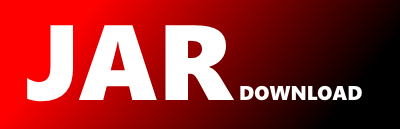
com.pulumi.azurenative.security.kotlin.inputs.GcpCredentialsDetailsPropertiesArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.security.kotlin.inputs
import com.pulumi.azurenative.security.inputs.GcpCredentialsDetailsPropertiesArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* GCP cloud account connector based service to service credentials, the credentials are composed of the organization ID and a JSON API key (write only)
* @property authProviderX509CertUrl Auth provider x509 certificate URL field of the API key (write only)
* @property authUri Auth URI field of the API key (write only)
* @property authenticationType Connect to your cloud account, for AWS use either account credentials or role-based authentication. For GCP use account organization credentials.
* Expected value is 'gcpCredentials'.
* @property clientEmail Client email field of the API key (write only)
* @property clientId Client ID field of the API key (write only)
* @property clientX509CertUrl Client x509 certificate URL field of the API key (write only)
* @property organizationId The organization ID of the GCP cloud account
* @property privateKey Private key field of the API key (write only)
* @property privateKeyId Private key ID field of the API key (write only)
* @property projectId Project ID field of the API key (write only)
* @property tokenUri Token URI field of the API key (write only)
* @property type Type field of the API key (write only)
*/
public data class GcpCredentialsDetailsPropertiesArgs(
public val authProviderX509CertUrl: Output,
public val authUri: Output,
public val authenticationType: Output,
public val clientEmail: Output,
public val clientId: Output,
public val clientX509CertUrl: Output,
public val organizationId: Output,
public val privateKey: Output,
public val privateKeyId: Output,
public val projectId: Output,
public val tokenUri: Output,
public val type: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.security.inputs.GcpCredentialsDetailsPropertiesArgs = com.pulumi.azurenative.security.inputs.GcpCredentialsDetailsPropertiesArgs.builder()
.authProviderX509CertUrl(authProviderX509CertUrl.applyValue({ args0 -> args0 }))
.authUri(authUri.applyValue({ args0 -> args0 }))
.authenticationType(authenticationType.applyValue({ args0 -> args0 }))
.clientEmail(clientEmail.applyValue({ args0 -> args0 }))
.clientId(clientId.applyValue({ args0 -> args0 }))
.clientX509CertUrl(clientX509CertUrl.applyValue({ args0 -> args0 }))
.organizationId(organizationId.applyValue({ args0 -> args0 }))
.privateKey(privateKey.applyValue({ args0 -> args0 }))
.privateKeyId(privateKeyId.applyValue({ args0 -> args0 }))
.projectId(projectId.applyValue({ args0 -> args0 }))
.tokenUri(tokenUri.applyValue({ args0 -> args0 }))
.type(type.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [GcpCredentialsDetailsPropertiesArgs].
*/
@PulumiTagMarker
public class GcpCredentialsDetailsPropertiesArgsBuilder internal constructor() {
private var authProviderX509CertUrl: Output? = null
private var authUri: Output? = null
private var authenticationType: Output? = null
private var clientEmail: Output? = null
private var clientId: Output? = null
private var clientX509CertUrl: Output? = null
private var organizationId: Output? = null
private var privateKey: Output? = null
private var privateKeyId: Output? = null
private var projectId: Output? = null
private var tokenUri: Output? = null
private var type: Output? = null
/**
* @param value Auth provider x509 certificate URL field of the API key (write only)
*/
@JvmName("sjbatolmjqoypfgs")
public suspend fun authProviderX509CertUrl(`value`: Output) {
this.authProviderX509CertUrl = value
}
/**
* @param value Auth URI field of the API key (write only)
*/
@JvmName("ucmcskadobnsivim")
public suspend fun authUri(`value`: Output) {
this.authUri = value
}
/**
* @param value Connect to your cloud account, for AWS use either account credentials or role-based authentication. For GCP use account organization credentials.
* Expected value is 'gcpCredentials'.
*/
@JvmName("bhadvxhfrnmyikxn")
public suspend fun authenticationType(`value`: Output) {
this.authenticationType = value
}
/**
* @param value Client email field of the API key (write only)
*/
@JvmName("vqnuhskgogvipevo")
public suspend fun clientEmail(`value`: Output) {
this.clientEmail = value
}
/**
* @param value Client ID field of the API key (write only)
*/
@JvmName("dwjmsdxmiomvequm")
public suspend fun clientId(`value`: Output) {
this.clientId = value
}
/**
* @param value Client x509 certificate URL field of the API key (write only)
*/
@JvmName("ifvrcgfkencilkxo")
public suspend fun clientX509CertUrl(`value`: Output) {
this.clientX509CertUrl = value
}
/**
* @param value The organization ID of the GCP cloud account
*/
@JvmName("civvrpdmtqnjgfmw")
public suspend fun organizationId(`value`: Output) {
this.organizationId = value
}
/**
* @param value Private key field of the API key (write only)
*/
@JvmName("bosewkvwointhufq")
public suspend fun privateKey(`value`: Output) {
this.privateKey = value
}
/**
* @param value Private key ID field of the API key (write only)
*/
@JvmName("qivonhidgeyydknq")
public suspend fun privateKeyId(`value`: Output) {
this.privateKeyId = value
}
/**
* @param value Project ID field of the API key (write only)
*/
@JvmName("porxthmfidtqbmur")
public suspend fun projectId(`value`: Output) {
this.projectId = value
}
/**
* @param value Token URI field of the API key (write only)
*/
@JvmName("johnbkgechlyogsr")
public suspend fun tokenUri(`value`: Output) {
this.tokenUri = value
}
/**
* @param value Type field of the API key (write only)
*/
@JvmName("xulcihsnhmkakdvr")
public suspend fun type(`value`: Output) {
this.type = value
}
/**
* @param value Auth provider x509 certificate URL field of the API key (write only)
*/
@JvmName("cgiawmlibrlifyfa")
public suspend fun authProviderX509CertUrl(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.authProviderX509CertUrl = mapped
}
/**
* @param value Auth URI field of the API key (write only)
*/
@JvmName("esbumueiofuqdnyg")
public suspend fun authUri(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.authUri = mapped
}
/**
* @param value Connect to your cloud account, for AWS use either account credentials or role-based authentication. For GCP use account organization credentials.
* Expected value is 'gcpCredentials'.
*/
@JvmName("cvovgrbclbclsuht")
public suspend fun authenticationType(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.authenticationType = mapped
}
/**
* @param value Client email field of the API key (write only)
*/
@JvmName("lixqhouxoakbsqls")
public suspend fun clientEmail(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.clientEmail = mapped
}
/**
* @param value Client ID field of the API key (write only)
*/
@JvmName("boydymlljejiljxu")
public suspend fun clientId(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.clientId = mapped
}
/**
* @param value Client x509 certificate URL field of the API key (write only)
*/
@JvmName("hrykbigamcvreuqj")
public suspend fun clientX509CertUrl(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.clientX509CertUrl = mapped
}
/**
* @param value The organization ID of the GCP cloud account
*/
@JvmName("vqjsqioxawvwlqho")
public suspend fun organizationId(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.organizationId = mapped
}
/**
* @param value Private key field of the API key (write only)
*/
@JvmName("fpgpqavnjolgsiyq")
public suspend fun privateKey(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.privateKey = mapped
}
/**
* @param value Private key ID field of the API key (write only)
*/
@JvmName("hvogpdfelhsvhaqf")
public suspend fun privateKeyId(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.privateKeyId = mapped
}
/**
* @param value Project ID field of the API key (write only)
*/
@JvmName("trktkvtqfwefslox")
public suspend fun projectId(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.projectId = mapped
}
/**
* @param value Token URI field of the API key (write only)
*/
@JvmName("alighdxxcrydvdvm")
public suspend fun tokenUri(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.tokenUri = mapped
}
/**
* @param value Type field of the API key (write only)
*/
@JvmName("atgmanrfwsalmfyo")
public suspend fun type(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.type = mapped
}
internal fun build(): GcpCredentialsDetailsPropertiesArgs = GcpCredentialsDetailsPropertiesArgs(
authProviderX509CertUrl = authProviderX509CertUrl ?: throw
PulumiNullFieldException("authProviderX509CertUrl"),
authUri = authUri ?: throw PulumiNullFieldException("authUri"),
authenticationType = authenticationType ?: throw PulumiNullFieldException("authenticationType"),
clientEmail = clientEmail ?: throw PulumiNullFieldException("clientEmail"),
clientId = clientId ?: throw PulumiNullFieldException("clientId"),
clientX509CertUrl = clientX509CertUrl ?: throw PulumiNullFieldException("clientX509CertUrl"),
organizationId = organizationId ?: throw PulumiNullFieldException("organizationId"),
privateKey = privateKey ?: throw PulumiNullFieldException("privateKey"),
privateKeyId = privateKeyId ?: throw PulumiNullFieldException("privateKeyId"),
projectId = projectId ?: throw PulumiNullFieldException("projectId"),
tokenUri = tokenUri ?: throw PulumiNullFieldException("tokenUri"),
type = type ?: throw PulumiNullFieldException("type"),
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy