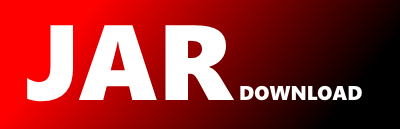
com.pulumi.azurenative.securityinsights.kotlin.WatchlistItemArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.securityinsights.kotlin
import com.pulumi.azurenative.securityinsights.WatchlistItemArgs.builder
import com.pulumi.azurenative.securityinsights.kotlin.inputs.WatchlistUserInfoArgs
import com.pulumi.azurenative.securityinsights.kotlin.inputs.WatchlistUserInfoArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Any
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Represents a Watchlist Item in Azure Security Insights.
* Azure REST API version: 2023-02-01. Prior API version in Azure Native 1.x: 2021-03-01-preview.
* Other available API versions: 2021-04-01, 2023-06-01-preview, 2023-07-01-preview, 2023-08-01-preview, 2023-09-01-preview, 2023-10-01-preview, 2023-11-01, 2023-12-01-preview, 2024-01-01-preview, 2024-03-01.
* ## Example Usage
* ### Create or update a watchlist item.
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var watchlistItem = new AzureNative.SecurityInsights.WatchlistItem("watchlistItem", new()
* {
* ItemsKeyValue = new Dictionary
* {
* ["Business tier"] = "10.0.2.0/24",
* ["Data tier"] = "10.0.2.0/24",
* ["Gateway subnet"] = "10.0.255.224/27",
* ["Private DMZ in"] = "10.0.0.0/27",
* ["Public DMZ out"] = "10.0.0.96/27",
* ["Web Tier"] = "10.0.1.0/24",
* },
* ResourceGroupName = "myRg",
* WatchlistAlias = "highValueAsset",
* WatchlistItemId = "82ba292c-dc97-4dfc-969d-d4dd9e666842",
* WorkspaceName = "myWorkspace",
* });
* });
* ```
* ```go
* package main
* import (
* securityinsights "github.com/pulumi/pulumi-azure-native-sdk/securityinsights/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := securityinsights.NewWatchlistItem(ctx, "watchlistItem", &securityinsights.WatchlistItemArgs{
* ItemsKeyValue: pulumi.Any(map[string]interface{}{
* "Business tier": "10.0.2.0/24",
* "Data tier": "10.0.2.0/24",
* "Gateway subnet": "10.0.255.224/27",
* "Private DMZ in": "10.0.0.0/27",
* "Public DMZ out": "10.0.0.96/27",
* "Web Tier": "10.0.1.0/24",
* }),
* ResourceGroupName: pulumi.String("myRg"),
* WatchlistAlias: pulumi.String("highValueAsset"),
* WatchlistItemId: pulumi.String("82ba292c-dc97-4dfc-969d-d4dd9e666842"),
* WorkspaceName: pulumi.String("myWorkspace"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.securityinsights.WatchlistItem;
* import com.pulumi.azurenative.securityinsights.WatchlistItemArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var watchlistItem = new WatchlistItem("watchlistItem", WatchlistItemArgs.builder()
* .itemsKeyValue(Map.ofEntries(
* Map.entry("Business tier", "10.0.2.0/24"),
* Map.entry("Data tier", "10.0.2.0/24"),
* Map.entry("Gateway subnet", "10.0.255.224/27"),
* Map.entry("Private DMZ in", "10.0.0.0/27"),
* Map.entry("Public DMZ out", "10.0.0.96/27"),
* Map.entry("Web Tier", "10.0.1.0/24")
* ))
* .resourceGroupName("myRg")
* .watchlistAlias("highValueAsset")
* .watchlistItemId("82ba292c-dc97-4dfc-969d-d4dd9e666842")
* .workspaceName("myWorkspace")
* .build());
* }
* }
* ```
* ## Import
* An existing resource can be imported using its type token, name, and identifier, e.g.
* ```sh
* $ pulumi import azure-native:securityinsights:WatchlistItem myresource1 /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.OperationalInsights/workspaces/{workspaceName}/providers/Microsoft.SecurityInsights/watchlists/{watchlistAlias}/watchlistItems/{watchlistItemId}
* ```
* @property created The time the watchlist item was created
* @property createdBy Describes a user that created the watchlist item
* @property entityMapping key-value pairs for a watchlist item entity mapping
* @property isDeleted A flag that indicates if the watchlist item is deleted or not
* @property itemsKeyValue key-value pairs for a watchlist item
* @property resourceGroupName The name of the resource group. The name is case insensitive.
* @property tenantId The tenantId to which the watchlist item belongs to
* @property updated The last time the watchlist item was updated
* @property updatedBy Describes a user that updated the watchlist item
* @property watchlistAlias The watchlist alias
* @property watchlistItemId The id (a Guid) of the watchlist item
* @property watchlistItemType The type of the watchlist item
* @property workspaceName The name of the workspace.
*/
public data class WatchlistItemArgs(
public val created: Output? = null,
public val createdBy: Output? = null,
public val entityMapping: Output? = null,
public val isDeleted: Output? = null,
public val itemsKeyValue: Output? = null,
public val resourceGroupName: Output? = null,
public val tenantId: Output? = null,
public val updated: Output? = null,
public val updatedBy: Output? = null,
public val watchlistAlias: Output? = null,
public val watchlistItemId: Output? = null,
public val watchlistItemType: Output? = null,
public val workspaceName: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.securityinsights.WatchlistItemArgs =
com.pulumi.azurenative.securityinsights.WatchlistItemArgs.builder()
.created(created?.applyValue({ args0 -> args0 }))
.createdBy(createdBy?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.entityMapping(entityMapping?.applyValue({ args0 -> args0 }))
.isDeleted(isDeleted?.applyValue({ args0 -> args0 }))
.itemsKeyValue(itemsKeyValue?.applyValue({ args0 -> args0 }))
.resourceGroupName(resourceGroupName?.applyValue({ args0 -> args0 }))
.tenantId(tenantId?.applyValue({ args0 -> args0 }))
.updated(updated?.applyValue({ args0 -> args0 }))
.updatedBy(updatedBy?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.watchlistAlias(watchlistAlias?.applyValue({ args0 -> args0 }))
.watchlistItemId(watchlistItemId?.applyValue({ args0 -> args0 }))
.watchlistItemType(watchlistItemType?.applyValue({ args0 -> args0 }))
.workspaceName(workspaceName?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [WatchlistItemArgs].
*/
@PulumiTagMarker
public class WatchlistItemArgsBuilder internal constructor() {
private var created: Output? = null
private var createdBy: Output? = null
private var entityMapping: Output? = null
private var isDeleted: Output? = null
private var itemsKeyValue: Output? = null
private var resourceGroupName: Output? = null
private var tenantId: Output? = null
private var updated: Output? = null
private var updatedBy: Output? = null
private var watchlistAlias: Output? = null
private var watchlistItemId: Output? = null
private var watchlistItemType: Output? = null
private var workspaceName: Output? = null
/**
* @param value The time the watchlist item was created
*/
@JvmName("lydoluyevswcdllr")
public suspend fun created(`value`: Output) {
this.created = value
}
/**
* @param value Describes a user that created the watchlist item
*/
@JvmName("enrpmghtovgnwwov")
public suspend fun createdBy(`value`: Output) {
this.createdBy = value
}
/**
* @param value key-value pairs for a watchlist item entity mapping
*/
@JvmName("bvqdiebphkaaqlmx")
public suspend fun entityMapping(`value`: Output) {
this.entityMapping = value
}
/**
* @param value A flag that indicates if the watchlist item is deleted or not
*/
@JvmName("jgtygnbbpgxvbbjs")
public suspend fun isDeleted(`value`: Output) {
this.isDeleted = value
}
/**
* @param value key-value pairs for a watchlist item
*/
@JvmName("sfrmqegccwvxojjq")
public suspend fun itemsKeyValue(`value`: Output) {
this.itemsKeyValue = value
}
/**
* @param value The name of the resource group. The name is case insensitive.
*/
@JvmName("twcdcdrksibyqfme")
public suspend fun resourceGroupName(`value`: Output) {
this.resourceGroupName = value
}
/**
* @param value The tenantId to which the watchlist item belongs to
*/
@JvmName("twqoqhvdcxxanofl")
public suspend fun tenantId(`value`: Output) {
this.tenantId = value
}
/**
* @param value The last time the watchlist item was updated
*/
@JvmName("ihwjasbrtryjpinw")
public suspend fun updated(`value`: Output) {
this.updated = value
}
/**
* @param value Describes a user that updated the watchlist item
*/
@JvmName("nfsdylattafkekde")
public suspend fun updatedBy(`value`: Output) {
this.updatedBy = value
}
/**
* @param value The watchlist alias
*/
@JvmName("uxwegutvhwjfxmwo")
public suspend fun watchlistAlias(`value`: Output) {
this.watchlistAlias = value
}
/**
* @param value The id (a Guid) of the watchlist item
*/
@JvmName("qewsauwbtflwrgvt")
public suspend fun watchlistItemId(`value`: Output) {
this.watchlistItemId = value
}
/**
* @param value The type of the watchlist item
*/
@JvmName("twedalhxispkclgt")
public suspend fun watchlistItemType(`value`: Output) {
this.watchlistItemType = value
}
/**
* @param value The name of the workspace.
*/
@JvmName("vwptbodcuygwuhkg")
public suspend fun workspaceName(`value`: Output) {
this.workspaceName = value
}
/**
* @param value The time the watchlist item was created
*/
@JvmName("lkgbimnntsnofoop")
public suspend fun created(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.created = mapped
}
/**
* @param value Describes a user that created the watchlist item
*/
@JvmName("wuyajibeaqdlxpfx")
public suspend fun createdBy(`value`: WatchlistUserInfoArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.createdBy = mapped
}
/**
* @param argument Describes a user that created the watchlist item
*/
@JvmName("xfwxxksilwnhtfti")
public suspend fun createdBy(argument: suspend WatchlistUserInfoArgsBuilder.() -> Unit) {
val toBeMapped = WatchlistUserInfoArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.createdBy = mapped
}
/**
* @param value key-value pairs for a watchlist item entity mapping
*/
@JvmName("jmuimtrmnjvytpxu")
public suspend fun entityMapping(`value`: Any?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.entityMapping = mapped
}
/**
* @param value A flag that indicates if the watchlist item is deleted or not
*/
@JvmName("jtpffeptuujqihme")
public suspend fun isDeleted(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.isDeleted = mapped
}
/**
* @param value key-value pairs for a watchlist item
*/
@JvmName("bnroqjxealquttnf")
public suspend fun itemsKeyValue(`value`: Any?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.itemsKeyValue = mapped
}
/**
* @param value The name of the resource group. The name is case insensitive.
*/
@JvmName("upcbtvjivaqgnvhb")
public suspend fun resourceGroupName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.resourceGroupName = mapped
}
/**
* @param value The tenantId to which the watchlist item belongs to
*/
@JvmName("xekvlnbdthkcmuoe")
public suspend fun tenantId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.tenantId = mapped
}
/**
* @param value The last time the watchlist item was updated
*/
@JvmName("ltgirtcyxpfsmcai")
public suspend fun updated(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.updated = mapped
}
/**
* @param value Describes a user that updated the watchlist item
*/
@JvmName("jaosiaxwhrrlmjxd")
public suspend fun updatedBy(`value`: WatchlistUserInfoArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.updatedBy = mapped
}
/**
* @param argument Describes a user that updated the watchlist item
*/
@JvmName("ifnfgrwhraklbloe")
public suspend fun updatedBy(argument: suspend WatchlistUserInfoArgsBuilder.() -> Unit) {
val toBeMapped = WatchlistUserInfoArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.updatedBy = mapped
}
/**
* @param value The watchlist alias
*/
@JvmName("wchupgsvwqgemtol")
public suspend fun watchlistAlias(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.watchlistAlias = mapped
}
/**
* @param value The id (a Guid) of the watchlist item
*/
@JvmName("qlmxejhbaptwsjww")
public suspend fun watchlistItemId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.watchlistItemId = mapped
}
/**
* @param value The type of the watchlist item
*/
@JvmName("rtsbqrcnkcxbvdwo")
public suspend fun watchlistItemType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.watchlistItemType = mapped
}
/**
* @param value The name of the workspace.
*/
@JvmName("idwcppkhshsgqtcw")
public suspend fun workspaceName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.workspaceName = mapped
}
internal fun build(): WatchlistItemArgs = WatchlistItemArgs(
created = created,
createdBy = createdBy,
entityMapping = entityMapping,
isDeleted = isDeleted,
itemsKeyValue = itemsKeyValue,
resourceGroupName = resourceGroupName,
tenantId = tenantId,
updated = updated,
updatedBy = updatedBy,
watchlistAlias = watchlistAlias,
watchlistItemId = watchlistItemId,
watchlistItemType = watchlistItemType,
workspaceName = workspaceName,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy