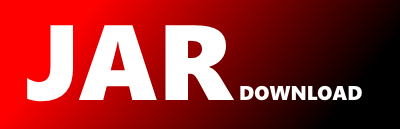
com.pulumi.azurenative.servicefabric.kotlin.ManagedCluster.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.servicefabric.kotlin
import com.pulumi.azurenative.servicefabric.kotlin.outputs.ApplicationTypeVersionsCleanupPolicyResponse
import com.pulumi.azurenative.servicefabric.kotlin.outputs.AzureActiveDirectoryResponse
import com.pulumi.azurenative.servicefabric.kotlin.outputs.ClientCertificateResponse
import com.pulumi.azurenative.servicefabric.kotlin.outputs.IPTagResponse
import com.pulumi.azurenative.servicefabric.kotlin.outputs.LoadBalancingRuleResponse
import com.pulumi.azurenative.servicefabric.kotlin.outputs.NetworkSecurityRuleResponse
import com.pulumi.azurenative.servicefabric.kotlin.outputs.ServiceEndpointResponse
import com.pulumi.azurenative.servicefabric.kotlin.outputs.SettingsSectionDescriptionResponse
import com.pulumi.azurenative.servicefabric.kotlin.outputs.SkuResponse
import com.pulumi.azurenative.servicefabric.kotlin.outputs.SubnetResponse
import com.pulumi.azurenative.servicefabric.kotlin.outputs.SystemDataResponse
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import com.pulumi.azurenative.servicefabric.kotlin.outputs.ApplicationTypeVersionsCleanupPolicyResponse.Companion.toKotlin as applicationTypeVersionsCleanupPolicyResponseToKotlin
import com.pulumi.azurenative.servicefabric.kotlin.outputs.AzureActiveDirectoryResponse.Companion.toKotlin as azureActiveDirectoryResponseToKotlin
import com.pulumi.azurenative.servicefabric.kotlin.outputs.ClientCertificateResponse.Companion.toKotlin as clientCertificateResponseToKotlin
import com.pulumi.azurenative.servicefabric.kotlin.outputs.IPTagResponse.Companion.toKotlin as iPTagResponseToKotlin
import com.pulumi.azurenative.servicefabric.kotlin.outputs.LoadBalancingRuleResponse.Companion.toKotlin as loadBalancingRuleResponseToKotlin
import com.pulumi.azurenative.servicefabric.kotlin.outputs.NetworkSecurityRuleResponse.Companion.toKotlin as networkSecurityRuleResponseToKotlin
import com.pulumi.azurenative.servicefabric.kotlin.outputs.ServiceEndpointResponse.Companion.toKotlin as serviceEndpointResponseToKotlin
import com.pulumi.azurenative.servicefabric.kotlin.outputs.SettingsSectionDescriptionResponse.Companion.toKotlin as settingsSectionDescriptionResponseToKotlin
import com.pulumi.azurenative.servicefabric.kotlin.outputs.SkuResponse.Companion.toKotlin as skuResponseToKotlin
import com.pulumi.azurenative.servicefabric.kotlin.outputs.SubnetResponse.Companion.toKotlin as subnetResponseToKotlin
import com.pulumi.azurenative.servicefabric.kotlin.outputs.SystemDataResponse.Companion.toKotlin as systemDataResponseToKotlin
/**
* Builder for [ManagedCluster].
*/
@PulumiTagMarker
public class ManagedClusterResourceBuilder internal constructor() {
public var name: String? = null
public var args: ManagedClusterArgs = ManagedClusterArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend ManagedClusterArgsBuilder.() -> Unit) {
val builder = ManagedClusterArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): ManagedCluster {
val builtJavaResource =
com.pulumi.azurenative.servicefabric.ManagedCluster(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return ManagedCluster(builtJavaResource)
}
}
/**
* The managed cluster resource
* Azure REST API version: 2023-03-01-preview. Prior API version in Azure Native 1.x: 2020-01-01-preview.
* Other available API versions: 2020-01-01-preview, 2022-01-01, 2022-10-01-preview, 2023-07-01-preview, 2023-09-01-preview, 2023-11-01-preview, 2023-12-01-preview, 2024-02-01-preview, 2024-04-01, 2024-06-01-preview.
* ## Example Usage
* ### Put a cluster with maximum parameters
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var managedCluster = new AzureNative.ServiceFabric.ManagedCluster("managedCluster", new()
* {
* AddonFeatures = new[]
* {
* AzureNative.ServiceFabric.ManagedClusterAddOnFeature.DnsService,
* AzureNative.ServiceFabric.ManagedClusterAddOnFeature.BackupRestoreService,
* AzureNative.ServiceFabric.ManagedClusterAddOnFeature.ResourceMonitorService,
* },
* AdminPassword = "{vm-password}",
* AdminUserName = "vmadmin",
* AllowRdpAccess = true,
* ApplicationTypeVersionsCleanupPolicy = new AzureNative.ServiceFabric.Inputs.ApplicationTypeVersionsCleanupPolicyArgs
* {
* MaxUnusedVersionsToKeep = 3,
* },
* AuxiliarySubnets = new[]
* {
* new AzureNative.ServiceFabric.Inputs.SubnetArgs
* {
* EnableIpv6 = true,
* Name = "testSubnet1",
* NetworkSecurityGroupId = "/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/resRg/providers/Microsoft.Network/networkSecurityGroups/sn1",
* PrivateEndpointNetworkPolicies = AzureNative.ServiceFabric.PrivateEndpointNetworkPolicies.Enabled,
* PrivateLinkServiceNetworkPolicies = AzureNative.ServiceFabric.PrivateLinkServiceNetworkPolicies.Enabled,
* },
* },
* ClientConnectionPort = 19000,
* ClusterCodeVersion = "7.1.168.9494",
* ClusterName = "myCluster",
* ClusterUpgradeMode = AzureNative.ServiceFabric.ClusterUpgradeMode.Manual,
* DnsName = "myCluster",
* EnableAutoOSUpgrade = true,
* EnableIpv6 = true,
* FabricSettings = new[]
* {
* new AzureNative.ServiceFabric.Inputs.SettingsSectionDescriptionArgs
* {
* Name = "ManagedIdentityTokenService",
* Parameters = new[]
* {
* new AzureNative.ServiceFabric.Inputs.SettingsParameterDescriptionArgs
* {
* Name = "IsEnabled",
* Value = "true",
* },
* },
* },
* },
* HttpGatewayConnectionPort = 19080,
* IpTags = new[]
* {
* new AzureNative.ServiceFabric.Inputs.IPTagArgs
* {
* IpTagType = "FirstPartyUsage",
* Tag = "SQL",
* },
* },
* LoadBalancingRules = new[]
* {
* new AzureNative.ServiceFabric.Inputs.LoadBalancingRuleArgs
* {
* BackendPort = 80,
* FrontendPort = 80,
* ProbePort = 80,
* ProbeProtocol = AzureNative.ServiceFabric.ProbeProtocol.Http,
* Protocol = "http",
* },
* new AzureNative.ServiceFabric.Inputs.LoadBalancingRuleArgs
* {
* BackendPort = 443,
* FrontendPort = 443,
* ProbePort = 443,
* ProbeProtocol = AzureNative.ServiceFabric.ProbeProtocol.Http,
* Protocol = "http",
* },
* new AzureNative.ServiceFabric.Inputs.LoadBalancingRuleArgs
* {
* BackendPort = 10000,
* FrontendPort = 10000,
* LoadDistribution = "Default",
* ProbePort = 10000,
* ProbeProtocol = AzureNative.ServiceFabric.ProbeProtocol.Http,
* Protocol = AzureNative.ServiceFabric.Protocol.Tcp,
* },
* },
* Location = "eastus",
* NetworkSecurityRules = new[]
* {
* new AzureNative.ServiceFabric.Inputs.NetworkSecurityRuleArgs
* {
* Access = AzureNative.ServiceFabric.Access.Allow,
* Description = "Test description",
* DestinationAddressPrefixes = new[]
* {
* "*",
* },
* DestinationPortRanges = new[]
* {
* "*",
* },
* Direction = AzureNative.ServiceFabric.Direction.Inbound,
* Name = "TestName",
* Priority = 1010,
* Protocol = AzureNative.ServiceFabric.NsgProtocol.Tcp,
* SourceAddressPrefixes = new[]
* {
* "*",
* },
* SourcePortRanges = new[]
* {
* "*",
* },
* },
* new AzureNative.ServiceFabric.Inputs.NetworkSecurityRuleArgs
* {
* Access = AzureNative.ServiceFabric.Access.Allow,
* DestinationAddressPrefix = "*",
* DestinationPortRange = "33500-33699",
* Direction = AzureNative.ServiceFabric.Direction.Inbound,
* Name = "AllowARM",
* Priority = 2002,
* Protocol = "*",
* SourceAddressPrefix = "AzureResourceManager",
* SourcePortRange = "*",
* },
* },
* PublicIPPrefixId = "/subscriptions/00000000-0000-0000-0000-000000000000/resourcegroups/resRg/providers/Microsoft.Network/publicIPPrefixes/myPublicIPPrefix",
* ResourceGroupName = "resRg",
* ServiceEndpoints = new[]
* {
* new AzureNative.ServiceFabric.Inputs.ServiceEndpointArgs
* {
* Locations = new[]
* {
* "eastus2",
* "usnorth",
* },
* Service = "Microsoft.Storage",
* },
* },
* Sku = new AzureNative.ServiceFabric.Inputs.SkuArgs
* {
* Name = AzureNative.ServiceFabric.SkuName.Basic,
* },
* Tags = null,
* UseCustomVnet = true,
* ZonalResiliency = true,
* ZonalUpdateMode = AzureNative.ServiceFabric.ZonalUpdateMode.Fast,
* });
* });
* ```
* ```go
* package main
* import (
* servicefabric "github.com/pulumi/pulumi-azure-native-sdk/servicefabric/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := servicefabric.NewManagedCluster(ctx, "managedCluster", &servicefabric.ManagedClusterArgs{
* AddonFeatures: pulumi.StringArray{
* pulumi.String(servicefabric.ManagedClusterAddOnFeatureDnsService),
* pulumi.String(servicefabric.ManagedClusterAddOnFeatureBackupRestoreService),
* pulumi.String(servicefabric.ManagedClusterAddOnFeatureResourceMonitorService),
* },
* AdminPassword: pulumi.String("{vm-password}"),
* AdminUserName: pulumi.String("vmadmin"),
* AllowRdpAccess: pulumi.Bool(true),
* ApplicationTypeVersionsCleanupPolicy: &servicefabric.ApplicationTypeVersionsCleanupPolicyArgs{
* MaxUnusedVersionsToKeep: pulumi.Int(3),
* },
* AuxiliarySubnets: servicefabric.SubnetArray{
* &servicefabric.SubnetArgs{
* EnableIpv6: pulumi.Bool(true),
* Name: pulumi.String("testSubnet1"),
* NetworkSecurityGroupId: pulumi.String("/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/resRg/providers/Microsoft.Network/networkSecurityGroups/sn1"),
* PrivateEndpointNetworkPolicies: pulumi.String(servicefabric.PrivateEndpointNetworkPoliciesEnabled),
* PrivateLinkServiceNetworkPolicies: pulumi.String(servicefabric.PrivateLinkServiceNetworkPoliciesEnabled),
* },
* },
* ClientConnectionPort: pulumi.Int(19000),
* ClusterCodeVersion: pulumi.String("7.1.168.9494"),
* ClusterName: pulumi.String("myCluster"),
* ClusterUpgradeMode: pulumi.String(servicefabric.ClusterUpgradeModeManual),
* DnsName: pulumi.String("myCluster"),
* EnableAutoOSUpgrade: pulumi.Bool(true),
* EnableIpv6: pulumi.Bool(true),
* FabricSettings: servicefabric.SettingsSectionDescriptionArray{
* &servicefabric.SettingsSectionDescriptionArgs{
* Name: pulumi.String("ManagedIdentityTokenService"),
* Parameters: servicefabric.SettingsParameterDescriptionArray{
* &servicefabric.SettingsParameterDescriptionArgs{
* Name: pulumi.String("IsEnabled"),
* Value: pulumi.String("true"),
* },
* },
* },
* },
* HttpGatewayConnectionPort: pulumi.Int(19080),
* IpTags: servicefabric.IPTagArray{
* &servicefabric.IPTagArgs{
* IpTagType: pulumi.String("FirstPartyUsage"),
* Tag: pulumi.String("SQL"),
* },
* },
* LoadBalancingRules: servicefabric.LoadBalancingRuleArray{
* &servicefabric.LoadBalancingRuleArgs{
* BackendPort: pulumi.Int(80),
* FrontendPort: pulumi.Int(80),
* ProbePort: pulumi.Int(80),
* ProbeProtocol: pulumi.String(servicefabric.ProbeProtocolHttp),
* Protocol: pulumi.String("http"),
* },
* &servicefabric.LoadBalancingRuleArgs{
* BackendPort: pulumi.Int(443),
* FrontendPort: pulumi.Int(443),
* ProbePort: pulumi.Int(443),
* ProbeProtocol: pulumi.String(servicefabric.ProbeProtocolHttp),
* Protocol: pulumi.String("http"),
* },
* &servicefabric.LoadBalancingRuleArgs{
* BackendPort: pulumi.Int(10000),
* FrontendPort: pulumi.Int(10000),
* LoadDistribution: pulumi.String("Default"),
* ProbePort: pulumi.Int(10000),
* ProbeProtocol: pulumi.String(servicefabric.ProbeProtocolHttp),
* Protocol: pulumi.String(servicefabric.ProtocolTcp),
* },
* },
* Location: pulumi.String("eastus"),
* NetworkSecurityRules: servicefabric.NetworkSecurityRuleArray{
* &servicefabric.NetworkSecurityRuleArgs{
* Access: pulumi.String(servicefabric.AccessAllow),
* Description: pulumi.String("Test description"),
* DestinationAddressPrefixes: pulumi.StringArray{
* pulumi.String("*"),
* },
* DestinationPortRanges: pulumi.StringArray{
* pulumi.String("*"),
* },
* Direction: pulumi.String(servicefabric.DirectionInbound),
* Name: pulumi.String("TestName"),
* Priority: pulumi.Int(1010),
* Protocol: pulumi.String(servicefabric.NsgProtocolTcp),
* SourceAddressPrefixes: pulumi.StringArray{
* pulumi.String("*"),
* },
* SourcePortRanges: pulumi.StringArray{
* pulumi.String("*"),
* },
* },
* &servicefabric.NetworkSecurityRuleArgs{
* Access: pulumi.String(servicefabric.AccessAllow),
* DestinationAddressPrefix: pulumi.String("*"),
* DestinationPortRange: pulumi.String("33500-33699"),
* Direction: pulumi.String(servicefabric.DirectionInbound),
* Name: pulumi.String("AllowARM"),
* Priority: pulumi.Int(2002),
* Protocol: pulumi.String("*"),
* SourceAddressPrefix: pulumi.String("AzureResourceManager"),
* SourcePortRange: pulumi.String("*"),
* },
* },
* PublicIPPrefixId: pulumi.String("/subscriptions/00000000-0000-0000-0000-000000000000/resourcegroups/resRg/providers/Microsoft.Network/publicIPPrefixes/myPublicIPPrefix"),
* ResourceGroupName: pulumi.String("resRg"),
* ServiceEndpoints: servicefabric.ServiceEndpointArray{
* &servicefabric.ServiceEndpointArgs{
* Locations: pulumi.StringArray{
* pulumi.String("eastus2"),
* pulumi.String("usnorth"),
* },
* Service: pulumi.String("Microsoft.Storage"),
* },
* },
* Sku: &servicefabric.SkuArgs{
* Name: pulumi.String(servicefabric.SkuNameBasic),
* },
* Tags: nil,
* UseCustomVnet: pulumi.Bool(true),
* ZonalResiliency: pulumi.Bool(true),
* ZonalUpdateMode: pulumi.String(servicefabric.ZonalUpdateModeFast),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.servicefabric.ManagedCluster;
* import com.pulumi.azurenative.servicefabric.ManagedClusterArgs;
* import com.pulumi.azurenative.servicefabric.inputs.ApplicationTypeVersionsCleanupPolicyArgs;
* import com.pulumi.azurenative.servicefabric.inputs.SubnetArgs;
* import com.pulumi.azurenative.servicefabric.inputs.SettingsSectionDescriptionArgs;
* import com.pulumi.azurenative.servicefabric.inputs.IPTagArgs;
* import com.pulumi.azurenative.servicefabric.inputs.LoadBalancingRuleArgs;
* import com.pulumi.azurenative.servicefabric.inputs.NetworkSecurityRuleArgs;
* import com.pulumi.azurenative.servicefabric.inputs.ServiceEndpointArgs;
* import com.pulumi.azurenative.servicefabric.inputs.SkuArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var managedCluster = new ManagedCluster("managedCluster", ManagedClusterArgs.builder()
* .addonFeatures(
* "DnsService",
* "BackupRestoreService",
* "ResourceMonitorService")
* .adminPassword("{vm-password}")
* .adminUserName("vmadmin")
* .allowRdpAccess(true)
* .applicationTypeVersionsCleanupPolicy(ApplicationTypeVersionsCleanupPolicyArgs.builder()
* .maxUnusedVersionsToKeep(3)
* .build())
* .auxiliarySubnets(SubnetArgs.builder()
* .enableIpv6(true)
* .name("testSubnet1")
* .networkSecurityGroupId("/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/resRg/providers/Microsoft.Network/networkSecurityGroups/sn1")
* .privateEndpointNetworkPolicies("enabled")
* .privateLinkServiceNetworkPolicies("enabled")
* .build())
* .clientConnectionPort(19000)
* .clusterCodeVersion("7.1.168.9494")
* .clusterName("myCluster")
* .clusterUpgradeMode("Manual")
* .dnsName("myCluster")
* .enableAutoOSUpgrade(true)
* .enableIpv6(true)
* .fabricSettings(SettingsSectionDescriptionArgs.builder()
* .name("ManagedIdentityTokenService")
* .parameters(SettingsParameterDescriptionArgs.builder()
* .name("IsEnabled")
* .value("true")
* .build())
* .build())
* .httpGatewayConnectionPort(19080)
* .ipTags(IPTagArgs.builder()
* .ipTagType("FirstPartyUsage")
* .tag("SQL")
* .build())
* .loadBalancingRules(
* LoadBalancingRuleArgs.builder()
* .backendPort(80)
* .frontendPort(80)
* .probePort(80)
* .probeProtocol("http")
* .protocol("http")
* .build(),
* LoadBalancingRuleArgs.builder()
* .backendPort(443)
* .frontendPort(443)
* .probePort(443)
* .probeProtocol("http")
* .protocol("http")
* .build(),
* LoadBalancingRuleArgs.builder()
* .backendPort(10000)
* .frontendPort(10000)
* .loadDistribution("Default")
* .probePort(10000)
* .probeProtocol("http")
* .protocol("tcp")
* .build())
* .location("eastus")
* .networkSecurityRules(
* NetworkSecurityRuleArgs.builder()
* .access("allow")
* .description("Test description")
* .destinationAddressPrefixes("*")
* .destinationPortRanges("*")
* .direction("inbound")
* .name("TestName")
* .priority(1010)
* .protocol("tcp")
* .sourceAddressPrefixes("*")
* .sourcePortRanges("*")
* .build(),
* NetworkSecurityRuleArgs.builder()
* .access("allow")
* .destinationAddressPrefix("*")
* .destinationPortRange("33500-33699")
* .direction("inbound")
* .name("AllowARM")
* .priority(2002)
* .protocol("*")
* .sourceAddressPrefix("AzureResourceManager")
* .sourcePortRange("*")
* .build())
* .publicIPPrefixId("/subscriptions/00000000-0000-0000-0000-000000000000/resourcegroups/resRg/providers/Microsoft.Network/publicIPPrefixes/myPublicIPPrefix")
* .resourceGroupName("resRg")
* .serviceEndpoints(ServiceEndpointArgs.builder()
* .locations(
* "eastus2",
* "usnorth")
* .service("Microsoft.Storage")
* .build())
* .sku(SkuArgs.builder()
* .name("Basic")
* .build())
* .tags()
* .useCustomVnet(true)
* .zonalResiliency(true)
* .zonalUpdateMode("Fast")
* .build());
* }
* }
* ```
* ### Put a cluster with minimum parameters
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var managedCluster = new AzureNative.ServiceFabric.ManagedCluster("managedCluster", new()
* {
* AdminPassword = "{vm-password}",
* AdminUserName = "vmadmin",
* ClusterName = "myCluster",
* ClusterUpgradeCadence = AzureNative.ServiceFabric.ClusterUpgradeCadence.Wave1,
* ClusterUpgradeMode = AzureNative.ServiceFabric.ClusterUpgradeMode.Automatic,
* DnsName = "myCluster",
* FabricSettings = new[]
* {
* new AzureNative.ServiceFabric.Inputs.SettingsSectionDescriptionArgs
* {
* Name = "ManagedIdentityTokenService",
* Parameters = new[]
* {
* new AzureNative.ServiceFabric.Inputs.SettingsParameterDescriptionArgs
* {
* Name = "IsEnabled",
* Value = "true",
* },
* },
* },
* },
* Location = "eastus",
* ResourceGroupName = "resRg",
* Sku = new AzureNative.ServiceFabric.Inputs.SkuArgs
* {
* Name = AzureNative.ServiceFabric.SkuName.Basic,
* },
* });
* });
* ```
* ```go
* package main
* import (
* servicefabric "github.com/pulumi/pulumi-azure-native-sdk/servicefabric/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := servicefabric.NewManagedCluster(ctx, "managedCluster", &servicefabric.ManagedClusterArgs{
* AdminPassword: pulumi.String("{vm-password}"),
* AdminUserName: pulumi.String("vmadmin"),
* ClusterName: pulumi.String("myCluster"),
* ClusterUpgradeCadence: pulumi.String(servicefabric.ClusterUpgradeCadenceWave1),
* ClusterUpgradeMode: pulumi.String(servicefabric.ClusterUpgradeModeAutomatic),
* DnsName: pulumi.String("myCluster"),
* FabricSettings: servicefabric.SettingsSectionDescriptionArray{
* &servicefabric.SettingsSectionDescriptionArgs{
* Name: pulumi.String("ManagedIdentityTokenService"),
* Parameters: servicefabric.SettingsParameterDescriptionArray{
* &servicefabric.SettingsParameterDescriptionArgs{
* Name: pulumi.String("IsEnabled"),
* Value: pulumi.String("true"),
* },
* },
* },
* },
* Location: pulumi.String("eastus"),
* ResourceGroupName: pulumi.String("resRg"),
* Sku: &servicefabric.SkuArgs{
* Name: pulumi.String(servicefabric.SkuNameBasic),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.servicefabric.ManagedCluster;
* import com.pulumi.azurenative.servicefabric.ManagedClusterArgs;
* import com.pulumi.azurenative.servicefabric.inputs.SettingsSectionDescriptionArgs;
* import com.pulumi.azurenative.servicefabric.inputs.SkuArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var managedCluster = new ManagedCluster("managedCluster", ManagedClusterArgs.builder()
* .adminPassword("{vm-password}")
* .adminUserName("vmadmin")
* .clusterName("myCluster")
* .clusterUpgradeCadence("Wave1")
* .clusterUpgradeMode("Automatic")
* .dnsName("myCluster")
* .fabricSettings(SettingsSectionDescriptionArgs.builder()
* .name("ManagedIdentityTokenService")
* .parameters(SettingsParameterDescriptionArgs.builder()
* .name("IsEnabled")
* .value("true")
* .build())
* .build())
* .location("eastus")
* .resourceGroupName("resRg")
* .sku(SkuArgs.builder()
* .name("Basic")
* .build())
* .build());
* }
* }
* ```
* ## Import
* An existing resource can be imported using its type token, name, and identifier, e.g.
* ```sh
* $ pulumi import azure-native:servicefabric:ManagedCluster myCluster /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.ServiceFabric/managedClusters/{clusterName}
* ```
*/
public class ManagedCluster internal constructor(
override val javaResource: com.pulumi.azurenative.servicefabric.ManagedCluster,
) : KotlinCustomResource(javaResource, ManagedClusterMapper) {
/**
* List of add-on features to enable on the cluster.
*/
public val addonFeatures: Output>?
get() = javaResource.addonFeatures().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 -> args0 })
}).orElse(null)
})
/**
* VM admin user password.
*/
public val adminPassword: Output?
get() = javaResource.adminPassword().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* VM admin user name.
*/
public val adminUserName: Output
get() = javaResource.adminUserName().applyValue({ args0 -> args0 })
/**
* Setting this to true enables RDP access to the VM. The default NSG rule opens RDP port to Internet which can be overridden with custom Network Security Rules. The default value for this setting is false.
*/
public val allowRdpAccess: Output?
get() = javaResource.allowRdpAccess().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The policy used to clean up unused versions.
*/
public val applicationTypeVersionsCleanupPolicy:
Output?
get() = javaResource.applicationTypeVersionsCleanupPolicy().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
applicationTypeVersionsCleanupPolicyResponseToKotlin(args0)
})
}).orElse(null)
})
/**
* Auxiliary subnets for the cluster.
*/
public val auxiliarySubnets: Output>?
get() = javaResource.auxiliarySubnets().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 -> args0.let({ args0 -> subnetResponseToKotlin(args0) }) })
}).orElse(null)
})
/**
* The AAD authentication settings of the cluster.
*/
public val azureActiveDirectory: Output?
get() = javaResource.azureActiveDirectory().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> azureActiveDirectoryResponseToKotlin(args0) })
}).orElse(null)
})
/**
* The port used for client connections to the cluster.
*/
public val clientConnectionPort: Output?
get() = javaResource.clientConnectionPort().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Client certificates that are allowed to manage the cluster.
*/
public val clients: Output>?
get() = javaResource.clients().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> clientCertificateResponseToKotlin(args0) })
})
}).orElse(null)
})
/**
* List of thumbprints of the cluster certificates.
*/
public val clusterCertificateThumbprints: Output>
get() = javaResource.clusterCertificateThumbprints().applyValue({ args0 ->
args0.map({ args0 ->
args0
})
})
/**
* The Service Fabric runtime version of the cluster. This property is required when **clusterUpgradeMode** is set to 'Manual'. To get list of available Service Fabric versions for new clusters use [ClusterVersion API](./ClusterVersion.md). To get the list of available version for existing clusters use **availableClusterVersions**.
*/
public val clusterCodeVersion: Output?
get() = javaResource.clusterCodeVersion().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* A service generated unique identifier for the cluster resource.
*/
public val clusterId: Output
get() = javaResource.clusterId().applyValue({ args0 -> args0 })
/**
* The current state of the cluster.
*/
public val clusterState: Output
get() = javaResource.clusterState().applyValue({ args0 -> args0 })
/**
* Indicates when new cluster runtime version upgrades will be applied after they are released. By default is Wave0. Only applies when **clusterUpgradeMode** is set to 'Automatic'.
*/
public val clusterUpgradeCadence: Output?
get() = javaResource.clusterUpgradeCadence().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The upgrade mode of the cluster when new Service Fabric runtime version is available.
*/
public val clusterUpgradeMode: Output?
get() = javaResource.clusterUpgradeMode().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The cluster dns name.
*/
public val dnsName: Output
get() = javaResource.dnsName().applyValue({ args0 -> args0 })
/**
* Setting this to true enables automatic OS upgrade for the node types that are created using any platform OS image with version 'latest'. The default value for this setting is false.
*/
public val enableAutoOSUpgrade: Output?
get() = javaResource.enableAutoOSUpgrade().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Setting this to true creates IPv6 address space for the default VNet used by the cluster. This setting cannot be changed once the cluster is created. The default value for this setting is false.
*/
public val enableIpv6: Output?
get() = javaResource.enableIpv6().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Setting this to true will link the IPv4 address as the ServicePublicIP of the IPv6 address. It can only be set to True if IPv6 is enabled on the cluster.
*/
public val enableServicePublicIP: Output?
get() = javaResource.enableServicePublicIP().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Azure resource etag.
*/
public val etag: Output
get() = javaResource.etag().applyValue({ args0 -> args0 })
/**
* The list of custom fabric settings to configure the cluster.
*/
public val fabricSettings: Output>?
get() = javaResource.fabricSettings().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
settingsSectionDescriptionResponseToKotlin(args0)
})
})
}).orElse(null)
})
/**
* The fully qualified domain name associated with the public load balancer of the cluster.
*/
public val fqdn: Output
get() = javaResource.fqdn().applyValue({ args0 -> args0 })
/**
* The port used for HTTP connections to the cluster.
*/
public val httpGatewayConnectionPort: Output?
get() = javaResource.httpGatewayConnectionPort().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The list of IP tags associated with the default public IP address of the cluster.
*/
public val ipTags: Output>?
get() = javaResource.ipTags().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> iPTagResponseToKotlin(args0) })
})
}).orElse(null)
})
/**
* The IPv4 address associated with the public load balancer of the cluster.
*/
public val ipv4Address: Output
get() = javaResource.ipv4Address().applyValue({ args0 -> args0 })
/**
* IPv6 address for the cluster if IPv6 is enabled.
*/
public val ipv6Address: Output
get() = javaResource.ipv6Address().applyValue({ args0 -> args0 })
/**
* Load balancing rules that are applied to the public load balancer of the cluster.
*/
public val loadBalancingRules: Output>?
get() = javaResource.loadBalancingRules().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
loadBalancingRuleResponseToKotlin(args0)
})
})
}).orElse(null)
})
/**
* Azure resource location.
*/
public val location: Output
get() = javaResource.location().applyValue({ args0 -> args0 })
/**
* Azure resource name.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* Custom Network Security Rules that are applied to the Virtual Network of the cluster.
*/
public val networkSecurityRules: Output>?
get() = javaResource.networkSecurityRules().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
networkSecurityRuleResponseToKotlin(args0)
})
})
}).orElse(null)
})
/**
* The provisioning state of the managed cluster resource.
*/
public val provisioningState: Output
get() = javaResource.provisioningState().applyValue({ args0 -> args0 })
/**
* Specify the resource id of a public IP prefix that the load balancer will allocate a public IP address from. Only supports IPv4.
*/
public val publicIPPrefixId: Output?
get() = javaResource.publicIPPrefixId().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Service endpoints for subnets in the cluster.
*/
public val serviceEndpoints: Output>?
get() = javaResource.serviceEndpoints().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
serviceEndpointResponseToKotlin(args0)
})
})
}).orElse(null)
})
/**
* The sku of the managed cluster
*/
public val sku: Output
get() = javaResource.sku().applyValue({ args0 ->
args0.let({ args0 ->
skuResponseToKotlin(args0)
})
})
/**
* If specified, the node types for the cluster are created in this subnet instead of the default VNet. The **networkSecurityRules** specified for the cluster are also applied to this subnet. This setting cannot be changed once the cluster is created.
*/
public val subnetId: Output?
get() = javaResource.subnetId().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* Metadata pertaining to creation and last modification of the resource.
*/
public val systemData: Output
get() = javaResource.systemData().applyValue({ args0 ->
args0.let({ args0 ->
systemDataResponseToKotlin(args0)
})
})
/**
* Azure resource tags.
*/
public val tags: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy