAddressType = mapped
}
/**
* @param value Gets or sets the name of the network interface.
*/
@JvmName("geqgotdxrfyspdmo")
public suspend fun name(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value Gets or sets the nic id.
*/
@JvmName("lotknjifmxlxffcd")
public suspend fun nicId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.nicId = mapped
}
/**
* @param value Gets or sets the ARM Id of the Microsoft.ScVmm/virtualNetwork resource to connect the nic.
*/
@JvmName("tqqxqnqwtpslerxt")
public suspend fun virtualNetworkId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.virtualNetworkId = mapped
}
internal fun build(): NetworkInterfacesArgs = NetworkInterfacesArgs(
ipv4AddressType = ipv4AddressType,
ipv6AddressType = ipv6AddressType,
macAddress = macAddress,
macAddressType = macAddressType,
name = name,
nicId = nicId,
virtualNetworkId = virtualNetworkId,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy