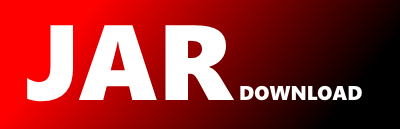
com.pulumi.azurenative.scvmm.kotlin.inputs.VirtualDiskArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.scvmm.kotlin.inputs
import com.pulumi.azurenative.scvmm.inputs.VirtualDiskArgs.builder
import com.pulumi.azurenative.scvmm.kotlin.enums.CreateDiffDisk
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Virtual disk model
* @property bus Gets or sets the disk bus.
* @property busType Gets or sets the disk bus type.
* @property createDiffDisk Gets or sets a value indicating diff disk.
* @property diskId Gets or sets the disk id.
* @property diskSizeGB Gets or sets the disk total size.
* @property lun Gets or sets the disk lun.
* @property name Gets or sets the name of the disk.
* @property storageQoSPolicy The QoS policy for the disk.
* @property templateDiskId Gets or sets the disk id in the template.
* @property vhdType Gets or sets the disk vhd type.
*/
public data class VirtualDiskArgs(
public val bus: Output? = null,
public val busType: Output? = null,
public val createDiffDisk: Output>? = null,
public val diskId: Output? = null,
public val diskSizeGB: Output? = null,
public val lun: Output? = null,
public val name: Output? = null,
public val storageQoSPolicy: Output? = null,
public val templateDiskId: Output? = null,
public val vhdType: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.scvmm.inputs.VirtualDiskArgs =
com.pulumi.azurenative.scvmm.inputs.VirtualDiskArgs.builder()
.bus(bus?.applyValue({ args0 -> args0 }))
.busType(busType?.applyValue({ args0 -> args0 }))
.createDiffDisk(
createDiffDisk?.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.diskId(diskId?.applyValue({ args0 -> args0 }))
.diskSizeGB(diskSizeGB?.applyValue({ args0 -> args0 }))
.lun(lun?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.storageQoSPolicy(storageQoSPolicy?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.templateDiskId(templateDiskId?.applyValue({ args0 -> args0 }))
.vhdType(vhdType?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [VirtualDiskArgs].
*/
@PulumiTagMarker
public class VirtualDiskArgsBuilder internal constructor() {
private var bus: Output? = null
private var busType: Output? = null
private var createDiffDisk: Output>? = null
private var diskId: Output? = null
private var diskSizeGB: Output? = null
private var lun: Output? = null
private var name: Output? = null
private var storageQoSPolicy: Output? = null
private var templateDiskId: Output? = null
private var vhdType: Output? = null
/**
* @param value Gets or sets the disk bus.
*/
@JvmName("bqlxerstyfjqhcxu")
public suspend fun bus(`value`: Output) {
this.bus = value
}
/**
* @param value Gets or sets the disk bus type.
*/
@JvmName("boovtyxxnthwnoae")
public suspend fun busType(`value`: Output) {
this.busType = value
}
/**
* @param value Gets or sets a value indicating diff disk.
*/
@JvmName("ujulocggbgvblifo")
public suspend fun createDiffDisk(`value`: Output>) {
this.createDiffDisk = value
}
/**
* @param value Gets or sets the disk id.
*/
@JvmName("iyjulvwgwjacqxcf")
public suspend fun diskId(`value`: Output) {
this.diskId = value
}
/**
* @param value Gets or sets the disk total size.
*/
@JvmName("ujemxgplyfrmakmg")
public suspend fun diskSizeGB(`value`: Output) {
this.diskSizeGB = value
}
/**
* @param value Gets or sets the disk lun.
*/
@JvmName("oqspxjwbrejbnfqc")
public suspend fun lun(`value`: Output) {
this.lun = value
}
/**
* @param value Gets or sets the name of the disk.
*/
@JvmName("paehydudchbkclqy")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value The QoS policy for the disk.
*/
@JvmName("vrqfsdnrwunscgvo")
public suspend fun storageQoSPolicy(`value`: Output) {
this.storageQoSPolicy = value
}
/**
* @param value Gets or sets the disk id in the template.
*/
@JvmName("raxyfrqqlybyiddh")
public suspend fun templateDiskId(`value`: Output) {
this.templateDiskId = value
}
/**
* @param value Gets or sets the disk vhd type.
*/
@JvmName("jkbkxmmchwddiidx")
public suspend fun vhdType(`value`: Output) {
this.vhdType = value
}
/**
* @param value Gets or sets the disk bus.
*/
@JvmName("lpxxriamiikigjvp")
public suspend fun bus(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.bus = mapped
}
/**
* @param value Gets or sets the disk bus type.
*/
@JvmName("snokboqypitosewg")
public suspend fun busType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.busType = mapped
}
/**
* @param value Gets or sets a value indicating diff disk.
*/
@JvmName("borungruetcvswrh")
public suspend fun createDiffDisk(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.createDiffDisk = mapped
}
/**
* @param value Gets or sets a value indicating diff disk.
*/
@JvmName("ljsvkfpwoeugyneq")
public fun createDiffDisk(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.createDiffDisk = mapped
}
/**
* @param value Gets or sets a value indicating diff disk.
*/
@JvmName("wghkjyqrislxbwor")
public fun createDiffDisk(`value`: CreateDiffDisk) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.createDiffDisk = mapped
}
/**
* @param value Gets or sets the disk id.
*/
@JvmName("hoclmsiiifuctorm")
public suspend fun diskId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.diskId = mapped
}
/**
* @param value Gets or sets the disk total size.
*/
@JvmName("thajfhdgmllwbkft")
public suspend fun diskSizeGB(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.diskSizeGB = mapped
}
/**
* @param value Gets or sets the disk lun.
*/
@JvmName("wgdtwqheyatqrvvb")
public suspend fun lun(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.lun = mapped
}
/**
* @param value Gets or sets the name of the disk.
*/
@JvmName("sfwqdburlskkrlqx")
public suspend fun name(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value The QoS policy for the disk.
*/
@JvmName("cfiyfcvktubvcktq")
public suspend fun storageQoSPolicy(`value`: StorageQoSPolicyDetailsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.storageQoSPolicy = mapped
}
/**
* @param argument The QoS policy for the disk.
*/
@JvmName("eyclvykueanuulbc")
public suspend fun storageQoSPolicy(argument: suspend StorageQoSPolicyDetailsArgsBuilder.() -> Unit) {
val toBeMapped = StorageQoSPolicyDetailsArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.storageQoSPolicy = mapped
}
/**
* @param value Gets or sets the disk id in the template.
*/
@JvmName("iatotvxxxrmapuka")
public suspend fun templateDiskId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.templateDiskId = mapped
}
/**
* @param value Gets or sets the disk vhd type.
*/
@JvmName("deggtqqyxajavjbe")
public suspend fun vhdType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.vhdType = mapped
}
internal fun build(): VirtualDiskArgs = VirtualDiskArgs(
bus = bus,
busType = busType,
createDiffDisk = createDiffDisk,
diskId = diskId,
diskSizeGB = diskSizeGB,
lun = lun,
name = name,
storageQoSPolicy = storageQoSPolicy,
templateDiskId = templateDiskId,
vhdType = vhdType,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy