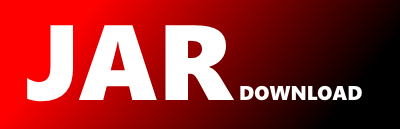
com.pulumi.azurenative.servicefabric.kotlin.inputs.SubnetArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.servicefabric.kotlin.inputs
import com.pulumi.azurenative.servicefabric.inputs.SubnetArgs.builder
import com.pulumi.azurenative.servicefabric.kotlin.enums.PrivateEndpointNetworkPolicies
import com.pulumi.azurenative.servicefabric.kotlin.enums.PrivateLinkServiceNetworkPolicies
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Describes a Subnet.
* @property enableIpv6 Indicates wether to enable Ipv6 or not. If not provided, it will take the same configuration as the cluster.
* @property name Subnet name.
* @property networkSecurityGroupId Full resource id for the network security group.
* @property privateEndpointNetworkPolicies Enable or Disable apply network policies on private end point in the subnet.
* @property privateLinkServiceNetworkPolicies Enable or Disable apply network policies on private link service in the subnet.
*/
public data class SubnetArgs(
public val enableIpv6: Output? = null,
public val name: Output,
public val networkSecurityGroupId: Output? = null,
public val privateEndpointNetworkPolicies: Output>? =
null,
public val privateLinkServiceNetworkPolicies: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.servicefabric.inputs.SubnetArgs =
com.pulumi.azurenative.servicefabric.inputs.SubnetArgs.builder()
.enableIpv6(enableIpv6?.applyValue({ args0 -> args0 }))
.name(name.applyValue({ args0 -> args0 }))
.networkSecurityGroupId(networkSecurityGroupId?.applyValue({ args0 -> args0 }))
.privateEndpointNetworkPolicies(
privateEndpointNetworkPolicies?.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 -> args0.let({ args0 -> args0.toJava() }) })
}),
)
.privateLinkServiceNetworkPolicies(
privateLinkServiceNetworkPolicies?.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 -> args0.let({ args0 -> args0.toJava() }) })
}),
).build()
}
/**
* Builder for [SubnetArgs].
*/
@PulumiTagMarker
public class SubnetArgsBuilder internal constructor() {
private var enableIpv6: Output? = null
private var name: Output? = null
private var networkSecurityGroupId: Output? = null
private var privateEndpointNetworkPolicies:
Output>? = null
private var privateLinkServiceNetworkPolicies:
Output>? = null
/**
* @param value Indicates wether to enable Ipv6 or not. If not provided, it will take the same configuration as the cluster.
*/
@JvmName("jsicvdyfsrajyevn")
public suspend fun enableIpv6(`value`: Output) {
this.enableIpv6 = value
}
/**
* @param value Subnet name.
*/
@JvmName("uxyynydbjykgvjmg")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value Full resource id for the network security group.
*/
@JvmName("bstgjqgiurymqjty")
public suspend fun networkSecurityGroupId(`value`: Output) {
this.networkSecurityGroupId = value
}
/**
* @param value Enable or Disable apply network policies on private end point in the subnet.
*/
@JvmName("pwpxxssgmvkcroys")
public suspend fun privateEndpointNetworkPolicies(`value`: Output>) {
this.privateEndpointNetworkPolicies = value
}
/**
* @param value Enable or Disable apply network policies on private link service in the subnet.
*/
@JvmName("gtfrlemmogrgtsnr")
public suspend fun privateLinkServiceNetworkPolicies(`value`: Output>) {
this.privateLinkServiceNetworkPolicies = value
}
/**
* @param value Indicates wether to enable Ipv6 or not. If not provided, it will take the same configuration as the cluster.
*/
@JvmName("ecvkycrnqikabkyf")
public suspend fun enableIpv6(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.enableIpv6 = mapped
}
/**
* @param value Subnet name.
*/
@JvmName("rvvpceveldtgygji")
public suspend fun name(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value Full resource id for the network security group.
*/
@JvmName("cqukxnvgolivevpg")
public suspend fun networkSecurityGroupId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.networkSecurityGroupId = mapped
}
/**
* @param value Enable or Disable apply network policies on private end point in the subnet.
*/
@JvmName("ybthcmbdgpmuakxa")
public suspend fun privateEndpointNetworkPolicies(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.privateEndpointNetworkPolicies = mapped
}
/**
* @param value Enable or Disable apply network policies on private end point in the subnet.
*/
@JvmName("fbsyynpbcxxvxwho")
public fun privateEndpointNetworkPolicies(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.privateEndpointNetworkPolicies = mapped
}
/**
* @param value Enable or Disable apply network policies on private end point in the subnet.
*/
@JvmName("asmwkxjsgucljopx")
public fun privateEndpointNetworkPolicies(`value`: PrivateEndpointNetworkPolicies) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.privateEndpointNetworkPolicies = mapped
}
/**
* @param value Enable or Disable apply network policies on private link service in the subnet.
*/
@JvmName("kgrdvxtlkxiriukq")
public suspend fun privateLinkServiceNetworkPolicies(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.privateLinkServiceNetworkPolicies = mapped
}
/**
* @param value Enable or Disable apply network policies on private link service in the subnet.
*/
@JvmName("yaektsbllokqxpgd")
public fun privateLinkServiceNetworkPolicies(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.privateLinkServiceNetworkPolicies = mapped
}
/**
* @param value Enable or Disable apply network policies on private link service in the subnet.
*/
@JvmName("pweurssmkpvhmlmp")
public fun privateLinkServiceNetworkPolicies(`value`: PrivateLinkServiceNetworkPolicies) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.privateLinkServiceNetworkPolicies = mapped
}
internal fun build(): SubnetArgs = SubnetArgs(
enableIpv6 = enableIpv6,
name = name ?: throw PulumiNullFieldException("name"),
networkSecurityGroupId = networkSecurityGroupId,
privateEndpointNetworkPolicies = privateEndpointNetworkPolicies,
privateLinkServiceNetworkPolicies = privateLinkServiceNetworkPolicies,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy