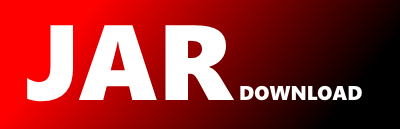
com.pulumi.azurenative.securityinsights.kotlin.MicrosoftSecurityIncidentCreationAlertRuleArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.securityinsights.kotlin
import com.pulumi.azurenative.securityinsights.MicrosoftSecurityIncidentCreationAlertRuleArgs.builder
import com.pulumi.azurenative.securityinsights.kotlin.enums.AlertSeverity
import com.pulumi.azurenative.securityinsights.kotlin.enums.MicrosoftSecurityProductName
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Represents MicrosoftSecurityIncidentCreation rule.
* Azure REST API version: 2023-02-01. Prior API version in Azure Native 1.x: 2020-01-01.
* ## Example Usage
* ### Creates or updates a Fusion alert rule.
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var microsoftSecurityIncidentCreationAlertRule = new AzureNative.SecurityInsights.MicrosoftSecurityIncidentCreationAlertRule("microsoftSecurityIncidentCreationAlertRule", new()
* {
* ResourceGroupName = "myRg",
* RuleId = "myFirstFusionRule",
* WorkspaceName = "myWorkspace",
* });
* });
* ```
* ```go
* package main
* import (
* securityinsights "github.com/pulumi/pulumi-azure-native-sdk/securityinsights/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := securityinsights.NewMicrosoftSecurityIncidentCreationAlertRule(ctx, "microsoftSecurityIncidentCreationAlertRule", &securityinsights.MicrosoftSecurityIncidentCreationAlertRuleArgs{
* ResourceGroupName: pulumi.String("myRg"),
* RuleId: pulumi.String("myFirstFusionRule"),
* WorkspaceName: pulumi.String("myWorkspace"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.securityinsights.MicrosoftSecurityIncidentCreationAlertRule;
* import com.pulumi.azurenative.securityinsights.MicrosoftSecurityIncidentCreationAlertRuleArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var microsoftSecurityIncidentCreationAlertRule = new MicrosoftSecurityIncidentCreationAlertRule("microsoftSecurityIncidentCreationAlertRule", MicrosoftSecurityIncidentCreationAlertRuleArgs.builder()
* .resourceGroupName("myRg")
* .ruleId("myFirstFusionRule")
* .workspaceName("myWorkspace")
* .build());
* }
* }
* ```
* ### Creates or updates a MicrosoftSecurityIncidentCreation rule.
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var microsoftSecurityIncidentCreationAlertRule = new AzureNative.SecurityInsights.MicrosoftSecurityIncidentCreationAlertRule("microsoftSecurityIncidentCreationAlertRule", new()
* {
* DisplayName = "testing displayname",
* Enabled = true,
* Kind = "MicrosoftSecurityIncidentCreation",
* ProductFilter = AzureNative.SecurityInsights.MicrosoftSecurityProductName.Microsoft_Cloud_App_Security,
* ResourceGroupName = "myRg",
* RuleId = "microsoftSecurityIncidentCreationRuleExample",
* WorkspaceName = "myWorkspace",
* });
* });
* ```
* ```go
* package main
* import (
* securityinsights "github.com/pulumi/pulumi-azure-native-sdk/securityinsights/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := securityinsights.NewMicrosoftSecurityIncidentCreationAlertRule(ctx, "microsoftSecurityIncidentCreationAlertRule", &securityinsights.MicrosoftSecurityIncidentCreationAlertRuleArgs{
* DisplayName: pulumi.String("testing displayname"),
* Enabled: pulumi.Bool(true),
* Kind: pulumi.String("MicrosoftSecurityIncidentCreation"),
* ProductFilter: pulumi.String(securityinsights.MicrosoftSecurityProductName_Microsoft_Cloud_App_Security),
* ResourceGroupName: pulumi.String("myRg"),
* RuleId: pulumi.String("microsoftSecurityIncidentCreationRuleExample"),
* WorkspaceName: pulumi.String("myWorkspace"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.securityinsights.MicrosoftSecurityIncidentCreationAlertRule;
* import com.pulumi.azurenative.securityinsights.MicrosoftSecurityIncidentCreationAlertRuleArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var microsoftSecurityIncidentCreationAlertRule = new MicrosoftSecurityIncidentCreationAlertRule("microsoftSecurityIncidentCreationAlertRule", MicrosoftSecurityIncidentCreationAlertRuleArgs.builder()
* .displayName("testing displayname")
* .enabled(true)
* .kind("MicrosoftSecurityIncidentCreation")
* .productFilter("Microsoft Cloud App Security")
* .resourceGroupName("myRg")
* .ruleId("microsoftSecurityIncidentCreationRuleExample")
* .workspaceName("myWorkspace")
* .build());
* }
* }
* ```
* ### Creates or updates a Scheduled alert rule.
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var microsoftSecurityIncidentCreationAlertRule = new AzureNative.SecurityInsights.MicrosoftSecurityIncidentCreationAlertRule("microsoftSecurityIncidentCreationAlertRule", new()
* {
* ResourceGroupName = "myRg",
* RuleId = "73e01a99-5cd7-4139-a149-9f2736ff2ab5",
* WorkspaceName = "myWorkspace",
* });
* });
* ```
* ```go
* package main
* import (
* securityinsights "github.com/pulumi/pulumi-azure-native-sdk/securityinsights/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := securityinsights.NewMicrosoftSecurityIncidentCreationAlertRule(ctx, "microsoftSecurityIncidentCreationAlertRule", &securityinsights.MicrosoftSecurityIncidentCreationAlertRuleArgs{
* ResourceGroupName: pulumi.String("myRg"),
* RuleId: pulumi.String("73e01a99-5cd7-4139-a149-9f2736ff2ab5"),
* WorkspaceName: pulumi.String("myWorkspace"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.securityinsights.MicrosoftSecurityIncidentCreationAlertRule;
* import com.pulumi.azurenative.securityinsights.MicrosoftSecurityIncidentCreationAlertRuleArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var microsoftSecurityIncidentCreationAlertRule = new MicrosoftSecurityIncidentCreationAlertRule("microsoftSecurityIncidentCreationAlertRule", MicrosoftSecurityIncidentCreationAlertRuleArgs.builder()
* .resourceGroupName("myRg")
* .ruleId("73e01a99-5cd7-4139-a149-9f2736ff2ab5")
* .workspaceName("myWorkspace")
* .build());
* }
* }
* ```
* ## Import
* An existing resource can be imported using its type token, name, and identifier, e.g.
* ```sh
* $ pulumi import azure-native:securityinsights:MicrosoftSecurityIncidentCreationAlertRule 73e01a99-5cd7-4139-a149-9f2736ff2ab5 /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.OperationalInsights/workspaces/{workspaceName}/providers/Microsoft.SecurityInsights/alertRules/{ruleId}
* ```
* @property alertRuleTemplateName The Name of the alert rule template used to create this rule.
* @property description The description of the alert rule.
* @property displayName The display name for alerts created by this alert rule.
* @property displayNamesExcludeFilter the alerts' displayNames on which the cases will not be generated
* @property displayNamesFilter the alerts' displayNames on which the cases will be generated
* @property enabled Determines whether this alert rule is enabled or disabled.
* @property kind The kind of the alert rule
* Expected value is 'MicrosoftSecurityIncidentCreation'.
* @property productFilter The alerts' productName on which the cases will be generated
* @property resourceGroupName The name of the resource group. The name is case insensitive.
* @property ruleId Alert rule ID
* @property severitiesFilter the alerts' severities on which the cases will be generated
* @property workspaceName The name of the workspace.
*/
public data class MicrosoftSecurityIncidentCreationAlertRuleArgs(
public val alertRuleTemplateName: Output? = null,
public val description: Output? = null,
public val displayName: Output? = null,
public val displayNamesExcludeFilter: Output>? = null,
public val displayNamesFilter: Output>? = null,
public val enabled: Output? = null,
public val kind: Output? = null,
public val productFilter: Output>? = null,
public val resourceGroupName: Output? = null,
public val ruleId: Output? = null,
public val severitiesFilter: Output>>? = null,
public val workspaceName: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.securityinsights.MicrosoftSecurityIncidentCreationAlertRuleArgs =
com.pulumi.azurenative.securityinsights.MicrosoftSecurityIncidentCreationAlertRuleArgs.builder()
.alertRuleTemplateName(alertRuleTemplateName?.applyValue({ args0 -> args0 }))
.description(description?.applyValue({ args0 -> args0 }))
.displayName(displayName?.applyValue({ args0 -> args0 }))
.displayNamesExcludeFilter(
displayNamesExcludeFilter?.applyValue({ args0 ->
args0.map({ args0 ->
args0
})
}),
)
.displayNamesFilter(displayNamesFilter?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.enabled(enabled?.applyValue({ args0 -> args0 }))
.kind(kind?.applyValue({ args0 -> args0 }))
.productFilter(
productFilter?.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.resourceGroupName(resourceGroupName?.applyValue({ args0 -> args0 }))
.ruleId(ruleId?.applyValue({ args0 -> args0 }))
.severitiesFilter(
severitiesFilter?.applyValue({ args0 ->
args0.map({ args0 ->
args0.transform({ args0 -> args0 }, { args0 -> args0.let({ args0 -> args0.toJava() }) })
})
}),
)
.workspaceName(workspaceName?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [MicrosoftSecurityIncidentCreationAlertRuleArgs].
*/
@PulumiTagMarker
public class MicrosoftSecurityIncidentCreationAlertRuleArgsBuilder internal constructor() {
private var alertRuleTemplateName: Output? = null
private var description: Output? = null
private var displayName: Output? = null
private var displayNamesExcludeFilter: Output>? = null
private var displayNamesFilter: Output>? = null
private var enabled: Output? = null
private var kind: Output? = null
private var productFilter: Output>? = null
private var resourceGroupName: Output? = null
private var ruleId: Output? = null
private var severitiesFilter: Output>>? = null
private var workspaceName: Output? = null
/**
* @param value The Name of the alert rule template used to create this rule.
*/
@JvmName("ofsjuoqcstsfsaoq")
public suspend fun alertRuleTemplateName(`value`: Output) {
this.alertRuleTemplateName = value
}
/**
* @param value The description of the alert rule.
*/
@JvmName("pgtqukmlnliyixsc")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value The display name for alerts created by this alert rule.
*/
@JvmName("ixerwrkoqxslqprg")
public suspend fun displayName(`value`: Output) {
this.displayName = value
}
/**
* @param value the alerts' displayNames on which the cases will not be generated
*/
@JvmName("cautkbdmjkryakxd")
public suspend fun displayNamesExcludeFilter(`value`: Output>) {
this.displayNamesExcludeFilter = value
}
@JvmName("xxpmjoxevpytuict")
public suspend fun displayNamesExcludeFilter(vararg values: Output) {
this.displayNamesExcludeFilter = Output.all(values.asList())
}
/**
* @param values the alerts' displayNames on which the cases will not be generated
*/
@JvmName("yjmgtppibcwkwxti")
public suspend fun displayNamesExcludeFilter(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy