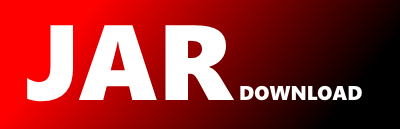
com.pulumi.azurenative.servicefabric.kotlin.inputs.ApplicationUpgradePolicyArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.servicefabric.kotlin.inputs
import com.pulumi.azurenative.servicefabric.inputs.ApplicationUpgradePolicyArgs.builder
import com.pulumi.azurenative.servicefabric.kotlin.enums.RollingUpgradeMode
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Double
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Describes the policy for a monitored application upgrade.
* @property applicationHealthPolicy Defines a health policy used to evaluate the health of an application or one of its children entities.
* @property forceRestart If true, then processes are forcefully restarted during upgrade even when the code version has not changed (the upgrade only changes configuration or data).
* @property instanceCloseDelayDuration Duration in seconds, to wait before a stateless instance is closed, to allow the active requests to drain gracefully. This would be effective when the instance is closing during the application/cluster upgrade, only for those instances which have a non-zero delay duration configured in the service description.
* @property recreateApplication Determines whether the application should be recreated on update. If value=true, the rest of the upgrade policy parameters are not allowed.
* @property rollingUpgradeMonitoringPolicy The policy used for monitoring the application upgrade
* @property upgradeMode The mode used to monitor health during a rolling upgrade. The values are Monitored, and UnmonitoredAuto.
* @property upgradeReplicaSetCheckTimeout The maximum amount of time to block processing of an upgrade domain and prevent loss of availability when there are unexpected issues. When this timeout expires, processing of the upgrade domain will proceed regardless of availability loss issues. The timeout is reset at the start of each upgrade domain. Valid values are between 0 and 42949672925 inclusive. (unsigned 32-bit integer).
*/
public data class ApplicationUpgradePolicyArgs(
public val applicationHealthPolicy: Output? = null,
public val forceRestart: Output? = null,
public val instanceCloseDelayDuration: Output? = null,
public val recreateApplication: Output? = null,
public val rollingUpgradeMonitoringPolicy: Output? = null,
public val upgradeMode: Output>? = null,
public val upgradeReplicaSetCheckTimeout: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.servicefabric.inputs.ApplicationUpgradePolicyArgs =
com.pulumi.azurenative.servicefabric.inputs.ApplicationUpgradePolicyArgs.builder()
.applicationHealthPolicy(
applicationHealthPolicy?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.forceRestart(forceRestart?.applyValue({ args0 -> args0 }))
.instanceCloseDelayDuration(instanceCloseDelayDuration?.applyValue({ args0 -> args0 }))
.recreateApplication(recreateApplication?.applyValue({ args0 -> args0 }))
.rollingUpgradeMonitoringPolicy(
rollingUpgradeMonitoringPolicy?.applyValue({ args0 ->
args0.let({ args0 -> args0.toJava() })
}),
)
.upgradeMode(
upgradeMode?.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.upgradeReplicaSetCheckTimeout(
upgradeReplicaSetCheckTimeout?.applyValue({ args0 ->
args0
}),
).build()
}
/**
* Builder for [ApplicationUpgradePolicyArgs].
*/
@PulumiTagMarker
public class ApplicationUpgradePolicyArgsBuilder internal constructor() {
private var applicationHealthPolicy: Output? = null
private var forceRestart: Output? = null
private var instanceCloseDelayDuration: Output? = null
private var recreateApplication: Output? = null
private var rollingUpgradeMonitoringPolicy: Output? = null
private var upgradeMode: Output>? = null
private var upgradeReplicaSetCheckTimeout: Output? = null
/**
* @param value Defines a health policy used to evaluate the health of an application or one of its children entities.
*/
@JvmName("pesmeqrorjeauixd")
public suspend fun applicationHealthPolicy(`value`: Output) {
this.applicationHealthPolicy = value
}
/**
* @param value If true, then processes are forcefully restarted during upgrade even when the code version has not changed (the upgrade only changes configuration or data).
*/
@JvmName("xhbxfejlcqrdfnli")
public suspend fun forceRestart(`value`: Output) {
this.forceRestart = value
}
/**
* @param value Duration in seconds, to wait before a stateless instance is closed, to allow the active requests to drain gracefully. This would be effective when the instance is closing during the application/cluster upgrade, only for those instances which have a non-zero delay duration configured in the service description.
*/
@JvmName("lefrbbracipnhhyv")
public suspend fun instanceCloseDelayDuration(`value`: Output) {
this.instanceCloseDelayDuration = value
}
/**
* @param value Determines whether the application should be recreated on update. If value=true, the rest of the upgrade policy parameters are not allowed.
*/
@JvmName("htcavwkbfodbvftc")
public suspend fun recreateApplication(`value`: Output) {
this.recreateApplication = value
}
/**
* @param value The policy used for monitoring the application upgrade
*/
@JvmName("mnsxyheuugmhkori")
public suspend fun rollingUpgradeMonitoringPolicy(`value`: Output) {
this.rollingUpgradeMonitoringPolicy = value
}
/**
* @param value The mode used to monitor health during a rolling upgrade. The values are Monitored, and UnmonitoredAuto.
*/
@JvmName("gttwndjfjbdlepkn")
public suspend fun upgradeMode(`value`: Output>) {
this.upgradeMode = value
}
/**
* @param value The maximum amount of time to block processing of an upgrade domain and prevent loss of availability when there are unexpected issues. When this timeout expires, processing of the upgrade domain will proceed regardless of availability loss issues. The timeout is reset at the start of each upgrade domain. Valid values are between 0 and 42949672925 inclusive. (unsigned 32-bit integer).
*/
@JvmName("hkfavsqfgoywblao")
public suspend fun upgradeReplicaSetCheckTimeout(`value`: Output) {
this.upgradeReplicaSetCheckTimeout = value
}
/**
* @param value Defines a health policy used to evaluate the health of an application or one of its children entities.
*/
@JvmName("nuehtoqgufmhpuak")
public suspend fun applicationHealthPolicy(`value`: ApplicationHealthPolicyArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.applicationHealthPolicy = mapped
}
/**
* @param argument Defines a health policy used to evaluate the health of an application or one of its children entities.
*/
@JvmName("yuypujydrwtoewxg")
public suspend fun applicationHealthPolicy(argument: suspend ApplicationHealthPolicyArgsBuilder.() -> Unit) {
val toBeMapped = ApplicationHealthPolicyArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.applicationHealthPolicy = mapped
}
/**
* @param value If true, then processes are forcefully restarted during upgrade even when the code version has not changed (the upgrade only changes configuration or data).
*/
@JvmName("vunnokulqonqhiuw")
public suspend fun forceRestart(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.forceRestart = mapped
}
/**
* @param value Duration in seconds, to wait before a stateless instance is closed, to allow the active requests to drain gracefully. This would be effective when the instance is closing during the application/cluster upgrade, only for those instances which have a non-zero delay duration configured in the service description.
*/
@JvmName("feenfutmuugofipc")
public suspend fun instanceCloseDelayDuration(`value`: Double?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.instanceCloseDelayDuration = mapped
}
/**
* @param value Determines whether the application should be recreated on update. If value=true, the rest of the upgrade policy parameters are not allowed.
*/
@JvmName("lvjbliadfvliijnr")
public suspend fun recreateApplication(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.recreateApplication = mapped
}
/**
* @param value The policy used for monitoring the application upgrade
*/
@JvmName("vhgipjfjfvopdnqj")
public suspend fun rollingUpgradeMonitoringPolicy(`value`: RollingUpgradeMonitoringPolicyArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.rollingUpgradeMonitoringPolicy = mapped
}
/**
* @param argument The policy used for monitoring the application upgrade
*/
@JvmName("pgqqeyvhkgdatybm")
public suspend fun rollingUpgradeMonitoringPolicy(argument: suspend RollingUpgradeMonitoringPolicyArgsBuilder.() -> Unit) {
val toBeMapped = RollingUpgradeMonitoringPolicyArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.rollingUpgradeMonitoringPolicy = mapped
}
/**
* @param value The mode used to monitor health during a rolling upgrade. The values are Monitored, and UnmonitoredAuto.
*/
@JvmName("fhmfmvsoiekfqqiy")
public suspend fun upgradeMode(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.upgradeMode = mapped
}
/**
* @param value The mode used to monitor health during a rolling upgrade. The values are Monitored, and UnmonitoredAuto.
*/
@JvmName("aruhuvxncwelflwk")
public fun upgradeMode(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.upgradeMode = mapped
}
/**
* @param value The mode used to monitor health during a rolling upgrade. The values are Monitored, and UnmonitoredAuto.
*/
@JvmName("mbmnshltdxqxxfxl")
public fun upgradeMode(`value`: RollingUpgradeMode) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.upgradeMode = mapped
}
/**
* @param value The maximum amount of time to block processing of an upgrade domain and prevent loss of availability when there are unexpected issues. When this timeout expires, processing of the upgrade domain will proceed regardless of availability loss issues. The timeout is reset at the start of each upgrade domain. Valid values are between 0 and 42949672925 inclusive. (unsigned 32-bit integer).
*/
@JvmName("cvjcxafgcxusfxxm")
public suspend fun upgradeReplicaSetCheckTimeout(`value`: Double?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.upgradeReplicaSetCheckTimeout = mapped
}
internal fun build(): ApplicationUpgradePolicyArgs = ApplicationUpgradePolicyArgs(
applicationHealthPolicy = applicationHealthPolicy,
forceRestart = forceRestart,
instanceCloseDelayDuration = instanceCloseDelayDuration,
recreateApplication = recreateApplication,
rollingUpgradeMonitoringPolicy = rollingUpgradeMonitoringPolicy,
upgradeMode = upgradeMode,
upgradeReplicaSetCheckTimeout = upgradeReplicaSetCheckTimeout,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy