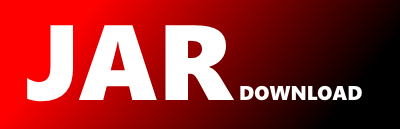
com.pulumi.azurenative.security.kotlin.AssessmentMetadataInSubscriptionArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.security.kotlin
import com.pulumi.azurenative.security.AssessmentMetadataInSubscriptionArgs.builder
import com.pulumi.azurenative.security.kotlin.enums.AssessmentType
import com.pulumi.azurenative.security.kotlin.enums.Categories
import com.pulumi.azurenative.security.kotlin.enums.ImplementationEffort
import com.pulumi.azurenative.security.kotlin.enums.Severity
import com.pulumi.azurenative.security.kotlin.enums.Tactics
import com.pulumi.azurenative.security.kotlin.enums.Techniques
import com.pulumi.azurenative.security.kotlin.enums.Threats
import com.pulumi.azurenative.security.kotlin.enums.UserImpact
import com.pulumi.azurenative.security.kotlin.inputs.SecurityAssessmentMetadataPartnerDataArgs
import com.pulumi.azurenative.security.kotlin.inputs.SecurityAssessmentMetadataPartnerDataArgsBuilder
import com.pulumi.azurenative.security.kotlin.inputs.SecurityAssessmentMetadataPropertiesResponsePublishDatesArgs
import com.pulumi.azurenative.security.kotlin.inputs.SecurityAssessmentMetadataPropertiesResponsePublishDatesArgsBuilder
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Security assessment metadata response
* Azure REST API version: 2021-06-01. Prior API version in Azure Native 1.x: 2020-01-01.
* ## Example Usage
* ### Create security assessment metadata for subscription
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var assessmentMetadataInSubscription = new AzureNative.Security.AssessmentMetadataInSubscription("assessmentMetadataInSubscription", new()
* {
* AssessmentMetadataName = "ca039e75-a276-4175-aebc-bcd41e4b14b7",
* AssessmentType = AzureNative.Security.AssessmentType.CustomerManaged,
* Categories = new[]
* {
* AzureNative.Security.Categories.Compute,
* },
* Description = "Install an endpoint protection solution on your virtual machines scale sets, to protect them from threats and vulnerabilities.",
* DisplayName = "Install endpoint protection solution on virtual machine scale sets",
* ImplementationEffort = AzureNative.Security.ImplementationEffort.Low,
* RemediationDescription = "To install an endpoint protection solution: 1. Follow the instructions in How do I turn on antimalware in my virtual machine scale set",
* Severity = AzureNative.Security.Severity.Medium,
* Threats = new[]
* {
* AzureNative.Security.Threats.DataExfiltration,
* AzureNative.Security.Threats.DataSpillage,
* AzureNative.Security.Threats.MaliciousInsider,
* },
* UserImpact = AzureNative.Security.UserImpact.Low,
* });
* });
* ```
* ```go
* package main
* import (
* security "github.com/pulumi/pulumi-azure-native-sdk/security/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := security.NewAssessmentMetadataInSubscription(ctx, "assessmentMetadataInSubscription", &security.AssessmentMetadataInSubscriptionArgs{
* AssessmentMetadataName: pulumi.String("ca039e75-a276-4175-aebc-bcd41e4b14b7"),
* AssessmentType: pulumi.String(security.AssessmentTypeCustomerManaged),
* Categories: pulumi.StringArray{
* pulumi.String(security.CategoriesCompute),
* },
* Description: pulumi.String("Install an endpoint protection solution on your virtual machines scale sets, to protect them from threats and vulnerabilities."),
* DisplayName: pulumi.String("Install endpoint protection solution on virtual machine scale sets"),
* ImplementationEffort: pulumi.String(security.ImplementationEffortLow),
* RemediationDescription: pulumi.String("To install an endpoint protection solution: 1. Follow the instructions in How do I turn on antimalware in my virtual machine scale set"),
* Severity: pulumi.String(security.SeverityMedium),
* Threats: pulumi.StringArray{
* pulumi.String(security.ThreatsDataExfiltration),
* pulumi.String(security.ThreatsDataSpillage),
* pulumi.String(security.ThreatsMaliciousInsider),
* },
* UserImpact: pulumi.String(security.UserImpactLow),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.security.AssessmentMetadataInSubscription;
* import com.pulumi.azurenative.security.AssessmentMetadataInSubscriptionArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var assessmentMetadataInSubscription = new AssessmentMetadataInSubscription("assessmentMetadataInSubscription", AssessmentMetadataInSubscriptionArgs.builder()
* .assessmentMetadataName("ca039e75-a276-4175-aebc-bcd41e4b14b7")
* .assessmentType("CustomerManaged")
* .categories("Compute")
* .description("Install an endpoint protection solution on your virtual machines scale sets, to protect them from threats and vulnerabilities.")
* .displayName("Install endpoint protection solution on virtual machine scale sets")
* .implementationEffort("Low")
* .remediationDescription("To install an endpoint protection solution: 1. Follow the instructions in How do I turn on antimalware in my virtual machine scale set")
* .severity("Medium")
* .threats(
* "dataExfiltration",
* "dataSpillage",
* "maliciousInsider")
* .userImpact("Low")
* .build());
* }
* }
* ```
* ## Import
* An existing resource can be imported using its type token, name, and identifier, e.g.
* ```sh
* $ pulumi import azure-native:security:AssessmentMetadataInSubscription ca039e75-a276-4175-aebc-bcd41e4b14b7 /subscriptions/{subscriptionId}/providers/Microsoft.Security/assessmentMetadata/{assessmentMetadataName}
* ```
* @property assessmentMetadataName The Assessment Key - Unique key for the assessment type
* @property assessmentType BuiltIn if the assessment based on built-in Azure Policy definition, Custom if the assessment based on custom Azure Policy definition
* @property categories
* @property description Human readable description of the assessment
* @property displayName User friendly display name of the assessment
* @property implementationEffort The implementation effort required to remediate this assessment
* @property partnerData Describes the partner that created the assessment
* @property plannedDeprecationDate
* @property preview True if this assessment is in preview release status
* @property publishDates
* @property remediationDescription Human readable description of what you should do to mitigate this security issue
* @property severity The severity level of the assessment
* @property tactics
* @property techniques
* @property threats
* @property userImpact The user impact of the assessment
*/
public data class AssessmentMetadataInSubscriptionArgs(
public val assessmentMetadataName: Output? = null,
public val assessmentType: Output>? = null,
public val categories: Output>>? = null,
public val description: Output? = null,
public val displayName: Output? = null,
public val implementationEffort: Output>? = null,
public val partnerData: Output? = null,
public val plannedDeprecationDate: Output? = null,
public val preview: Output? = null,
public val publishDates: Output? =
null,
public val remediationDescription: Output? = null,
public val severity: Output>? = null,
public val tactics: Output>>? = null,
public val techniques: Output>>? = null,
public val threats: Output>>? = null,
public val userImpact: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.security.AssessmentMetadataInSubscriptionArgs =
com.pulumi.azurenative.security.AssessmentMetadataInSubscriptionArgs.builder()
.assessmentMetadataName(assessmentMetadataName?.applyValue({ args0 -> args0 }))
.assessmentType(
assessmentType?.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.categories(
categories?.applyValue({ args0 ->
args0.map({ args0 ->
args0.transform(
{ args0 -> args0 },
{ args0 -> args0.let({ args0 -> args0.toJava() }) },
)
})
}),
)
.description(description?.applyValue({ args0 -> args0 }))
.displayName(displayName?.applyValue({ args0 -> args0 }))
.implementationEffort(
implementationEffort?.applyValue({ args0 ->
args0.transform(
{ args0 -> args0 },
{ args0 -> args0.let({ args0 -> args0.toJava() }) },
)
}),
)
.partnerData(partnerData?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.plannedDeprecationDate(plannedDeprecationDate?.applyValue({ args0 -> args0 }))
.preview(preview?.applyValue({ args0 -> args0 }))
.publishDates(publishDates?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.remediationDescription(remediationDescription?.applyValue({ args0 -> args0 }))
.severity(
severity?.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.tactics(
tactics?.applyValue({ args0 ->
args0.map({ args0 ->
args0.transform(
{ args0 -> args0 },
{ args0 -> args0.let({ args0 -> args0.toJava() }) },
)
})
}),
)
.techniques(
techniques?.applyValue({ args0 ->
args0.map({ args0 ->
args0.transform(
{ args0 -> args0 },
{ args0 -> args0.let({ args0 -> args0.toJava() }) },
)
})
}),
)
.threats(
threats?.applyValue({ args0 ->
args0.map({ args0 ->
args0.transform(
{ args0 -> args0 },
{ args0 -> args0.let({ args0 -> args0.toJava() }) },
)
})
}),
)
.userImpact(
userImpact?.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
).build()
}
/**
* Builder for [AssessmentMetadataInSubscriptionArgs].
*/
@PulumiTagMarker
public class AssessmentMetadataInSubscriptionArgsBuilder internal constructor() {
private var assessmentMetadataName: Output? = null
private var assessmentType: Output>? = null
private var categories: Output>>? = null
private var description: Output? = null
private var displayName: Output? = null
private var implementationEffort: Output>? = null
private var partnerData: Output? = null
private var plannedDeprecationDate: Output? = null
private var preview: Output? = null
private var publishDates: Output? =
null
private var remediationDescription: Output? = null
private var severity: Output>? = null
private var tactics: Output>>? = null
private var techniques: Output>>? = null
private var threats: Output>>? = null
private var userImpact: Output>? = null
/**
* @param value The Assessment Key - Unique key for the assessment type
*/
@JvmName("iujtfyiiveodfkmr")
public suspend fun assessmentMetadataName(`value`: Output) {
this.assessmentMetadataName = value
}
/**
* @param value BuiltIn if the assessment based on built-in Azure Policy definition, Custom if the assessment based on custom Azure Policy definition
*/
@JvmName("iwxatvpocmltohvj")
public suspend fun assessmentType(`value`: Output>) {
this.assessmentType = value
}
/**
* @param value
*/
@JvmName("uehghvhbwxeynvya")
public suspend fun categories(`value`: Output>>) {
this.categories = value
}
@JvmName("wdgxjqxdctwvhiar")
public suspend fun categories(vararg values: Output>) {
this.categories = Output.all(values.asList())
}
/**
* @param values
*/
@JvmName("srtlwbueuydbcgya")
public suspend fun categories(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy