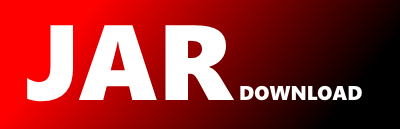
com.pulumi.azurenative.security.kotlin.inputs.DefenderForContainersAwsOfferingArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.security.kotlin.inputs
import com.pulumi.azurenative.security.inputs.DefenderForContainersAwsOfferingArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Double
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* The Defender for Containers AWS offering
* @property autoProvisioning Is audit logs pipeline auto provisioning enabled
* @property cloudWatchToKinesis The cloudwatch to kinesis connection configuration
* @property containerVulnerabilityAssessment The container vulnerability assessment configuration
* @property containerVulnerabilityAssessmentTask The container vulnerability assessment task configuration
* @property enableContainerVulnerabilityAssessment Enable container vulnerability assessment feature
* @property kinesisToS3 The kinesis to s3 connection configuration
* @property kubeAuditRetentionTime The retention time in days of kube audit logs set on the CloudWatch log group
* @property kubernetesScubaReader The kubernetes to scuba connection configuration
* @property kubernetesService The kubernetes service connection configuration
* @property offeringType The type of the security offering.
* Expected value is 'DefenderForContainersAws'.
* @property scubaExternalId The externalId used by the data reader to prevent the confused deputy attack
*/
public data class DefenderForContainersAwsOfferingArgs(
public val autoProvisioning: Output? = null,
public val cloudWatchToKinesis: Output? =
null,
public val containerVulnerabilityAssessment: Output? = null,
public val containerVulnerabilityAssessmentTask: Output? = null,
public val enableContainerVulnerabilityAssessment: Output? = null,
public val kinesisToS3: Output? = null,
public val kubeAuditRetentionTime: Output? = null,
public val kubernetesScubaReader: Output? = null,
public val kubernetesService: Output? =
null,
public val offeringType: Output,
public val scubaExternalId: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.security.inputs.DefenderForContainersAwsOfferingArgs = com.pulumi.azurenative.security.inputs.DefenderForContainersAwsOfferingArgs.builder()
.autoProvisioning(autoProvisioning?.applyValue({ args0 -> args0 }))
.cloudWatchToKinesis(
cloudWatchToKinesis?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.containerVulnerabilityAssessment(
containerVulnerabilityAssessment?.applyValue({ args0 ->
args0.let({ args0 -> args0.toJava() })
}),
)
.containerVulnerabilityAssessmentTask(
containerVulnerabilityAssessmentTask?.applyValue({ args0 ->
args0.let({ args0 -> args0.toJava() })
}),
)
.enableContainerVulnerabilityAssessment(
enableContainerVulnerabilityAssessment?.applyValue({ args0 ->
args0
}),
)
.kinesisToS3(kinesisToS3?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.kubeAuditRetentionTime(kubeAuditRetentionTime?.applyValue({ args0 -> args0 }))
.kubernetesScubaReader(
kubernetesScubaReader?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.kubernetesService(kubernetesService?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.offeringType(offeringType.applyValue({ args0 -> args0 }))
.scubaExternalId(scubaExternalId?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [DefenderForContainersAwsOfferingArgs].
*/
@PulumiTagMarker
public class DefenderForContainersAwsOfferingArgsBuilder internal constructor() {
private var autoProvisioning: Output? = null
private var cloudWatchToKinesis: Output? =
null
private var containerVulnerabilityAssessment:
Output? = null
private var containerVulnerabilityAssessmentTask:
Output? = null
private var enableContainerVulnerabilityAssessment: Output? = null
private var kinesisToS3: Output? = null
private var kubeAuditRetentionTime: Output? = null
private var kubernetesScubaReader:
Output? = null
private var kubernetesService: Output? =
null
private var offeringType: Output? = null
private var scubaExternalId: Output? = null
/**
* @param value Is audit logs pipeline auto provisioning enabled
*/
@JvmName("thrbqvxnndwpdnql")
public suspend fun autoProvisioning(`value`: Output) {
this.autoProvisioning = value
}
/**
* @param value The cloudwatch to kinesis connection configuration
*/
@JvmName("vhhwopgwmonfebbt")
public suspend fun cloudWatchToKinesis(`value`: Output) {
this.cloudWatchToKinesis = value
}
/**
* @param value The container vulnerability assessment configuration
*/
@JvmName("cisqqwohkuupykej")
public suspend fun containerVulnerabilityAssessment(`value`: Output) {
this.containerVulnerabilityAssessment = value
}
/**
* @param value The container vulnerability assessment task configuration
*/
@JvmName("odfegiqwbwluvumf")
public suspend fun containerVulnerabilityAssessmentTask(`value`: Output) {
this.containerVulnerabilityAssessmentTask = value
}
/**
* @param value Enable container vulnerability assessment feature
*/
@JvmName("nvforigtmdxhsgsi")
public suspend fun enableContainerVulnerabilityAssessment(`value`: Output) {
this.enableContainerVulnerabilityAssessment = value
}
/**
* @param value The kinesis to s3 connection configuration
*/
@JvmName("jtxmopvnlewylalj")
public suspend fun kinesisToS3(`value`: Output) {
this.kinesisToS3 = value
}
/**
* @param value The retention time in days of kube audit logs set on the CloudWatch log group
*/
@JvmName("iuapitwldvfripvu")
public suspend fun kubeAuditRetentionTime(`value`: Output) {
this.kubeAuditRetentionTime = value
}
/**
* @param value The kubernetes to scuba connection configuration
*/
@JvmName("hkbfuhbxtrsltobw")
public suspend fun kubernetesScubaReader(`value`: Output) {
this.kubernetesScubaReader = value
}
/**
* @param value The kubernetes service connection configuration
*/
@JvmName("dpcagiccikhgmkao")
public suspend fun kubernetesService(`value`: Output) {
this.kubernetesService = value
}
/**
* @param value The type of the security offering.
* Expected value is 'DefenderForContainersAws'.
*/
@JvmName("sjtkdkffrgxhtatu")
public suspend fun offeringType(`value`: Output) {
this.offeringType = value
}
/**
* @param value The externalId used by the data reader to prevent the confused deputy attack
*/
@JvmName("jeqtbgurpnedjfyc")
public suspend fun scubaExternalId(`value`: Output) {
this.scubaExternalId = value
}
/**
* @param value Is audit logs pipeline auto provisioning enabled
*/
@JvmName("xduyaixmsmtbtlfh")
public suspend fun autoProvisioning(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.autoProvisioning = mapped
}
/**
* @param value The cloudwatch to kinesis connection configuration
*/
@JvmName("caqektrdbvhmetvl")
public suspend fun cloudWatchToKinesis(`value`: DefenderForContainersAwsOfferingCloudWatchToKinesisArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.cloudWatchToKinesis = mapped
}
/**
* @param argument The cloudwatch to kinesis connection configuration
*/
@JvmName("wwugbgqjavtixoru")
public suspend fun cloudWatchToKinesis(argument: suspend DefenderForContainersAwsOfferingCloudWatchToKinesisArgsBuilder.() -> Unit) {
val toBeMapped = DefenderForContainersAwsOfferingCloudWatchToKinesisArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.cloudWatchToKinesis = mapped
}
/**
* @param value The container vulnerability assessment configuration
*/
@JvmName("bwbbshvlkdnhhwnf")
public suspend fun containerVulnerabilityAssessment(`value`: DefenderForContainersAwsOfferingContainerVulnerabilityAssessmentArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.containerVulnerabilityAssessment = mapped
}
/**
* @param argument The container vulnerability assessment configuration
*/
@JvmName("igrqadskbxkbhrdn")
public suspend fun containerVulnerabilityAssessment(argument: suspend DefenderForContainersAwsOfferingContainerVulnerabilityAssessmentArgsBuilder.() -> Unit) {
val toBeMapped =
DefenderForContainersAwsOfferingContainerVulnerabilityAssessmentArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.containerVulnerabilityAssessment = mapped
}
/**
* @param value The container vulnerability assessment task configuration
*/
@JvmName("uxufwerqenoteqcg")
public suspend fun containerVulnerabilityAssessmentTask(`value`: DefenderForContainersAwsOfferingContainerVulnerabilityAssessmentTaskArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.containerVulnerabilityAssessmentTask = mapped
}
/**
* @param argument The container vulnerability assessment task configuration
*/
@JvmName("ikdyavqxyelnpbdi")
public suspend fun containerVulnerabilityAssessmentTask(argument: suspend DefenderForContainersAwsOfferingContainerVulnerabilityAssessmentTaskArgsBuilder.() -> Unit) {
val toBeMapped =
DefenderForContainersAwsOfferingContainerVulnerabilityAssessmentTaskArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.containerVulnerabilityAssessmentTask = mapped
}
/**
* @param value Enable container vulnerability assessment feature
*/
@JvmName("wnahnltyjuulvxrf")
public suspend fun enableContainerVulnerabilityAssessment(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.enableContainerVulnerabilityAssessment = mapped
}
/**
* @param value The kinesis to s3 connection configuration
*/
@JvmName("psxjyfbtjwrvuhfl")
public suspend fun kinesisToS3(`value`: DefenderForContainersAwsOfferingKinesisToS3Args?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.kinesisToS3 = mapped
}
/**
* @param argument The kinesis to s3 connection configuration
*/
@JvmName("payeofkgqjgqcinh")
public suspend fun kinesisToS3(argument: suspend DefenderForContainersAwsOfferingKinesisToS3ArgsBuilder.() -> Unit) {
val toBeMapped = DefenderForContainersAwsOfferingKinesisToS3ArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.kinesisToS3 = mapped
}
/**
* @param value The retention time in days of kube audit logs set on the CloudWatch log group
*/
@JvmName("cfdaqwqniwkmtvki")
public suspend fun kubeAuditRetentionTime(`value`: Double?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.kubeAuditRetentionTime = mapped
}
/**
* @param value The kubernetes to scuba connection configuration
*/
@JvmName("iafbximlytufqydc")
public suspend fun kubernetesScubaReader(`value`: DefenderForContainersAwsOfferingKubernetesScubaReaderArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.kubernetesScubaReader = mapped
}
/**
* @param argument The kubernetes to scuba connection configuration
*/
@JvmName("gfwakuwkdwybtjsb")
public suspend fun kubernetesScubaReader(argument: suspend DefenderForContainersAwsOfferingKubernetesScubaReaderArgsBuilder.() -> Unit) {
val toBeMapped =
DefenderForContainersAwsOfferingKubernetesScubaReaderArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.kubernetesScubaReader = mapped
}
/**
* @param value The kubernetes service connection configuration
*/
@JvmName("fwxtathnirxmuskv")
public suspend fun kubernetesService(`value`: DefenderForContainersAwsOfferingKubernetesServiceArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.kubernetesService = mapped
}
/**
* @param argument The kubernetes service connection configuration
*/
@JvmName("anotsocwvlllyupn")
public suspend fun kubernetesService(argument: suspend DefenderForContainersAwsOfferingKubernetesServiceArgsBuilder.() -> Unit) {
val toBeMapped = DefenderForContainersAwsOfferingKubernetesServiceArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.kubernetesService = mapped
}
/**
* @param value The type of the security offering.
* Expected value is 'DefenderForContainersAws'.
*/
@JvmName("ympsbrfaxiabmqwb")
public suspend fun offeringType(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.offeringType = mapped
}
/**
* @param value The externalId used by the data reader to prevent the confused deputy attack
*/
@JvmName("okayjevupukqtisu")
public suspend fun scubaExternalId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.scubaExternalId = mapped
}
internal fun build(): DefenderForContainersAwsOfferingArgs = DefenderForContainersAwsOfferingArgs(
autoProvisioning = autoProvisioning,
cloudWatchToKinesis = cloudWatchToKinesis,
containerVulnerabilityAssessment = containerVulnerabilityAssessment,
containerVulnerabilityAssessmentTask = containerVulnerabilityAssessmentTask,
enableContainerVulnerabilityAssessment = enableContainerVulnerabilityAssessment,
kinesisToS3 = kinesisToS3,
kubeAuditRetentionTime = kubeAuditRetentionTime,
kubernetesScubaReader = kubernetesScubaReader,
kubernetesService = kubernetesService,
offeringType = offeringType ?: throw PulumiNullFieldException("offeringType"),
scubaExternalId = scubaExternalId,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy