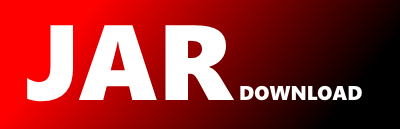
com.pulumi.azurenative.search.kotlin.SearchFunctions.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.search.kotlin
import com.pulumi.azurenative.search.SearchFunctions.getPrivateEndpointConnectionPlain
import com.pulumi.azurenative.search.SearchFunctions.getServicePlain
import com.pulumi.azurenative.search.SearchFunctions.getSharedPrivateLinkResourcePlain
import com.pulumi.azurenative.search.SearchFunctions.listAdminKeyPlain
import com.pulumi.azurenative.search.SearchFunctions.listQueryKeyBySearchServicePlain
import com.pulumi.azurenative.search.kotlin.inputs.GetPrivateEndpointConnectionPlainArgs
import com.pulumi.azurenative.search.kotlin.inputs.GetPrivateEndpointConnectionPlainArgsBuilder
import com.pulumi.azurenative.search.kotlin.inputs.GetServicePlainArgs
import com.pulumi.azurenative.search.kotlin.inputs.GetServicePlainArgsBuilder
import com.pulumi.azurenative.search.kotlin.inputs.GetSharedPrivateLinkResourcePlainArgs
import com.pulumi.azurenative.search.kotlin.inputs.GetSharedPrivateLinkResourcePlainArgsBuilder
import com.pulumi.azurenative.search.kotlin.inputs.ListAdminKeyPlainArgs
import com.pulumi.azurenative.search.kotlin.inputs.ListAdminKeyPlainArgsBuilder
import com.pulumi.azurenative.search.kotlin.inputs.ListQueryKeyBySearchServicePlainArgs
import com.pulumi.azurenative.search.kotlin.inputs.ListQueryKeyBySearchServicePlainArgsBuilder
import com.pulumi.azurenative.search.kotlin.outputs.GetPrivateEndpointConnectionResult
import com.pulumi.azurenative.search.kotlin.outputs.GetServiceResult
import com.pulumi.azurenative.search.kotlin.outputs.GetSharedPrivateLinkResourceResult
import com.pulumi.azurenative.search.kotlin.outputs.ListAdminKeyResult
import com.pulumi.azurenative.search.kotlin.outputs.ListQueryKeyBySearchServiceResult
import kotlinx.coroutines.future.await
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import com.pulumi.azurenative.search.kotlin.outputs.GetPrivateEndpointConnectionResult.Companion.toKotlin as getPrivateEndpointConnectionResultToKotlin
import com.pulumi.azurenative.search.kotlin.outputs.GetServiceResult.Companion.toKotlin as getServiceResultToKotlin
import com.pulumi.azurenative.search.kotlin.outputs.GetSharedPrivateLinkResourceResult.Companion.toKotlin as getSharedPrivateLinkResourceResultToKotlin
import com.pulumi.azurenative.search.kotlin.outputs.ListAdminKeyResult.Companion.toKotlin as listAdminKeyResultToKotlin
import com.pulumi.azurenative.search.kotlin.outputs.ListQueryKeyBySearchServiceResult.Companion.toKotlin as listQueryKeyBySearchServiceResultToKotlin
public object SearchFunctions {
/**
* Gets the details of the private endpoint connection to the search service in the given resource group.
* Azure REST API version: 2022-09-01.
* Other available API versions: 2019-10-01-preview, 2023-11-01, 2024-03-01-preview, 2024-06-01-preview.
* @param argument null
* @return Describes an existing Private Endpoint connection to the Azure Cognitive Search service.
*/
public suspend fun getPrivateEndpointConnection(argument: GetPrivateEndpointConnectionPlainArgs): GetPrivateEndpointConnectionResult =
getPrivateEndpointConnectionResultToKotlin(getPrivateEndpointConnectionPlain(argument.toJava()).await())
/**
* @see [getPrivateEndpointConnection].
* @param privateEndpointConnectionName The name of the private endpoint connection to the Azure Cognitive Search service with the specified resource group.
* @param resourceGroupName The name of the resource group within the current subscription. You can obtain this value from the Azure Resource Manager API or the portal.
* @param searchServiceName The name of the Azure Cognitive Search service associated with the specified resource group.
* @return Describes an existing Private Endpoint connection to the Azure Cognitive Search service.
*/
public suspend fun getPrivateEndpointConnection(
privateEndpointConnectionName: String,
resourceGroupName: String,
searchServiceName: String,
): GetPrivateEndpointConnectionResult {
val argument = GetPrivateEndpointConnectionPlainArgs(
privateEndpointConnectionName = privateEndpointConnectionName,
resourceGroupName = resourceGroupName,
searchServiceName = searchServiceName,
)
return getPrivateEndpointConnectionResultToKotlin(getPrivateEndpointConnectionPlain(argument.toJava()).await())
}
/**
* @see [getPrivateEndpointConnection].
* @param argument Builder for [com.pulumi.azurenative.search.kotlin.inputs.GetPrivateEndpointConnectionPlainArgs].
* @return Describes an existing Private Endpoint connection to the Azure Cognitive Search service.
*/
public suspend fun getPrivateEndpointConnection(argument: suspend GetPrivateEndpointConnectionPlainArgsBuilder.() -> Unit): GetPrivateEndpointConnectionResult {
val builder = GetPrivateEndpointConnectionPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getPrivateEndpointConnectionResultToKotlin(getPrivateEndpointConnectionPlain(builtArgument.toJava()).await())
}
/**
* Gets the search service with the given name in the given resource group.
* Azure REST API version: 2022-09-01.
* Other available API versions: 2021-04-01-preview, 2023-11-01, 2024-03-01-preview, 2024-06-01-preview.
* @param argument null
* @return Describes an Azure Cognitive Search service and its current state.
*/
public suspend fun getService(argument: GetServicePlainArgs): GetServiceResult =
getServiceResultToKotlin(getServicePlain(argument.toJava()).await())
/**
* @see [getService].
* @param resourceGroupName The name of the resource group within the current subscription. You can obtain this value from the Azure Resource Manager API or the portal.
* @param searchServiceName The name of the Azure Cognitive Search service associated with the specified resource group.
* @return Describes an Azure Cognitive Search service and its current state.
*/
public suspend fun getService(resourceGroupName: String, searchServiceName: String): GetServiceResult {
val argument = GetServicePlainArgs(
resourceGroupName = resourceGroupName,
searchServiceName = searchServiceName,
)
return getServiceResultToKotlin(getServicePlain(argument.toJava()).await())
}
/**
* @see [getService].
* @param argument Builder for [com.pulumi.azurenative.search.kotlin.inputs.GetServicePlainArgs].
* @return Describes an Azure Cognitive Search service and its current state.
*/
public suspend fun getService(argument: suspend GetServicePlainArgsBuilder.() -> Unit): GetServiceResult {
val builder = GetServicePlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getServiceResultToKotlin(getServicePlain(builtArgument.toJava()).await())
}
/**
* Gets the details of the shared private link resource managed by the search service in the given resource group.
* Azure REST API version: 2022-09-01.
* Other available API versions: 2023-11-01, 2024-03-01-preview, 2024-06-01-preview.
* @param argument null
* @return Describes a Shared Private Link Resource managed by the Azure Cognitive Search service.
*/
public suspend fun getSharedPrivateLinkResource(argument: GetSharedPrivateLinkResourcePlainArgs): GetSharedPrivateLinkResourceResult =
getSharedPrivateLinkResourceResultToKotlin(getSharedPrivateLinkResourcePlain(argument.toJava()).await())
/**
* @see [getSharedPrivateLinkResource].
* @param resourceGroupName The name of the resource group within the current subscription. You can obtain this value from the Azure Resource Manager API or the portal.
* @param searchServiceName The name of the Azure Cognitive Search service associated with the specified resource group.
* @param sharedPrivateLinkResourceName The name of the shared private link resource managed by the Azure Cognitive Search service within the specified resource group.
* @return Describes a Shared Private Link Resource managed by the Azure Cognitive Search service.
*/
public suspend fun getSharedPrivateLinkResource(
resourceGroupName: String,
searchServiceName: String,
sharedPrivateLinkResourceName: String,
): GetSharedPrivateLinkResourceResult {
val argument = GetSharedPrivateLinkResourcePlainArgs(
resourceGroupName = resourceGroupName,
searchServiceName = searchServiceName,
sharedPrivateLinkResourceName = sharedPrivateLinkResourceName,
)
return getSharedPrivateLinkResourceResultToKotlin(getSharedPrivateLinkResourcePlain(argument.toJava()).await())
}
/**
* @see [getSharedPrivateLinkResource].
* @param argument Builder for [com.pulumi.azurenative.search.kotlin.inputs.GetSharedPrivateLinkResourcePlainArgs].
* @return Describes a Shared Private Link Resource managed by the Azure Cognitive Search service.
*/
public suspend fun getSharedPrivateLinkResource(argument: suspend GetSharedPrivateLinkResourcePlainArgsBuilder.() -> Unit): GetSharedPrivateLinkResourceResult {
val builder = GetSharedPrivateLinkResourcePlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getSharedPrivateLinkResourceResultToKotlin(getSharedPrivateLinkResourcePlain(builtArgument.toJava()).await())
}
/**
* Gets the primary and secondary admin API keys for the specified Azure Cognitive Search service.
* Azure REST API version: 2022-09-01.
* Other available API versions: 2015-02-28, 2021-04-01-preview, 2023-11-01, 2024-03-01-preview, 2024-06-01-preview.
* @param argument null
* @return Response containing the primary and secondary admin API keys for a given Azure Cognitive Search service.
*/
public suspend fun listAdminKey(argument: ListAdminKeyPlainArgs): ListAdminKeyResult =
listAdminKeyResultToKotlin(listAdminKeyPlain(argument.toJava()).await())
/**
* @see [listAdminKey].
* @param resourceGroupName The name of the resource group within the current subscription. You can obtain this value from the Azure Resource Manager API or the portal.
* @param searchServiceName The name of the Azure Cognitive Search service associated with the specified resource group.
* @return Response containing the primary and secondary admin API keys for a given Azure Cognitive Search service.
*/
public suspend fun listAdminKey(resourceGroupName: String, searchServiceName: String): ListAdminKeyResult {
val argument = ListAdminKeyPlainArgs(
resourceGroupName = resourceGroupName,
searchServiceName = searchServiceName,
)
return listAdminKeyResultToKotlin(listAdminKeyPlain(argument.toJava()).await())
}
/**
* @see [listAdminKey].
* @param argument Builder for [com.pulumi.azurenative.search.kotlin.inputs.ListAdminKeyPlainArgs].
* @return Response containing the primary and secondary admin API keys for a given Azure Cognitive Search service.
*/
public suspend fun listAdminKey(argument: suspend ListAdminKeyPlainArgsBuilder.() -> Unit): ListAdminKeyResult {
val builder = ListAdminKeyPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return listAdminKeyResultToKotlin(listAdminKeyPlain(builtArgument.toJava()).await())
}
/**
* Returns the list of query API keys for the given Azure Cognitive Search service.
* Azure REST API version: 2022-09-01.
* Other available API versions: 2021-04-01-preview, 2023-11-01, 2024-03-01-preview, 2024-06-01-preview.
* @param argument null
* @return Response containing the query API keys for a given Azure Cognitive Search service.
*/
public suspend fun listQueryKeyBySearchService(argument: ListQueryKeyBySearchServicePlainArgs): ListQueryKeyBySearchServiceResult =
listQueryKeyBySearchServiceResultToKotlin(listQueryKeyBySearchServicePlain(argument.toJava()).await())
/**
* @see [listQueryKeyBySearchService].
* @param resourceGroupName The name of the resource group within the current subscription. You can obtain this value from the Azure Resource Manager API or the portal.
* @param searchServiceName The name of the Azure Cognitive Search service associated with the specified resource group.
* @return Response containing the query API keys for a given Azure Cognitive Search service.
*/
public suspend fun listQueryKeyBySearchService(
resourceGroupName: String,
searchServiceName: String,
): ListQueryKeyBySearchServiceResult {
val argument = ListQueryKeyBySearchServicePlainArgs(
resourceGroupName = resourceGroupName,
searchServiceName = searchServiceName,
)
return listQueryKeyBySearchServiceResultToKotlin(listQueryKeyBySearchServicePlain(argument.toJava()).await())
}
/**
* @see [listQueryKeyBySearchService].
* @param argument Builder for [com.pulumi.azurenative.search.kotlin.inputs.ListQueryKeyBySearchServicePlainArgs].
* @return Response containing the query API keys for a given Azure Cognitive Search service.
*/
public suspend fun listQueryKeyBySearchService(argument: suspend ListQueryKeyBySearchServicePlainArgsBuilder.() -> Unit): ListQueryKeyBySearchServiceResult {
val builder = ListQueryKeyBySearchServicePlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return listQueryKeyBySearchServiceResultToKotlin(listQueryKeyBySearchServicePlain(builtArgument.toJava()).await())
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy