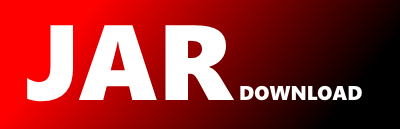
com.pulumi.azurenative.securityinsights.kotlin.ScheduledAlertRule.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.securityinsights.kotlin
import com.pulumi.azurenative.securityinsights.kotlin.outputs.AlertDetailsOverrideResponse
import com.pulumi.azurenative.securityinsights.kotlin.outputs.EntityMappingResponse
import com.pulumi.azurenative.securityinsights.kotlin.outputs.EventGroupingSettingsResponse
import com.pulumi.azurenative.securityinsights.kotlin.outputs.IncidentConfigurationResponse
import com.pulumi.azurenative.securityinsights.kotlin.outputs.SystemDataResponse
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import com.pulumi.azurenative.securityinsights.kotlin.outputs.AlertDetailsOverrideResponse.Companion.toKotlin as alertDetailsOverrideResponseToKotlin
import com.pulumi.azurenative.securityinsights.kotlin.outputs.EntityMappingResponse.Companion.toKotlin as entityMappingResponseToKotlin
import com.pulumi.azurenative.securityinsights.kotlin.outputs.EventGroupingSettingsResponse.Companion.toKotlin as eventGroupingSettingsResponseToKotlin
import com.pulumi.azurenative.securityinsights.kotlin.outputs.IncidentConfigurationResponse.Companion.toKotlin as incidentConfigurationResponseToKotlin
import com.pulumi.azurenative.securityinsights.kotlin.outputs.SystemDataResponse.Companion.toKotlin as systemDataResponseToKotlin
/**
* Builder for [ScheduledAlertRule].
*/
@PulumiTagMarker
public class ScheduledAlertRuleResourceBuilder internal constructor() {
public var name: String? = null
public var args: ScheduledAlertRuleArgs = ScheduledAlertRuleArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend ScheduledAlertRuleArgsBuilder.() -> Unit) {
val builder = ScheduledAlertRuleArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): ScheduledAlertRule {
val builtJavaResource =
com.pulumi.azurenative.securityinsights.ScheduledAlertRule(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return ScheduledAlertRule(builtJavaResource)
}
}
/**
* Represents scheduled alert rule.
* Azure REST API version: 2023-02-01. Prior API version in Azure Native 1.x: 2020-01-01.
* ## Example Usage
* ### Creates or updates a Fusion alert rule.
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var scheduledAlertRule = new AzureNative.SecurityInsights.ScheduledAlertRule("scheduledAlertRule", new()
* {
* ResourceGroupName = "myRg",
* RuleId = "myFirstFusionRule",
* WorkspaceName = "myWorkspace",
* });
* });
* ```
* ```go
* package main
* import (
* securityinsights "github.com/pulumi/pulumi-azure-native-sdk/securityinsights/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := securityinsights.NewScheduledAlertRule(ctx, "scheduledAlertRule", &securityinsights.ScheduledAlertRuleArgs{
* ResourceGroupName: pulumi.String("myRg"),
* RuleId: pulumi.String("myFirstFusionRule"),
* WorkspaceName: pulumi.String("myWorkspace"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.securityinsights.ScheduledAlertRule;
* import com.pulumi.azurenative.securityinsights.ScheduledAlertRuleArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var scheduledAlertRule = new ScheduledAlertRule("scheduledAlertRule", ScheduledAlertRuleArgs.builder()
* .resourceGroupName("myRg")
* .ruleId("myFirstFusionRule")
* .workspaceName("myWorkspace")
* .build());
* }
* }
* ```
* ### Creates or updates a MicrosoftSecurityIncidentCreation rule.
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var scheduledAlertRule = new AzureNative.SecurityInsights.ScheduledAlertRule("scheduledAlertRule", new()
* {
* ResourceGroupName = "myRg",
* RuleId = "microsoftSecurityIncidentCreationRuleExample",
* WorkspaceName = "myWorkspace",
* });
* });
* ```
* ```go
* package main
* import (
* securityinsights "github.com/pulumi/pulumi-azure-native-sdk/securityinsights/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := securityinsights.NewScheduledAlertRule(ctx, "scheduledAlertRule", &securityinsights.ScheduledAlertRuleArgs{
* ResourceGroupName: pulumi.String("myRg"),
* RuleId: pulumi.String("microsoftSecurityIncidentCreationRuleExample"),
* WorkspaceName: pulumi.String("myWorkspace"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.securityinsights.ScheduledAlertRule;
* import com.pulumi.azurenative.securityinsights.ScheduledAlertRuleArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var scheduledAlertRule = new ScheduledAlertRule("scheduledAlertRule", ScheduledAlertRuleArgs.builder()
* .resourceGroupName("myRg")
* .ruleId("microsoftSecurityIncidentCreationRuleExample")
* .workspaceName("myWorkspace")
* .build());
* }
* }
* ```
* ### Creates or updates a Scheduled alert rule.
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var scheduledAlertRule = new AzureNative.SecurityInsights.ScheduledAlertRule("scheduledAlertRule", new()
* {
* AlertDetailsOverride = new AzureNative.SecurityInsights.Inputs.AlertDetailsOverrideArgs
* {
* AlertDescriptionFormat = "Suspicious activity was made by {{ComputerIP}}",
* AlertDisplayNameFormat = "Alert from {{Computer}}",
* AlertDynamicProperties = new[]
* {
* new AzureNative.SecurityInsights.Inputs.AlertPropertyMappingArgs
* {
* AlertProperty = AzureNative.SecurityInsights.AlertProperty.ProductComponentName,
* Value = "ProductComponentNameCustomColumn",
* },
* new AzureNative.SecurityInsights.Inputs.AlertPropertyMappingArgs
* {
* AlertProperty = AzureNative.SecurityInsights.AlertProperty.ProductName,
* Value = "ProductNameCustomColumn",
* },
* new AzureNative.SecurityInsights.Inputs.AlertPropertyMappingArgs
* {
* AlertProperty = AzureNative.SecurityInsights.AlertProperty.AlertLink,
* Value = "Link",
* },
* },
* },
* CustomDetails =
* {
* { "OperatingSystemName", "OSName" },
* { "OperatingSystemType", "OSType" },
* },
* Description = "An example for a scheduled rule",
* DisplayName = "My scheduled rule",
* Enabled = true,
* EntityMappings = new[]
* {
* new AzureNative.SecurityInsights.Inputs.EntityMappingArgs
* {
* EntityType = AzureNative.SecurityInsights.EntityMappingType.Host,
* FieldMappings = new[]
* {
* new AzureNative.SecurityInsights.Inputs.FieldMappingArgs
* {
* ColumnName = "Computer",
* Identifier = "FullName",
* },
* },
* },
* new AzureNative.SecurityInsights.Inputs.EntityMappingArgs
* {
* EntityType = AzureNative.SecurityInsights.EntityMappingType.IP,
* FieldMappings = new[]
* {
* new AzureNative.SecurityInsights.Inputs.FieldMappingArgs
* {
* ColumnName = "ComputerIP",
* Identifier = "Address",
* },
* },
* },
* },
* EventGroupingSettings = new AzureNative.SecurityInsights.Inputs.EventGroupingSettingsArgs
* {
* AggregationKind = AzureNative.SecurityInsights.EventGroupingAggregationKind.AlertPerResult,
* },
* IncidentConfiguration = new AzureNative.SecurityInsights.Inputs.IncidentConfigurationArgs
* {
* CreateIncident = true,
* GroupingConfiguration = new AzureNative.SecurityInsights.Inputs.GroupingConfigurationArgs
* {
* Enabled = true,
* GroupByAlertDetails = new[]
* {
* AzureNative.SecurityInsights.AlertDetail.DisplayName,
* },
* GroupByCustomDetails = new[]
* {
* "OperatingSystemType",
* "OperatingSystemName",
* },
* GroupByEntities = new[]
* {
* AzureNative.SecurityInsights.EntityMappingType.Host,
* },
* LookbackDuration = "PT5H",
* MatchingMethod = AzureNative.SecurityInsights.MatchingMethod.Selected,
* ReopenClosedIncident = false,
* },
* },
* Kind = "Scheduled",
* Query = "Heartbeat",
* QueryFrequency = "PT1H",
* QueryPeriod = "P2DT1H30M",
* ResourceGroupName = "myRg",
* RuleId = "73e01a99-5cd7-4139-a149-9f2736ff2ab5",
* Severity = AzureNative.SecurityInsights.AlertSeverity.High,
* SuppressionDuration = "PT1H",
* SuppressionEnabled = false,
* Tactics = new[]
* {
* AzureNative.SecurityInsights.AttackTactic.Persistence,
* AzureNative.SecurityInsights.AttackTactic.LateralMovement,
* },
* TriggerOperator = AzureNative.SecurityInsights.TriggerOperator.GreaterThan,
* TriggerThreshold = 0,
* WorkspaceName = "myWorkspace",
* });
* });
* ```
* ```go
* package main
* import (
* securityinsights "github.com/pulumi/pulumi-azure-native-sdk/securityinsights/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := securityinsights.NewScheduledAlertRule(ctx, "scheduledAlertRule", &securityinsights.ScheduledAlertRuleArgs{
* AlertDetailsOverride: &securityinsights.AlertDetailsOverrideArgs{
* AlertDescriptionFormat: pulumi.String("Suspicious activity was made by {{ComputerIP}}"),
* AlertDisplayNameFormat: pulumi.String("Alert from {{Computer}}"),
* AlertDynamicProperties: securityinsights.AlertPropertyMappingArray{
* &securityinsights.AlertPropertyMappingArgs{
* AlertProperty: pulumi.String(securityinsights.AlertPropertyProductComponentName),
* Value: pulumi.String("ProductComponentNameCustomColumn"),
* },
* &securityinsights.AlertPropertyMappingArgs{
* AlertProperty: pulumi.String(securityinsights.AlertPropertyProductName),
* Value: pulumi.String("ProductNameCustomColumn"),
* },
* &securityinsights.AlertPropertyMappingArgs{
* AlertProperty: pulumi.String(securityinsights.AlertPropertyAlertLink),
* Value: pulumi.String("Link"),
* },
* },
* },
* CustomDetails: pulumi.StringMap{
* "OperatingSystemName": pulumi.String("OSName"),
* "OperatingSystemType": pulumi.String("OSType"),
* },
* Description: pulumi.String("An example for a scheduled rule"),
* DisplayName: pulumi.String("My scheduled rule"),
* Enabled: pulumi.Bool(true),
* EntityMappings: securityinsights.EntityMappingArray{
* &securityinsights.EntityMappingArgs{
* EntityType: pulumi.String(securityinsights.EntityMappingTypeHost),
* FieldMappings: securityinsights.FieldMappingArray{
* &securityinsights.FieldMappingArgs{
* ColumnName: pulumi.String("Computer"),
* Identifier: pulumi.String("FullName"),
* },
* },
* },
* &securityinsights.EntityMappingArgs{
* EntityType: pulumi.String(securityinsights.EntityMappingTypeIP),
* FieldMappings: securityinsights.FieldMappingArray{
* &securityinsights.FieldMappingArgs{
* ColumnName: pulumi.String("ComputerIP"),
* Identifier: pulumi.String("Address"),
* },
* },
* },
* },
* EventGroupingSettings: &securityinsights.EventGroupingSettingsArgs{
* AggregationKind: pulumi.String(securityinsights.EventGroupingAggregationKindAlertPerResult),
* },
* IncidentConfiguration: &securityinsights.IncidentConfigurationArgs{
* CreateIncident: pulumi.Bool(true),
* GroupingConfiguration: &securityinsights.GroupingConfigurationArgs{
* Enabled: pulumi.Bool(true),
* GroupByAlertDetails: pulumi.StringArray{
* pulumi.String(securityinsights.AlertDetailDisplayName),
* },
* GroupByCustomDetails: pulumi.StringArray{
* pulumi.String("OperatingSystemType"),
* pulumi.String("OperatingSystemName"),
* },
* GroupByEntities: pulumi.StringArray{
* pulumi.String(securityinsights.EntityMappingTypeHost),
* },
* LookbackDuration: pulumi.String("PT5H"),
* MatchingMethod: pulumi.String(securityinsights.MatchingMethodSelected),
* ReopenClosedIncident: pulumi.Bool(false),
* },
* },
* Kind: pulumi.String("Scheduled"),
* Query: pulumi.String("Heartbeat"),
* QueryFrequency: pulumi.String("PT1H"),
* QueryPeriod: pulumi.String("P2DT1H30M"),
* ResourceGroupName: pulumi.String("myRg"),
* RuleId: pulumi.String("73e01a99-5cd7-4139-a149-9f2736ff2ab5"),
* Severity: pulumi.String(securityinsights.AlertSeverityHigh),
* SuppressionDuration: pulumi.String("PT1H"),
* SuppressionEnabled: pulumi.Bool(false),
* Tactics: pulumi.StringArray{
* pulumi.String(securityinsights.AttackTacticPersistence),
* pulumi.String(securityinsights.AttackTacticLateralMovement),
* },
* TriggerOperator: securityinsights.TriggerOperatorGreaterThan,
* TriggerThreshold: pulumi.Int(0),
* WorkspaceName: pulumi.String("myWorkspace"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.securityinsights.ScheduledAlertRule;
* import com.pulumi.azurenative.securityinsights.ScheduledAlertRuleArgs;
* import com.pulumi.azurenative.securityinsights.inputs.AlertDetailsOverrideArgs;
* import com.pulumi.azurenative.securityinsights.inputs.EntityMappingArgs;
* import com.pulumi.azurenative.securityinsights.inputs.EventGroupingSettingsArgs;
* import com.pulumi.azurenative.securityinsights.inputs.IncidentConfigurationArgs;
* import com.pulumi.azurenative.securityinsights.inputs.GroupingConfigurationArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var scheduledAlertRule = new ScheduledAlertRule("scheduledAlertRule", ScheduledAlertRuleArgs.builder()
* .alertDetailsOverride(AlertDetailsOverrideArgs.builder()
* .alertDescriptionFormat("Suspicious activity was made by {{ComputerIP}}")
* .alertDisplayNameFormat("Alert from {{Computer}}")
* .alertDynamicProperties(
* AlertPropertyMappingArgs.builder()
* .alertProperty("ProductComponentName")
* .value("ProductComponentNameCustomColumn")
* .build(),
* AlertPropertyMappingArgs.builder()
* .alertProperty("ProductName")
* .value("ProductNameCustomColumn")
* .build(),
* AlertPropertyMappingArgs.builder()
* .alertProperty("AlertLink")
* .value("Link")
* .build())
* .build())
* .customDetails(Map.ofEntries(
* Map.entry("OperatingSystemName", "OSName"),
* Map.entry("OperatingSystemType", "OSType")
* ))
* .description("An example for a scheduled rule")
* .displayName("My scheduled rule")
* .enabled(true)
* .entityMappings(
* EntityMappingArgs.builder()
* .entityType("Host")
* .fieldMappings(FieldMappingArgs.builder()
* .columnName("Computer")
* .identifier("FullName")
* .build())
* .build(),
* EntityMappingArgs.builder()
* .entityType("IP")
* .fieldMappings(FieldMappingArgs.builder()
* .columnName("ComputerIP")
* .identifier("Address")
* .build())
* .build())
* .eventGroupingSettings(EventGroupingSettingsArgs.builder()
* .aggregationKind("AlertPerResult")
* .build())
* .incidentConfiguration(IncidentConfigurationArgs.builder()
* .createIncident(true)
* .groupingConfiguration(GroupingConfigurationArgs.builder()
* .enabled(true)
* .groupByAlertDetails("DisplayName")
* .groupByCustomDetails(
* "OperatingSystemType",
* "OperatingSystemName")
* .groupByEntities("Host")
* .lookbackDuration("PT5H")
* .matchingMethod("Selected")
* .reopenClosedIncident(false)
* .build())
* .build())
* .kind("Scheduled")
* .query("Heartbeat")
* .queryFrequency("PT1H")
* .queryPeriod("P2DT1H30M")
* .resourceGroupName("myRg")
* .ruleId("73e01a99-5cd7-4139-a149-9f2736ff2ab5")
* .severity("High")
* .suppressionDuration("PT1H")
* .suppressionEnabled(false)
* .tactics(
* "Persistence",
* "LateralMovement")
* .triggerOperator("GreaterThan")
* .triggerThreshold(0)
* .workspaceName("myWorkspace")
* .build());
* }
* }
* ```
* ## Import
* An existing resource can be imported using its type token, name, and identifier, e.g.
* ```sh
* $ pulumi import azure-native:securityinsights:ScheduledAlertRule 73e01a99-5cd7-4139-a149-9f2736ff2ab5 /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.OperationalInsights/workspaces/{workspaceName}/providers/Microsoft.SecurityInsights/alertRules/{ruleId}
* ```
*/
public class ScheduledAlertRule internal constructor(
override val javaResource: com.pulumi.azurenative.securityinsights.ScheduledAlertRule,
) : KotlinCustomResource(javaResource, ScheduledAlertRuleMapper) {
/**
* The alert details override settings
*/
public val alertDetailsOverride: Output?
get() = javaResource.alertDetailsOverride().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> alertDetailsOverrideResponseToKotlin(args0) })
}).orElse(null)
})
/**
* The Name of the alert rule template used to create this rule.
*/
public val alertRuleTemplateName: Output?
get() = javaResource.alertRuleTemplateName().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Dictionary of string key-value pairs of columns to be attached to the alert
*/
public val customDetails: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy